1 Install Elasticsearch
The installations of windows and linux are similar. All operations in this chapter are based on Docker containers.
docker virtual machine IP address: 10.211.55.4
1.1 Docker image download
docker pull elasticsearch:5.6.8
1.2 Install es container
docker run -di --name=elasticsearch -p 9200:9200 -p 9300:9300 elasticsearch:5.6.8
1.3 Check whether the installation is successful
http://10.211.55.4:9200/
9200端口(Web管理平台端口) 9300(服务默认端口)
1.4 Open remote connection
elasticsearch从5版本以后默认不开启远程连接,所以现在连接是会报错的需要修改es开启远程连接的
1.4.1 Login container
docker exec -it elasticsearch /bin/bash
1.4.2 Enter the es container directory
cd /usr/share/elasticsearch/config
1.4.3 Modify the elasticsearch.yml file to the following configuration
http.host: 0.0.0.0
transport.host: 0.0.0.0
#discovery.zen.minimum_master_nodes: 1
#集群节点的名称 my-application
cluster.name: my-application
# 开启跨域
http.cors.enabled: true
# 允许哪些可以跨域
http.cors.allow-origin: "*"
# 网络地址
network.host: 10.211.55.4
1.4.4 Restart es and set it to start at boot
docker restart elasticsearch
docker update --restart=always elasticsearch
2 Install IK tokenizer
Remarks When using, including kibana, es, ik tokenizer versions must be the same to use
2.1 Download and unzip the ik tokenizer
https://github.com/medcl/elasticsearch-analysis-ik/releases
unzip elasticsearch-analysis-ik-5.6.8.zip
mv elasticsearch ik
2.2 Copy the ik directory to the plugins directory of the docker container
docker cp ./ik changgou_elasticsearch:/usr/share/elasticsearch/plugins
2.3 ik tokenizer test
访问:http://10.211.55.4:9200/_analyze?analyzer=ik_smart&pretty=true&text=我爱你中国
3 Create a Spring Boot project as follows
Remarks
controller 访问控制器
