1. What is a process
Process (Process) is a running activity of a program in a computer on a certain data set. It is the basic unit of resource allocation and scheduling in the system, and the basis of the operating system structure. It is not just the code of the program, but also the current activity, indicated by the value of the program counter and the contents of the processing register.
·The concept of process has two main points: First, the process is an entity. Each process has its own address space, which generally includes text region, data region and stack region. The text area stores code executed by the processor; the data area stores variables and dynamically allocated memory used during process execution; the stack area stores instructions and local variables for active procedure calls. Second, a process is a "program in execution". A program is an inanimate entity. Only when the processor gives the program life (the operating system executes it) can it become an active entity, which we call a process.
Process characteristics:
2. Process creation and termination
The following types of operations create and terminate threads.
A create process
(1) System initialization will create a new process
(2) When a running process, if the system call to create a process is executed, a new process will also be created
(3) The user makes a request to create a process
(4) When a batch job is initialized, a new thread is also created
Essentially, there is technically only one way to create a new process, that is, in an existing process, through a system call to create a new process.
In Linux, you can use the fork function to create a new process. As shown in the following code:
#include<stdio.h> #include<sys/types.h> #include<unistd.h> int main(){ pid_t ret = fork(); printf("hello proc:%d,ret = %d\n",getpid(),ret); return 0; }
Process B terminates
(1) Exit normally
(2) Error exit
(3) Fatal error
(4) Killed by other processes
3. The state transition of the process
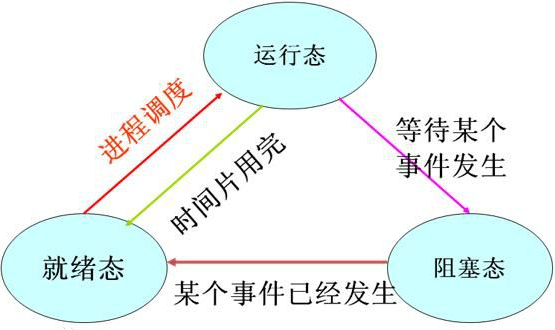
4. Process scheduling algorithm
Time slice round robin scheduling algorithm:
Each process is assigned a time period, called its time slice, which is the time the process is allowed to run. If the process is still running at the end of the time slice, the CPU will be taken away and assigned to another process. If the process blocks or ends before the time slice ends, the CPU switches immediately. All the scheduler has to do is maintain a list of ready processes, and when a process has used up its time slice, it is moved to the end of the queue.
The algorithm takes a very fair approach by having each process on the ready queue run for only one time slice at a time. If there are N processes on the ready queue, each process gets about 1/N of the processor time at a time.
· The size of the time slice has a great impact on system performance. If you choose a small time slice, it will be beneficial to short jobs, but it means that process scheduling and process context switching will be performed frequently, which will undoubtedly increase the system overhead. Conversely, if the time slice is selected too long and each process can be completed within a time slice, the time round-robin scheduling algorithm will degenerate into a first-come, first-served algorithm, which cannot meet the needs of short jobs and interactive users.
Algorithm running flow chart:
Definition of program control block:
typedef struct node { char name[20]; /*进程的名字*/ int round; /*分配CPU的时间片*/ int cputime; /*CPU执行时间*/ int needtime; /*进程执行所需要的时间*/ char state; /*进程的状态,W--就绪态,R--执行态,F--完成态*/ int count; /*记录执行的次数*/ struct node *next; /*链表指针*/ }PCB;
void RoundRun() /*时间片轮转调度算法*/ { int flag = 1; GetFirst(); while(run != NULL) { while(flag) { run->count++; run->cputime++; run->needtime--; if(run->needtime == 0) { run ->state = 'F'; InsertFinish(run); flag = 0; } else if(run->count == run->round) { run->state = 'W'; run->count = 0; InsertTime(run); flag = 0; } } flag = 1; GetFirst(); } }
对于源码的分析:
首先设置一个标志位为1,从就绪队列获取第一个节点,当获取的节点不为空和标志位为1时,这个进程所执行的次数加一,CPU执行的时间也进行自加,进程所需的时间自减,然后进行判断,当进程所需的时间为0也就是进程执行完毕时,把进程的状态设置为完成态,然后将进程插入到完成队列尾部,否则当时间片用完时,进程的状态设置为就绪态,计数器清零,将进程插入到就绪队列尾部,将标志位置为0,重新开始从就绪队列获取第一个节点。直到所有进程执行结束。
5.自己对操作系统模型的看法
操作系统是用户和计算机的接口,同时也是计算机硬件和其他软件的接口。是直接运行在机器上的最基本的系统软件,其他任何软件都必须在操作系统的支持下才能运行。所以操作系统对于计算机和学习计算机的我们是非常重要的。其中,进程又是操作系统最基本和核心的东西,我们需要了解与掌握进程,这对于我们以后更深入的学习操作体统也有很重要的意义。