If your knowledge of "agency model" is not clear, you can look at my other blog post - proxy mode , thank you
Two kinds of agents model structure (the difference between the two models?):
(1) static agent in before the program runs on pre-written proxy class, you can write by hand can also use the tool to generate, the disadvantage is that each business class should correspond to a proxy class, especially not flexible nor easy, so there dynamic proxies.
(2) dynamic proxy is a program run during a dynamic proxy object and build a mechanism for dynamic invocation proxy approach.
Dynamic proxy implementations?
There implementations JDK Proxy and CGLib (Code Generation Library ) two kinds.
JDK Proxy and CGLib difference?
(1) JDK Proxy is a Java language comes with features, without implementing third-party classes by loading; and CGLib is a tool provided by third parties.
(2) different implementations
- JDK Proxy through the realization of plus reflected interceptors way , only the proxy class inheritance interface , implementation, and calls relatively simple;
- CGLib is based on the ASM ( a Java bytecode manipulation frame) implemented by the interface need not be realized, which is to complete the call by implementation subclass , the performance is relatively high;
Dynamic proxy usage scenarios What?
Common usage scenarios dynamic proxy RPC an encapsulation frame, AOP (aspect-oriented programming) implemented, JDBC connection and the like.
Spring dynamic proxy is achieved by what means?
Spring framework uses two simultaneous dynamic proxy JDKProxy and CGLib, when Bean implements the interface when, Spring will use the JDK Proxy, CGLib will be used in the absence of implementation of the interface, we can also specify mandatory use CGLib in configuration only Spring configuration need to add <aop: aspectj-autoproxy proxy-target-class = "true" /> to.
JDK Proxy
JDK Proxy achieved without reference to third-party dynamic proxy class, only need to implement InvocationHandler interface rewrite invoke () method can be.
The example code
First, we assume that a service scenario, the user needs to implement CRUD operations, so we created a UserService UserServiceImpl interfaces and implementation class.
The user interface
/ ** * @author Gators Java programmer * @Since 1.0.0 * / public interface UserService { / ** * Add user * / void addUser (); / ** * delete users * / void updateUser (); }
Users implementation class
/ ** * @author Gators Java programmer * @Since 1.0.0 * / public class UserServiceImpl the implements UserService { public void the addUser () { System.out.println ( "Add a user" ); } public void the updateUser () { System.out.println ( "update a user" ); } }
Acting Class
/ ** * the JDK Proxy * @author Gators Java programmer * @Since 1.0.0 * / public class JdkDynamicProxy the implements InvocationHandler { / ** * proxy object * / Private Object target; / ** * Gets the proxy object * * JDk help us to achieve a dynamic proxy, using newProxyInstance (ClassLoader loader, class <?> [] in the interfaces, InvocationHandler H) * loader ,: ClassLoader current target object using the specified class loader * class <?> [] interfaces ,: Acting class needs to implement the interface list * InvocationHandler h: call handler, the method of the target object assigned to the call handler * @param target *@return * / public Object the getInstance (Object target) { the this .target = target; // acquired proxy object return the Proxy.newProxyInstance (target.getClass () getClassLoader (), target.getClass () The getInterfaces (),.. the this ) ; } / ** * proxy execution method * @param proxy proxy object * @param method proxy method * @param args parameter method * @return * @throws a InvocationTargetException * @throws IllegalAccessException * * / public Object Invoke (Object Proxy, Method, Method, Object [] args) throws a InvocationTargetException, IllegalAccessException { // extended functionality, before the dynamic proxy service processing. System.out.println ( "logs into the front ------ ------------- " ); // calling the method Object the Result = Method.invoke (target, args); // . implement business processing extensions, dynamic proxies after System.out. println ( "------ rear insert log -------------" ); return Result; } }
Test category
/** * @author 佛大Java程序员 * @since 1.0.0 */ public class JdkDynamicProxyTest { public static void main(String [] args) { // 执行 JDK Proxy JdkDynamicProxy dynamicProxy = new JdkDynamicProxy(); UserService userService = (UserService) dynamicProxy.getInstance(new UserServiceImpl()); userService.addUser(); userService.updateUser(); } }
operation result:
It can be seen JDK Proxy to achieve the core dynamic proxy is to achieve Invocation interface, we see Invocation source code, you will find there is only one invoke () method, the source code is as follows:
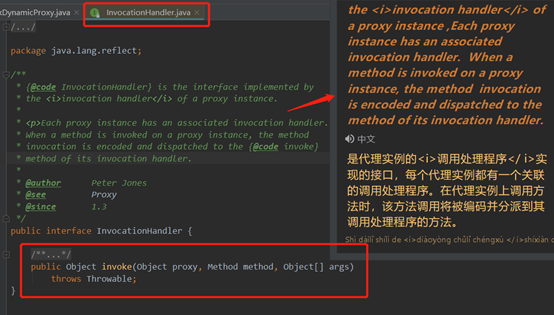
This is because there is an important role that is the agent in a dynamic proxy, which is a proxy for the unified management of the object, it is clear that this InvocationHandler agent, and invoke () method is to perform the method of triggering agents, by implementing our Invocation Interface to have the ability to dynamically proxy.
