Common Embedded Interview Questions in C Language-Issue 1
-
- Preface
- Issue 1:
-
- 1. The role of the keyword static
- 2. What is the meaning of the keyword const
- 3. What is the meaning of the keyword volatile, and give three different examples
- 4. What is the difference between references and pointers
- 5. The role of ifndef/define/endif in the .h header file
- 6. The difference between global variables and local variables in memory
- 7. The difference between array and linked list
- 8. What causes stack overflow in general
- 9. Memory allocation in C/C++
- 10. Can't do the data type of switch() parameter
- 11. Can local variables have the same name as global variables
- 12. How to reference a global variable that has been defined
- 13. Can global variables be defined in header files that can be included by multiple .C files? Why?
- 14. The difference between static global variables, local variables, functions and ordinary global variables, local variables, and functions
- 15. What is pre-compilation and when do you need pre-compilation
- 16. The difference between structure and union
- 17. Please tell me what are the advantages of const compared to #define
- 18. Briefly describe the difference between arrays and pointers
- 19 Discuss the advantages and disadvantages of parameter-containing macros and functions
- 20. Accessing fixed memory locations (Accessing fixed memory locations)
- to sum up
Preface
This blog aims to share the relevant knowledge compiled during the learning process, mainly involving C language, C++ and embedded related issues. If it involves quoting parts that are not sourced, please write to or comment in time. The wrong part of the blog content will be corrected in time. If you are lucky enough to be reprinted, please indicate the source~ Thank you for your support!
Issue 1:
1. The role of the keyword static
In the C language, static is mainly used to define global static variables, local static variables and static functions.
Global static variables : allocate memory in the global data area, the scope is only visible in the defined file, and the life cycle is until the end of the program.
Local static variables : allocate memory in the global data area, the scope is only visible in the defined module, and the period is stored until the end of the program.
Static function : It can only be used in this source file. If there are functions with the same name in other files, they will not interfere with each other.
Two new functions are added in C++: defining static data members or static function members.
Static data members must be defined and initialized outside the class, static functions cannot access non-static data members
2. What is the meaning of the keyword const
Simple understanding: read-only
(1) const int a;
(2) int const a;
(3) const int *a;
(4) int * const a;
(5) int const * a const;
where (1) (2) ) Has the same effect, a is a constant integer number. (3) Means a is a pointer to a constant integer (integer is unmodifiable, but pointers can). (4) The meaning a is a constant pointer to an integer (the integer pointed to by the pointer can be modified, but the pointer is not). In (5), a is a constant pointer to a constant integer (the integer pointed to by the pointer is unmodifiable, and the pointer is also unmodifiable).
Protect some important addresses and data, pass information to people who read the code, and prevent the code from being modified unintentionally
1). The function of the keyword const is to convey very useful information to people who read your code
2). By giving the optimizer With some additional information, using the keyword const may produce more compact code.
3). Reasonable use of the keyword const can make the compiler naturally protect those parameters that do not want to be changed, and prevent them from being unintentionally modified by the code. In short, this can reduce the appearance of bugs
3. What is the meaning of the keyword volatile, and give three different examples
Answer: Without compiler optimization, the program will re-read this variable every time it uses it, instead of using the backup saved in the register
1). The hardware register of the parallel device (such as the status register)
2). An interrupt Non-automatic variables that will be accessed in service subroutines (Non-automatic variables)
3) Variables shared by several tasks in multithreaded applications
1. Can a parameter be either const or volatile? Explain why.
The volatile modifier tells the supplier that the variable value can be changed in any way that is not explicitly specified by the program. The most common example is the value of the external port. Its change can be changed without any assignment statement in the program. This kind of variable is It can be modified with volatile, and the complier will not optimize it. Variables modified by const cannot be changed in the program, but can be modified by things outside the program, such as the value of an external port. If only const is used, the compiler may optimize these variables, and volatile will not be a problem.
