Effect picture
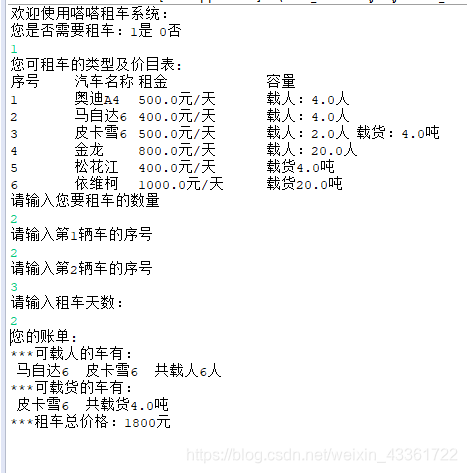
1.car parent
public class Car {
private String name;
private double rent;
public Car(String name, double rent){
this.name = name;
this.rent = rent;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getRent() {
return rent;
}
public void setRent(double rent) {
this.rent = rent;
}
}
2. Passenger Car
public class PassengerCar extends Car {
private int peopleCapacity;
public PassengerCar(String name, double rent, int peopleCapacity){
super(name,rent);
this.peopleCapacity = peopleCapacity;
}
public double getPeopleCapacity() {
return peopleCapacity;
}
public void setPeopleCapacity(int peopleCapacity) {
this.peopleCapacity = peopleCapacity;
}
}
3. Truck loaded with cargo
public class Truck extends Car {
double cargoCapacity;
public Truck (String name, double rent, double cargoCapacity){
super(name,rent);
this.cargoCapacity = cargoCapacity;
}
public double getCargoCapacity() {
return cargoCapacity;
}
public void setCargoCapacity(double cargoCapacity) {
this.cargoCapacity = cargoCapacity;
}
}
4.PickUp pickup truck
public class PickUp extends Car {
double cargoCapacity;
int peopleCapacity;
public PickUp(String name, double rent, double cargoCapacity, int peopleCapacity){
super(name,rent);
this.cargoCapacity = cargoCapacity;
this.peopleCapacity = peopleCapacity;
}
public double getCargoCapacity() {
return cargoCapacity;
}
public void setCargoCapacity(double cargoCapacity) {
this.cargoCapacity = cargoCapacity;
}
public double getPeopleCapacity() {
return peopleCapacity;
}
public void setPeopleCapacity(int peopleCapacity) {
this.peopleCapacity = peopleCapacity;
}
}
5. DadaInitial startup class
import java.util.Scanner;
public class DadaInitial {
public static void main(String[] args) {
Car[] list = {
new PassengerCar("奥迪A4", 500, 4),
new PassengerCar("马自达6", 400, 4),
new PickUp("皮卡雪6", 500, 4, 2),
new PassengerCar("金龙", 800, 20),
new Truck("松花江", 400, 4),
new Truck("依维柯", 1000, 20)
};
System.out.println("欢迎使用嗒嗒租车系统:");
System.out.println("您是否需要租车:1是 0否");
Scanner scan = new Scanner(System.in);
int isIn = scan.nextInt();
if(isIn == 1){
System.out.println("您可租车的类型及价目表:");
System.out.println("序号\t汽车名称\t租金\t\t容量");
int index = 1;
for(Car currentCar: list){
if(currentCar instanceof PassengerCar){
PassengerCar car = (PassengerCar) currentCar;
System.out.println(index + "\t" + car.getName() + "\t" + car.getRent() + "元/天\t载人:" + car.getPeopleCapacity() + "人 ");
}else if(currentCar instanceof Truck){
Truck car = (Truck) currentCar;
System.out.println(index + "\t" + car.getName() + "\t" + car.getRent() + "元/天\t载货" + car.getCargoCapacity() + "吨");
}else if(currentCar instanceof PickUp){
PickUp car = (PickUp) currentCar;
System.out.println(index + "\t" + car.getName() + "\t" + car.getRent() + "元/天\t载人:" + car.getPeopleCapacity() + "人 " + "载货:" + car.getCargoCapacity() + "吨");
}
index++;
}
System.out.println("请输入您要租车的数量");
int carSum = scan.nextInt();
int moneySum = 0;
String peopleName = "";
int peopleSum = 0;
String cargoName = "";
double cargoSum = 0;
for(int p = 1; p<=carSum; p++){
System.out.println("请输入第" + p + "辆车的序号");
int no = scan.nextInt();
moneySum += list[no-1].getRent();
if(list[no-1] instanceof PassengerCar){
PassengerCar chooseCar = (PassengerCar) list[no-1];
peopleName += " " + chooseCar.getName();
peopleSum += chooseCar.getPeopleCapacity();
}else if(list[no-1] instanceof Truck){
Truck chooseCar = (Truck) list[no-1];
cargoName += " " + chooseCar.getName();
cargoSum += chooseCar.getCargoCapacity();
}else if(list[no-1] instanceof PickUp){
PickUp chooseCar = (PickUp) list[no-1];
peopleName += " " + chooseCar.getName();
peopleSum += chooseCar.getPeopleCapacity();
cargoName +=" " + chooseCar.getName();
cargoSum += chooseCar.getCargoCapacity();
}
}
System.out.println("请输入租车天数:");
int day = scan.nextInt();
System.out.println("您的账单:");
System.out.println("***可载人的车有:");
System.out.println(peopleName + " 共载人" + peopleSum + "人");
System.out.println("***可载货的车有:");
System.out.println(cargoName + " 共载货" + cargoSum + "吨");
System.out.println("***租车总价格:" + (day*moneySum) + "元");
}else{
System.out.println("期待您的下次光临,再见");
}
}
}