Case of this article: Create a brand new table minfo with the user name (name) found from the user table (sys_user) as the new column name.
After understanding the requirements, start writing stored procedures.
The first step: open the PL / SQL visualization tool, find "Procedures" from the lower left side, right-click the "Procedures" folder, click "New ...", the following pop-up window appears
Step 2: Write the name of the stored procedure (Hump Naming Rules) and the required parameters (optional)
After writing the information, click "OK" and a new stored procedure will appear, as shown below
The third step: write a stored procedure (the code behind contains comments to facilitate understanding)
create or replace procedure testFunction(officeId VARCHAR2) is
str1 varchar2(4000); --用于存放 获取原数据的SQL语句
str2 varchar2(200); --用于存放 原数据中的单个元素
ds sys_refcursor; --游标,指针用于循环【相当于for循环中的下标】
createsql varchar2(4000); --用于存放 创建新表的SQL语句
a number; --用于 计数某表是否存在于数据库中
dl varchar2(4000); --用于存放 删除的SQL语句
dorpsql varchar2(4000); --用于存放 查询表是否存在的SQL语句
begin
DBMS_OUTPUT.ENABLE(buffer_size => null);
str1:='SELECT DISTINCT name from sys_user where office_id ='''||officeId||'''';
dl:='drop table minfo';
createsql:='create table minfo(合计 varchar2(4000)';
open ds for str1; --将获取到的原数据进行循环,ds作为过渡元素使用
LOOP
FETCH ds INTO str2; --将ds中的结果依次插入到str2中
EXIT WHEN ds%NOTFOUND; --没有数据的时候,循环结束
createsql:=createsql||','||str2||' varchar2(4000)'; --拼接字符串【原数据中的元素】
END LOOP;
CLOSE ds;
createsql:=createsql||')';
dorpsql:='select count(*) from user_tables where TABLE_NAME =upper(''minfo'')'; --查询表是否存在
execute immediate dorpsql into a; --将查询是否存在的结果赋值给计数变量a
if a = 1 then --表 minfo 存在
execute immediate dl; --执行删除语句
end if;
dbms_output.put_line(createsql); --打印 创建表结构 的语句
execute immediate createsql; --执行创建新表的语句
end testFunction;
Step 4: After writing, execute the stored procedure: click the button marked in red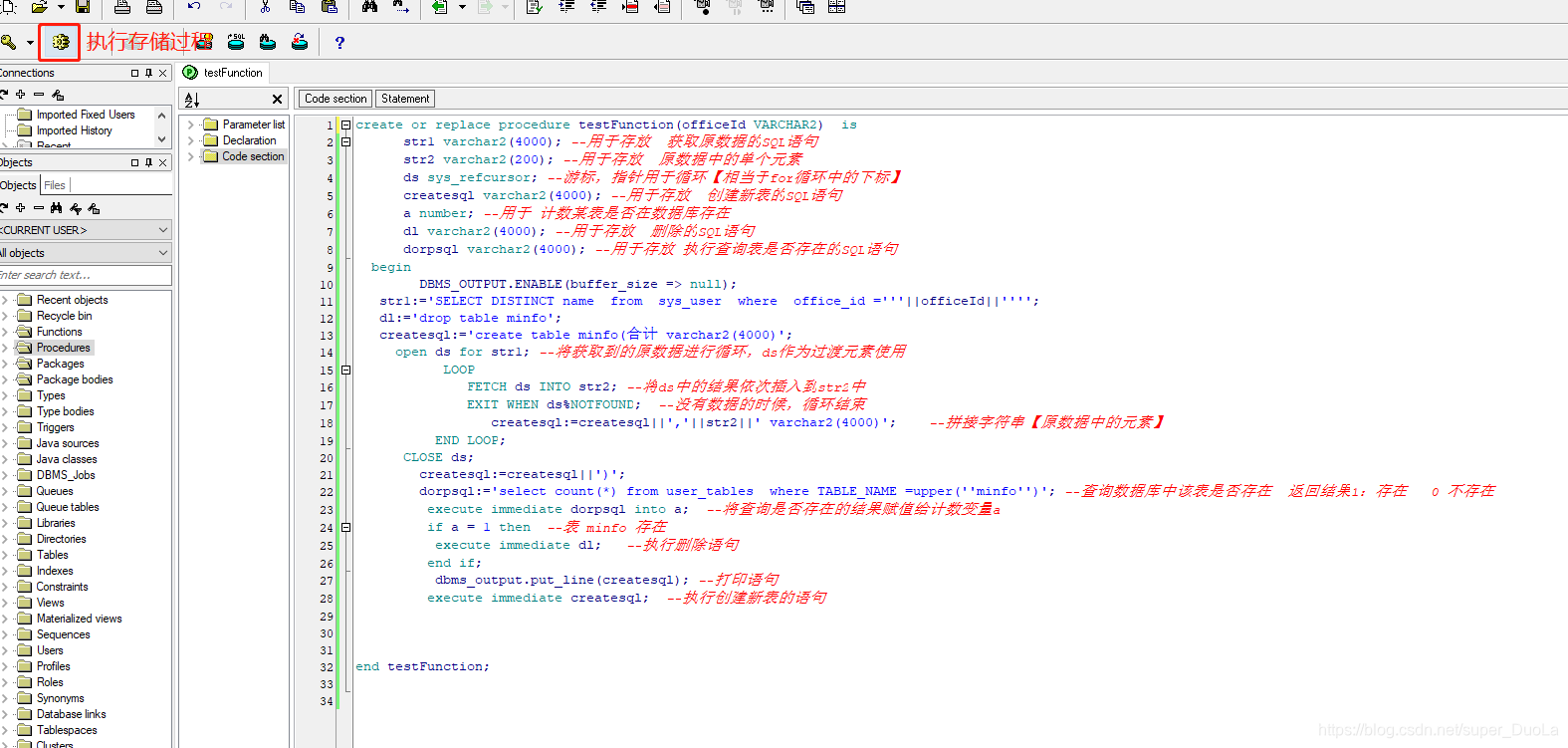