dao mybatis mapper接口
entity 自己封装的返回体
es es mapper 接口
pojo mysql和es的实体封装
servie与impl 接口与实现层
application.yml is configured as follows
server:
port: 10086
spring:
application:
name: search
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://127.0.0.1:3306/es?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
username: root
password: 123456
data:
elasticsearch:
cluster-name: my-application
cluster-nodes: 10.211.55.4:9300
ribbon:
ReadTimeout: 300000
mybatis:
configuration:
map-underscore-to-camel-case: true
mapper-locations: classpath:mapper/*Mapper.xml
type-aliases-package: com.elasticsearch.pojo
logging:
level:
com.elasticsearch.dao: debug
Project structure
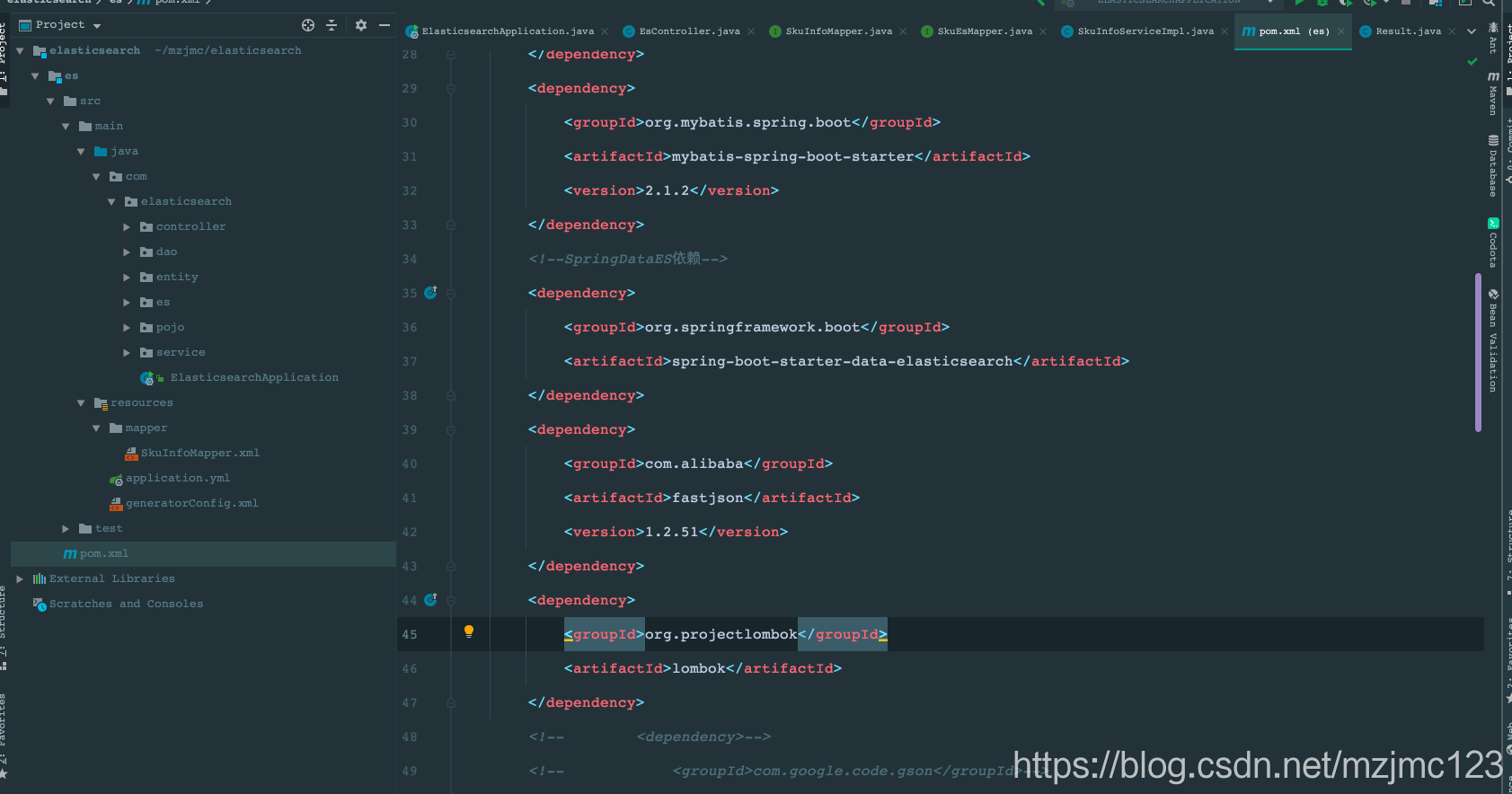
3.1 Add spring-data-es
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
3.2 Add startup class
package com.elasticsearch;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.data.elasticsearch.repository.config.EnableElasticsearchRepositories;
@SpringBootApplication
@MapperScan(basePackages = {
"com.elasticsearch.dao"})
@EnableElasticsearchRepositories(basePackages = "com.elasticsearch.es")
public class ElasticsearchApplication {
public static void main(String[] args) {
SpringApplication.run(ElasticsearchApplication.class, args);
}
}
3.2.1 Create Controller
package com.elasticsearch.controller;
import com.elasticsearch.entity.Result;
import com.elasticsearch.entity.StatusCode;
import com.elasticsearch.service.SkuInfoService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RequestMapping("/search")
@RestController
@CrossOrigin
public class EsController {
@Autowired
private SkuInfoService skuInfoService;
@GetMapping("/import")
public Result search(){
