1. Why cross-domain issues arise
The cross-domain problem comes from the same-origin strategy of JavaScript
That is, only if the protocol + host name + port number (if it exists) is the same, mutual access is allowed
That is to say, JavaScript can only access and operate resources in its own domain, and cannot access and operate resources in other domains.
Cross-domain issues are for JS and ajax. HTML itself has no cross-domain issues, such as a tag, script tag, or even form tag
2. What is cross-domain
In the process of accessing another Url through one Url, if
any one of their
protocol, domain name, and port
is different, it is cross-domain
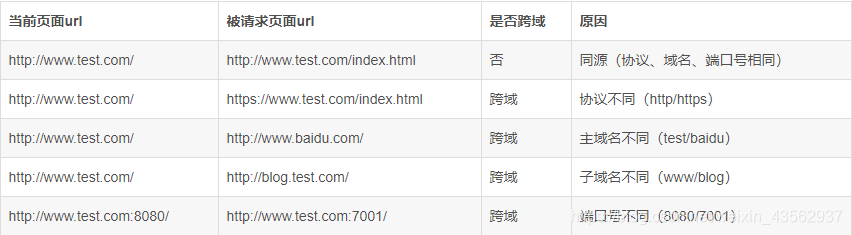
3. How to solve cross-domain
1
.
Modify browser settings
--disable-web-security--user-data-dir# Find Chrome.exe in the path and modify the settings of the browser through the previous command
2. Modification request method:
J
sonp
$("#Test").click(function(){
$.ajax({
url : 'http://www.xiaozhao.top/Index/Test/crossDomain',
data : {},
type : 'get',
dataType : 'jsonp',
success : function (result) {
alert(result);
}
});
});
Disadvantages: J sonp only supports GET requests
Only supports cross-domain HTTP requests
There may be many other restrictions...
3.CORS
1. What is CORS
CORS is a W3C standard, the full name is "Cross-origin resource sharing" (Cross-origin resource sharing)
protocol:
-
Traditional Ajax requests can only obtain resources under the same domain name, but HTML5 breaks this limitation and allows Ajax to initiate cross-domain requests.
-
Browsers can initiate cross-domain requests. For example, you can link a picture or script from a foreign domain. But the Javascript script cannot get the content of these resources, it can only be executed or rendered by the browser.
-
In Flash and Silverlight, the server needs to create a crossdomain.xml file to allow cross-domain requests. If this file declares " http://your.site " allow from " http://my.site request", it comes from " http://my.site request" access to all " HTTP: // your. site ". This is a control mode at the entire site level. Either you allow access to a site from a foreign domain or deny it.
-
COR is different, it is a page-level control mode. Each page needs to return an HTTP header named'Access-Control-Allow-Origin' to allow access to sites from outside domains. You can only expose limited resources and limited external domain site access. In the COR model, the responsibility of access control can be placed in the hands of the page developer, not the server administrator. Of course, page developers need to write special processing code to allow access by external domains.
Another major difference is that the crossdomain.xml file of a certain site is first acquired and analyzed by the browser. If a site in a foreign domain is not allowed to be accessed, the browser will not issue a cross-domain request at all.
COR is the opposite. Javascript first makes a cross-domain request, and then checks the response's'Access-Control-Allow-Origin' header. If this header allows access to the external domain, then Javascript can read the reply, otherwise access is forbidden. If the request is not a simple COR, then send a pre-check request to the external domain server. If the response header allows access, then send a cross-domain request, otherwise it is prohibited.
The implementation standard of COR is the CORS protocol.
2. Requirements
CORS requires both browser and server support. Currently, all browsers support this function, and IE browser cannot be lower than IE10
3. Request classification
Simple request (simple request) and non-simple request (not-so-simple request)
4. Solve
1. Modify the code setting header information of the server
res.setHeader('Access-Control-Allow-Origin', "*"); //For which domain name can be accessed, * means all
res.setHeader('Access-Control-Allow-Credentials', true); //Is it possible to carry cookies
res.setHeader('Access-Control-Allow-Methods', 'POST, GET, PUT, DELETE, OPTIONS');
2. Via @CrossOrigin
3. Configure via gateway