Article Directory
Assignment 2: TDD practice report
lab environment
Operating System : Mac OS
Compiler : VScode
Iterative chapter exercises
Exercise 1
- Modify the test code so that the caller can specify the number of character repetitions, and then fix the code
First test file prepared repeat_test.go
substantially the same sections of code and given only need to change Repeat()
the function of the two parameters, is the need to repeat a string, and the other is the number of repetitions, as follows
package iteration
import "testing"
func TestRepeat(t *testing.T) {
repeated := Repeat("a", 4 )
expected := "aaaaa"
if repeated != expected {
t.Errorf("expected '%q' but got '%q'", expected, repeated)
}
}
First run the test code and get the following results
You can see that the compilation will fail because the function to be tested is not written
Begin writing Repeat()
function, the function takes two parameters, a string type, and the other is an int, a method is implemented in the form of a for loop, as follows
package iteration
func Repeat(character string, count int) string {
var ret string = ""
for i := 0; i < count; i++ {
ret += character
}
return ret
}
After the test code write, compile and run, either directly in the command line VScode to go test
a command to test the results obtained are shown below
You can see the results FAIL
, because the reason I chose to test repeat 4 times, so the results are not as desired, re-test the code change, you can get the right result, it will appear in the test file PASS
as follows:
Exercise 2
- Write a
ExampleRepeat
to improve your function documentation
On the usage example, the previous chapter: integer mentioned before, directly in repeat_test.go
creating a new function ExampleRepeat
, this function is used to output the result obtained ( note : since it needs to be output at the beginning of the code need to import the fmt
package), Compare with the content in the following comments, if the same, it will succeed, otherwise it will fail. The code is as follows:
func ExampleRepeat() {
s := Repeat("a", 5)
fmt.Println(s)
// Output: aaaa
}
You can see that I set the iteration 5 times, and the output commented below is iterated four times, so I should get the wrong result, as shown below:
Re-modify the following comments to make it iterate five times, and the test results are as follows:
It can be seen from the result that the test was successful PASS
Exercise 3
- Take a look at the strings package . Find functions that you think might be useful and write some tests for them. Investing time in learning the standard library will slowly pay off.
func Compare(a, b string) int
Function, this function is used to compare the lexicographical size of the string before and after, if a <b, return -1; if a = b, return 0; if a> b, return 1, the test code file iscompare_test.go
package iteration
import (
"strings"
"testing"
)
func TestCompare(t *testing.T) {
ans := strings.Compare("h", "z")
expected := -1
if ans != expected {
t.Errorf("expected '%d' but got '%d'", expected, ans)
}
}
Then test, the results are as follows:
The result shows that the test is successful
func Count(s, substr string) int
Function, this function is to count the number of substring substr in the string s, and then return the value, the test code file iscount_test.go
package iteration
import (
"strings"
"testing"
)
func TestCount(t *testing.T) {
ans := strings.Count("cheeze", "e")
expected := 3
if ans != expected {
t.Errorf("expected '%d' but got '%d'", expected, ans)
}
}
Then test, the results are as follows:
It can be seen from the result that the test was successful
func ReplaceAll(s, old, new string) string
Function, this function replaces all old strings with new strings in the s string. The tested file isreplace_test.go
package iteration
import (
"strings"
"testing"
)
func TestReplace(t *testing.T) {
ans := strings.ReplaceAll("oink oink oink", "oink", "moo")
expected := "moo moo moo"
if ans != expected {
t.Errorf("expected '%s' but got '%s'", expected, ans)
}
}
Then test, the results are as follows:
It can be seen from the result that the test was successful
Fast sorting algorithm implementation
Algorithm introduction
Quicksort (Quicksort) is an improvement to bubble sort. The main steps of the algorithm are:
First set a boundary value, and divide the array into left and right parts by the boundary value.
Collect data greater than or equal to the cutoff value to the right of the array, and data less than the cutoff value to the left of the array. At this time, each element in the left part is less than or equal to the cutoff value, and each element in the right part is greater than or equal to the cutoff value.
