Reprinted: https://www.baidu.com/link?url=Ff1LI1DdYiSqJFWYUWV1BRINqohtKj9_iw6BAgP4vZoOlt5LgWQO4cTdwHohwNCzq_-vL6Ch0LwbZtOFoAV_wq&wd=&eqid=9d7ebfcc0005a63800000055ac8bb22
routing system
In short, the role of django's routing system is to establish a mapping relationship between the functions that process data in views and the requested url. After the request arrives, according to the relationship entry in urls.py, find the processing method corresponding to the request, so as to return the http page data to the client
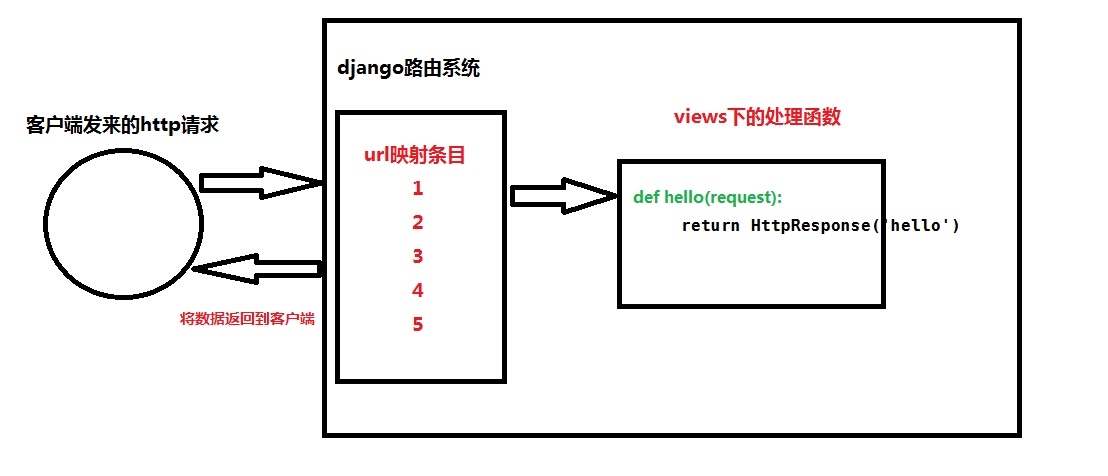
The url rule definitions in the django project are placed in the project's urls.py directory. The
default is as follows:
from django.conf.urls import url
from django.contrib import admin
urlpatterns = [
url(r'^admin/', admin.site.urls), ]
The url() function can pass 4 parameters, 2 of which are required: regex and view, and 2 optional parameters: kwargs and name. The following is a specific explanation:
- regex:
regex is a general abbreviation for regular expression, which is a syntax for matching strings or url addresses. Django takes the url address requested by the user and compares each entry in the urlpatterns list from the beginning in the urls.py file. Once a match is encountered, the view function or secondary route mapped by the entry is executed immediately. Subsequent entries will not continue to match. Therefore, the order in which url routes are written is critical!
It should be noted that regex will not try to match GET or POST parameters or domain names, for example for https://www.example.com/myapp/, regex will only try to match myapp/. For https://www.example.com/myapp/?page=3, the regex also only tries to match myapp/.
If you want to study regular expressions in depth, you can read some related books or monographs, but in practice with Django, you don't need much advanced knowledge of regular expressions.
Performance note: The regex is precompiled when the URLconf module is loaded, so its match search is so fast that you usually don't notice it.
view:
When the regular expression matches an entry, the encapsulated HttpRequest object is automatically taken as the first parameter, and the value "captured" by the regular expression is taken as the second parameter, and passed to the view specified by the entry. The captured value is passed as a positional argument in the case of a simple capture, or as a keyword argument in the case of a named capture.kwargs:
Any number of keyword arguments can be passed to the target view as a dictionary.name: Name
your URL, allowing you to refer to it explicitly anywhere in Django, especially in templates. It is equivalent to taking a global variable name for the URL. You only need to modify the value of this global variable, and the places where it is referenced throughout Django will also be changed. This is extremely old, simple, and useful design thinking, and it's everywhere.
