1. What is the difference between MVVM and MVC?
(1) In MVC, M represents the Model model, V represents the view view, and C represents the controller controller; in MVVM, M represents the model model, V represents the view view, and VM represents the viewmodel;
(2) The view of MVC is that every change on the interface is an event. We only need to write a bunch of code for each event to convert the user's input into an object in the model, and the code for this conversion is controller. In short, MVC is a one-way communication, and the view and model must be connected through the controller.
MVVM's point of view is that I also define a corresponding data object for various controls in the view. Only need to modify the data object, the content displayed in the view will be automatically updated, and if any operation is performed in the view, the data object will also be Follow the update. This is called two-way data binding. The viewmodel is the model corresponding to the interface view, because the database structure often cannot directly correspond to the interface controls one-to-one, so we need to define a data object specifically corresponding to the controls on the view, and the responsibility of the viewmodel is to encapsulate the model object into a displayable object. and an interface data object that accepts input. In simple terms, viewmodel is the connector between view and model, and view and model realize two-way binding through viewmodel.
(3) Each part of MVC communicates as follows:
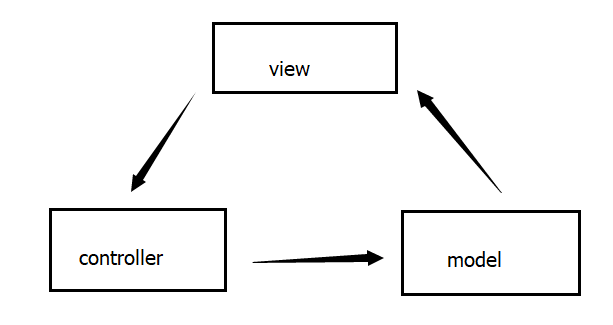
The view sends instructions to the controller. After the controller completes the business logic, it asks the model to change the state, the model sends new data to the view, and the user gets feedback.
Each part of MVVM communicates as follows:
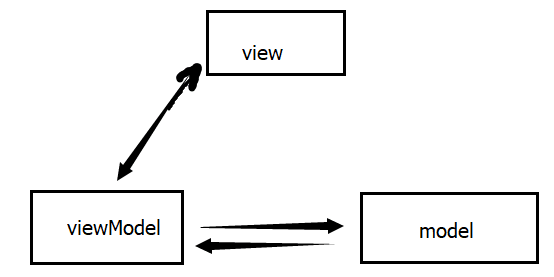
The communication between the various parts is bidirectional. The view and the model are not connected, but are passed through the viewmodel. The view is very thin and does not deploy any business logic. It is called a 'passive view', that is, there is no initiative, and the viewmodel is very thick. , where all the logic is deployed.
The main difference between MVVM and MVP is that MVVM uses two-way binding, and changes to the view are automatically reflected on the viewmodel, and vice versa. Angular, ember, and vue all adopt this pattern.
2. What is the difference between Mobx and Redux?
(1) Mobx's advantage comes from variable data and observable data; Redux's advantage comes from immutable data.
(2) Both redux and mobx do not use the traditional mvc/mvvm form, but adopt the structure of flux, action handles the request, and then dispatches the request to the store. This design is also very consistent with the concept of react one-way data flow. The flux structure used by the two is also slightly different. Mobx uses two-way binding to process data in store and view. Two-way binding will undoubtedly increase performance consumption, but mobx disables react's own refresh while two-way binding.
(3) Mobx is better in terms of framework experience, development efficiency and learning cost
3. What is the difference between angular and vue?
(1) Data binding
Both angular and vue are two-way binding, but vue enforces a single data flow between different components, which makes the data flow in the application clearer and easier to understand.
(2) Instructions and components
Directives and components are clearly separated in Vue. Instructions only encapsulate dom operations, while components represent self-sufficient independent units that have their own views and data logic.
In angular, everything is done by directives, and components are just a special kind of directive.
