Canvas --- overview + simple example
If we understand Canvas in a popular way, we can understand that it is similar to the drawing tool that comes with our computer. Canvas first selects a canvas, then depicts what we want to draw on this canvas, and displays it to users after drawing .
Of course, it can not only draw dynamic pictures, but even draw 3D effects.
1. Overview of canvas
1. What is canvas
Canvas is a new tag provided by HTML5.
<canvas></canvas>
The canvas tag is just a graphics container, equivalent to a canvas, and the canvas element itself has no drawing ability. All drawing work must be done inside JavaScript, which is equivalent to using a brush to draw on the canvas.
Canvas has multiple methods for drawing paths, rectangles, circles, characters, and adding images.
2. Features of canvas
1. Interactivity : Canvas supports interaction and can respond well to user operations. We can monitor keyboard, mouse, and touch device related events through Javascript.
2. Animation : Any graphics drawn by canvas can be animated, and simple bouncing balls or complex HTML5 games can be implemented.
3. Flexibility : developers can use canvas to draw any content, such as straight lines, graphics, text, pictures, etc., which can include animation or not. At the same time you can add audio or video browser support.
4. Popularity : canvas is currently very popular, and many developers use it to develop similar games or drawing applications.
5.web standard : only need a browser to run, instead of flash or silverlight, you need to install related plug-ins.
3. Canvas application field
