One: Mysql parameter optimization
1. View mysql parameter maximum connection
Increase the mysql parameter connection (maximum can be set to 12384)
2. View all processes and kill useless ones
3. View currently used connections
show global status like 'max_connections';
Two: Use the query cache to optimize queries
1. Most mysql servers have query caching enabled, which is one of the effective ways to improve performance, and is processed by the mysql engine. When there are many identical queries that are executed multiple times, the query results will be placed. into a cache, so that subsequent identical queries can directly access the cached results without operations.
Therefore, SQL functions like NOW() and RAND() or other such functions will not enable query caching, because the return of these functions will be volatile and volatile. So, all you need is to replace the MySQL function with a variable to enable caching .
For example, select username from table where date='2018-04-25' is better than select username from table where date =CURDATE();
2. Use the explain keyword to detect queries
Using the EXPLAIN keyword can let us know how MySQL processes SQL statements, which can help us analyze the performance bottlenecks of our query statements or table structures; the query results of EXPLAIN will also tell us how the index primary key is used, the data How the table is searched or sorted....etc. The syntax format is: EXPLAIN +SELECT statement;
Use the explain statement
Create normal index/drop index
Create a unique index / delete a unique index (note: to delete a unique index is to delete the field where the index is located)
Create primary key index/delete primary key index
mysql> alter table t_table add primary key(id);
mysql> alter table t_table drop primary key;
The second statement is only used when dropping a PRIMARY KEY index, because a table can only have one PRIMARY KEY index, so there is no need to specify an index name. If no PRIMARY KEY index is created, but the table has one or more UNIQUE indexes, MySQL will drop the first UNIQUE index.
Indexes are affected if a column is removed from the table. For multi-column indexes, if you delete one of the columns, the column will also be deleted from the index. If you drop all the columns that make up the index, the entire index will be dropped.
CREATE INDEX can add a common index or a UNIQUE index to the table.
CREATE INDEX index_name ON table_name (column_list) CREATE UNIQUE INDEX index_name ON table_name (column_list)
View the index (you can also use show keys from table name);
Non_unique: 0 if the index cannot include duplicate words. 1 if it can.
Seq_in_index: The sequence number in the index, starting from 1.
collation: how the column is stored in the index. In MySQL, there are values 'A' (ascending) or NULL (no sort).
Cardinality: An estimate of the number of unique values in the index. Updates can be made by running ANALYZE TABLE or myisamchk -a. Cardinality is counted against statistics stored as integers, so even for small tables the value does not have to be exact. The larger the cardinality, the greater the chance that MySQL will use that index when doing a union.
sub_part: If the column is only partially indexed, the number of characters indexed. NULL if the entire column is indexed.
NUll: If the column contains NULL, it contains YES. If not, the column contains NO.
Index_type: The index method used (BTREE, FULLTEXT, HASH, RTREE).
Combined index: Combined index, also known as multi-column index, is to specify multiple field attributes when creating an index. It is somewhat similar to a dictionary directory. For example, when querying the pinyin word 'guo', first look for the letter g, then look up the list of the second letter u in the search range of g, and finally look up the last letter in the range of u. word for o. For example, the combined index (a, b, c), abc are all sorted, b under any segment a is sorted, and c under any segment b is sorted

There is no breakpoint in the middle of the three index sequences in the figure below, and they all play a role. Note: Even if the order is disrupted, it does not affect, because the main function of the joint index is whether it is used all, not the order.
In this case, the id plays a role and the status does not play a role. At this time, the name is the breakpoint.
In this case, the id is the breakpoint, and neither the name nor the status has any effect.
In addition: For ordinary indexes, when using like for wildcard fuzzy query, if wildcards are used between the beginning and the end, the index is invalid.
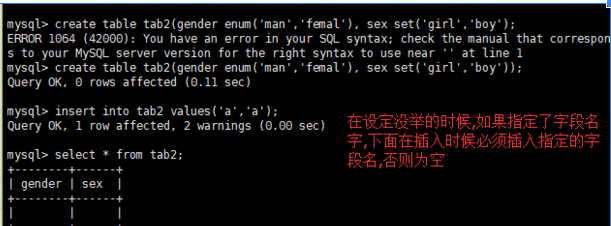
9. Try not to assign values to null,
10. Set up a fixed-length table, because a fixed-length table is easy to calculate an offset, and a fixed-length field will waste some space, because whether we use a fixed-length field or not, he has to allocate so much space. In addition, use trim to remove spaces when retrieving values.
11. The smaller the column type is set, the faster it is, which reduces the access to the hard disk. If a table has only a few columns (such as dictionary table, configuration table), then we have no reason to use INT as the primary key , it is more economical to use MEDIUMINT, SMALLINT or smaller TINYINT. If we don't need to keep track of time, using DATE is much better than DATETIME.
12. Choose the right storage engine
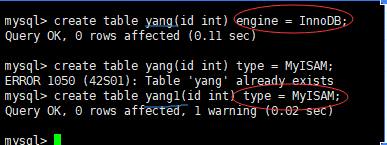
The above is the commonly used Mysql optimization. If you have other optimization methods, please leave a message!