Then, the data on the left and right can be sorted independently. For the array data on the left, you can also take a demarcation value to divide this part of data into left and right parts, and also place the smaller value on the left and the larger value on the right. The array data on the right can also be processed similarly.
Repeat the above process, you can see that this is a recursive definition. After recursively sorting the left part, recursively sort the right part. When the data in the left and right parts are sorted, the sorting of the entire array is completed.
Through the above description of the algorithm, first complete a main function to test whether the quick sort is successfully implemented, the code is as follows:
package main
import "fmt"
const MAXN = 10
var arr = []int{
7, 4, 8, 5, 3, 6, 9, 1, 10, 2}
// 快速排序递归实现
func QuickSort(arr []int, l, r int) {
pivot := arr[l] // 选取中间值
pos := l // 中间值的位置
i, j := l, r
for i <= j {
for j >= pos && arr[j] >= pivot {
j--
}
if j >= pos {
arr[pos] = arr[j]
pos = j
}
for i <= pos && arr[i] <= pivot {
i++
}
if i <= pos {
arr[pos] = arr[i]
pos = i
}
}
arr[pos] = pivot
if pos-l > 1 {
QuickSort(arr, l, pos-1)
}
if r-pos > 1 {
QuickSort(arr, pos+1, r)
}
}
func main() {
QuickSort(arr, 0, len(arr)-1)
for i := 0; i < MAXN; i++ {
fmt.Printf("%d\t", arr[i])
}
}
By running the entire code, you can see the result as follows:
Write test first
First write a test file QuickSort_test.go
, but not write QuickSort.go
files to see if the test file output
package Qsort
import "testing"
const MAXN = 10
var arr = []int{
7, 4, 8, 5, 3, 6, 9, 1, 10, 2}
func TestQuickSort(t *testing.T) {
QuickSort(arr, 0, MAXN-1)
expected := []int{
1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
flag := true
for i := 0; i < MAXN; i++ {
if arr[i] != expected[i] {
flag = false
}
}
if flag == false {
t.Errorf("expected ")
for i := 0; i < MAXN; i++ {
t.Errorf("%d\t", expected[i])
}
t.Errorf("but got")
for i := 0; i < MAXN; i++ {
t.Errorf("%d\t", arr[i])
}
}
}
After writing the code, run it directly and get the following results
It can be seen that due to the lack of the tested file, a compilation error will occur
Minimal code run test
Start writing the code file to be tested QuickSort.go
