Thread 线程生命周期 常用方法 java
生命周期
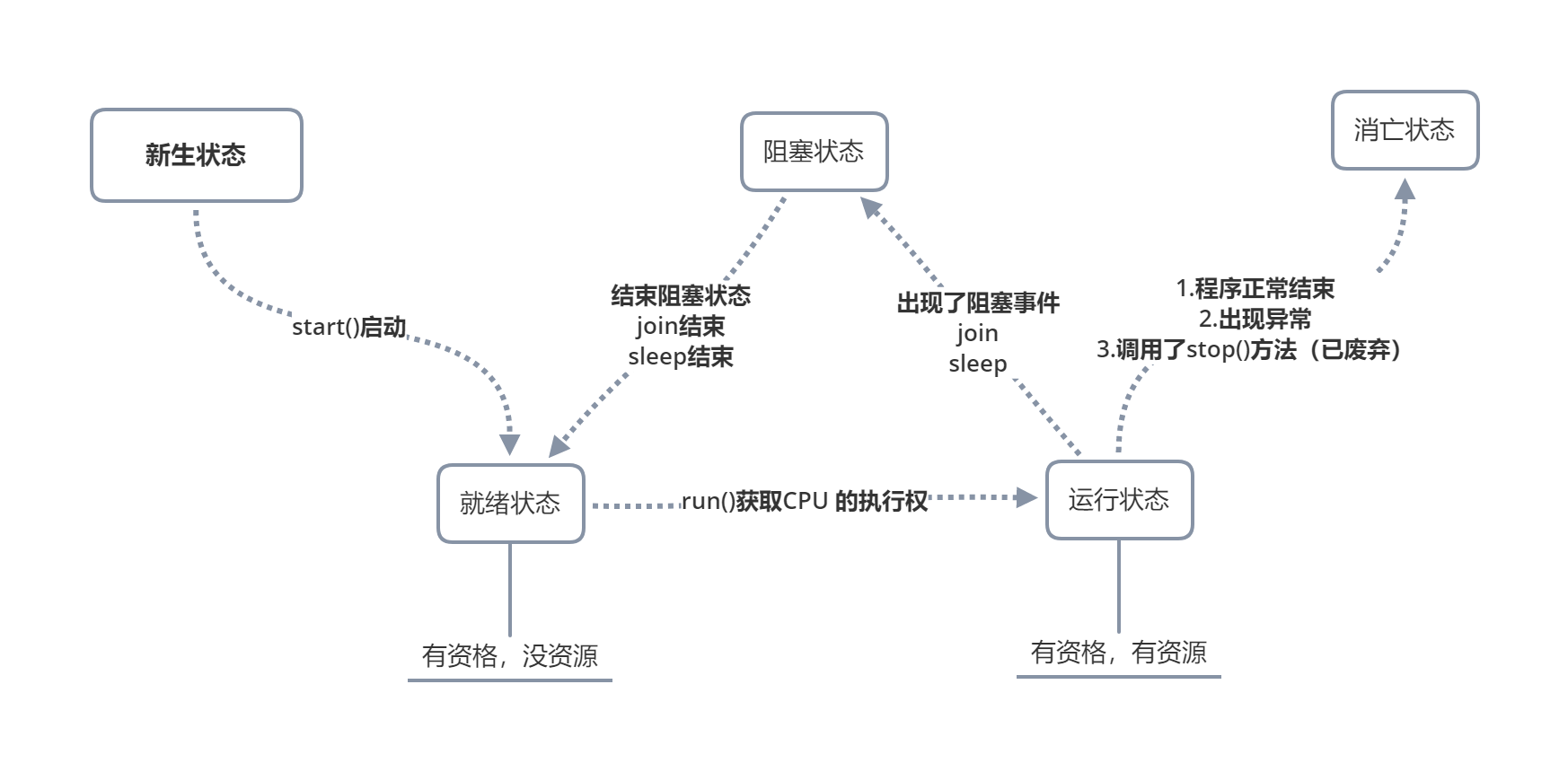
线程生命周期
运行
start()
: 启动当前线程,表面上调用start方法,实际在调用线程里面的run方法run()
: 线程类 继承 Thread类 或者 实现Runnable接口的时候,都要重新实现这个run方法,run方法里面是线程要执行的内容
命名
-
setName()
设置线程名字 -
getName()
获取线程名字 -
Thread.currentThread()
Thread类中一个静态方法:获取当前正在执行的线程 -
public Thread(String name) { init(null, null, name, 0);}
调用父类Thread有参构造器
public class CThread extends Thread {
public CThread(String name) {
super(name);//调用父类的有参构造器
}
public CThread() {
}
@Override
public void run() {
//子线程输出
System.out.println(this.getName() + "---this is thread---");
}
}
public class Test {
public static void main(String[] args) {
//Thread.currentThread()作用获取当前正在执行的线程,给main方法这个主线程设置名字:
Thread.currentThread().setName("主线程");
System.out.println(Thread.currentThread().getName() + "---1---");
CThread tt = new CThread();
tt.setName("子线程");
tt.start();
CThread tt2 = new CThread("子线程2");
tt2.start();
System.out.println(Thread.currentThread().getName() + "---2---");
}
}
主线程---1---
主线程---2---
子线程---this is thread---
子线程2---this is thread---
优先级
- 同优先级别的线程,采取的策略就是先到先服务(FCFS),使用时间片策略
- 如果优先级别高,被CPU调度的概率就高,但并不绝对
- 级别:1-10 默认的级别为5
/**
* The minimum priority that a thread can have.线程的最小优先级。
* The maximum priority that a thread can have.线程的最大优先级。
* The default priority that is assigned to a thread.分配给线程的默认优先级。
*/
public final static int MIN_PRIORITY = 1;
public final static int NORM_PRIORITY = 5;
public final static int MAX_PRIORITY = 10;
public class TestThread01 extends Thread {
@Override
public void run() {
System.out.println("线程01,优先级" + this.getPriority());
}
}
class TestThread02 extends Thread {
@Override
public void run() {
System.out.println("线程02,优先级" + this.getPriority());
}
}
class Test {
//这是main方法,程序的入口
public static void main(String[] args) {
//创建两个子线程,让这两个子线程争抢资源:
TestThread01 t1 = new TestThread01();
t1.setPriority(1);//优先级别低
TestThread02 t2 = new TestThread02();
t2.setPriority(10);//优先级别高
t1.start();
t2.start();
}
}
线程02,优先级10
线程01,优先级1
join()
- 当一个线程调用了join方法,这个线程就会先被执行,它执行结束以后才可以去执行其余的线程。
注意:必须先start,再join才有效。join(long millis)
等待该线程死亡的时间最多为millis。millis
—等待的时间,以毫秒为单位join(long millis, int nanos)
等待该线程的时间最多为millis毫秒加nanos纳秒。
public class TestThread extends Thread {
public TestThread(String name){
super(name);
}
@Override
public void run() {
