First, the use of the File class
- An object of the File class, representing a file or a file directory
- Relative path: Compared with a path, the specified path
- Absolute path: the path of the file or file directory including the drive letter
- Path separator
- windows:\\
- Unix:/
@Test
public void test1() {
File file = new File("a.txt");
System.out.println(file);
}
1.1 Common methods
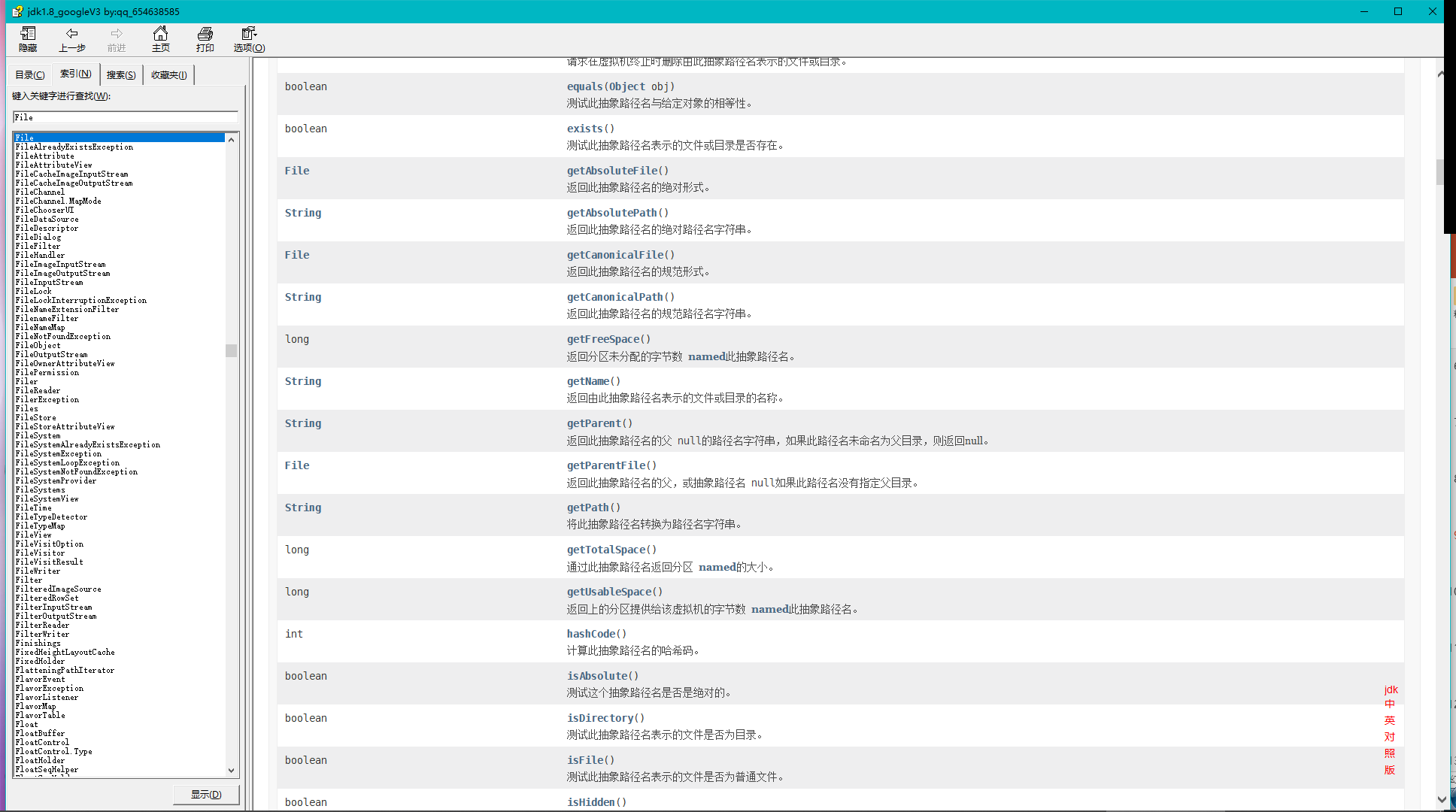
@Test
public void test2() {
File file = new File("cn/imut/hello.txt");
//获取绝对路径
System.out.println(file.getAbsoluteFile());
//获取路径
System.out.println(file.getPath());
//获取名称
System.out.println(file.getName());
//获得上层文件目录路径,无则返回null
System.out.println(file.getParent());
//获取文件长度(字节数)
System.out.println(file.length());
}
@Test
public void test3() {
File file = new File("hello.txt");
//判断是否是文件目录
System.out.println(file.isDirectory());
//判断是否是文件
System.out.println(file.isFile());
//判断是否存在
System.out.println(file.exists());
//判断是否可写
System.out.println(file.canWrite());
}
Second, the IO flow principle
- I / O is an abbreviation of Input / Output, and I / O technology is used to process data transmission between devices
- In Java programs, data input / output operations are performed in a "Stream" mode
Input input: read external data into memory
Output: output memory data to disk and CD
Third, the classification of flow
According to the data unit
- Byte stream: 8 bit (InputStream, OutputStream)
- Character stream: 16bit (Reader, Writer)
Distinguish according to the flow direction
- Input stream
- Output stream
Distinguish according to the role of the stream
- Node flow
- Processing flow
3.1 IO streaming system
classification | Byte input stream | Byte output stream | Character input stream | Character output stream |
---|---|---|---|---|
Abstract base class | InputStream | OutputStream | Reader | Writer |
Access file | FileInputStream | FileOutputStream | FileReader | FileWriter |
Access array | ByteArrayInputStream | ByteArrayOutputStream | CharArrayReader | CharArrayWriter |
Access pipeline | PipedInputStream | PipedOutputStream | PipedReader | PipedWriter |
Access string | StringReader | StringWriter | ||
Buffer stream | BufferedInputStream | BufferedOutputStream | BuffedReader | BufferedWriter |
Conversion stream | InputStreamReader | OutputStreamWriter | ||
Object stream | ObjectInputStream | ObjectOutputStream | ||
FilterInputStream | FilterOutputStream | FilterReader | FilterWriter | |
Print stream | PrintStream | PrintWriter | ||
Bounce input stream | PushbackInputStream | PushbackReader | ||
Special flow | DataInputStream | DataOutputStream |
Fourth, the file stream
File import
package cn.imut;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class IOTest {
public static void main(String[] args) {
FileReader fileReader = null;
try {
//1.File类实例化
