This article comes from the video tutorial on getting started with Shiro security framework on MOOC . The following content is the summary of the author after watching the video.
1. What is Shiro
- A powerful and flexible open source security framework under Apache
- Authentication, authorization, enterprise session management, secure encryption
- It can help to quickly complete the development of the rights management module
- The former is simple and flexible ------- the latter is complex and cumbersome
- The former can be separated from spring------the latter cannot be separated from spring
- The former has coarser authority control granularity -- the latter has finer authority control
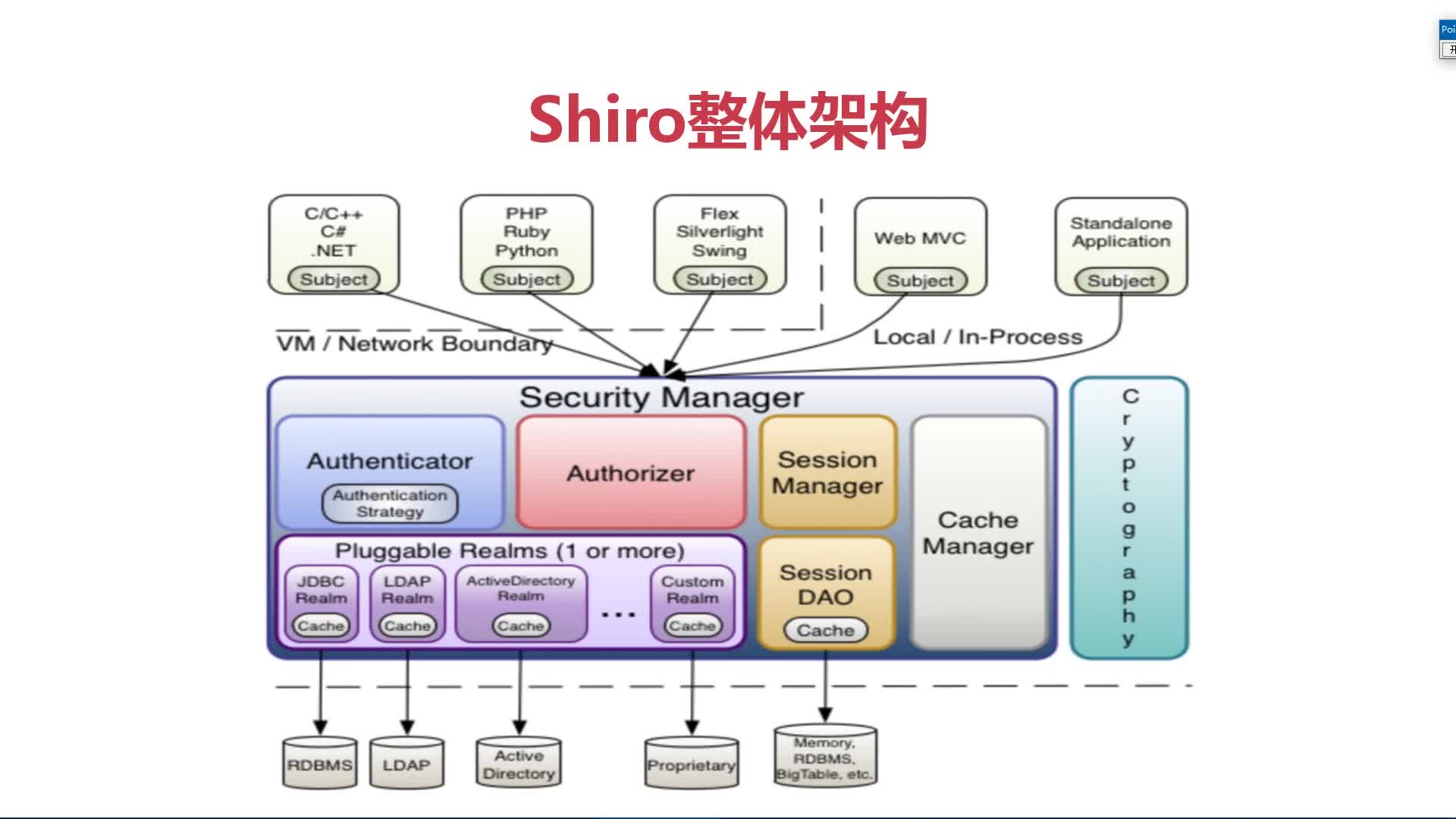
public class AuthenticationTest { SimpleAccountRealm simpleAccountRealm = new SimpleAccountRealm(); @Before public void add(){ simpleAccountRealm.addAccount("yxf", "123"); } @Test public void testAuthentication(){ //1.构建SecurityManager环境 DefaultSecurityManager defaultSecurityManager = new DefaultSecurityManager(); defaultSecurityManager.setRealm(simpleAccountRealm); // 2. The subject submits the authentication request SecurityUtils.setSecurityManager(defaultSecurityManager); Subject subject = SecurityUtils.getSubject(); UsernamePasswordToken token = new UsernamePasswordToken("yxf", "123"); subject.login(token); System.out.println("isAuthenticated:" + subject.isAuthenticated()); } }
The authentication process visible in the code above,
1. Create a Realm and add the username and password to it.
2. Build the SecurityManager environment and put Realm into the security management environment.
3. Receive the authentication request submitted by the subject (here we use a manually created token for the convenience of testing).
4. Authenticate the request.
Certification result:
1. User is logged in: The method will return true.
2. The user is not logged in: the method will return false.
2. Incorrect username: In the login method, the program will throw an exception org.apache.shiro.authc.UnknownAccountException (unknown user).
3. Incorrect password: In the login method, the program will throw an exception org.apache.shiro.authc.IncorrectCredentialsException (illegal credentials).
4. The username and password are incorrect: same as 2.
5. Shiro authorization
Generate a simple authorization code as follows
public class AuthenticationTest { SimpleAccountRealm simpleAccountRealm = new SimpleAccountRealm(); @Before public void add(){ simpleAccountRealm.addAccount("yxf", "123", "admin", "user"); } @Test public void testAuthentication(){ //1.构建SecurityManager环境 DefaultSecurityManager defaultSecurityManager = new DefaultSecurityManager(); defaultSecurityManager.setRealm(simpleAccountRealm); // 2. The subject submits the authentication request SecurityUtils.setSecurityManager(defaultSecurityManager); Subject subject = SecurityUtils.getSubject(); UsernamePasswordToken token = new UsernamePasswordToken("yxf", "123"); subject.login(token); //认证 System.out.println("isAuthenticated:" + subject.isAuthenticated()); subject.checkRole("admin"); subject.checkRole("user"); subject.checkRoles("admin", "user"); } }
In the above code, the user is authenticated first, and after the authentication is passed, the authority can be checked. You can check one permission at a time, or you can check multiple permissions at once. If the permission does not exist, the program will throw the following exception:
org.apache.shiro.authz.UnauthorizedException: Subject does not have role [user2]