skuInfoService.importData();
return new Result(true, StatusCode.OK,"导入数据到索引库成功");
}
}
3.2.2 Create service interface
package com.elasticsearch.service;
public interface SkuInfoService {
void importData();
}
3.2.3 Create mysqlMapper and esMapper
is
package com.elasticsearch.es;
import com.elasticsearch.pojo.SkuMap;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface SkuEsMapper extends ElasticsearchRepository<SkuMap, Long> {
}
mysql
package com.elasticsearch.dao;
import com.elasticsearch.pojo.SkuInfo;
import java.util.List;
public interface SkuInfoMapper {
List<SkuInfo> findAll();
}
3.2.4 Create mysql entity and es entity
mysql entity skuinfo
package com.elasticsearch.pojo;
import lombok.Data;
import java.util.Date;
@Data
public class SkuInfo {
private Long id;
private String sn;
private String name;
private Integer price;
private Integer num;
private Integer alertNum;
private String image;
private String images;
private Integer weight;
private Date createTime;
private Date updateTime;
private Long spuId;
private Integer categoryId;
private String categoryName;
private String brandName;
private String spec;
private Integer saleNum;
private Integer commentNum;
private String status;
}
es entity skuMap
package com.elasticsearch.pojo;
import lombok.Data;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
import java.io.Serializable;
import java.util.Date;
import java.util.Map;
@Data
@Document(indexName = "skumap", type = "docs")
public class SkuMap implements Serializable {
@Id
private Long id;
@Field(type = FieldType.Text, analyzer = "ik_smart")
private String name;
@Field(type = FieldType.Integer)
private Integer price;
private Integer num;
