Article Directory
-
- 1. Lambda expression
- 2. Static and Final
- 3. Collections in Java
- 4. Functional Interface
- 5. String
- 6. Stream data flow
- 7. Input和Output
- 8. The difference between Equals and ==
- 9. Application of String
- 10. Array
- 11. Class access permissions
- 12. Inheritance
- 13. Generate random numbers
- 14. format method
- 15. Interface
- 16. Relationship Between Classes
- 17. Throwable类
- 18. Generic
- 19. Enumeration
- 20. Variables
- 21. The super keyword
1. Lambda expression
2. Static and Final
static and final modifiers in java
1, static variables
According to whether the class member variables are statically classified, there are two types: one is the variable modified by static, called static variable or class variable; the other is the variable not modified by static, called instance variable. The difference between the two is that there is only one copy of static variables in memory (memory saving). JVM only allocates memory once for static. The memory allocation of static variables is completed in the process of loading the class, which can be directly accessed by the class name, of course. Access through objects (but this is not recommended).
2. Static method
Static methods can be called directly by the class name, and any instance can be called. Therefore, the this and super keywords cannot be used in static methods, and the instance variables and instance methods of the class cannot be directly accessed (that is, member variables and members without static Member method), can only access the static member variables and member methods of the class to which they belong. Because instance members are associated with specific objects! Because the static method is independent of any instance, the static method must be implemented, not an abstract abstract
3. Attributes in the final modification class
Regardless of whether the attribute is a basic type or an object class, the role of final is that the "value" stored in the variable cannot be changed. This value, for basic types, puts real values in the variables, such as 1, "abc" and so on. The reference type variable contains an address, so the final modification of a reference type variable means that the address in it cannot be changed, not that the content of the object or array pointed to by the address cannot be changed. This must be paid attention to.
Final modifies the attribute, you can not assign a value when declaring a variable, and once the value is assigned, it cannot be modified. The final attribute can be assigned in three places: at the time of declaration, in the initialization block, and in the construction method. In short, it must be assigned.
3. Collections in Java
4. Functional Interface
Java8 four core functional interfaces: Function, Consumer, Supplier, Predicate
Original: https://blog.csdn.net/a879611951/article/details/80104014
Function<T, R>
T: input parameter type, R: output parameter type
Calling method: R apply(T t);
Example of function definition: Function<Integer, Integer> func = p -> p * 10; // 10 times the input and output parameters
Example of calling function: func.apply(10); // result 100
Consumer
T: Type of input; no output
Calling method: void accept(T t);
Definition function example: Consumer consumer= p -> System.out.println§; // Because there is no output parameter, it is often used for consumer actions such as printing and sending SMS
Example of calling function: consumer.accept("18800008888");
Supplier
T: parameter type; no parameter input
Calling method: T get();
Example of a defined function: Supplier supplier= () -> 100; // It is often used in the application scenario where the business “conditional operation” is met, and then called to obtain the result when the conditions are met; the running result must be defined in advance, but not run.
Example of calling function: supplier.get();
Predicate
T: input parameter type; output parameter type is Boolean
Calling method: boolean test(T t);
Example of function definition: Predicate predicate = p -> p% 2 == 0; // Determine whether or not it is even
Example of calling function: predicate.test(100); // The running result is true
5. String
Similarities and differences between C language and Java strings
The character array in C language needs the terminator'\0' to end, but Java does not
From https://blog.csdn.net/weixin_30539625/article/details/98595479
6. Stream data flow
7. Input和Output
Scancer:
The next() method input string cannot read spaces, you can use nextLine() to read a line.
Summary: When the next encounters a space, the input ends with a newline. And nextLine only ends the input with a line feed (carriage return).
User Input Command Line Argument can only pass input into the program when the program is running, and cannot be used during the running of the program. We can use the Scanner class to solve this problem. The role of Scanner is similar to scanf in C language, but it provides us with more functions (method). Scanner (Java Platform SE 8) (oracle.com)
Read Files :
The first method, using FileReader and Scanner
Advantages: scanner can help us Parse data => nextInt()
The second method, using FileReader and BufferedReader
Advantages: larger buffer, higher efficiency => can read larger files, faster
Differences:
Scanner's nextLine(): If there is no content, it will report an error. Use hasNextLine() to check first but br's readLine() will not report an error. If there is no content, it will return null.
Read the csv file:
Standard Output :
Usually we print some content to the terminal to debug or print out the results we want
System.out.println(): Print a String in the standard output and automatically add \n blank lines
System.out.printf(): Print the string according to the format we set, and will not automatically blank lines!
System.out.format(): Same as above
8. The difference between Equals and ==
Comparison of == and equal() in java
8 basic data types in Java:
Floating point type: float(4 byte), double(8 byte)
整型:byte(1 byte), short(2 byte), int(4 byte) , long(8 byte)
Character type: char(2 byte)
Boolean: boolean (the JVM specification does not clearly specify the size of the space it occupies, only that it can only take the literal values "true" and "false")
For the variables of these 8 basic data types, the variable directly stores the "value", so when the relational operator == is used for comparison, the comparison is the "value" itself. It should be noted that both floating-point and integer types are signed types, while char is an unsigned type (char type ranges from 0 to 2^16-1).
