Combine strings alternately
Give you two strings word1 and word2. Please start with word1 and combine the strings by alternately adding letters. If one string is longer than another string, the extra letters are appended to the end of the combined string.
class Solution {
public:
string mergeAlternately(string word1, string word2) {
string res = "";
int size1 = word1.size();
int size2 = word2.size();
for (int i = 0; i < max(size1, size2); i++)
{
if(i<size1)
res += word1[i];
if(i<size2)
res += word2[i];
}
return res;
}
};
The minimum number of operations required to move all balls to each box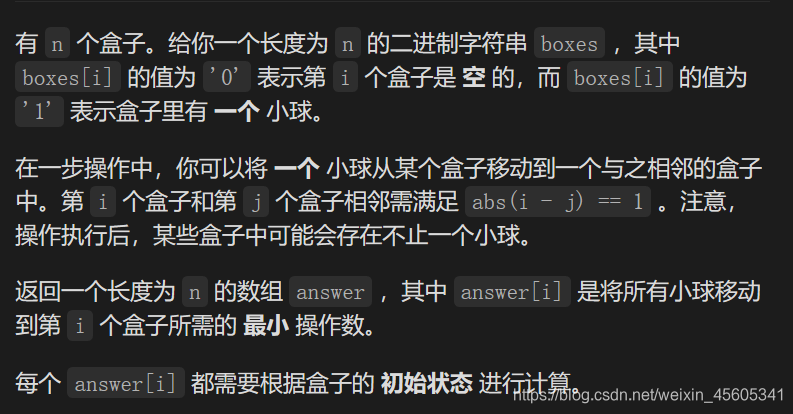
Note the following points, the box has only two states, 0 1. The movement can only be left or right. Then a box moving to a distance is the difference between the array subscripts.
class Solution {
public:
vector<int> minOperations(string boxes) {
vector<int> res(boxes.size(), 0);
int size = boxes.size();
for (int i = 0; i < size; i++)
{
int num = boxes[i] - '0';
if (num) {
for (int j = 0; j < size; j++)
{
res[j] += (abs(j - i) * num);
}
}
}
return res;
}
};
Maximum score to perform multiplication
m is 10 to the 3rd power, so dynamic programming. State transition is not easy to think about.
Let dp[i][j] be the left side of the nums array with i and the right side with j. When i+j==m is yes, just update the answer.
The number of enumeration is k, and the left is l, and the right is r=kl. Then the corresponding nums subscript on the left is l-1, and the right is nr.
Then l==0, all take the right side, and l ==k all take the left side.
class Solution {
public:
typedef long long ll;
int maximumScore(vector<int>& nums, vector<int>& multipliers) {
vector<vector<ll>>dp(1005, vector<ll>(1005, 0));
ll n = nums.size();
ll m = multipliers.size();
ll res = INT_MIN;
for (int k = 1; k <=m; k++)
{
//k为取的总数
for (int j = 0; j <= k; j++)
{
int l = j, r = k - j;
if (l == 0)dp[l][r] = dp[l][r - 1] + multipliers[k-1] * nums[n - r];
else if (l == k) dp[l][r] = dp[l - 1][r] + multipliers[k-1] * nums[l - 1];
else dp[l][r] = max(dp[l - 1][r] + multipliers[k-1] * nums[l-1],
dp[l][r - 1] + multipliers[k-1] * nums[n - r]);
if (k == m) res = max(res, dp[l][r]);
}
}
return res;
}
};
The length of the longest palindrome constructed from the subsequence
Similar to the longest palindrome substring solution method, let dp[i][j] be the length of the palindrome string in the ij interval.
There are s[i]==s[j], dp[i][j]=dp[i+1][j-1]+2.
In other cases dp[i][j]=max(dp[i+1][j],dp[i][j-1]). If there is no palindrome, the maximum update on both sides is required.
It should be noted that there are two strings in this question, and the answer update condition is i and j respectively correspond to the same letters in the two strings at the same time.
class Solution {
public:
int longestPalindrome(string word1, string word2) {
int size1 = word1.size();
int size2 = word2.size();
string s = word1 + word2;
int len_s = s.size();
int res = 0;
vector<vector<int>>dp(len_s, vector<int>(len_s));
for (int len = 1; len <= len_s; len++)
{
//枚举长度和起点
for (int i = 0; i + len - 1 < len_s; i++)
{
int j = i + len - 1;
if (len == 1)
dp[i][j] = 1;
else {
if (s[i] == s[j])
dp[i][j] = dp[i + 1][j - 1] + 2;
else dp[i][j] = max(dp[i + 1][j],dp[i][j-1]);
}
if (dp[i][j] > res&& i < size1 && j >= size1&&s[i]==s[j])
res = dp[i][j];
}
}
return res;
}
};