4、axios
(1) Helper function for processing concurrent requests (the operation is performed after both requests are completed)
axios.all(iterable),axios.spread(callback)
function getUserAccount() { return axios.get('/user/123456') } function getUserPermissions() { return axios.get('/user/123456/permissions') } axios.all([getUserAccount(), getUserPermissions()]) .then(axios.spread(function(acct, perms) { // Both requests are now complete }))
(2) Interceptor: Intercept requests or responses before they are processed by then or catch
// Add request interceptor axios.interceptors.request.use( function (config) { // what to do before sending the request return config }, function (error) { // Do something about the request error return Promise.reject(error) }); // Add a response interceptor axios.interceptors.response.use( function (response){ // What to do with the response data return response }, function (error) { // do something to the response error return Promise.reject(error) })
If you want to remove the interceptor later:
var myInterceptor = axios.interceptors.request.use(function(){}); axios.interceptors.request.eject(myInterceptor);
(3), use cancel token to cancel the request
Cancel tokens can be created using the CancelToken.source factory method:
var CancelToken = axios.CancelToken; var source = CancelToken.source(); axios.get('/user/123456', { cancelToken: source.token }).catch(function(throw) { if (axios.isCancel(throw)) { console.log('request cancel', throw.message) } else { // handle errors } }) // Cancel the request, the message parameter is optional source.cancel('Operation canceled by the user');
A cancelToken can also be created by passing an executor function to the CancelToken constructor:
var CancelToken = axiox.CancelToken; var cancel; axios.get('/user/123', { cancelToken: new CancelToken( function executor(c) { // The executor function receives a cancel as a parameter cancel = c }) }) // Cancel the request cancel();
Multiple requests can be cancelled with the same cancel token.
5、promise
Promise is to solve the problem of callback hell and is a solution to asynchronous programming. In a nutshell, it's a container for an event that will end in the future (usually the result of an asynchronous operation). Syntactically, it is an object (constructor) from which messages for asynchronous operations can be obtained. It provides a unified API, and various asynchronous operations can be handled in the same way.
The principle of promise is not difficult. It has three states: pending, fulfilled, and rejected. pending is the initial state after the object is created, it becomes fulfilled when the object is fulfilled (successful), and becomes rejected when the object is rejected (failed). And it can only change from pending to fulfilled or rejected, but not reverse or from fulfilled to rejected or rejected to fulfilled.
(1) The promise.all() method is to wait for all asynchronous operations to be executed before executing the then callback
(2)promise.race()
Race means race, so it means that as long as one asynchronous operation is completed, the then callback will be executed immediately, and other asynchronous operations that have not been completed will continue to be executed instead of stopped.
6. less, sass (reference address: https://www.w3cplus.com/css/an-introduction-to-less-and-comparison-to-sass.html )
(1) Less and sass have some commonalities in syntax: (8)
-
Mixing: class in class (introduce a defined class A into another class B, so that class B inherits all the properties of class A simply)
-
Parameter mixing: classes can be passed like function parameters
-
Nesting rules: classes are nested within classes to reduce repetitive code (nesting a selector within another selector to achieve inheritance)
-
Operation: Mathematical calculation in css (using addition, subtraction, multiplication and division to perform mathematical calculations in css, mainly used in the operation of attribute values and colors)
-
Color function: you can edit your color (function operation of color, the color will be converted into HSL color space first, and then operate at the channel level)
-
Namespace: styles are grouped so that they can be easily called (package some variables or mixed modules to better organize the reuse of css and property sets)
-
Scope: local modification style (first look for variables or mixed modules locally, if not found, it will go to the parent scope to find it until it is found, which is very similar to the scope of other programming languages)
-
js expression: use js expression to assign value in css style (you can use js expression in less or sass file to assign value)
(2) The difference between less and sass:
The main difference between them lies in the different implementation methods. Less is based on js, so less is processed on the client side; sass is based on ruby and processed on the server side.
