[Persistence Framework] Mybatis Introduction and Principle
When you see the frame diagram of Mybatis, you can clearly see the overall core object of Mybatis. I prefer to use my own diagram to express the entire execution process of Mybatis. As shown below:
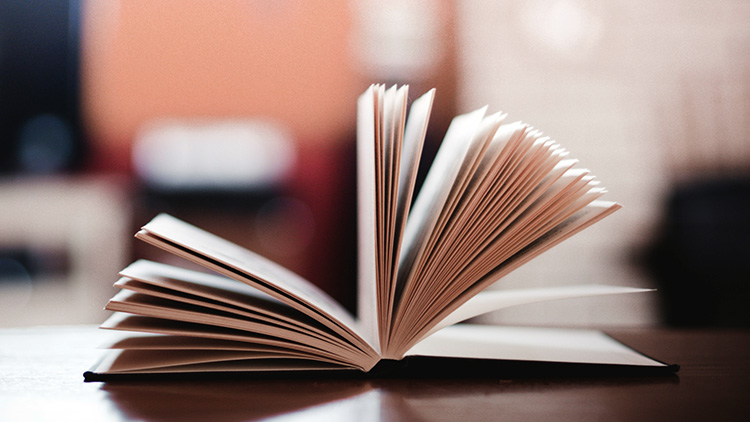
Detailed explanation of the principle:
The MyBatis application creates the SqlSessionFactory according to the XML configuration file. The SqlSessionFactory is based on the configuration. The configuration comes from two places, one is the configuration file, and the other is the annotation of the Java code to obtain a SqlSession. SqlSession contains all the methods required to execute sql. You can directly run the mapped sql statement through the SqlSession instance, complete the addition, deletion, modification, and transaction submission of data, etc., and close the SqlSession after use.
Pros and cons of MyBatis
advantage:
1. Easy to learn
mybatis itself is small and simple. Without any third-party dependencies, the simplest installation only requires two jar files + configuration of several sql mapping files. It is easy to learn and use. Through the documentation and source code, you can fully grasp its design ideas and implementation.
2. Flexible
mybatis does not impose any impact on the existing design of the application or database. SQL is written in xml, which is convenient for unified management and optimization. Basically everything we can do without using a data access framework is possible through sql, maybe more.
3. Decouple sql and program code
By providing the DAL layer, the business logic and data access logic are separated, making the system design clearer, easier to maintain, and easier to unit test. The separation of sql and code improves maintainability.
4. Provide mapping labels to support orm field relationship mapping between objects and databases
5. Provide object relationship mapping labels to support object relationship formation and maintenance
6. Provide xml tags to support writing dynamic sql.
shortcoming:
1. There is a lot of work when writing SQL statements, especially when there are many fields and many associated tables.
2. The SQL statement depends on the database, resulting in poor database portability and the database cannot be replaced.
3. The framework is still relatively simple, and the functions are still missing. Although the data binding code is simplified, the entire underlying database query actually needs to be written by itself, the workload is relatively large, and it is not easy to adapt to rapid database modification.
