Android事件分发机制之View篇
We sometimes encounter some such problems in the development of android mobile phones: 1. When sliding the screen up and down, the ListView does not scroll with it? 2. Press some buttons (such as Button), and setOnclickListener related monitoring has been set, but the button does not respond? Why is this? This is the Android event distribution that I'm going to talk about in this article. Let's explain it through a very simple example, which is really very simple:
Let's take a demo first:
1. Demo's layout file
activity_main.xml文件:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center" >
<cn.ithm.eventdemo.MyButton
android:id="@+id/mybutton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
</LinearLayout>
Second, the custom Button
MyButton class file is as follows:
public class MyButton extends Button {
private static final String TAG = "MyButton";
public MyButton(Context context, AttributeSet attrs) {
super(context, attrs);
}
@Override
public boolean dispatchTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.i(TAG, "MyButton dispatchTouchEvent--ACTION_DOWN");
break;
case MotionEvent.ACTION_UP:
Log.i(TAG, "MyButton dispatchTouchEvent--ACTION_UP");
break;
default:
break;
}
return super.dispatchTouchEvent(event);
// return true;
}
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.i(TAG, "MyButton onTouchEvent--ACTION_DOWN");
break;
case MotionEvent.ACTION_UP:
Log.i(TAG, "MyButton onTouchEvent--ACTION_UP");
break;
default:
break;
}
return super.onTouchEvent(event);
}
}
3. The MainActivity.java file is as follows:
public class MainActivity extends Activity {
protected static final String TAG = "MainActivity";
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button = (Button) findViewById(R.id.mybutton);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Log.i(TAG, "MyButton onclick");
}
});
button.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View arg0, MotionEvent arg1) {
switch (arg1.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.i(TAG, "MyButton setOnTouchListener--ACTION_DOWN");
break;
case MotionEvent.ACTION_UP:
Log.i(TAG, "MyButton setOnTouchListener--ACTION_UP");
break;
default:
break;
}
return false;
// return true;
}
});
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
switch (ev.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.i(TAG, "MainActivity dispatchTouchEvent--ACTION_DOWN");
break;
case MotionEvent.ACTION_UP:
Log.i(TAG, "MainActivity dispatchTouchEvent--ACTION_UP");
break;
default:
break;
}
return super.dispatchTouchEvent(ev);
// return true;
}
}
我们准备好上面文件后,就开始研究我们的事件分发了。首先我们点击“hello word”这个按键后,LogCat里打印出如下结果:
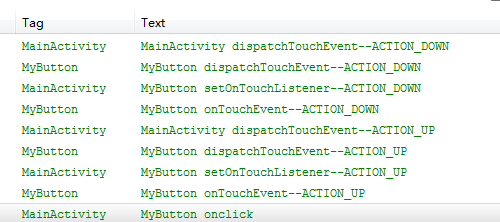
From the above results, we can see that the starting point of android event distribution is the dispatchTouchEvent method of the Activity itself, then to the dispatchTouchEvent->onTouch->onTouchEvent of the child View, and then the up event, which is the same as the down sequence, and finally executed MyButton's onClick event.
Here we probably know how the event distribution of android is performed. Next, we make a slight modification to the code, just modify the return value of dispatchTouchEvent in Activity to make it return true; then we look at the print result of LogCat:
from the above result, we are surprised that there are no subsequent touch events. implement. What's the reason for this? It's actually very simple. It's caused by the return value of the dispatchTouchEvent of the Activity. It returns true, which means that the Activity has consumed the event, and subsequent child Views cannot receive the event, so the following are not implemented.
good, very good! Next, we restore the code to its original appearance, then return true in the button.setOnTouchListener method, run it and see the print result:
we found that MyButton's onTouchEvent and onClick are not executed, and the same is true, MyButton consumes events in onTouch It's gone, so I won't post it anymore.
4. Summary
Through this simple demo example, we can summarize as follows:
1. The order of the View of Android event distribution is dispatchTouchEvent of Activity->dispatchTouchEvent of child View->OnTouch of child View->onTouchEvent of child View->onClick
2. Event distribution is executed sequentially. If the event event is consumed at a certain point, the event will not be transmitted further.
Finally, since I just learned Android not long ago, the above content is not well written, please criticize and correct me! thanks