content
3.4 Operation - dequeue (highest priority)
3.5 Implementing a Priority Queue by Borrowing the Heap
2. See the previous section for the complete code of the heap
1. Priority queue
1.1 Concept
In many applications, we usually need to process the objects to be processed according to the priority situation, for example, the object with the highest priority is processed first, and then the object with the second highest priority is processed. The simplest example is that when playing a game on a mobile phone, if there is an incoming call, the system should give priority to the incoming call. In this case, our data structure should provide two most basic operations, one to return the highest priority object and one to add a new object. This data structure is the Priority Queue.
1.2 Internal Principles
There are many ways to implement priority queues, but the most common is to use the heap to build.
1.3 Operation - Procession
Process (take a large heap as an example):
1. First put into the array by tail insertion2. Compare the size of the value with its parent. If the value of the parent is large, the properties of the heap are satisfied, and the insertion ends.3. Otherwise, exchange the value of its parent position and repeat steps 2 and 34. Until the root node
Icon:
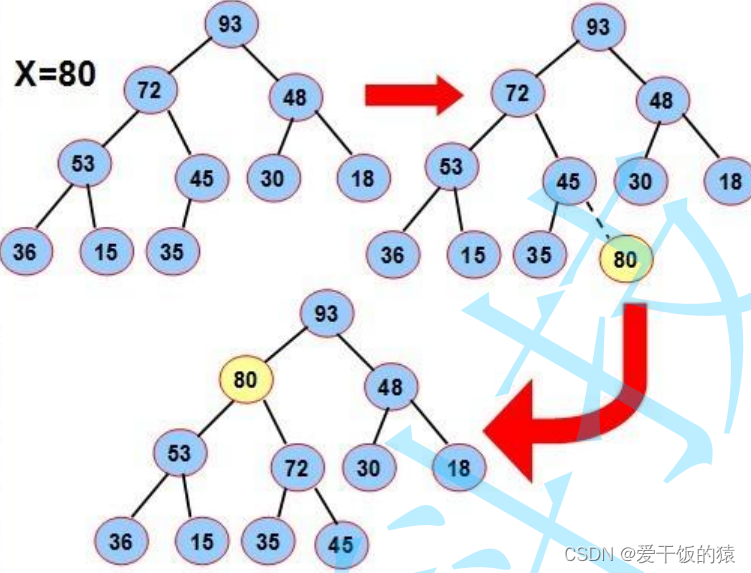
3.4 Operation - dequeue (highest priority)
In order to prevent the structure of the heap from being destroyed, the top element of the heap is not directly deleted when deleting, but the top element of the heap is replaced with the last element of the array, and then the heap is re-adjusted by downward adjustment.
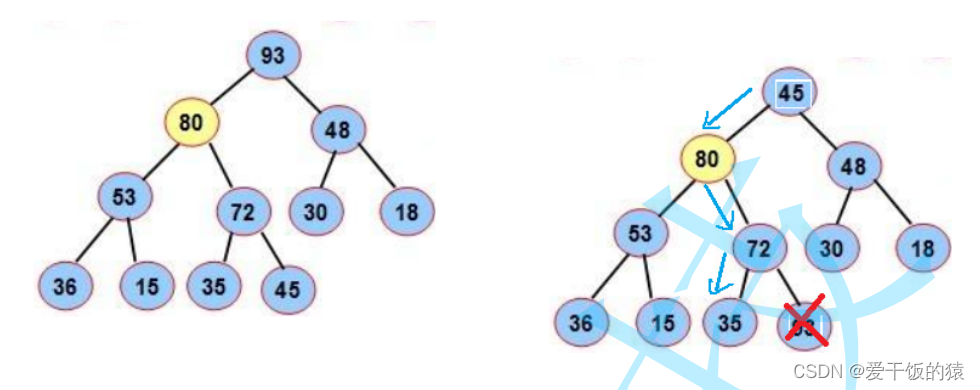
3.5 Implementing a Priority Queue by Borrowing the Heap
1. Implement an interface
public interface IQueue<E> {
// 入队
void offer(E val);
//出队
E poll();
//返回队首元素
E peek();
//判断队列是否为空
boolean isEmpty();
}
2. See the previous section for the complete code of the heap
3. Priority queue
/**
* 基于最大堆的优先级队列,值越大优先级越高
* 队首元素就是优先级最大的元素(值最大)
*/
import stack_queue.queue.IQueue;
public class PriorityQueue implements IQueue<Integer> {
MaxHeap heap = new MaxHeap();
public PriorityQueue(){
heap = new MaxHeap();
}
@Override
public void offer(Integer val) {
heap.add(val);
}
@Override
public Integer poll() {
return heap.extractMax();
}
@Override
public Integer peek() {
return heap.peekMax();
}
@Override
public boolean isEmpty() {
return heap.isEmpty();
}
}
3.6 Testing
import java.util.Comparator;
import java.util.PriorityQueue;
import java.util.Queue;
public class PriorityQueueTest {
public static void main(String[] args) {
// 通过构造方法传入比较器
// 默认是最小堆,"值"(比较器compare的返回值)越小,优先级就越高
// 当传入一个降序的比较器时,值(比较器compare的返回值,值越大,返回负数)
// Queue<Student> queue = new PriorityQueue<>(new StudentComDesc());
// Queue<Student> queue = new PriorityQueue<>(new Comparator<Student>() {
// @Override
// public int compare(Student o1, Student o2) {
// return o2.getAge() - o1.getAge();
// }
// });
Queue<Student> queue = new PriorityQueue<>(new StudentCom());
Student stu1 = new Student(18,"雷昊");
Student stu2 = new Student(20,"张超");
Student stu3 = new Student(16,"钺浦");
queue.offer(stu1);
queue.offer(stu2);
queue.offer(stu3);
while (!queue.isEmpty()){
System.out.println(queue.poll());
}
}
}
//升序(最小堆)
class StudentCom implements Comparator<Student> {
@Override
public int compare(Student o1, Student o2) {
return o1.getAge() - o2.getAge();
}
}
//降序(最大堆)
class StudentComDesc implements Comparator<Student> {
@Override
public int compare(Student o1, Student o2) {
return o2.getAge() - o1.getAge();
}
}
class Student{
private int age;
private String name;
public int getAge() {
return age;
}
public Student(int age, String name) {
this.age = age;
this.name = name;
}
@Override
public String toString() {
return "Student{" +
"age=" + age +
", name='" + name + '\'' +
'}';
}
}
The results are as follows: "Java heap & priority queue (on)"