1. Visualized data : various statistical charts, such as Baidu's echart
2. Scene show : using canvas to achieve dynamic advertising effects can run very harmoniously across platforms. Such as: mobile phone micro-products. Mobile compatibility is very good.
3. Games : canvas is more three-dimensional and more compact than Flash in web-based image display, and canvas has become the first choice for HTML5 game development.
4. Other content that can be embedded in the website (mostly used for active pages, special effects) : like charts, audio, video, and many other elements can better integrate with the Web, and does not require any plug-ins.
5. Trend => Simulator : Whether in terms of visual effects or core functions, the simulator product can be completely implemented by JavaScript. Simulate real hardware environment, such as various types of mobile phones.
6. Trend => Remote computer control : Canvas allows developers to better implement Web-based data transmission and build a perfect visual control interface.
7. Trend => Graphic Editor : Photoshop graphic editor will be 100% web-based.
2. Basics of canvas drawing
1. Draw the canvas
<body>
<!--1.准备画布 画布默认白色的 默认长宽300*150-->
<!--1.1 设置画布的大小 width="600" height="400" -->
<canvas width="600" height="400" ></canvas>
<!--2.准备绘制工具-->
<script>
/*1.获取元素*/
var myCanvas = document.querySelector('canvas');
/*2.获取上下文 绘制工具箱 2d代表是2d效果 3d就是指3d效果 3d只有IE11才支持*/
var ctx = myCanvas.getContext('2d');
</script>
</body>
context is an object that encapsulates a lot of drawing functions. After we create a canvas tag on the page, we must first use getContext () to get the context of the canvas. The getContext ("2d") object is inside
