table of Contents
Thanks to Jianshu [Tang Xian-Seng]'s blog post data structure: Heap , this blog post has part of the content for reference.
One, heap introduction
The heap is a complete binary tree with a fixed order, usually represented by an array .
1. Using an array to represent the heap, how to distinguish between parent nodes and child nodes?
The following figure is a schematic diagram of a commonly used numbering method for a heap : the root node is numbered 0, the left child node of the root node is numbered 1, the right child node is numbered 2, and then 3, 4, 5, 6... Numbering from top to bottom, from left to right.
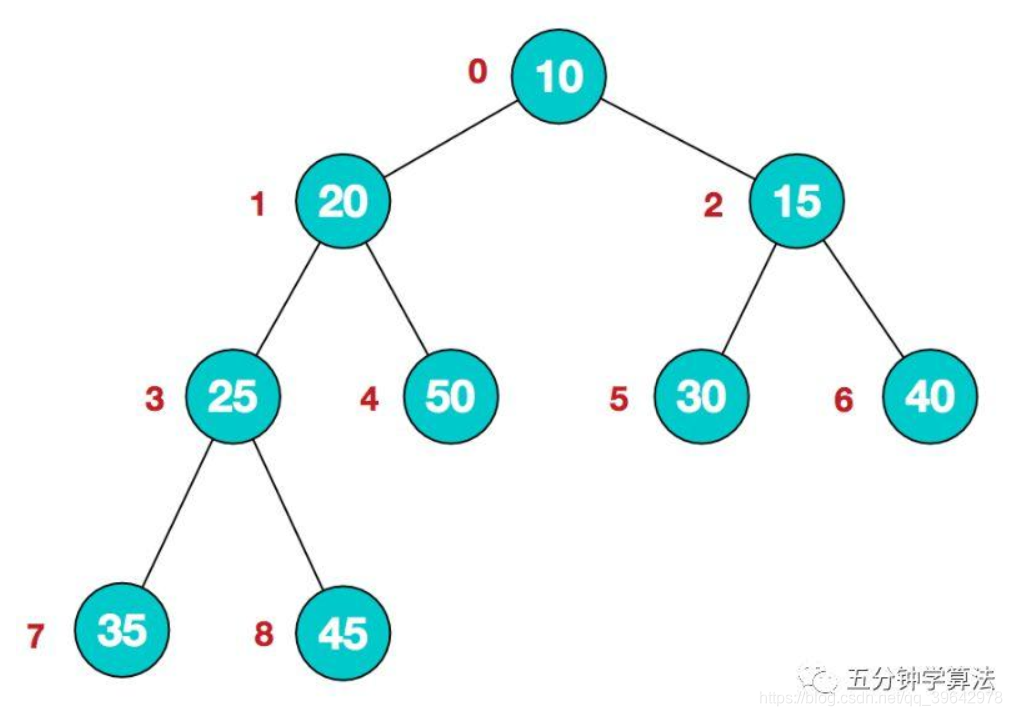
In the array, it is saved according to the above subscript. As shown in the figure above, it is saved as an array {10, 20, 15, 25, 50, 30, 40, 35, 45}.
In such an array, the subscript of the parent node of the node with subscript i is (i-1) / 2 (the division is rounded down); and the subscript of the left child node of the node with subscript i is 2. * i + 1 , the subscript of the right child node is 2 * i + 2.
Example:
第3个元素(25)的左子节点是第**2 * 3 + 1 = 7**个元素(35),
而它的右子节点是第**2 * 3 + 2 = 8**个元素(45)。
2. What kind of heap?
Heaps are generally divided into two types, namely the largest heap and the smallest heap.
The parent node of each node in the largest (small) heap must be larger (smaller) than the current node;
In other words, all nodes in the left and right subtrees of each node must be smaller (larger) than this node.
As shown in the figure below, it is a maximum heap:
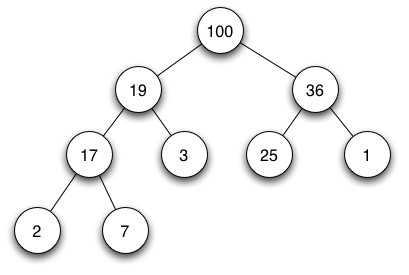
Two, heap insertion and deletion operations
In order to ensure that after we insert or delete any node, the maximum (small) heap can still guarantee its maximum (small) characteristics, we need to study a set of established and regular operations to complete the insertion and deletion.
1. Several basic operations
Before talking about the insertion and deletion of the heap, it is necessary to clarify several basic operations of the heap, and insertion and deletion are all composed of these basic operations:
shiftUp()
: If a node is larger (maximum heap) or smaller (minimum heap) than its parent node, then it needs to exchange positions with the parent node. In this way, the position of this node in the array rises.
shiftDown()
: If a node is smaller (maximum heap) or larger (minimum heap) than its child nodes, then it needs to be moved down. This operation is also called "heapify".
ShiftUp or shiftDown is a recursive process, so its time complexity is O(log n).
2. Heap Insertion
First, insert this new element at the first blank position of the heap, and then recursively call the above shiftUp()
operation until its parent node is larger (the largest heap) or smaller (the smallest heap).
Example:
Let's take a look at the details of the insert operation through an insert example. We insert numbers 16
into this heap: the
first step is to insert new elements into the first blank position of the heap. Then the heap becomes:
Unfortunately, the heap does not satisfy the attributes of the heap now, because 2 is above 16, we need to put the big number on it (this is the largest heap), in order to restore the heap attributes, we need to swap 16
and 2
.
It is not finished yet, because 10 is also smaller than 16. We continue to swap our inserted element and its parent node until its parent node is larger than it or we reach the top of the tree. This is what is called shiftUp()
, and it needs to be done after every insert operation. It "floats" a number that is too large or too small to the top of the tree.
Finally, we get the heap:
each parent node is now larger than its child nodes, which satisfies the property of the largest heap.
3. Heap deletion
In order to fill in the vacancy after this node is deleted, first copy the value of the last element in the heap** (assuming value)** to this position (this is equivalent to deleting the original node at this position), and then Then delete the last element of the heap (because it has been assigned to the position of the deleted node), and then at the deleted position, use the current value of this position to compare with its parent node and child node, if it The relationship with the parent node destroys the largest (small) heap, then recursive call shiftUp()
to repair; if its relationship with the child node destroys the largest (small) heap, recursive call shiftDown()
to repair.
Example:
We delete (10) in this tree:
now there is an empty node at the top, how to deal with it?
When inserting a node, we return the new value to the end of the array. Now let's do the opposite: we take the last element in the array, put it at the top of the tree, and then fix the heap properties.
The value of the element at the deleted position is 1. Since it has no parent node, we only compare it with the child node to see if it violates the maximum heap property: now there are two numbers (7 and 2) available for exchange . We choose the larger of the two and call the maximum value and place it at the top of the tree, so swap 7 and 1, and now the tree becomes:
continue to stack until the node has no child nodes or it is more than two child nodes So far. For our heap, we only need to have another exchange to restore the heap attributes:
as above, the heap deletion operation is completed.