2. Can a pointer be volatile? Explain why.
Because pointers are the same as ordinary variables, there are sometimes uncontrollable changes in the program. Common example: When the interrupt service subroutine modifies a pointer to a buffer, volatile must be used to modify the pointer.
4. What is the difference between references and pointers
The reference must be initialized, the pointer need not.
The reference cannot be changed after initialization, and the pointer can change the object pointed to.
There is no reference to the null value, but there is a pointer to the null value.
After a pointer points to an object through a pointer variable, it indirectly operates on the variable it points to. The use of pointers in the program makes the program less readable; the reference itself is the alias of the target variable, and the operation of the reference is the operation of the target variable.
5. The role of ifndef/define/endif in the .h header file
Prevent the header file from being repeatedly quoted.
6. The difference between global variables and local variables in memory
Global variables are stored in the static data area, and local variables are in the stack.
The life cycle of a global variable is the entire program running period, and the life cycle of a local variable is the function interval in which the variable is declared.
7. The difference between array and linked list
An array is a continuous memory interval with a fixed size, and data can only be stored continuously.
The linked list can be a continuous memory interval or a discontinuous memory interval. The size is not fixed, and the data can be stored randomly.
8. What causes stack overflow in general
1) The function call level is too deep. When a function is called recursively, the system must keep saving the scene and variables generated when the function is called in the stack. If the recursive call is too deep, the stack will overflow, and the recursion cannot return at this time. When the function call level is too deep, the stack may not be able to accommodate the return address of these calls and cause stack overflow.
2) Dynamic application space is not released after use. Since there is no automatic garbage collection mechanism in the C language, the program needs to actively release the dynamic address space that is no longer in use. The requested dynamic space uses heap space, and the use of dynamic space will not cause heap overflow.
3) The array access is out of bounds. C language does not provide array subscript out-of-bounds check. If the array subscript access exceeds the array range in the program, memory access errors may occur during operation.
4) Illegal access to pointers. The pointer saves an illegal address, and a memory access error will occur when the address pointed to by such a pointer is accessed.
9. Memory allocation in C/C++
In C/C++, the memory is divided into 5 areas, namely stack area, heap area, global/static storage area, constant storage area, and code area. Usually the 32-bit Linux kernel virtual address space is divided into 0 3G as user space and 3 4G as kernel space. The distribution of Linux process address space is shown in the figure below:
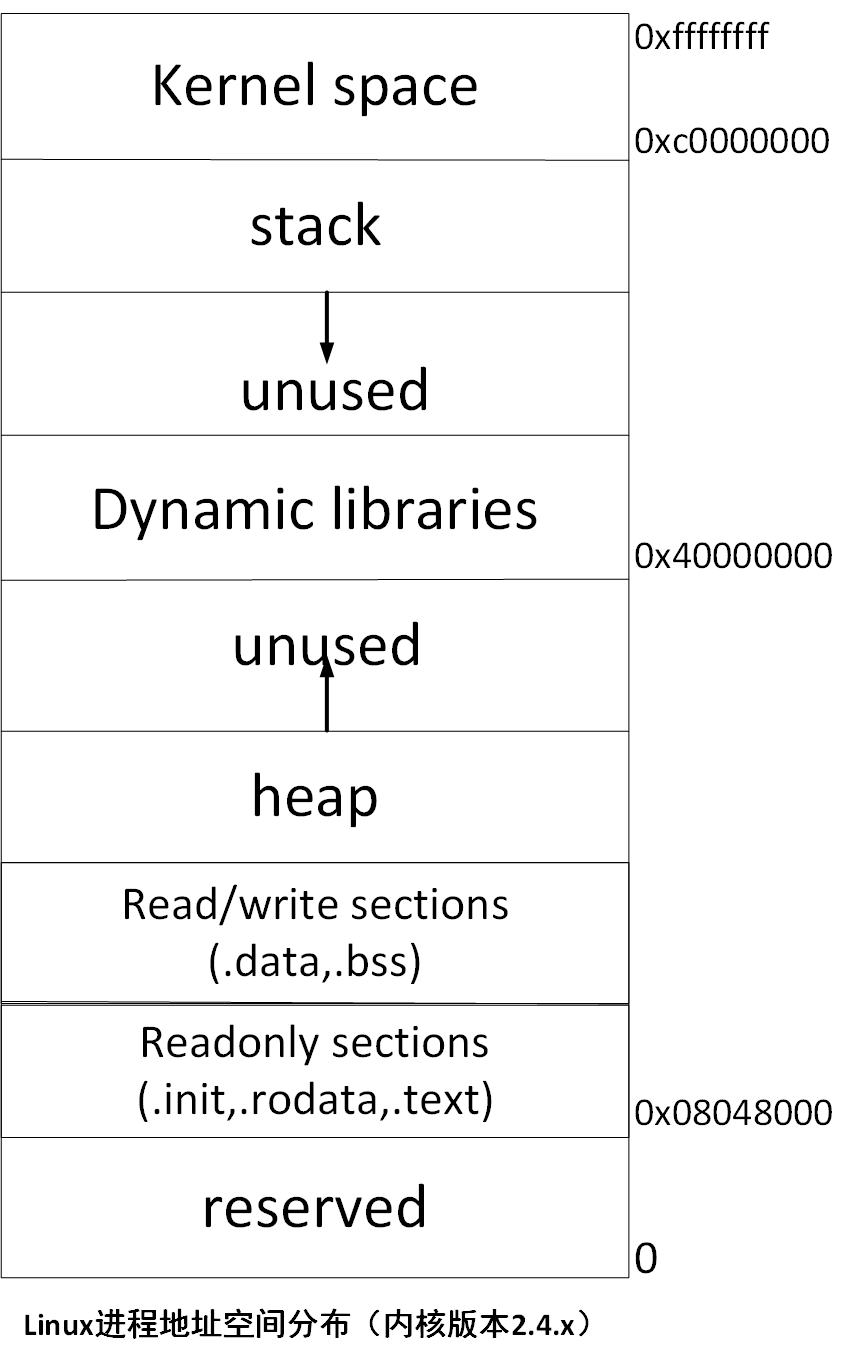
Stack area (stack) : It is allocated by the compiler when needed. When the function is executed, the formal parameters and local variables (excluding the variables declared by static) are allocated in the stack area. After the function runs, the memory is automatically released. Belongs to dynamic memory allocation
heap area (heap) : the memory segment that is dynamically allocated during the process of storage is allocated and released manually by the programmer. If the requested memory is not released, the system will automatically reclaim it after the program runs. In C language, memory is requested and released through malloc or calloc, realloc, and free functions. **It belongs to dynamic memory allocation
global/static storage area (.data section) : initialized global variables (including global static variables) and local static variables, which are released by the system after the program runs. Belongs to static memory allocation.
Constant storage area (.rodata section) : Contains global constants, const-modified global variables and string constants, and const-modified local variables are still in the stack area. Belongs to static memory allocation.
Code segment (.text segment) : Store the binary code of the program.
Supplement : BSS section (Block Started by Symbol): stores uninitialized global variables in the program and global variables initialized to 0. Belongs to static memory allocation.
Dynamic link library mapping area: if the program calls the dynamic link library, it will be this part. This area is used to map the loaded dynamic link library.
Reserved area: A memory area in the memory that is protected and forbidden to access.
10. Can't do the data type of switch() parameter
Answer: Except for floating point (single precision float, double precision double), string type (string). C/C++ supports byte, char, short, int, long, bool, integer types and enum (enumeration) types. Float, double, and string are not supported.
11. Can local variables have the same name as global variables
Answer: Yes, the whole will be shielded locally. To use global variables, you need to use "::".
Local variables can have the same name as global variables. When referencing this variable in a function, the local variable with the same name will be used instead of the global variable. For some compilers, multiple local variables with the same name can be defined in the same function. For example, a local variable with the same name is defined in two loop bodies, and the scope of that local variable is in that loop body.