4. Poor secondary cache mechanism
Summarize
mybatis的优点同样是mybatis的缺点,正因为mybatis使用简单,数据的可靠性、完整性的瓶颈便更多依赖于程序员对sql的使用水平上了。sql写在xml里,虽然方便了修改、优化和统一浏览,但可读性很低,调试也非常困难,也非常受限。
mybatis没有hibernate那么强大,但是mybatis最大的优点就是简单小巧易于上手,方便浏览修改sql语句。
深入浅出MyBatis:MyBatis解析和运行原理
本篇文章是「深入浅出MyBatis:技术原理与实践」书籍的总结笔记。
上一篇介绍了反射和动态代理基础,主要是为本篇文章做个铺垫,反射使配置和灵活性大大提高,可以给很多配置设置参数,动态代理可以在运行时创建代理对象,做一些特殊的处理。
本篇会介绍MyBatis解析和运行原理,下一篇介绍插件及应用,目的是更好地编写插件,通过本篇的介绍,你会了解到:
- 构建SqlSessionFactory过程
- 映射器的动态代理
- SqlSession的4大对象
- sql执行的过程
SqlSessionFactory和SqlSession是MyBatis的核心组件,在文章 JDBC和MyBatis介绍 中有详细说明。
构建SqlSessionFactory过程
构建主要分为2步:
- 通过XMLConfigBuilder解析配置的XML文件,读出配置参数,包括基础配置XML文件和映射器XML文件;
- 使用Configuration对象创建SqlSessionFactory,SqlSessionFactory是一个接口,提供了一个默认的实现类DefaultSqlSessionFactory。
说白了,就是将我们的所有配置解析为Configuration对象,在整个生命周期内,可以通过该对象获取需要的配置。
由于插件需要频繁访问映射器的内部组成,会重点这部分,了解这块配置抽象出来的对象:
MappedStatement
它保存映射器的一个节点(select|insert|delete|update),包括配置的SQL,SQL的id、缓存信息、resultMap、parameterType、resultType等重要配置内容。
它涉及的对象比较多,一般不去修改它。
SqlSource
它是MappedStatement的一个属性,主要作用是根据参数和其他规则组装SQL,也是很复杂的,一般也不用修改它。
BoundSql
对于参数和SQL,主要反映在BoundSql类对象上,在插件中,通过它获取到当前运行的SQL和参数以及参数规则,作出适当的修改,满足特殊的要求。
BoundSql提供3个主要的属性:parameterObject、parameterMappings和sql,下面分别来介绍。
parameterObject为参数本身,可以传递简单对象、POJO、Map或@Param注解的参数:
- 传递简单对象(int、float、String等),会把参数转换为对应的类,比如int会转换为Integer;
- 如果传递的是POJO或Map,paramterObject就是传入的POJO或Map不变;
- 如果传递多个参数,没有@Param注解,parameterObject就是一个Map<String,Object>对象,类似这样的形式{“1”:p1 , “2”:p2 , “3”:p3 … “param1”:p1 , “param2”:p2 , “param3”,p3 …},所以在编写的时候可以使用#{param1}或#{1}去引用第一个参数;
- 如果传递多个参数,有@Param注解,与没有注解的类似,只是将序号的key替换为@Param指定的name;
parameterMappings,它是一个List,元素是ParameterMapping对象,这个对象会描绘sql中的参数引用,包括名称、表达式、javaType、jdbcType、typeHandler等信息。
sql,是写在映射器里面的一条sql。
有了Configuration对象,构建SqlSessionFactory就简单了:
sqlSessionFactory = new SqlSessionFactoryBuilder().bulid(inputStream);
SqlSession运行过程
映射器的动态代理
Mapper映射是通过动态代理来实现的,使用JDK动态代理返回一个代理对象,供调用者访问。
首先看看实现InvocationHandler接口的类,它是执行本代理方法的关键,可以看到,Mapper是一个接口,会生成MapperMethod对象,调用execute方法。
public class MapperProxy<T> implements InvocationHandler, Serializable {
.....
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
try {
if (Object.class.equals(method.getDeclaringClass())) {
return method.invoke(this, args);
} else if (isDefaultMethod(method)) {
return invokeDefaultMethod(proxy, method, args);
}
} catch (Throwable t) {
throw ExceptionUtil.unwrapThrowable(t);
}
final MapperMethod mapperMethod = cachedMapperMethod(method);
return mapperMethod.execute(sqlSession, args);
}
}
看下面的代码,MapperMethod采用命令模式,根据不同的sql操作,做不同的处理。
public class MapperMethod {
public Object execute(SqlSession sqlSession, Object[] args) {
Object result;
switch (command.getType()) {
case INSERT: {
Object param = method.convertArgsToSqlCommandParam(args);
result = rowCountResult(sqlSession.insert(command.getName(), param));
break;
}
case UPDATE: {
Object param = method.convertArgsToSqlCommandParam(args);
result = rowCountResult(sqlSession.update(command.getName(), param));
break;
......
