Introduction to Quick Sort:
Quicksort is an improvement on bubble sort. The basic idea is: divide the data to be sorted into two independent parts by --trip sorting, all the data in one part is smaller than all the data in the other part, and then quickly sort the two parts of data according to this method. , the entire sorting process can be performed recursively, so that the entire data becomes an ordered sequence
Schematic diagram of quick sort method:
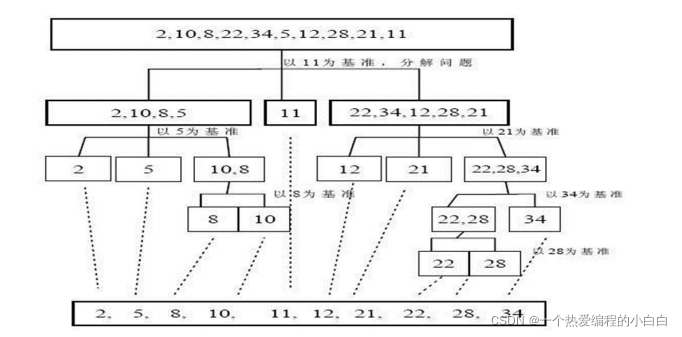
Quick sort code implementation:
import java.util.Arrays;
import java.util.Random;
public class QuickSort {
public static void main(String[] args) {
//产生一个随机数组
Random r = new Random();
int arr[] = new int[20];
for(int i=0;i<arr.length;i++){
//让随机数有正有负
if(r.nextInt()%2==0){
arr[i] =-r.nextInt(300);
}
else {
arr[i] =r.nextInt(300);
}
}
System.out.println("排序前:"+Arrays.toString(arr));
quickSort(arr, 0, arr.length - 1);
System.out.println("排序后:"+Arrays.toString(arr));
}
public static void quickSort(int[] arr, int left, int right) {
int l = left;//左下标
int r = right;//右下标
int pivot = arr[(left + right) / 2];//中轴值
int temp=0; //临时存储变量
while (l <= r) {
while (arr[l]<pivot){
l++;
}
while (arr[r]>pivot){
r--;
}
if (l>=r){
break;
}
//交换
temp=arr[r];
arr[r]=arr[l];
arr[l]=temp;
if (arr[l]==pivot){
r--;
}
if (arr[r]==pivot){
l++;
}
}
if (l==r){
l++;
r--;
}
//向左递归
if (left<r){
quickSort(arr,left,r);
}
//向右递归
if (right>l){
quickSort(arr,l,right);
}
}
}
operation result: