Method invocation mechanism principle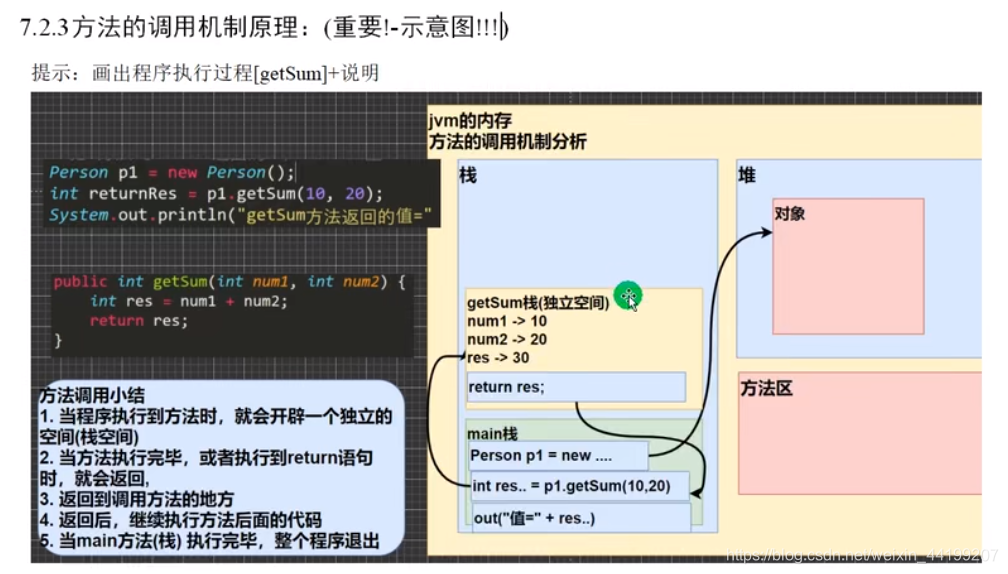
Method overloading (Overload)
In java, multiple methods with the same name are allowed in the same class.But the parameter list is required to be inconsistent
Precautions and usage details
-
method name: must be the same
-
The list of formal parameters must be different (the type or number or order of the formal parameters, at least one is different, the parameter name is not required)
-
No return type required
variable parameter
public int sum(int... nums)
int... Indicates that the variable parameter type that can be received is int, that is, it can receive multiple ints
When using variable parameters, it can be used as an array, that is, nums can be used as an array
detail:
- Arguments of variadic parameters can be arrays
- Variable parameters can be placed in the parameter list together with parameters of ordinary types,But you must ensure that the variable parameter is at the end
- There can only be one variable parameter in a parameter list
example
public class VarParameterExercise {
public static void main(String[] args) {
HspMethod hspMethod = new HspMethod();
System.out.println(hspMethod.showScore("zzn", 90, 100));
}
}
class HspMethod {
public String showScore(String name, double... scores) {
int res = 0;
for (int i = 0; i < scores.length; i++) {
res += scores[i];
}
return name +"的"+ scores.length + "门课成绩总分=" + res;
}
}
scope
-
In java programming, the main variables are properties (member variables) and local variables
-
When we talk about local variables, we generally refer to variables defined in member methods.
-
Classification of scopes in java
Global variables: that is, properties, the scope is in the entire class body
Local variables: that is, variables other than properties, the scope is { ... } in the code block where it is defined
-
Global variables (attributes) do not need to be assigned, because there are default values, you can use tips directly: the default value of the attribute is int short byte long0double float 为0.0char is\u0000boolean forfalseString isnull
Local variables must be assigned a value before they can be used
Precautions and usage details
-
Properties and local variables can have the same name, and follow the nearest access principle when accessing
-
In the same scope, such as in the same member method, two local variables cannot have the same name
-
Properties have a long life cycle, created with the creation of objects, and destroyed with the destruction of objects. Local variables, with a short life cycle, are created with the execution of its code block and destroyed with the end of the code block, that is, during a method call.