}
}
}
最后看下,生成代理类的方法,就是使用JDK动态代理Proxy来创建的。
public class MapperProxyFactory<T> {
public T newInstance(SqlSession sqlSession) {
final MapperProxy<T> mapperProxy = new MapperProxy<T>(sqlSession, mapperInterface, methodCache);
return newInstance(mapperProxy);
}
@SuppressWarnings("unchecked")
protected T newInstance(MapperProxy<T> mapperProxy) {
return (T) Proxy.newProxyInstance(mapperInterface.getClassLoader(), new Class[] { mapperInterface }, mapperProxy);
}
}
总结下映射器的调用过程,返回的Mapper对象是代理对象,当调用它的某个方法时,其实是调用MapperProxy#invoke方法,而映射器的XML文件的命名空间对应的就是这个接口的全路径,会根据全路径和方法名,便能够绑定起来,定位到sql,最后会使用SqlSession接口的方法使它能够执行查询。
SqlSession下的四大对象
通过上面的分析,映射器就是一个动态代理对象,进入到了MapperMethod的execute方法,它经过简单的判断就进入了SqlSession的删除、更新、插入、选择等方法,这些方法如何执行是下面要介绍的内容。
Mapper执行的过程是通过Executor、StatementHandler、ParameterHandler和ResultHandler来完成数据库操作和结果返回的,理解他们是编写插件的关键:
- Executor:执行器,由它统一调度其他三个对象来执行对应的SQL;
- StatementHandler:使用数据库的Statement执行操作;
- ParameterHandler:用于SQL对参数的处理;
- ResultHandler:进行最后数据集的封装返回处理;
在MyBatis中存在三种执行器:
- SIMPLE:简易执行器,默认的执行器;
- REUSE:执行重用预处理语句;
- BATCH:执行重用语句和批量更新,针对批量专用的执行器;
以SimpleExecutor为例,说明执行过程
public class SimpleExecutor extends BaseExecutor {
/**
* 执行查询操作
*/
public <E> List<E> doQuery(MappedStatement ms, Object parameter, RowBounds rowBounds, ResultHandler resultHandler, BoundSql boundSql) throws SQLException {
Statement stmt = null;
try {
Configuration configuration = ms.getConfiguration();
StatementHandler handler = configuration.newStatementHandler(this, ms, parameter, rowBounds, resultHandler, boundSql);
stmt = prepareStatement(handler, ms.getStatementLog());
return handler.<E>query(stmt, resultHandler);
} finally {
closeStatement(stmt);
}
}
/**
* 初始化StatementHandler
*/
private Statement prepareStatement(StatementHandler handler, Log statementLog) throws SQLException {
Statement stmt;
Connection connection = getConnection(statementLog);
stmt = handler.prepare(connection);
handler.parameterize(stmt);
return stmt;
}
/**
* 执行查询
*/
@Override
public <E> List<E> query(Statement statement, ResultHandler resultHandler) throws SQLException {
String sql = boundSql.getSql();
statement.execute(sql);
return resultSetHandler.<E>handleResultSets(statement);
}
}
可以看到最后会委托给StatementHandler会话器进行处理,它是一个接口,实际创建的是RoutingStatementHandler对象,但它不是真实的服务对象,它是通过适配器模式找到对应的StatementHandler执行的。在MyBatis中,StatementHandler和Executor一样分为三种:SimpleStatementHandler、PreparedStatementHandler、CallableStatementHandler。
Executor会先调用StatementHandler的prepare方法预编译SQL语句,同时设置一些基本运行的参数。然后调用parameterize()方法启用ParameterHandler设置参数,完成预编译,跟着执行查询,用ResultHandler封装结果返回给调用者。
参数处理器和结果处理器比较简单,就不在此介绍了。
本文由 @luobin 原创发布精品信息聚合网–只聚合精品 让一部分人先看到未来。未经许可,禁止转载。