@Component marks an ordinary Spring Bean; instantiate the ordinary pojo into the spring container, which is equivalent to <bean id="" class=""/> in the configuration file, and let the spring container manage it
@Controller : Annotate a controller component class;
@Service : mark a business logic component class;
@Repository : mark a Dao component;
<context:component-scan base-package=""/>
By default, all java classes annotated with @Component, @Controller, @Service, @Repository are automatically searched and treated as Spring Beans.
@Resource , using @Autoware annotation also does not require set and get methods. Because when spring instantiates an object, it injects the instance through java's reflection mechanism (that is, using Spring's annotations for automatic scanning and automatic assembly). @Resource(name="baseDao") privateBaseDao baseDao;
The @Resource annotation is used to activate dependency injection for a named resource. In a JavaEE application, the annotation is typically converted to an object bound to a JNDI context.
@RequestMapping to map URLs
The annotation @RequestMapping can be used in class definitions and method definitions.
Class definition: specifies the preliminary request mapping, relative to the root directory of the web application;
方法定义处:进一步细分请求映射,相对于类定义处的URL。如果类定义处没有使用该注解,则方法标记的URL相对于根目录而言;
@RequestMapping进行url映射,一个方法对应一个url,定义方法:在action方法上定义requestMapping
根路径+子路径
需求:为了很好的管理url,为了避免url的冲突,使用根路径+子路径
定义方法:
根路径:
在类名上边定义requestMapping

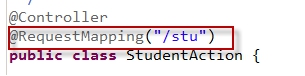
子路径:
在方法上边定义requestMapping


最终访问路径:根路径+子路径:/stu/editstudent.action
@responsebody
将java对象转json
表示该方法的返回结果直接写入HTTP response body中,而不是 “返回值通常解析为跳转路径”
@RequestBody @ResponseBody实现json数据交互
@Entity @Table
Java Persistence API定义了一种定义,可以将常规的普通Java对象(有时被称作POJO)映射到数据库。这些普通Java对象被称作Entity Bean。除了是用Java Persistence元数据将其映射到数据库外,Entity Bean与其他Java类没有任何区别。事实上,创建一个Entity Bean对象相当于新建一条记录,删除一个Entity Bean会同时从数据库中删除对应记录,修改一个Entity Bean时,容器会自动将Entity Bean的状态和数据库同步。
一面是一个例子:
@Entity
@Table(name = "sys_user")
@Cache(region = "all", usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class SysUser extends SysUserParameter {
private static final long serialVersionUID = 822330369002149147L;
@Id
@GeneratedValue
@Column(name = "id")
private Long id; // ID
@Column(name = "user_name", length = 30, nullable = false, unique = true)
private String userName; // 用户名
@Column(name = "password", length = 32, nullable = false)
private String password; // 密码
@Column(name = "real_name", length = 30, nullable = true)
private String realName; // 姓名
@Column(name = "tel", length = 15, nullable = true)
private String tel; // 手机号
@Column(name = "email", length = 30, nullable = true)
private String email; // 邮箱
@Column(name = "last_logintime")
@Temporal(TemporalType.TIMESTAMP)
private Date lastLoginTime; // 最后登录时间
@Column(name = "role", nullable = false)
private Short role; // 角色(被禁用:0,超级管理员:1,普通管理员:2,普通用户:3)
public SysUser() {
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getRealName() {
return realName;
}
public void setRealName(String realName) {
this.realName = realName;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Short getRole() {
return role;
}
public void setRole(Short role) {
this.role = role;
}
@JsonSerialize(using = DateTimeSerializer.class)
public Date getLastLoginTime() {
return lastLoginTime;
}
public void setLastLoginTime(Date lastLoginTime) {
this.lastLoginTime = lastLoginTime;
}
@Override
public boolean equals(Object obj) {
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
final SysUser other = (SysUser) obj;
return Objects.equal(this.id, other.id) && Objects.equal(this.userName, other.userName) && Objects.equal(this.password, other.password) && Objects.equal(this.realName, other.realName)
&& Objects.equal(this.tel, other.tel) && Objects.equal(this.email, other.email) && Objects.equal(this.lastLoginTime, other.lastLoginTime) && Objects.equal(this.role, other.role);
}
@Override
public int hashCode() {
return Objects.hashCode(this.id, this.userName, this.password, this.realName, this.tel, this.email, this.lastLoginTime, this.role);
}
}