In other words:
int n=3;
int m=3;
Variable n and variable m are the directly stored value "3", so the result is true when comparing with ==.
For variables of non-basic data types, they are called reference-type variables in some books. For example, str1 above is a variable of reference type. The variable of reference type stores not the "value" itself, but the address of its associated object in memory. For example, the following line of code:
String str1;
This sentence declares a variable of reference type, at this time it is not associated with any object.
And through new String ("hello") to generate an object (also known as an instance of the class String), and bind this object to str1:
str1= new String("hello");
Then str1 points to an object (str1 is also referred to as an object reference in many places). At this time, the variable str1 stores the storage address of the object it points to in memory, not the "value" itself, which means it is not The string "hello" stored directly. The references here are very similar to pointers in C/C++.
Therefore, when using == to compare str1 and str2 for the first time, the result is false. Therefore, they respectively point to different objects, which means that they actually store different memory addresses.
Some other classes, such as Double, Date, Integer, etc., have rewritten the equals method to compare whether the contents stored in the pointed object are equal.
In conclusion:
1) For ==, if it acts on a variable of a basic data type, directly compare whether the stored "value" is equal;
If it acts on a variable of reference type, the address of the object pointed to is compared
##
2) For the equals method, note: the equals method cannot be applied to variables of basic data types
If the equals method is not rewritten, the address of the object pointed to by the variable of the reference type is compared;
If classes such as String and Date rewrite the equals method, the comparison is the content of the pointed object.
From https://blog.csdn.net/baidu_38634017/article/details/85395614
The first interview (detailed explanation of equal and == in java)_flysening的博客-CSDN博客
9. Application of String
Compare() method
1. The compareto method in java
1. Returns the difference between the asc codes of the two strings before and after the comparison. If the first letters of the two strings are different, the method returns the difference between the asc codes of the first letter
String a1 = "a";
String a2 = "c";
System.out.println(a1.compareTo(a2));//结果为-2
2. That is, if the first character of the two strings involved in the comparison is the same, the next character will be compared until there are differences, and the asc code difference of the different characters will be returned.
String a1 = "aa";
String a2 = "ad";
System.out.println(a1.compareTo(a2));//结果为-3
3. If the two strings are not the same length, and the characters that can participate in the comparison are exactly the same, the difference between the lengths of the two strings will be returned
String a1 = "aa";
String a2 = "aa12345678";
System.out.println(a1.compareTo(a2));//结果为-8
4. Return a positive number to indicate a1>a2, return a negative number to indicate a1<a2, and return 0 to indicate a1==a2;
compareTo cannot be used for numeric types, and compareTo cannot be used to compare nt with int, directly use greater than (>) Less than (<) or equal to (==) not equal to (!=) to compare
int num1 = 4;
int num2 = 5;
num1.compareTo(num2);//Cannot invoke compareTo(int) on the primitive type int
You can first convert your int variable to String and then compare
int num1 = 4;
int num2 = 5;
//parse int to String
System.out.println((num1+"").compareTo(num2+""));//-1
System.out.println(new Integer(num1).toString(). compareTo(new Integer(num2).toString()));//-1
System.out.println(String.valueOf(num1).compareTo(String.valueOf(num2)));//-1
Use the format function to format:
Note: All the above String operations will not change the original String value, but get a new String (String is immutable)
10. Array
The one-dimensional arrays forming the two-dimensional array do not have to have the same length, and the lengths can be specified separately (the number of allocated elements must be specified):
inta[][]=newint[3][];
a[0]=newint[6];
a[1]=newint[12];
a[2]=newint[4];
11. Class access permissions
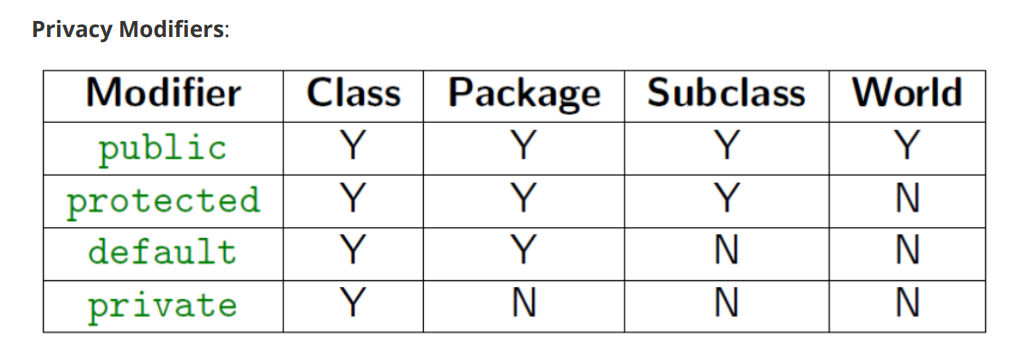
12. Inheritance
When the subclass and the parent class are in the same package, the subclass naturally inherits the member variables and methods that are not private in the parent class, but does not inherit the construction method.