Many developers don't choose less because less needs to rely on the js engine to output the modified css to the browser, and the js engine needs extra time to process the code. There are many ways to do this, I choose to use less only in development. Once development is complete, I copy-paste the less output into a compressor and then into a separate css file to replace the less file. Another way is to use the less app to compile and compress your less files. Both ways will be to minimize your style output, thus avoiding any problems that may arise due to user's browser not supporting js.
7、es6
(1), the let and const commands have been added
let is used to declare variables. The usage is similar to var, but the declared variables are only valid in the code block where the let command is located.
There is no variable promotion in let and const, that is to say, it can only be used after declaration, otherwise an error will be reported;
Both let and const have a 'temporary dead zone', that is, as long as there is a variable declared by the const or let command in the block-level scope, the variable declared by it will be bound to this area and will no longer be affected by external influences. This is called 'Temporary dead zone';
let and const do not allow the same variable to be declared repeatedly in the same scope;
let and const actually add block-level scope to js.
(2), the string is expanded
For example a template string:
Template strings are enhanced strings, denoted by backticks (`), which can be used as normal strings, used to define multi-line strings, or to embed variables in strings. To embed variables in the template string, you need to write the variable name in ${}.
(3), arrow function
After the arrow function is defined, the pointing of this will not change, no matter how it is called, the pointing of this will not change. because:
The this object in the function body is the object where it is defined, not the object where it is used;
It cannot be used as a constructor, that is to say, the new command cannot be used, otherwise an error will be reported;
The arguments object cannot be used. This object does not exist in the function body. If you want to use it, you can use the rest parameter instead;
The yield command cannot be used, so arrow functions cannot be used as Generator functions.
eg:
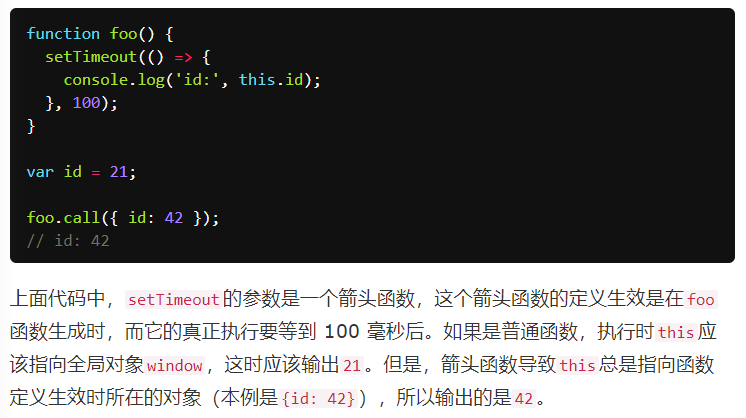
(4)promise
(5) Destructuring assignment of variables
ES6 allows to extract values from arrays and objects and assign values to variables according to a certain pattern, which is called destructuring. Essentially, this way of writing is 'pattern matching', as long as the pattern on both sides of the equal sign is the same, the variable on the left will be assigned the corresponding value.
There is an important difference between the destructuring of an object and an array. The elements of an array are arranged in order, and the value of a variable is determined by its position; while the properties of an object have no order, and the variable must have the same name as the property to get the correct value.
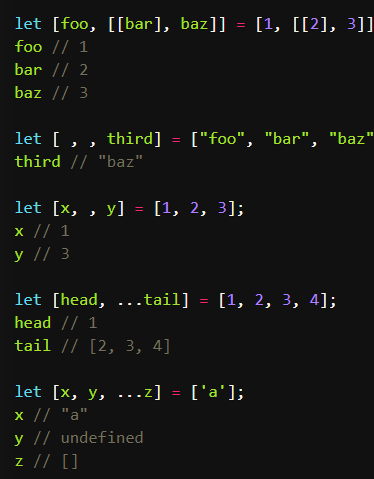
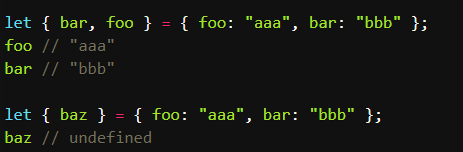
Destructuring assignment allows specifying default values:
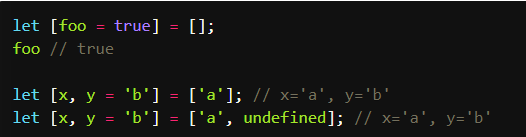
There are a lot more. . . . .
