1. Preparation
1.1 Generate the keytab file of the main hive/hive to the specified directory /var/keytab/hive.keytab
[root@fan102 ~]# kadmin.local -q "xst -k /var/keytab/hive.keytab hive/[email protected]"
1.2 View keytab content
[root@fan102 ~]# cd var/keytab
[root@fan102 keytab]# klist -e -k hive.keytab
Keytab name: FILE:hive.keytab
KVNO Principal
---- --------------------------------------------------------------------------
3 hive/[email protected] (aes128-cts-hmac-sha1-96)
3 hive/[email protected] (des3-cbc-sha1)
3 hive/[email protected] (arcfour-hmac)
3 hive/[email protected] (camellia256-cts-cmac)
3 hive/[email protected] (camellia128-cts-cmac)
3 hive/[email protected] (des-hmac-sha1)
3 hive/[email protected] (des-cbc-md5)
1.3 Verify whether the login is successful
[root@fan102 ~]# kinit -kt /var/keytab/hive.keytab hive/[email protected]
[root@fan102 ~]# klist
Ticket cache: FILE:/tmp/krb5cc_0
Default principal: hive/[email protected]
Valid starting Expires Service principal
07/14/2020 14:08:26 07/15/2020 14:08:26 krbtgt/[email protected]
renew until 07/21/2020 14:08:26
1.4 Copy the kerberos configuration file and keytab file to the local Windows (my path is: D:\keytab)
[root@fan102 ~]# cat /etc/krb5.conf
[root@fan102 ~]# cat /var/keytab/hive.keytab
1.5 Check the content of the IP location under realms in the krb5.conf file. If it is the server name, replace it with the server's IP address. The original IP address does not need to be modified
[realms]
HADOOP.COM = {
kdc = IP
admin_server = IP
}
2.java connection part
2.1 dependency (my version is 2.6)
<dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.hadoop/hadoop-common -->
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>2.6.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.hive/hive-jdbc -->
<dependency>
<groupId>org.apache.hive</groupId>
<artifactId>hive-jdbc</artifactId>
<version>1.1.1</version>
</dependency>
</dependencies>
2.2 Code (replace the IP with your own IP)
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.security.UserGroupInformation;
import java.sql.*;
public class KerberosTest {
private static String JDBC_DRIVER = "org.apache.hive.jdbc.HiveDriver";
private static String CONNECTION_URL = "jdbc:hive2://IP:10000/;principal=hive/[email protected]";
static {
try {
Class.forName(JDBC_DRIVER);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static void main(String[] args) throws Exception {
Class.forName(JDBC_DRIVER);
//登录Kerberos账号
System.setProperty("java.security.krb5.conf", "D:\\keytab\\krb5.conf");
Configuration configuration = new Configuration();
configuration.set("hadoop.security.authentication", "Kerberos");
UserGroupInformation.setConfiguration(configuration);
UserGroupInformation.loginUserFromKeytab("hive/[email protected]",
"D:\\keytab\\hive.keytab");
Connection connection = null;
ResultSet rs = null;
PreparedStatement ps = null;
try {
connection = DriverManager.getConnection(CONNECTION_URL);
ps = connection.prepareStatement("show databases");
rs = ps.executeQuery();
ResultSetMetaData metaData = null;
while (rs.next()) {
metaData = rs.getMetaData();
System.err.println(rs.getString(1));
}
System.err.println(metaData.getColumnName(1));
} catch (Exception e) {
e.printStackTrace();
}
}
}
3 results
3.1 java query results
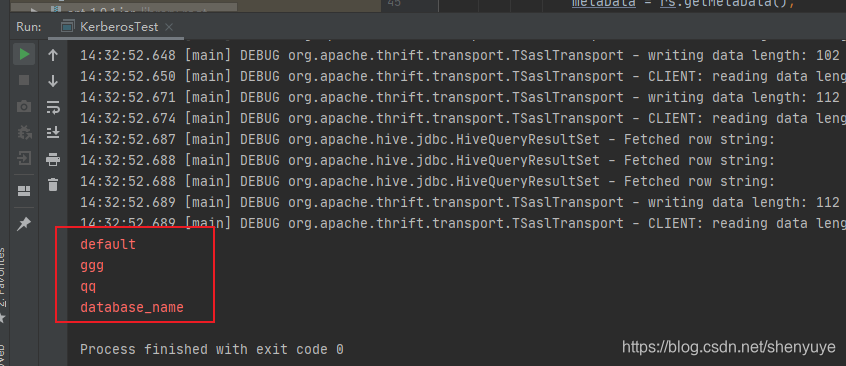
3.2 On Linux, beeline verification results
Note: 1.3 Step operation is not abnormal
[root@fan102 ~]# beeline -u "jdbc:hive2://fan102:10000/;principal=hive/[email protected]"
> show databases;
+++++++++++++++++++++++++++++++++++++++++
+ If you have any questions, you can +Q: 1602701980 Discuss together +
+++++++++++++++++++++++++++++++++++++++++
+ If you have any questions, you can +Q: 1602701980 Discuss together +
+++++++++++++++++++++++++++++++++++++++++