Rewrite:
13. Generate random numbers
(1) Call the static method random() of the Math class to return a random number: [0.0, 1.0)
Example: The following code gets a random integer between 1 and 100 (including 1 and 100):
(int)(Math.random()*100)+1
(2) Use the Random class in java.util
Construction method: public Random();
public Random(long seed); (use the current machine time as a seed to create a Random object)
example:
Random random=new Random();
random.nextInt(); (returns a random integer)
random.nextInt(m); (return value [0,m))
random.nextDouble; (return value [0.0,1.0))
random.nextBoolean();
14. format method
The format() method of the String class
Format the number:
(1) %d: decimal integer
%o: octal integer
%x: Hexadecimal integer
%X: Hexadecimal integer (uppercase)
String s=String.format("%d,%o,%x,%X",703576,703576,703576,703576);
Output s: 703576, 2536130, abc58, ABC58
(2) %+d is forced to add a + sign
%,d: formatted integers are grouped by thousands, separated by commas
(3) Control length
%md: Add a space to the left to make m digits
%0md: Add 0 to the left to make m bits
(4) %f: formatted as a decimal floating point number, with six decimal places reserved
%e(%E): A decimal floating point number formatted in scientific notation (%E capitalizes the exponent part)
Example: String s=String.format("%f,%e",13579.98,13579.98);
Output s: 13579.980000, 1.357998e+04
(5)%.nf: Keep n decimal places
Formatting time:
format (formatting mode, date list)
format(Locale locale, formatting mode, date list)
Example: Locale.US
Date nowTime=new Date();
If you want to format the same date, you can use the "<" symbol
example:
String str1=String.format("%tY年%<tm月%<td日",nowTime);
Output str1: October 01, 2016
The %tx conversion character in the string format is specially used to format the date and time. The x in the %tx conversion symbol represents another conversion symbol that handles the date and time format.
From https://blog.csdn.net/shayu8nian/article/details/49492783
15. Interface
Abstract classes can have ordinary methods, but interfaces only have abstract methods, and the access rights of all constants (no variables) in the interface body must be public, and they must be static and final constants (public, final and static are allowed to be omitted), all The permissions of abstract methods must be public (public abstract is allowed to be omitted).
Detailed explanation of java's Comparable interface-Mrfanl-博客园(cnblogs.com)
16. Relationship Between Classes
17. Throwable类
Throwable exceptions and errors in java
Error:
It is an error that the program cannot handle, indicating a serious problem in running the application. Most errors have nothing to do with the actions performed by the code writer, but represent problems with the JVM (Java Virtual Machine) when the code is running. For example, a Java virtual machine running error (Virtual MachineError), when the JVM no longer has the memory resources needed to continue the operation, an OutOfMemoryError will occur. When these exceptions occur, the Java Virtual Machine (JVM) generally chooses the thread to terminate.
Exception:
It is an exception that can be handled by the program itself.
Exceptions in Java are divided into two categories, runtime exceptions and compilation time translation exceptions. Except for runtime exceptions (RuntimeException), the rest are compile-time exceptions.
-
1. Unchecked exceptions (runtime exceptions)
are all exceptions of the RuntimeException class and its subclasses, which are the exceptions that appear in the console when the program terminates when we test functions in development, such as: -
- NullPointerException (null pointer exception),
- IndexOutOfBoundsException (subscript out of bounds exception)
- ClassCastException (class conversion exception)
- ArrayStoreException (data storage exception, inconsistent type when operating array)
- BufferOverflowException for IO operation
-
2. Checked exceptions
non-runtime exceptions (compiled exceptions): are exceptions other than RuntimeException, which belong to the Exception class and its subclasses.
This exception can be predicted, and the compiler will force the exception to be caught or declared at compile time.
From the perspective of program syntax, it is an exception that must be handled. If it is not handled, the program cannot be compiled and passed. Such as -
- IOException, when a certain I/O exception occurs, this exception is thrown.
- SQLException, this exception indicates that the ongoing query violates the SQL syntax rules. When an exception occurs, this exception is thrown.
- EOFException,
- FileNotFoundException, etc. and user-defined Exception exceptions, generally do not customize check exceptions.
In layman's terms, there are red lines when writing code, and exceptions that occur when try catch or throws are needed!
18. Generic
19. Enumeration
20. Variables
21. The super keyword
Once the subclass hides the inherited member variable/method, the object created by the subclass no longer owns the variable/method, and the variable is owned by the keyword super.
- When super calls a hidden method, the member variables that appear in the method are member variables hidden by subclasses or inherited member variables.
When using the subclass's construction method to create an object of a subclass, the subclass's construction method always first calls a certain construction method of the parent class. Since the subclass does not inherit the construction method of the parent class, super() is used by default if there is no obvious call. Therefore, when the parent class defines multiple construction methods, a construction method without parameters should be included.