I came across two interesting programs in C language tonight, and I also checked other people's ideas on the Internet, but I always felt that I didn't understand it very well, and the writing was not so clear. Then I spent 20 minutes typing the code. At present, the first version can meet the functions required by the title. I don’t know if there are other bugs. Let’s write it here and share it.
**The first question:** Given two integer arrays (array length <=20), find out the elements that are not common to both and put them into the third array, and output the result.
输入样例:
请输入:第一个数组的元素个数: 10
请输入第一个数组: 3 -5 2 8 0 3 5 -15 9 100
请输入第二个数组的元素个数: 11
请输入第二个数组: 6 4 8 2 6 -5 9 0 100 8 1
输出样例:
不是共有的元素有: 3 5 -15 6 4 1
Source program:
#include <stdio.h>
#include <stdlib.h>
#define maxArraySize 20
int main()
{
int firstArray[maxArraySize]; //定义第一个数组
int secondArray[maxArraySize]; //定义第二个数组
int differentElement[maxArraySize]; //定义非公有元素数组
int resultArray[maxArraySize]; //定义最终结果数组
int firstNum; //定义第一个数组元素个数
int secondNum; //定义第二个数组元素个数
int i,j; //定义循环变量
int k = 0;
int m = 0;
int flag; //定义是否公有的标志变量
printf("请输入第一个数组的元素个数:");
scanf("%d",&firstNum);
printf("请输入第一个数组:");
for(i = 0;i<firstNum;i++)
{
scanf("%d",&firstArray[i]);
}
printf("请输入第二个数组的元素个数:");
scanf("%d",&secondNum);
printf("请输入第二个数组:");
for(i = 0;i<secondNum;i++)
{
scanf("%d",&secondArray[i]);
}
for(i = 0;i<firstNum;i++)
{
flag = 1;
for(j = 0;j<secondNum;j++)
{
if(firstArray[i]==secondArray[j])
{
flag = 0;
}
}
if(flag==1)
{
differentElement[k] = firstArray[i];
k++;
}
}
for(i = 0;i<secondNum;i++)
{
flag = 1;
for(j = 0;j<firstNum;j++)
{
if(secondArray[i]==firstArray[j])
{
flag = 0;
}
}
if(flag==1)
{
differentElement[k] = secondArray[i];
k++;
}
}
for(i = 0;i<k;i++)
{
flag = 1;
for(j = i+1;j<k;j++)
{
if(differentElement[i]==differentElement[j])
{
flag = 0;
}
}
if(flag==1)
{
resultArray[m] = differentElement[i];
m++;
}
}
printf("不是公有的元素有:");
for(i = 0;i<m;i++)
{
printf("%d ",resultArray[i]);
}
return 0;
}
result:
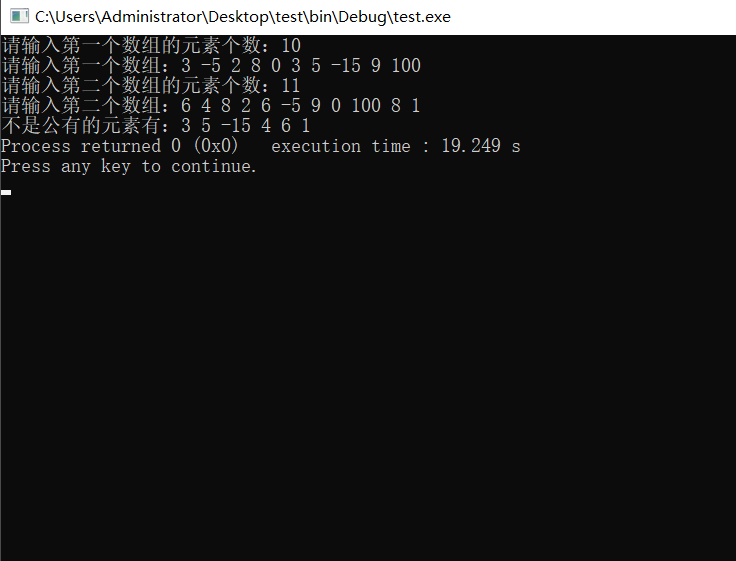
**Second question:** This question requires the implementation of a simple function that counts the number of specified numbers in an integer.
Function interface definition: int CountDigit( int number, int digit )
Where number is an integer up to a long integer, and digit is an integer in the range [0, 9]. The function CountDigit should return the number of occurrences of digit in number.
Requirement: Input two numbers in the main function, call the CountDigit function, and output the result in the main function.
输入样例1:
-21252 2
输出样例1:
Number of digit 2 in -21252: 3
输入样例2:
12345 0
输入样例2:
Number of digit 0 in 12345: 0
Source program:
#include <stdio.h>
#include <stdlib.h>
int CountDigit(int number, int digit);
int main()
{
int number;
int digit;
int times;
printf("请输入一个整数和要统计的是0-9中的哪个整数\n");
scanf("%d %d",&number, &digit);
times = CountDigit(number, digit);
printf("Number of digit %d in %d:%d",digit,number,times);
return 0;
}
int CountDigit(int number, int digit)
{
int quotient; //定义商
int remainder; //定义余数
int times = 0; //定义出现的次数
if(number<0)
{
number = -number;
}
quotient = number;
while(quotient!=0)
{
remainder = quotient%10;
quotient = quotient/10;
if(remainder==digit)
{
times++;
}
}
return times;
}
result:
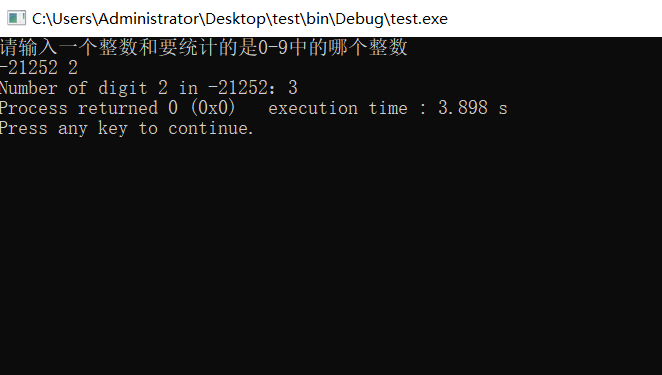
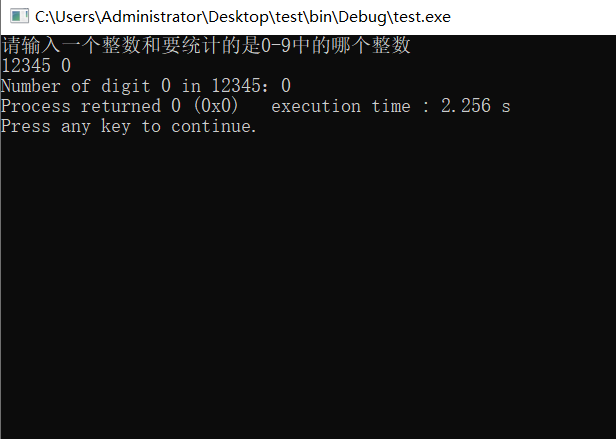