File file = new File("D:\\Test\\IO\\hello.txt");
//2.FileReader流实例化
fileReader = new FileReader(file);
//3.读入操作
char[] c = new char[5]; //表示每次读入5个
int len;
while ((len = fileReader.read(c)) != -1) {
for (char value : c) {
System.out.print(value);
}
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fileReader != null) {
//4.资源关闭
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
File out
package cn.imut;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class IOTest2 {
public static void main(String[] args) {
FileWriter fileWriter = null;
try {
//1.提供File类对象,指明写出到的文件
File file = new File("D:\\Test\\IO\\hello.txt");
//2.提供FileWriter对象,用于数据的写出
fileWriter = new FileWriter(file);
//3.写出操作
fileWriter.write("hehe,I am you 's me");
fileWriter.write("you a sb");
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.流资源关闭
try {
assert fileWriter != null;
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Note: In the output operation, File may not exist. If it exists, the constructor used by the stream is FileWriter (file, false), and the original file will be overwritten. If it is FileWrith (file, true), the original file will not be overwritten!
package cn.imut;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class IOTest3 {
public static void main(String[] args) {
FileReader fr = null;
FileWriter fw = null;
try {
//1.创建File类的对象,指明读入和写出的文件
File srcFile = new File("D:\\Test\\IO\\hello.txt");
File destFile = new File("D:\\Test\\IO\\hello1.txt");
//2.创建输入流和输出流的对象
fr = new FileReader(srcFile);
fw = new FileWriter(destFile);
//3.数据的读入和写出操作
char[] ch = new char[5];
int len;
while ((len = fr.read(ch)) != -1) {
//每次写出 len个字符
fw.write(ch,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
assert fw != null;
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
FileInputStream
package cn.imut;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.io.IOException;
public class IOTest4 {
public static void main(String[] args) {
FileInputStream fileInputStream = null;
try {
//1.造文件
File file = new File("D:\\Test\\IO\\hello2.txt");
//2.造流
fileInputStream = new FileInputStream(file);
//3.读数据
byte[] buffer = new byte[5];
int len; //记录每次读取的字节个数
while ((len = fileInputStream.read(buffer)) != -1) {
String str = new String(buffer,0,len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.关闭资源
try {
assert fileInputStream != null;
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
to sum up
- For text files, use character streams (FileReader / Writer)
- For non-text files, use byte stream (FileInputStream / OutputStream)
Five, buffer flow
In order to improve the speed of data reading and writing, the Java API provides stream classes with buffering functions. When using these stream classes, an internal buffer array is created. By default , a buffer of 8192 bytes (8Kb) is used.