The built HTML5 object has a variety of ways to draw paths, rectangles, circles, characters and add images.
The above code we get 2D context through canvas. In the 2D structure of HTML5 Canvas, the coordinates (0,0) are on the upper left, which is not the same as traditional coordinates. Everyone needs to pay attention, as shown in the following figure:
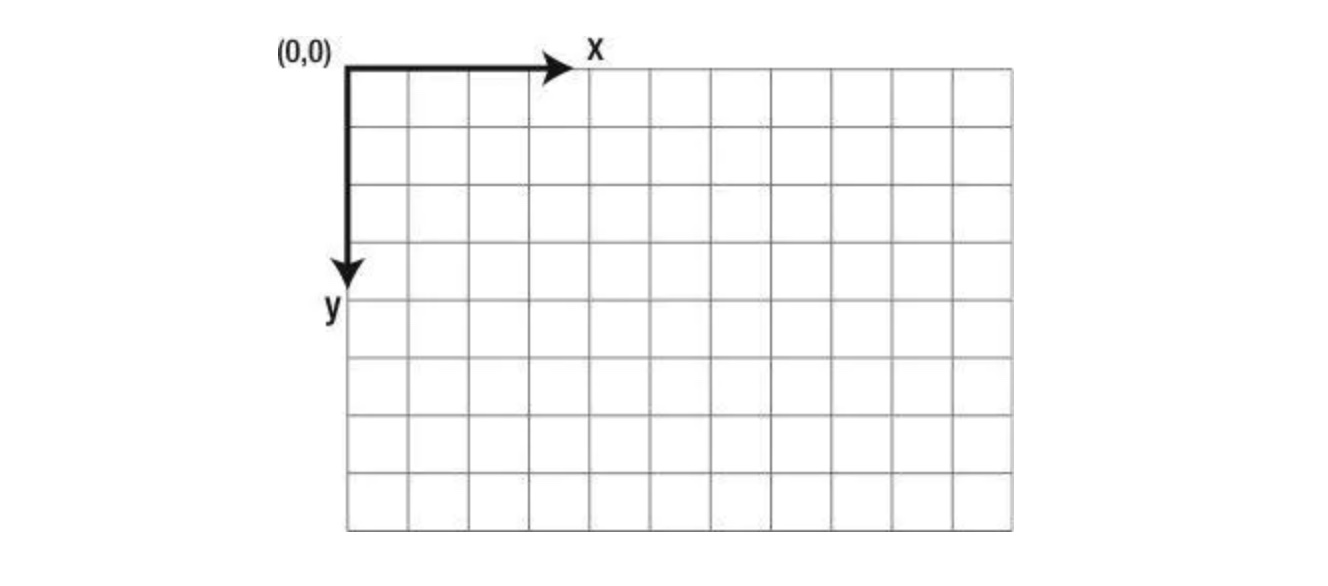
2. Draw the basic path
1), draw starting point (moveTo)
* 语法:ctx.moveTo(x, y);
* 解释:设置上下文绘制路径的起点。相当于移动画笔到某个位置
* 参数:x,y 都是相对于 canvas盒子的最左上角。
* 注意:**绘制线段前必须先设置起点,不然绘制无效。**
2), draw a straight line (lineTo)
* 语法:ctx.lineTo(x, y);
* 解释:从x,y的位置绘制一条直线到起点或者上一个线头点。
* 参数:x,y 线头点坐标。
3), path start and close
* 开始路径:ctx.beginPath();
* 闭合路径:ctx.closePath();
* 解释:如果复杂路径绘制,必须使用路径开始和结束。闭合路径会自动把最后的线头和开始的线头连在一起。
* beginPath: 核心的作用是将 不同绘制的形状进行隔离,
每次执行此方法,表示重新绘制一个路径,跟之前的绘制的墨迹可以进行分开样式设置和管理。
4) Stroke
* 语法:ctx.stroke();
* 解释:根据路径绘制线。路径只是草稿,真正绘制线必须执行stroke
5). Summarize
the basic steps of canvas drawing:
第一步:获得上下文 =>canvasElem.getContext('2d');
第二步:开始路径规划 =>ctx.beginPath()
第三步:移动起始点 =>ctx.moveTo(x, y)
第四步:绘制线(矩形、圆形、图片...) =>ctx.lineTo(x, y)
第五步:闭合路径 =>ctx.closePath();
第六步:绘制描边 =>ctx.stroke();
Three, draw line example
1. Draw a line
Code
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
canvas{ border: 2px solid #00CED1; }
</style>
</head>
<body>
<canvas width="300" height="200" ></canvas>
<script>
/*1.获取元素*/
var myCanvas = document.querySelector('canvas');
/*2.获取上下文 绘制工具箱 */
var ctx = myCanvas.getContext('2d');
/*3.移动画笔*/
ctx.moveTo(100,100);
/*4.绘制直线 (轨迹,绘制路径)*/