1. The most basic mapping
The user accesses http://127.0.0.1:8000/index and then the backend uses the index() function to process
urls.py
from django.conf.urls import include, url
from django.contrib import admin from app01 import views urlpatterns = [ url(r'^admin/', admin.site.urls), url(r'^index/$', views.index), ]
1、先从创建的app下的views.py面定义处理数据的函数
2、在urls.py里导入views
3、在urlpatterns里写入一条url与处理函数的l映射关系
4、url映射一般是一条正则表达式,“^” 字符串的开始,“$“ 字符串的结束
5、当写成\^$不输入任何url时不会在返回黄页,而是返回后面函数里对应的页面。一般这一条会写在url的最后。如
2.按照顺序放置的动态路由
可以使用正则来匹配URL,将一组url使用一条映射搞定
urlpatterns = [
url(r'^host/(\d+)$', views.host),
url(r'^host_list/(\d+)/(\d+)$', views.host_list),
]
\^host/(\d+)$
相对应的url是: ”http://127.0.0.1/host/2“ (\d+)是匹配任意的数字,在分页时灵活运用。
在views.host中需要指定一个形式参数来接受(\d+)\$ 的值
def user_list(request,id): return HttpResponse(id)
\^host_list/(\d+)/(\d+)$
相对应的url是: ”http://127.0.0.1/host/8/9“,匹配到的数字会以参数的形式按照顺序传递给views里面相对应的函数
在views.host_list中需要指定两个形式参数,注意:此参数的顺序严格按照url中匹配的顺序
def user_list(request,hid,hid2): return HttpResponse(hid+hid2)
3.传参形势的路由
利用正则表达式的分组方法,将url以参数的形式传递到函数,可以不按顺序排列
urlpatterns = [
url(r'^user_list/(?P<v1>\d+)/(?P<v2>\d+)$',views.user_list),
]
(?P<v1>\d+)
正则表达式的分组,相当于一个字典, key=v1, value=\d+。 {"v1":"\d+"}
然后将此参数传递到views里对应的函数,可以不按照顺序
def user_list(request,v2,v1): return HttpResponse(v1+v2) 参数v1 = (?P<v1>\d+) 参数v2 = (?P<v2>\d+)
4.根据不同的app来分发不同的url
如果一个项目下有很多的app,那么在urls.py里面就要写巨多的urls映射关系。这样看起来很不灵活,而且杂乱无章。
我们可以根据不同的app来分类不同的url请求。
首先,在urls.py里写入urls映射条目。注意要导入include方法
from django.conf.urls import include, url
from django.contrib import admin urlpatterns = [ url(r'^app01/', include('app01.urls')), url(r'^app02/', include('app02.urls')), ]
这条关系的意思是将url为”app01/“的请求都交给app01下的urls去处理
其次,在app01下创建一个urls.py文件,用来处理请求的url,使之与views建立映射
from django.conf.urls import include, url
from app01 import views urlpatterns = [ url(r'index/$', views.index), ]
想对于url请求为: "http://127.0.0.1/app01/index/"
5.通过反射机制,为django开发一套动态的路由系统
在urls.py里定义分类正则表达式
from django.conf.urls import patterns, include, url
from django.contrib import admin from DynamicRouter.activator import process urlpatterns = patterns('', # Examples: # url(r'^$', 'DynamicRouter.views.home', name='home'), # url(r'^blog/', include('blog.urls')), url(r'^admin/', include(admin.site.urls)), ('^(?P<app>(\w+))/(?P<function>(\w+))/(?P<page>(\d+))/(?P<id>(\d+))/$',process), ('^(?P<app>(\w+))/(?P<function>(\w+))/(?P<id>(\d+))/$',process), ('^(?P<app>(\w+))/(?P<function>(\w+))/$',process), ('^(?P<app>(\w+))/$',process,{'function':'index'}), )
在同目录下创建activater.py
#!/usr/bin/env python
#coding:utf-8
from django.shortcuts import render_to_response,HttpResponse,redirect def process(request,**kwargs): '''接收所有匹配url的请求,根据请求url中的参数,通过反射动态指定view中的方法''' app = kwargs.get('app',None) function = kwargs.get('function',None) try: appObj = __import__("%s.views" %app) viewObj = getattr(appObj, 'views') funcObj = getattr(viewObj, function) #执行view.py中的函数,并获取其返回值 result = funcObj(request,kwargs) except (ImportError,AttributeError),e: #导入失败时,自定义404错误 return HttpResponse('404 Not Found') except Exception,e: #代码执行异常时,自动跳转到指定页面 return redirect('/app01/index/') return result
6.FBV和CBV
所谓FBV和CBV 是指url 和view的对应关系
- FBV function base view /url/ --> 函数
- CBV class base view /url/ -->类
上述都是FBV的方式。下面对CBV进行说明:
- urls.py
url(r'^cbv',views.CBVtest.as_view()),
- views.py
class CBVtest(View): def dispatch(self, request, *args, **kwargs): print("类似装饰器:before") result = super(CBVtest, self).dispatch(request, *args, **kwargs) print("类似装饰器:after") return result def get(self, request): # 定义get方法,get请求执行这个方法 print(request.method) return HttpResponse('cbvget') def post(self, request): # 定义post方法,post请求执行这个方法 print(request.method) return HttpResponse('cbvpost')