The buffer flow should be "socketed" on the corresponding node flow. According to the data operation unit, the buffer flow can be divided into
- BufferedInputStream 和 BufferedOutputStream
- BufferedReader 和 BufferedWriter
** When reading data, the data is read into the buffer in blocks, and subsequent read operations directly access the buffer **
When using BufferedInputStream to read a byte file, BufferedInputStream will read 8192 (8Kb) from the file at one time and store it in the buffer until the buffer is full before reading the next 8192 bytes from the file again. Array
When writing bytes to the stream, it will not be written directly to the file. It will first be written to the buffer until the buffer is full. BufferedOutputStream will write the data in the buffer to the file at once. Use the method flush () to force the contents of the buffer to be written to the output stream
The order of closing the stream is the reverse of the order of opening the stream. As long as the outermost flow is closed, closing the outermost flow will also close the inner node flow accordingly
Use of flush () method: manually write the contents of buffer to a file
Picture copy
package cn.imut;
import java.io.*;
public class IOTest5 {
public static void main(String[] args) {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcFile = new File("D:\\Test\\IO\\Test.jpg");
File destFile = new File("D:\\Test\\IO\\Test1.jpg");
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
BufferedInputStream bis = new BufferedInputStream(fis);
BufferedOutputStream bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[10];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
assert fis != null;
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
assert fos != null;
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Six, conversion flow
Conversion stream provides conversion between byte stream and character stream
- InputStreamReader: Convert InputStream to Reader
- OutputStreamWriter: Convert Writer to OutputStrea
When the data in the byte stream are all characters, the operation of converting to a character stream is more efficient
package cn.imut;
import java.io.*;
public class IOTest6 {
public static void main(String[] args) {
InputStreamReader isr = null;
OutputStreamWriter osw = null;
try {
File file1 = new File("D:\\Test\\IO\\hello.txt");
File file2 = new File("D:\\Test\\IO\\www.txt");
FileInputStream fis = new FileInputStream(file1);
FileOutputStream fos = new FileOutputStream(file2);
isr = new InputStreamReader(fis,"utf-8");
osw = new OutputStreamWriter(fos,"utf-8");
char[] ch = new char[20];
int len;
while ((len = isr.read(ch)) != -1) {
osw.write(ch,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
assert isr != null;
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
assert osw != null;
osw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
6.1 Common coding table
- ASCII: American Standard Information Exchange Code, which can be expressed by 7 bytes of one byte
- ISO8859-1: Latin code table, European code table, expressed in 8 bits of one byte
- GB2312: China's Chinese encoding table, up to two bytes encode all characters
- GBK: China's Chinese coding table has been upgraded to incorporate more Chinese text symbols. Up to two byte encoding
- Unicode: International Standard Code, which combines all the characters currently used by humans. Assign a unique character code to each character. All text is represented by two bytes
- UTF-8: variable-length encoding, 1-4 bytes can be used to represent a character
6.2 Object serialization
The object serialization mechanism allows Java objects in memory to be converted into a platform-independent binary stream, which allows the binary stream to be persistently stored on disk, or transmitted to another network node through the network. When other programs obtain this binary stream, they can be restored to the original Java object
Serialization advantage is that any object that implements Serializable interface be converted to bytes of data , it can be saved and restored during transmission
Serialization is a mechanism that must be implemented for parameters and return values of RMI (Remote Method Invoke) procedures, and RMI is the foundation of JavaEE. Therefore, the serialization mechanism is the foundation of the JavaEE platform
If you need an object to support the serialization mechanism, you must make the class to which the object belongs and its attributes serializable. To make a class serializable, the class must implement one of the following two interfaces. Otherwise, a NotSerializableException will be thrown
- Serializable
- Externalizable
Classes that implement the Serializable interface have a static variable that represents the serialized version identifier
- private static final long serialVersionUID
- serialVersionUID is used to indicate compatibility between different versions of the class. In short, the purpose is to serialize objects for version control, and whether the versions are compatible when deserialized
- If the class does not explicitly define this static constant, its value is automatically generated by the Java runtime environment based on the internal details of the class. If the instance variable of the class is modified, serialVersionUID may change , so it is recommended to declare it explicitly!
Simply put, Java's serialization mechanism verifies version consistency by determining the serialVersionUID of the class at runtime. During deserialization, the JVM will compare the serialVersionUID in the incoming byte stream with the serialVersionUID of the corresponding local entity class. If they are the same, they are considered to be consistent and can be deserialized. Inconsistent exception. (InvalidCastException)
7. Standard input and output streams
System.in and System.out represent the system's standard input and output devices, respectively
The default input device is: keyboard, the output device is: display
** The type of System.in is InputStream, the type of System.out is PrintStream, which is a subclass of OutputStream, a subclass of FilterOutputStream **