12. How to reference a global variable that has been defined
Answer: You can use the header file or the extern keyword . If you use the header file method to refer to a global variable declared in the header file, assuming that you write that variable incorrectly, an error will be reported during compilation. If you use the extern method to quote, assume that you made the same mistake , Then no errors will be reported during compilation, but errors will be reported during connection.
13. Can global variables be defined in header files that can be included by multiple .C files? Why?
Answer: Yes, you can declare global variables with the same name in static form in different C files.
You can declare a global variable with the same name in different C files, provided that only one C file can be assigned an initial value for this variable, and the link will not go wrong at this time.
14. The difference between static global variables, local variables, functions and ordinary global variables, local variables, and functions
Scope of static global variables: only valid in the source file where the variable is defined. The scope of
static functions is different from ordinary functions, plus static can only be described and defined in the current source file.
The difference between static global variables and ordinary global variables: static global variables are only initialized once to prevent them from being referenced in other file units; the
difference between static local variables and ordinary local variables: static local variables are only initialized once, and the next time is based on the last time Result value; The
difference between static function and ordinary function: static function has only one copy in memory, and ordinary function maintains one copy for each call.
15. What is pre-compilation and when do you need pre-compilation
Answer: Pre-compilation is also called pre-processing, which is to replace some code text. #define Macro definition replacement, conditional compilation, etc.
16. The difference between structure and union
(1) Structures and unions are composed of multiple members of different data types. Only one selected member is stored in the union (all members share an address space), and all members of the structure exist (the storage addresses of different members) different).
(2) Assigning values to different members of the union will overwrite other members. The original member values no longer exist, and the assignment of different members of the structure does not affect each other.
17. Please tell me what are the advantages of const compared to #define
Answer: const functions: define constants, modify function parameters, and modify function return values. The things modified by const are subject to mandatory protection, which can prevent accidental changes and improve the robustness of the program.
(1) const constants have data types, while macro constants have no data types. The compiler can perform type safety checks on the former (const). define only performs character substitution, no type safety checks, and unexpected errors may occur in character substitution.
(2) Some integrated debugging tools can debug const constants, but cannot debug macro constants
18. Briefly describe the difference between arrays and pointers
Answer: The array is created in a static storage area (such as a global array) or is created on the stack. The name of the array corresponds to (rather than points to) a piece of memory, and its address and capacity remain unchanged during the lifetime, and only the contents of the array can be changed.
The pointer can point to any type of memory block at any time, which is more flexible.
19 Discuss the advantages and disadvantages of parameter-containing macros and functions
1. When a function is called, first find the value of the actual parameter expression, and then bring in the formal parameters. Using macros with parameters is just a simple character replacement.
2. Macro replacement does not take up running time, only compiling time; while function calls take up running time (allocate unit, reserve site, value transfer, return).
3. When the macro is defined, it can be any type of data. Types must be defined for the actual and formal parameters in the function. The type requirements of the two are consistent. If they are inconsistent, type conversion should be performed.
20. Accessing fixed memory locations (Accessing fixed memory locations)
Embedded systems often require programmers to access a specific memory location. In a project, it is required to set the value of an integer variable with an absolute address of 0x67a9 to 0xaa66. The compiler is a pure ANSI compiler. Write code to accomplish this task.
This question tests whether you know that it is legal to typecast an integer to a pointer in order to access an absolute address. The way to achieve this problem varies with personal styles. A typical similar code is as follows:
int *ptr;
ptr = (int *)0x67a9;//First take out the value of the address and pay to the pointer
*ptr = 0xaa66; //Then dereference the pointer
to sum up
In the near future, I will sort out embedded interview related questions, including C language, C++ and Linux operating system. Later, it will be updated one after another for in-depth analysis and discussion based on the key issues in the above three parts.
If the blog content is not rigorous or wrong, please comment or private message in time, I will add and correct it as soon as possible!
Please also give us your feedback~ Come on together!