8、cookie、sessionStorage、localStorage异同点
(1) Data life cycle
When the cookie is generated, a maxAge value will be specified, which is the life cycle of the cookie. During this period, the cookie is valid. The default is that the browser is closed and invalid;
sessionStorage is available during a page session;
localStorage persists unless the data is cleared.
(2) Storage data size
The cookie is about 4k, because every http request will carry a cookie;
sessionStorage and localStorage are generally 5M or larger;
(3) Communicate with the server
The cookie is passed by the request to the server and will be carried in the http header every time. If you use the cookie to save too much data, it will cause performance problems;
sessionStorage and localStorage data are not passed by each server request, but only use the data at the time of request and do not participate in the communication with the server
(4) Ease of use
Cookie needs to encapsulate setCookie and getCookie by itself;
sessionStorage and localStorage can use the native interface, or can be repackaged to have better support for object and array
(5) Common ground
They are all stored on the browser side, which is different from the session mechanism on the server side.
9. The principle of JSONP
Ajax requests are affected by the same-origin policy, and non-cross-domain requests are not allowed, but the links in the src attribute of the script tag can access cross-domain js scripts. Using this feature, the server no longer returns data in json format, but It returns a piece of js code that calls a function, and calls it in src, thus realizing cross-domain.
10. The life cycle of vue
beforeCreate、created、beforeMount、mounted、beforeUpdate、updated、beforeDestroy、destroyed
11. What is a closure?
Closures are functions that can read variables inside other functions. For example, in js, only sub-functions inside functions can read local variables, so closures can be understood as 'functions defined inside a function'. In essence, closures are the bridge between the inside of the function and the outside of the function.
12. Prototype chain
(1), the relationship between constructor, prototype and instance
Constructors have a proptotype property;
The prototype object prototype has a constructor property, which points to the constructor to which the prototype object belongs;
Instance objects have a __proto__ property, which also points to the prototype object of the constructor. It is a non-standard property and cannot be used for programming. It is used by the browser itself.
(2) The relationship between prototype and __proto__
prototype is the property of the constructor, __proto__ is the property of the instance object. Both of these point to the same object.
(3) Prototype chain attribute search (what is a prototype chain)?
When accessing a member of an object, it will first search from the object itself. If it cannot be found in the object, it will go to the prototype object of its constructor. If it is not found, it will go to its The prototype object is searched in the prototype object of the prototype object, so that it is searched layer by layer until the prototype of the prototype object of the object is null.
13. How to understand synchronization and asynchrony?
All tasks can be divided into two types, one is synchronous task (syn) and the other is asynchronous task (asyn). Synchronous tasks refer to tasks that are queued for execution on the main thread, and the next task can only be executed after the previous task is executed; asynchronous tasks refer to tasks that do not enter the main thread, but enter the 'task queue', only the 'task' The column' informs the main thread that an asynchronous task can be executed, and the task will enter the main thread for execution.
The operating mechanism is as follows:
(1) All synchronization tasks are executed on the main thread, forming an execution stack
(2) In addition to the main thread, there is also a task queue. As long as the asynchronous task has a running result, an event is placed in the task queue.
(3) Once all the synchronization tasks in the execution stack are executed, the system will read the task queue to see what events are in it. Those corresponding asynchronous tasks will end the waiting state, enter the execution stack, and start execution
(4) Repeat the above three steps on the main thread.
14、webpack
(1) What is webpack?
Webpack can be regarded as a module packer. What she does is to analyze your project structure, find js modules and other extension languages (sass, typescript, etc.) that cannot be run directly by browsers, and convert and pack them into suitable format for browser use.