ctx.lineTo(200,100);
/*5.描边*/
ctx.stroke();
</script>
</body>
</html>
running result
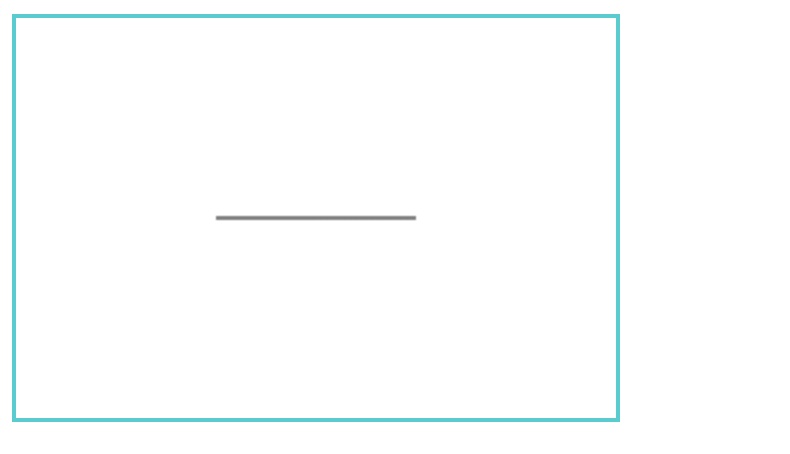
2. Draw parallel lines
Code
/*上面代码部分省略*/
/*第一条线*/
ctx.moveTo(100,100);
ctx.lineTo(200,100);
/*第二条线*/
ctx.moveTo(100,150);
ctx.lineTo(200,150);
/*描边*/
ctx.stroke();
operation result
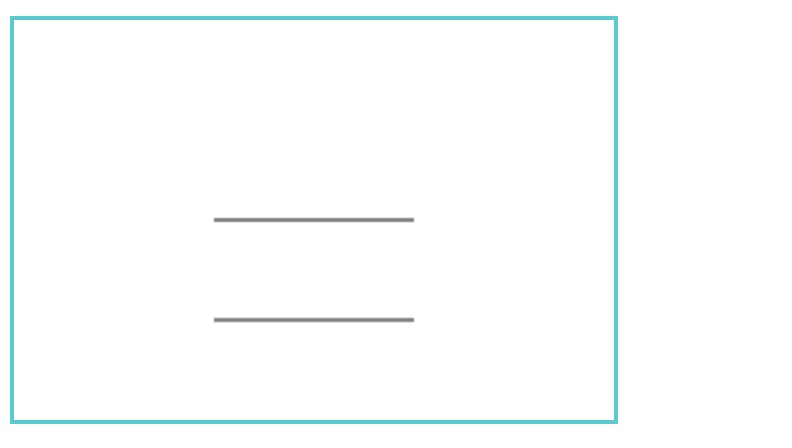
3. Draw a triangle
Code
/*1.绘制一个三角形*/
ctx.moveTo(100,100);
//第一条线
ctx.lineTo(150,100);
//第二条线
ctx.lineTo(150,150);
/*第三条线使用canvas的自动闭合 */
ctx.closePath();
//修改宽度
ctx.lineWidth = 5;
/*2.描边*/
ctx.stroke();
operation result
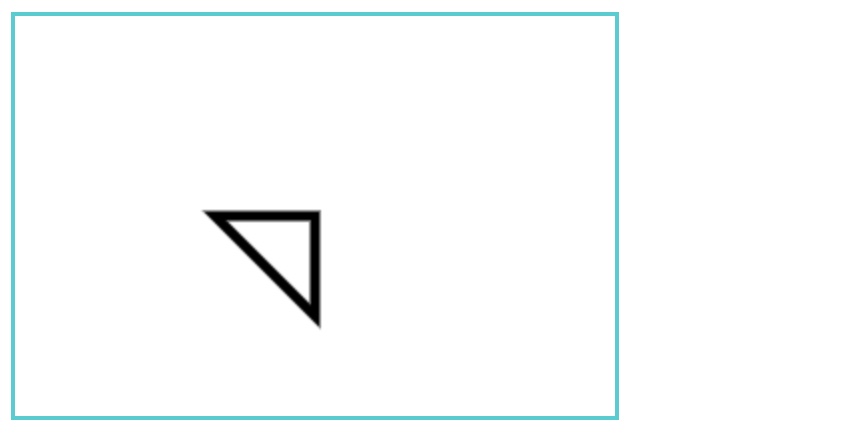
4. Draw a square
Code
/*1.绘制两个正方形 一大200一小100 套在一起*/
ctx.moveTo(100,100);
ctx.lineTo(300,100);
ctx.lineTo(300,300);
ctx.lineTo(100,300);
ctx.closePath();
//第二个正方形
ctx.moveTo(150,150);
ctx.lineTo(150,250);
ctx.lineTo(250,250);
ctx.lineTo(250,150);
ctx.closePath();
/*2.去填充*/
//ctx.stroke();
ctx.fillStyle = 'red';
ctx.fill();
operation result
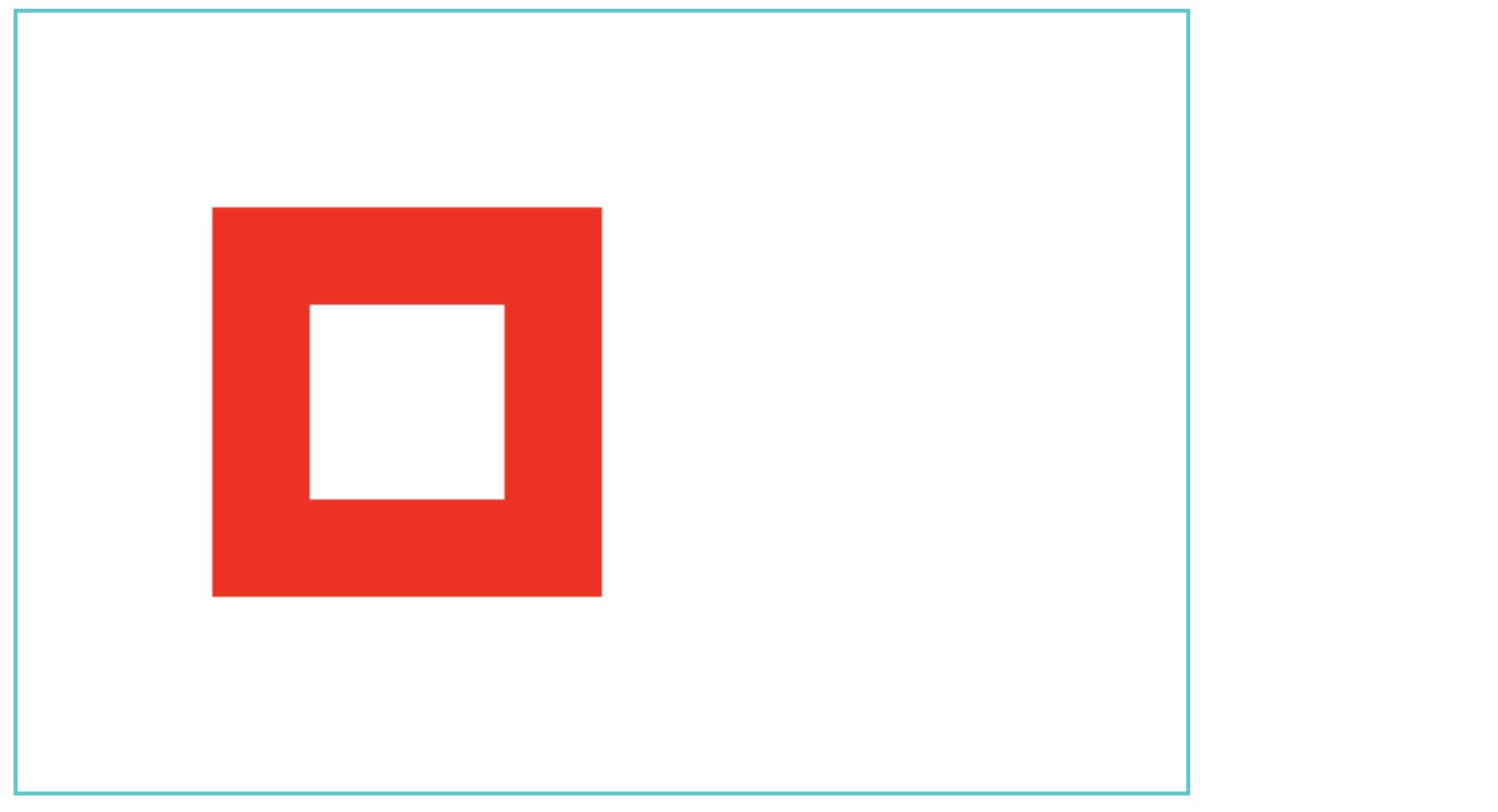
重点
: Follow when filling in the above 非零环绕规则
, this sentence is very understandable to understand, not very easy to explain, you can refer to this article Canvas tutorial (21)-non-zero wrapping
5. Set the style
Here are some commonly used methods to set the style, without specific examples.
- lineWidth 线宽,默认1px
- lineCap 线末端类型:(butt默认)、round、square
- lineJoin 相交线的拐点 miter(默认)、round、bevel
- strokeStyle 线的颜色
- fillStyle 填充颜色
- setLineDash() 设置虚线
- getLineDash() 获取虚线宽度集合
- lineDashOffset 设置虚线偏移量(负值向右偏移)
reference
3. Canvas from entry to change
别人骂我胖,我会生气,因为我心里承认了我胖。别人说我矮,我就会觉得好笑,因为我心里知道我不可能矮。这就是我们为什么会对别人的攻击生气。
攻我盾者,乃我内心之矛(9)
)。