System.out.println(this.getName()+"---#");
}
}
class Test{
public static void main(String[] args) throws InterruptedException {
for (int i = 1; i <= 3 ; i++) {
System.out.println("main-----"+i);
if(i == 2){
//创建子线程:
TestThread tt = new TestThread("子线程");
tt.start();
tt.join();//“半路杀出个程咬金”
}
}
}
}
sleep()
- 导致当前执行的线程睡眠(暂时停止执行)指定的毫秒数,但通过系统定时器和调度率的精度和准确性。 线程不会损失任何监视器的所有权。是Thread类下的一个方法。
sleep(long millis)
sleep(long millis, int nanos)
millis
-睡眠的时间长度,以毫秒为单位nanos
{@code 0-999999}额外的纳秒睡眠
public class TestThread {
public static void main(String[] args) {
//定义一个时间格式:
DateFormat df = new SimpleDateFormat("HH:mm:ss");
while(true){
//获取当前时间:
Date d = new Date();
//按照上面定义的格式将Date类型转为指定格式的字符串:
System.out.println(df.format(d));
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
每隔一秒打印一次时间
23:59:18
23:59:20
23:59:21
...
setDaemon()
- 将子线程设置为主线程的伴随线程,主线程停止的时候,子线程也停止执行了
setDaemon(boolean on)
将此线程标记为守护线程或用户线程。当唯一运行的线程都是守护线程时,Java虚拟机就会退出。这个方法必须在线程启动之前被调用。
public class TestThread extends Thread {
@Override
public void run() {
for (int i = 1; i <= 100 ; i++) {
System.out.println("thread----"+i);
}
}
}
class Test{
public static void main(String[] args) {
//创建并启动子线程:
TestThread tt = new TestThread();
tt.setDaemon(true);//设置伴随线程 注意:先设置,再启动
tt.start();
//主线程中输出1-20的数字:
for (int i = 1; i <= 10 ; i++) {
System.out.println("main---"+i);
}
}
}
此时的输出结果会出现抢资源现象,并且在主线程结束后子线程仍然会执行一点,消息延迟。
main---1 main---2 main---3 main---4 main---5 main---6 main---7 main---8 main---9 main---10 thread----1 thread----2 thread----3 thread----4 thread----5 thread----6 thread----7 thread----8 thread----9 thread----10 thread----11 thread----12 thread----13 thread----14 thread----15 thread----16 thread----17 thread----18 thread----19 thread----20 thread----21 thread----22 thread----23 thread----24 thread----25 thread----26 thread----27 thread----28 thread----29 thread----30 thread----31 thread----32 thread----33 thread----34 thread----35 thread----36 thread----37 thread----38 thread----39 thread----40 thread----41 thread----42 thread----43 thread----44 thread----45 thread----46 thread----47
stop()
Thread.currentThread().stop()
过期方法,不建议使用
public class TestStop {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
if (i == 6) {
Thread.currentThread().stop();
}
System.out.print(i);
}
}
}
12345