The fifth step: call the stored procedure
(1) Call in the database
--语法 call 方法名称(参数)
call testFunction('dea06cee037844cb8ccc1a5a2d29fb92')
(2) Call in the XML file
<select id="mysql" statementType="CALLABLE" parameterType="map" resultType="java.util.Map">
{call testFunction(#{officeId,jdbcType=VARCHAR,mode=IN})}
</select>
The sixth step: view the final result, the user name as a column to create a new table minfo successfully
PS: Some additional explanations for the examples
1. Check whether a table exists in the database [count (*) = 1, exists; equal to 0: does not exist]
select count(*) from user_tables where TABLE_NAME =upper(表名)
2. The upper () function: convert lowercase characters to uppercase characters
3. DISTINCT: returns a unique value, equivalent to group by
4. Unlimited output: When the content to be output is too large, an error will be reported, and this syntax can be avoided. buffer_size: The maximum number of buffer processing elements. [Probably meaning, no deep research]
DBMS_OUTPUT.ENABLE(buffer_size => null);
5. Print information, output content: equivalent to System.out.println () in Java code;
dbms_output.put_line(要输出的变量); --打印语句
The output result in the example is 'SQL statement to create a table structure': After executing the SQL statement, click "Output " to view
6. Query the original data
7. There are only single quotation marks in the storage process : if multiple places need to be escaped , that is, two '' will be escaped into one ' . There are two places in the example, the same color is marked as a pair
(1) Query statement, "||": splicing ; red mark: two single quotes will be escaped into one
(2) The two single quotation marks of the green logo will be escaped into one during execution
So far: This case has been realized and ended. The explanations / notes in the article are based on personal understanding. If there is anything wrong, thanks for correcting me!