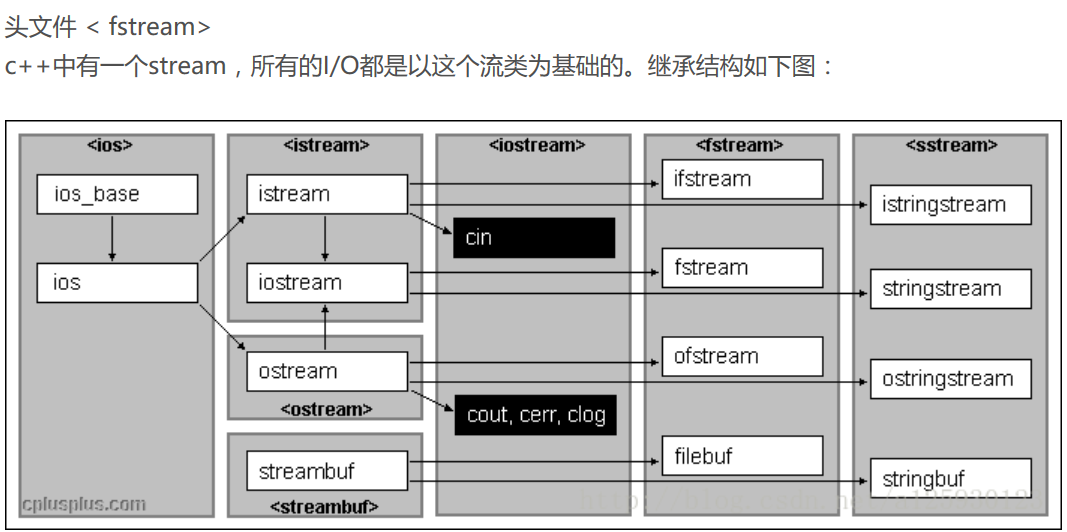
Note: "<<", inserter, input data to the stream
">>", extractor, output data from stream,
ifstream and ofstream are mainly included in the header file <fstream>. fstream can read and write the open file
ifstream <===> hard disk writes files to memory
ofstream <===> memory writes files to disk
ofstream out("out.txt"); if(out.is_open()) //is_open() returns true (1), which means the opening is successful { out<<"HELLO WORLD!"<<endl; out.close(); } HELLO WORLD! is written in the file out.txt ifstream in("out.txt"); cha buffer[200]; if(in.is_open()) { while(!in.eof()) { in.getline(buffer,100) cout<<buffer<<endl; out.close(); } }
open a file:
Close the file:
Judgment of file open status (verification of status identifier):
.bad() <===> Error in reading and writing files, such as opening for writing with r, or insufficient disk space, return true
.fail() <===> Same as above, and also returns true for data format read errors
.eof() <===> read the file to the end of the file, return true
.good() <===> is the most general, and returns false if any of the above returns true.
If the above flag is cleared, the .clear() function is called
Example complete code:
using namespace std;
int main(int argc, char **argv)
{
string lines;
ifstream infile(argv[1]);
ofstream outfile(argv[2]);
if(!infile.is_open())
{
cout << argv[ 1] << "Failed to open file" << endl;
return 0;
}
if(!outfile.is_open())
{
cout << argv[ 2] << "Failed to open file" << endl;
return 0;
}
while(getline(infile,lines))
{
if(infile.eof())
{
cout << "Failed to read file" << endl;
break;
}
istringstream strline(lines);
string tmp_string;
int i = 1;
strline>>tmp_string;
string linename = tmp_string;
while(strline>>tmp_string)
{
outfile<<"# "<<i<<" "<<linename<<" "<<i<<" "<<tmp_string<<endl;;
i++;
}
cout<<"total column is: "<<i<<endl;
}
infile.clear (); //For the portability and reusability of the code, it is best to bring this sentence to clear the flag. Some systems may have problems if they are not cleared.
infile.close();
outfile.clear (); //For the portability and reusability of the code, it is best to bring this sentence to clear the flag. Some systems may have problems if they are not cleaned up.
outfile.close();
return 0;
}