-
different scopes
Global variables/properties: can be used by this class, or other classes (by calling objects)
Local variable: can only be used in the corresponding method in this class
-
different modifiers
Global variables/properties can be modified
Local variables: no modifiers are allowed
Constructor/Constructor
The constructor, also known as the constructor, is a special method of a class whose main function is to complete theinitialization of new objects. The meaning is:When creating an object, there are multiple initialization versions to choose from
Basic syntax:
[modifier] method name (parameter list) {
method body;
}
illustrate
- Constructor modifiers can default
- Constructor has no return value
- Method name and class name must be the same
- Argument lists have the same rules as member methods
- The invocation of the constructor is done by the system
Example:
public class Constructor01 {
//编写一个main方法
public static void main(String[] args) {
//当我们new 一个对象时,直接通过构造器指定名字和年龄
Person p1 = new Person("smith", 80);
System.out.println("p1的信息如下");
System.out.println("p1对象name=" + p1.name);//smith
System.out.println("p1对象age=" + p1.age);//80
}
}
//在创建人类的对象时,就直接指定这个对象的年龄和姓名
//
class Person {
String name;
int age;
//构造器
//老韩解读
//1. 构造器没有返回值, 也不能写void
//2. 构造器的名称和类Person一样
//3. (String pName, int pAge) 是构造器形参列表,规则和成员方法一样
public Person(String pName, int pAge) {
//构造器
System.out.println("构造器被调用~~ 完成对象的属性初始化");
name = pName;
age = pAge;
}
}
Precautions and usage details
- A class can define multiple different constructors, that is, constructor overloading (it can be constructed with multiple parameters, or one or 0)
- Constructor and class name must be the same
- Constructor has no return value
- The constructor is to complete the initialization of the object,not creating objects
- When an object is created, the system automatically calls the constructor of the class
[External link image transfer failed, the source site may have anti-leech mechanism, it is recommended to save the image and upload it directly (img-k75IDkaG-1625566708358) (C:\Users\25394\AppData\Roaming\Typora\typora-user-images\ image-20210706121611716.png)]
An object is created when a class is initialized
p is the reference to the object or the name of the object,Not really an object
[External link image transfer failed, the source site may have anti-leech mechanism, it is recommended to save the image and upload it directly (img-DyumjWAi-1625566708362) (C:\Users\25394\AppData\Roaming\Typora\typora-user-images\ image-20210706124405484.png)]
this keyword
The java virtual machine assigns this to each object, and this represents the current object
[External link image transfer failed, the source site may have anti-leech mechanism, it is recommended to save the image and upload it directly (img-e0SBGhWh-1625566708364) (C:\Users\25394\AppData\Roaming\Typora\typora-user-images\ image-20210706132454881.png)]
This summary: Simply put, which object is called, this represents which object
Code:
public class This01 {
//编写一个main方法
public static void main(String[] args) {
Dog dog1 = new Dog("大壮", 3);
System.out.println("dog1的hashcode=" + dog1.hashCode());
//dog1调用了 info()方法
dog1.info();
System.out.println("============");
Dog dog2 = new Dog("大黄", 2);
System.out.println("dog2的hashcode=" + dog2.hashCode());
dog2.info();
}
}
class Dog{
//类
String name;
int age;
// public Dog(String dName, int dAge){//构造器
// name = dName;
// age = dAge;
// }
//如果我们构造器的形参,能够直接写成属性名,就更好了
//但是出现了一个问题,根据变量的作用域原则
//构造器的name 是局部变量,而不是属性
//构造器的age 是局部变量,而不是属性
//==> 引出this关键字来解决
public Dog(String name, int age){
//构造器
//this.name 就是当前对象的属性name
this.name = name;
//this.age 就是当前对象的属性age
this.age = age;
System.out.println("this.hashCode=" + this.hashCode());
}
public void info(){
//成员方法,输出属性x信息
System.out.println("this.hashCode=" + this.hashCode());//这里的this就是谁调用info方法的那个对象
System.out.println(name + "\t" + age + "\t");
}
}
Precautions and usage details
-
The this keyword can be used to access properties, methods, and constructors of this class
-
this is used to distinguish properties and local variables of the current class
-
The syntax for accessing member methods, this. method name (parameter list)
-
Access constructor syntax: this (parameter list);Note that it can only be used in the constructor
If accessing the constructor, it must be placed in the first statement
-
this cannot be used outside a class definition, only within a class-defined method