private String image;
private String status;
private Date createTime;
private Date updateTime;
private Long spuId;
private Long categoryId;
@Field(type = FieldType.Keyword)
private String categoryName;
@Field(type = FieldType.Keyword)
private String brandName;
private String spec;
private Map<String, Object> specMap;
}
3.2.5 service implementation layer
package com.elasticsearch.service.impl;
import com.alibaba.fastjson.JSON;
import com.elasticsearch.es.SkuEsMapper;
import com.elasticsearch.dao.SkuInfoMapper;
import com.elasticsearch.pojo.SkuInfo;
import com.elasticsearch.pojo.SkuMap;
import com.elasticsearch.service.SkuInfoService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Map;
@Service
public class SkuInfoServiceImpl implements SkuInfoService {
@Autowired
private SkuInfoMapper skuInfoMapper;
@Autowired
private SkuEsMapper skuEsMapper;
@Override
public void importData() {
List<SkuInfo> list = skuInfoMapper.findAll();
List<SkuMap> skuMapList= JSON.parseArray(JSON.toJSONString(list),SkuMap.class);
for (SkuMap map : skuMapList) {
Map<String, Object> specMap = JSON.parseObject(map.getSpec(),Map.class);
map.setSpecMap(specMap);
}
skuEsMapper.saveAll(skuMapList);
}
}
4 test
4.1 Request
http://127.0.0.1:10086/search/import
4.2 View Index Library Information
Port 9100 is the external port of es-head. You can also use kibana instead. There is no explanation here.
http://10.211.55.4:9100/
You can see that there is more skumap in the index library
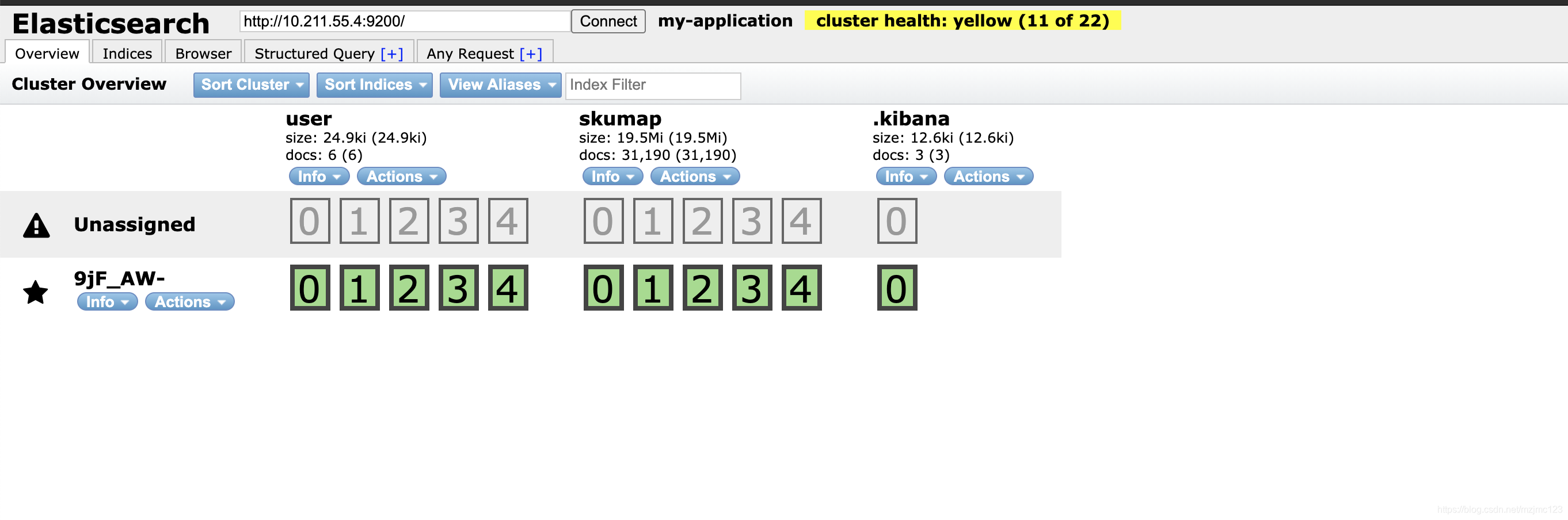
skumap data
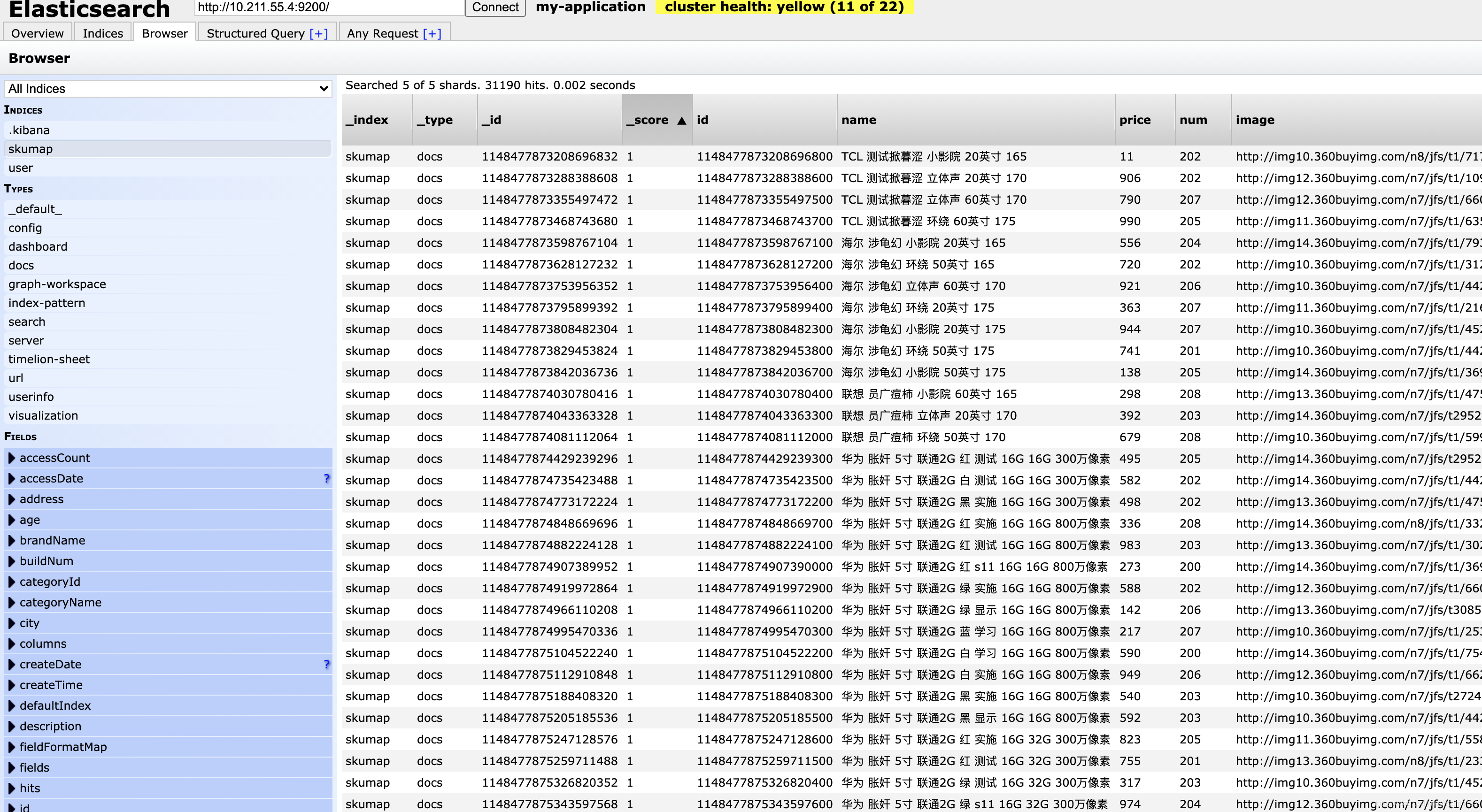
Later our operations in the index library
demo ruins
https://e.coding.net/mzjmc/elasticsearch/elasticsearch.git