CGLib implementation
Before using CGLib, we first introduced CGLib framework in the project, add the following configuration in pom.xml:
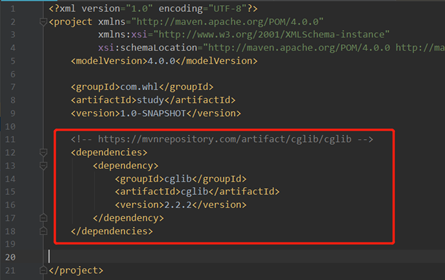
Sample code:
Description: JDK Proxy uses the above example, user a user interface implementation classes and user classes UserService UserServiceImpl
Acting Class
/ ** * @author Gators Java programmer * @Since 1.0.0 * * / public class CGLibProxy the implements MethodInterceptor { // proxy object Private Object target; public Object getInstance (Object target) { the this .target = target; Enhancer Enhancer = new new Enhancer (); // set the parent class instance class enhancer.setSuperclass ( the this .target.getClass ()); // set the callback method enhancer.setCallback ( the this); // create a proxy object (subclass object creates and returns) return enhancer.create (); } public Object Intercept (Object O, Method, Method, Object [] Objects, MethodProxy methodProxy) throws the Throwable { System.out.println ( "service processing method called before" ); // executing the call Object Object = methodProxy.invokeSuper (O, Objects); System.out.println ( "call back service processing method, the print log look" ); return Object; } }
Test category
/ ** * @author Gators Java programmer * @Since 1.0.0 * / public class CGLibProxyTest { / ** * method of execution CGLib call * @param args * / public static void main (String [] args) { / / Create CGLib proxy class CGLibProxy cgLibProxy = new new CGLibProxy (); // initialize the proxy object UserService that userService = (UserService) cgLibProxy.getInstance ( new new UserServiceImpl ()); // perform a method userService.addUser (); userService.updateUser (); } }
operation result:
CGLib implementation code and JDKProxy relatively similar, are achieved by an interface agent, and then invoke a method of a dynamic proxy completed, the only difference is that, when the initialization is CGLib class of agents, the object is achieved by the Enhancer proxy object to is a subclass of the proxy class to implement dynamic proxy. Therefore the agent class can not be modified keyword final, if the final modified, re-use will complain Enhancer set the parent class, build dynamic proxy will fail.
Common interview questions
(1) How to achieve dynamic proxy? JDK Proxy and CGLib What is the difference?
(2) dynamic and static proxy proxy What is the difference?
(3) dynamic proxy usage scenarios What?
(4) Spring dynamic proxy is achieved by what means?
Reference / Good Man
Java source code 34 interview Zhenti and say -
https://kaiwu.lagou.com/course/courseInfo.htm?courseId=59
Raindrops name - Design Patterns proxy mode
https://www.cnblogs.com/qdhxhz/p/9241412.html
Nuggets - three kinds agency model - understood Spring Aop
https://juejin.im/post/5cea0180e51d4550bf1ae7db#heading-3