The last article introduced you to " Python (1): Introduction to Python and Environment Configuration ". Today, I will introduce you to the content of Python courses gradually.
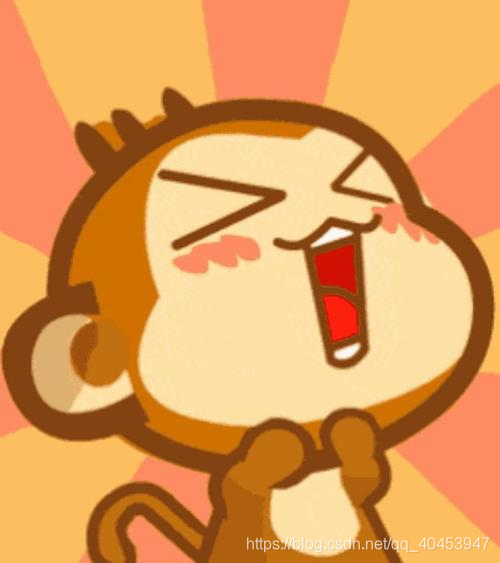
Python 变量类型
The value of a variable stored in memory, which means that a space is opened up in memory when the variable is created. Based on the data type of the variable, the interpreter allocates designated memory and decides what data can be stored in the memory. Therefore, variables can specify different data types, and these variables can store integers, decimals, or characters.
Variable assignment Variable assignment in
Python does not require a type declaration. Each variable is created in the memory, including the identification, name and data of the variable.
Each variable must be assigned a value before it is used, and the variable will be created after the variable is assigned.
The equal sign = is used to assign values to variables. The left side of the equal sign = operator is a variable name, and the right side of the equal sign = operator is the value stored in the variable. E.g:
#!/usr/bin/python
# -*- coding: UTF-8 -*-
counter = 100 # 赋值整型变量
miles = 1000.0 # 浮点型
name = "John" # 字符串
print counter
print miles
print name
In the above example, 100, 1000.0 and "John" are assigned to the counter, miles, and name variables respectively. Executing the above program will output the following results:
100
1000.0
John
Multiple variable assignment
Python allows you to assign values to multiple variables at the same time. E.g:
a = b = c = 1
The above example creates an integer object with a value of 1, and three variables are allocated to the same memory space. You can also specify multiple variables for multiple objects. E.g:
a, b, c = 1, 2, "john"
Standard data types
The data stored in the memory can be of multiple types. For example, a person's age can be stored in numbers, and his name can be stored in characters.
Python defines some standard types for storing various types of data. Python has five standard data types:
- Numbers
- String
- List
- Tuple (tuple)
- Dictionary
Python numbers
The numeric data type is used to store numeric values. They are immutable data types, which means that changing the numeric data type will allocate a new object. When you specify a value, the Number object is created:
var1 = 1
var2 = 10
You can also use the del statement to delete references to some objects. The syntax of the del statement is:
del var1[,var2[,var3[....,varN]]]
You can delete references to single or multiple objects by using the del statement. E.g:
del var
del var_a, var_b
Python supports four different number types:
- int (signed integer)
- long (long integer [can also represent octal and hexadecimal])
- float (floating point)
- complex (plural)
Python string:
A character string or string (String) is a string of characters composed of numbers, letters, and underscores. Generally marked as:
s = "a1a2···an" # n>=0
It is a data type that represents text in a programming language. Python's string list has 2 order of values:
- The index from left to right starts at 0 by default, and the maximum range is 1 less than the string length
- The index from right to left starts at -1 by default, and the maximum range is the beginning of the string
#!/usr/bin/python
# -*- coding: UTF-8 -*-
str = 'Hello World!'
print str # 输出完整字符串
print str[0] # 输出字符串中的第一个字符
print str[2:5] # 输出字符串中第三个至第六个之间的字符串
print str[2:] # 输出从第三个字符开始的字符串
print str * 2 # 输出字符串两次
print str + "TEST" # 输出连接的字符串
The output of the above example:
Hello World!
H
llo
llo World!
Hello World!Hello World!
Hello World!TEST
Python数据类型转换
Sometimes, we need to convert the built-in type of the data, the conversion of the data type, you only need to use the data type as the function name.
The following built-in functions can perform conversion between data types. These functions return a new object that represents the converted value.
function | description |
---|---|
int(x [,base]) | Convert x to an integer |
long(x [,base] ) | Convert x to a long integer |
float(x) | Convert x to a floating point number |
complex(real [,imag]) | Create a plural |
str(x) | Convert object x to string |
repr(x) | Convert object x to expression string |
eval(str) | Used to calculate valid Python expressions in strings and return an object |
tuple(s) | Convert sequence s into a tuple |
dict(d) | Create a dictionary. d must be a sequence (key, value) tuple. |
chr(x) | Convert an integer to a character |
… | … |
Python puzzle answers, as shown below
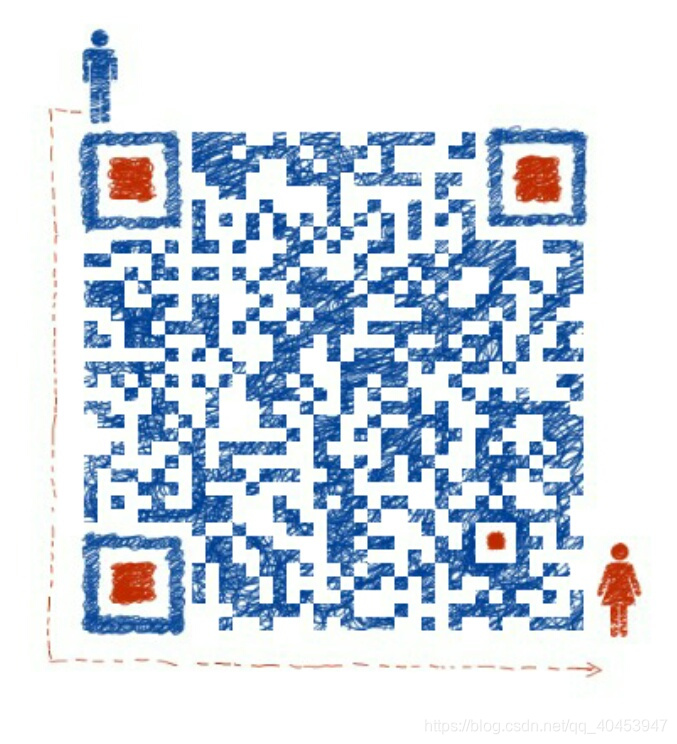