, so that the test can run successfully, so just write an empty code with correct syntax
package Qsort
// 快速排序递归实现
func QuickSort(arr []int, l, r int) {
}
After writing the code, test it, and the output result is as follows
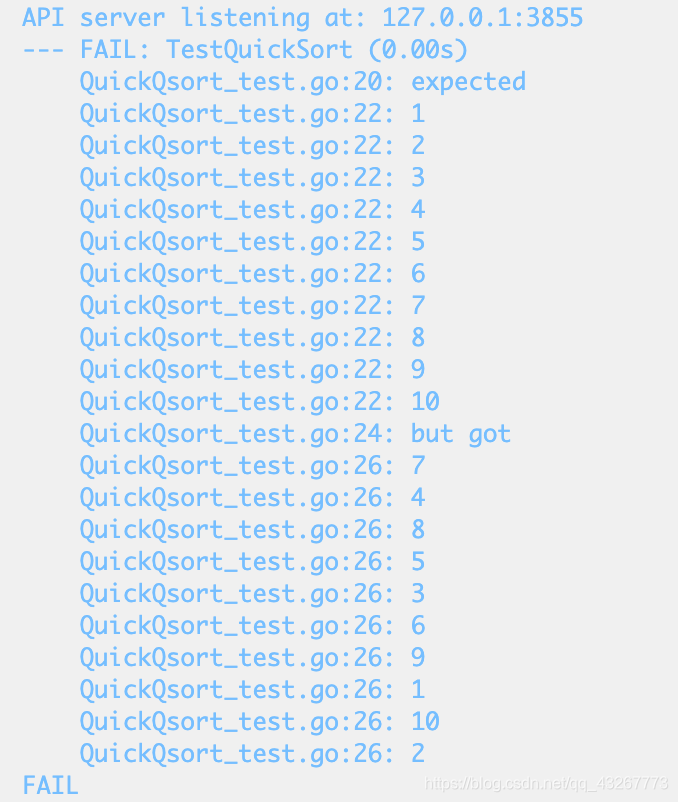
Successful code completion test
After the pre-test is over QuickSort.go
, modify the file and the modified code is
package Qsort
// 快速排序递归实现
func QuickSort(arr []int, l, r int) {
pivot := arr[l] // 选取中间值
pos := l // 中间值的位置
i, j := l, r
for i <= j {
for j >= pos && arr[j] >= pivot {
j--
}
if j >= pos {
arr[pos] = arr[j]
pos = j
}
for i <= pos && arr[i] <= pivot {
i++
}
if i <= pos {
arr[pos] = arr[i]
pos = i
}
}
arr[pos] = pivot
if pos-l > 1 {
QuickSort(arr, l, pos-1)
}
if r-pos > 1 {
QuickSort(arr, pos+1, r)
}
}
First test, change the 2 in expected in the test code to 3 to see if the test file can detect errors, the result is shown in the figure
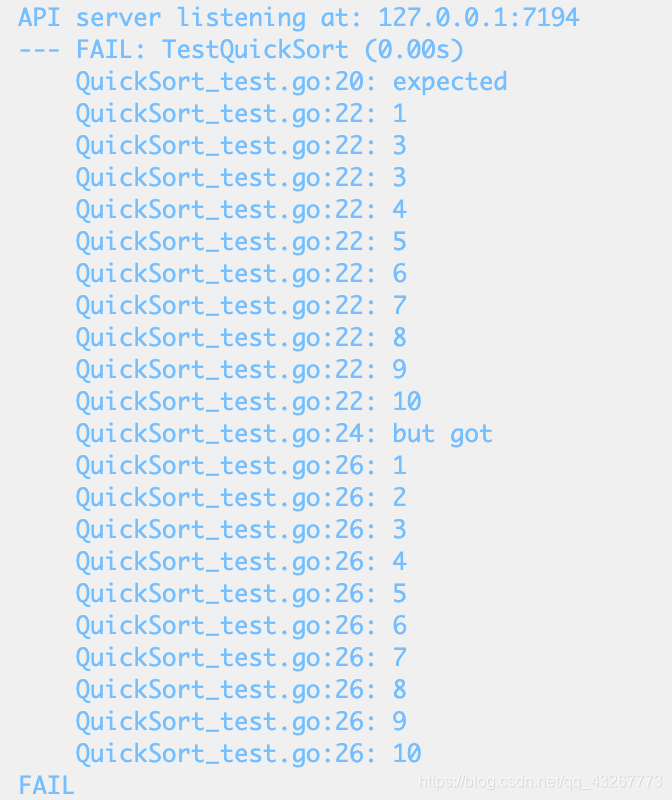
From the above figure, we can see that the test file can detect errors, and then perform the correct test. The result is
as shown in the above figure. You can see that the test is successful and passed, indicating that the quick sort code I designed is correct.
Refactor
Since the original algorithm does not have much to be modified, comments are made above the original code
Benchmarks
Write a benchmark test in the test file, make the test code run bN times, and test how long it takes, the added code is as follows
func BenchmarkQuickSort(b *testing.B) {
for i := 0; i < b.N; i++ {
var arrt = []int{
7, 4, 8, 5, 3, 6, 9, 1, 10, 2}
QuickSort(arrt, 0, MAXN-1)
}
}
Written after benchmarking code, enter the command in the command line, go test -bench=.
the time to run the test code, the result is as follows
So far, the entire testing process has been completed
github address
Code Portal: Portal
to sum up
Through this experiment, I learned the basic steps of testing, understood concepts such as TDD, refactoring, testing, benchmark testing, and further mastered some usages of the GO language. I can skillfully use it in code writing and in code writing. I also encountered some difficulties, which were solved through the learning of GO in the C language learning network .