Create grammar
var txt = new String("string");
or
var txt = "string";
String object properties
Attributes |
description |
constructor |
Reference to the function that created the object |
length |
The length of the string |
prototype |
Allows you to add properties and methods to objects |
String object methods
method |
描述 |
charAt() |
Returns the character at the specified position |
charCodeAt() |
Returns the Unicode encoding of the character at the specified position |
concat() |
Concatenate two or more strings and return a new string |
fromCharCode() |
Convert Unicode encoding to characters |
indexOf() |
Returns the first occurrence of a specified string value in a string |
lastIndexOf() |
Search string from back to front |
match() |
Find one or more regular expression matches |
replace() |
Find the matching substring in the string and replace the substring matching the regular expression |
search() |
Find the value that matches the regular expression |
slice() |
Extract a fragment of the string and return the extracted part in the new string |
split() |
Split string into string array |
substr() |
Extract the specified number of characters in the string from the starting index |
substring() |
Extract characters between two specified index numbers in a string |
toLowerCase() |
Convert string to lower case |
toUpperCase() |
Convert string to uppercase |
trim() |
Remove the white space on both sides of the string |
valueOf() |
Returns the original value of a string object |
HTML packaging method for String
方法 |
描述 |
anchor() |
Create HTML anchor |
big() |
Display strings in large font |
blink () displays the blinking string |
|
bold() |
Use bold to display strings |
fixed() |
Display string as typewriter text |
fontcolor () |
Use the specified color to display the string |
fontsize() |
Use the specified size to display the string |
italics() |
Use italics to display strings |
link() |
Display string as a link |
small() |
Use small fonts to display strings |
strike() |
Strikethrough character string |
sub() |
Display string as subscript |
sup() |
Display string as superscript |
Applications
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
</head>
<body>
<script>
var txt = "Hello World!";
document.write("<p>字体变大: " + txt.big() + "</p>");
document.write("<p>字体缩小: " + txt.small() + "</p>");
document.write("<p>字体加粗: " + txt.bold() + "</p>");
document.write("<p>斜体: " + txt.italics() + "</p>");
document.write("<p>固定定位: " + txt.fixed() + "</p>");
document.write("<p>加删除线: " + txt.strike() + "</p>");
document.write("<p>字体颜色: " + txt.fontcolor("green") + "</p>");
document.write("<p>字体大小: " + txt.fontsize(6) + "</p>");
document.write("<p>下标: " + txt.sub() + "</p>");
document.write("<p>上标: " + txt.sup() + "</p>");
document.write("<p>链接: " + txt.link("http://www.w3cschool.cc") + "</p>");
document.write("<p>闪动文本: " + txt.blink() + " (不能用于IE,Chrome,或者Safari)</p>");
</script>
</body>
</html>
String object learning mind map
Excerpt from W3Cschool website.
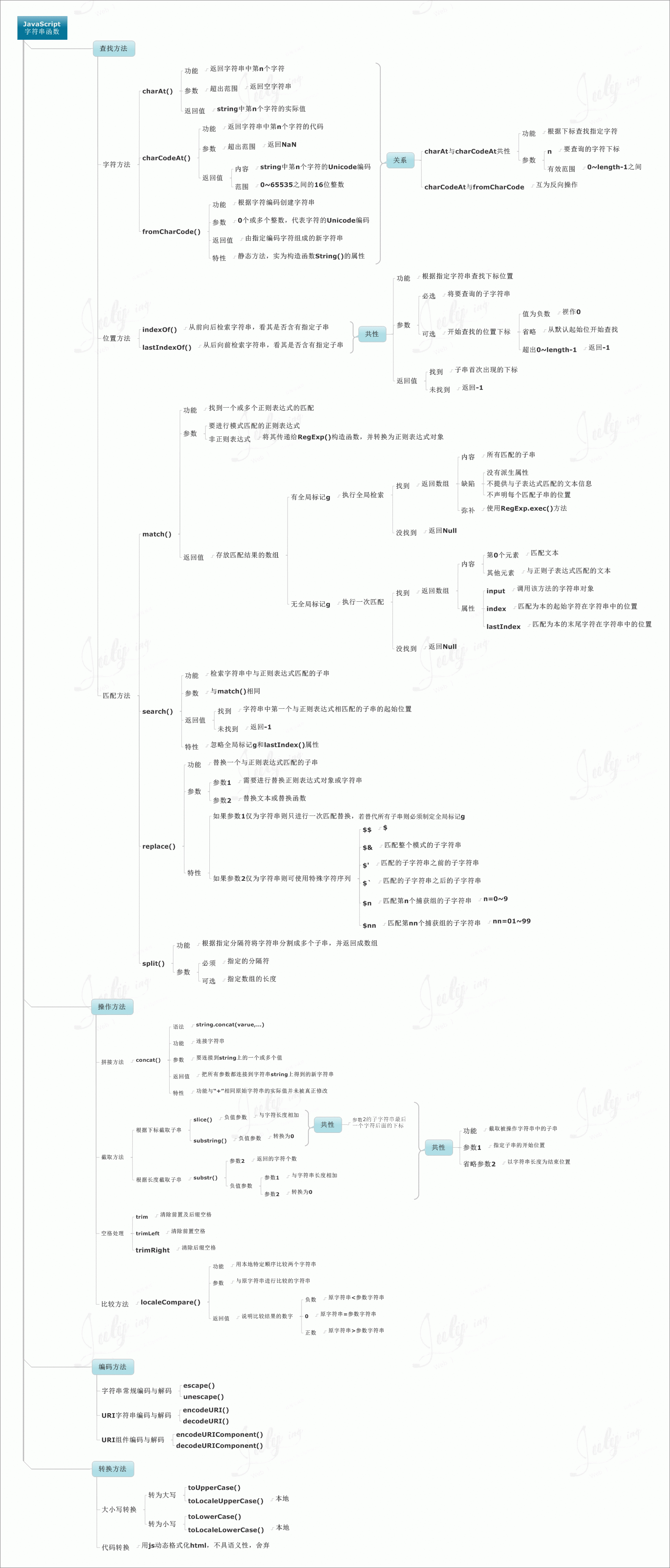