(2) How webpack works
The way wenpack works is that it treats your project as a whole, through a given main file (like index.js ), webpack will start with this file to find all your project's dependencies, process them using loaders, and finally package them as One or more browser-recognized js files
(3) Packaging principle
Package all dependencies into a bundle.js file, split the code into unit fragments and load them on demand.
(4) The core idea of webpack
Everything is a module: just as a js file can be a module, other files like css, image or html can also be considered modules, therefore, require('xxx.css'), which means we split things into smaller manageable ones fragments, so as to achieve the purpose of reuse.
Loading on demand: The traditional module packaging tool finally compiles all modules to generate a huge bundle.js file, but in a real app, the bundle.js file may be 10M or 15M in size, which may cause the application to keep loading all the time. medium status. So webpack uses many features to split the code and generate multiple bundle.js files, and load parts of the code asynchronously for on-demand loading.
15. The principle of vue two-way data binding
It is mainly achieved by rewriting the set and get functions of data through the defineProperty attribute of the object object.
16, React component life cycle process description
(1) instantiation
First instantiation:
-
getDefaultProps
-
getInitialState
-
componentWillMount
-
render
-
componentDidMount
Update after instantiation is complete:
-
getInitialState
-
componentWillMount
-
render
-
componentDidMount
(2) Existence period
State change when the component already exists:
-
componentWillReceiveProps
-
shouldComponentUpdate
-
componentWillUpdate
-
render
-
componentDidUpdate
(3) Destruction & Cleanup Period
-
componentWillUnmount
(4) Description
The declaration cycle provides a total of 10 different APIs
getDefaultProps: Acts on the component class, only called once, the returned object is used to set the default props, and for the reference value, it will be shared in the instance.
getInitialState: Acts on the instance of the component. It is called once when the instance is created to initialize the state of each instance. This.props can be accessed at this time.
componentWillMount: Called before the first render is complete, while the component's state can still be modified.
render: a required method to create a virtual DOM, this method has special rules:
-
Data can only be accessed through this.props and this.state
-
Can return null, false or any React component
-
Only one top-level component can appear (cannot return an array)
-
Can't change the state of the component
-
Cannot modify the output of the DOM
componentDidMount: Called after the real DOM is rendered. In this method, the real DOM element can be accessed through this.getDOMNode(). At this time, other libraries can be used to operate the DOM.
On the server side, the method will not be called.
componentWillReceiveProps: Called when the component receives new props, and uses it as the parameter nextProps. At this time, the component props and state can be changed.
componentWillReceiveProps: function(nextProps) { if (nextProps.bool) { this.setState({ bool: true }); } }
shouldComponentUpdate: Whether the component should render new props or state. Returning false means skipping subsequent lifecycle methods, usually not needed to avoid bugs. Appropriate optimizations can be made through this method when the bottleneck of the application occurs.
This method will not be called during the first render or after the forceUpdate method is called.
componentWillUpdate: After receiving new props or state, it is called before rendering, and changes to props or state are not allowed at this time.
componentDidUpdate: Called after rendering new props or state, and new DOM elements can be accessed at this time.
componentWillUnmount: Called before the component is removed. It can be used to do some cleanup work. All tasks added in the componentDidMount method need to be undone in this method, such as created timers or added event listeners.
17. React+Mobx core concepts
(1) state----state
State is the data that drives the application
(2)observable(value)&&@observable
Observable values can be JS primitive data types, reference types, plain objects, class instances, arrays and maps. Its modified state is exposed for use by observers.
(3) observer (observer)
The component decorated by observer will automatically re-render according to the change of the state decorated by the observable used in the component
(4) action (action)
Only in actions can the value of state in Mobx be modified.
Note: When you use the decorator pattern, this in @action is not bound to the current instance, use @action.bound to bind so that this is bound to the instance object
(5)computed
Computed values are values that can be derived from existing state or other computed values
getter: get the calculated new state and return
setters: cannot be used to directly change the value of computed properties, but they can be used for 'reverse' derivatives.