Table pot, I'm out! ! !
Watch pot, watch set, watch die, watch beauties, see what Jintian brought to Da Karma? ? ?
------------ Well, Jintian brought you three classic OJ questions about linked lists! (hahahahahaha)
1. Reverse a singly linked list: https://leetcode-cn.com/problems/reverse-linked-list/
2. The middle node of the linked list: https://leetcode-cn.com/problems/middle-of-the-linked-list/
3. Merge two ordered lists: https://leetcode-cn.com/problems/merge-two-sorted-lists/
Note: These questions are all from Likou , you can copy the link to answer the questions directly! ! !
Next, let's get into today's study! ! !
1. Reverse the singly linked list: Please reverse the linked list and return the reversed linked list.
examples:
Idea one:
Question 1: Why doesn't n3==NULL stop the loop? ? ?
Question 2: If the linked list is empty
Answer: If the linked list is empty, return NULL directly
Note: Repeated processes are generally solved with loops
Three elements of loop: initial value, iterative process, end condition
Idea-code implementation:
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* reverseList(struct ListNode* head){
if(head==NULL)
return NULL;
//初始化
struct ListNode* n1 = NULL,*n2 = head,*n3 = n2->next;
//结束条件
while(n2)
{
//迭代过程(重复过程)
n2->next = n1;
n1 = n2;
n2 = n3;
if(n3)
n3 = n3->next;
}
return n1;
}
Idea 2: head insertion method
Idea 2 code implementation:
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* reverseList(struct ListNode* head){
if(head==NULL)
{
return NULL;
}
struct ListNode *cur = head,*next = cur->next;
struct ListNode* newhead = NULL;
while(cur)
{
cur->next = newhead;
newhead = cur;
cur = next;
if(next)
next = next->next;
}
return newhead;
}
2. The intermediate node of the linked list:
Given a non-empty singly linked list whose head node is head, return the middle node of the linked list.
If there are two intermediate nodes, return the second intermediate node.
Example 1: Input [1,2,3,4,5] Output: 3
Example 2: Input [3,4,5,6] Output: 5
Ideas:
You can also see from the above idea that it is also an iterative process. The initial condition is slow, and fast points to the first node. In the iterative process, slow takes one step, fast takes two steps, and the end condition fast->next,fast Ends as long as one is empty.
Code:
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* middleNode(struct ListNode* head){
struct ListNode *slow = head,*fast = head;
while(fast && fast->next)
{
slow = slow->next;
fast = fast->next->next;
}
return slow;
}
3. Merge two ordered linked lists: Combine two ascending linked lists into a new ascending linked list and return. The new linked list is formed by splicing all the nodes of the given two linked lists.
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* mergeTwoLists(struct ListNode* list1, struct ListNode* list2){
if(list1==NULL)
{
return list2;
}
if(list2==NULL)
{
return list1;
}
struct ListNode *head = NULL,*tail = NULL;
while(list1 != NULL && list2 != NULL)
{
if(list1->val < list2->val)
{
if(tail == NULL)
{
head = tail = list1;
}
else
{
tail->next = list1;
tail = tail->next;
}
list1=list1->next;
}
else
{
if(tail == NULL)
{
head = tail = list2;
}
else
{
tail->next = list2;
tail=tail->next;
}
list2=list2->next;
}
}
if(list1)
{
tail->next = list1;
}
if(list2)
{
tail->next = list2;
}
return head;
}
Watch pots, watch sets, watch die, watch beauties are here today! ! !
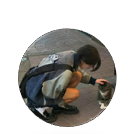
If you have any questions, feel free to add me! ! !