1. string initialization
#include <iostream>
#include <string>
using namespace std;
// 函数外部默认初始化
string s1_0;
int i1_0;
int main()
{
// 函数内部默认初始化
string s1_1; // string是类,类各自绝对决定初始化方式,如string未初始化则默认生成空串
int i1_1; //内置类型在函数内部未初始化,则其值未定义
// 拷贝初始化(含=)
string s2_0 = "s2";
string s2_1 = {"s2"};
string s2_2 = s2_0;
// 直接初始化
string s3_0{"s3"};
string s3_1("s3");
string s3_2(3, 's');
// 拓展
string s4_0 = string(5, 's');
string s4_1 = '1' + "23"; // 不会报错,但结果错误。加号两边至少有一边是string
string s4_2 = s1_0 + '1' + "23";
cout << "s1_0=" << s1_0 << endl;
cout << "s1_1=" << s1_1 << endl;
cout << "i1_0=" << i1_0 << endl;
cout << "i1_1=" << i1_1 << endl;
cout << "----------------------------------------------------" << endl;
cout << "s2_0=" << s2_0 << endl;
cout << "s2_1=" << s2_1 << endl;
cout << "s2_2=" << s2_2 << endl;
cout << "----------------------------------------------------" << endl;
cout << "s3_0=" << s3_0 << endl;
cout << "s3_1=" << s3_1 << endl;
cout << "s3_2=" << s3_2 << endl;
cout << "----------------------------------------------------" << endl;
cout << "s4_0=" << s4_0 << endl;
cout << "s4_1=" << s4_1 << endl;
cout << "s4_2=" << s4_2 << endl;
cout << "----------------------------------------------------" << endl;
return 0;
}
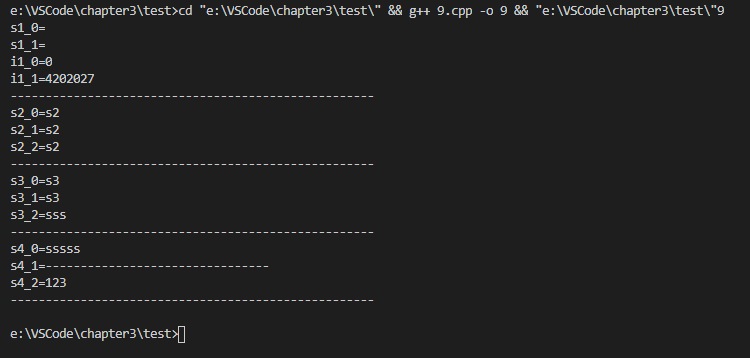
2. vector initialization
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int cout_vi(string name, vector<int> vi);
int cout_vs(string name, vector<string> vs);
// 函数外部默认初始化,vector<int>默认初始化时不含任何元素
vector<int> vi1_0;
int main()
{
// 函数内部默认初始化,vector<int>默认初始化时不含任何元素
vector<int> vi1_1;
// 拷贝初始化
vector<string> vs2_0 = {"ab", "cd", "efg"};
vector<string> vs2_1 = vs2_0;
// 直接初始化
vector<string> vs3_0{"1", "23", "456"}; // 等价于vector<string> vs3_0 = {"1", "23", "456"};
// vector<string> vs3_1("1", "23", "456");//列表初始化只能用{}
vector<string> vs3_2(vs3_0);
vector<string> vs3_3(3); // vector含3个string,string默认初始化
vector<string> vs3_4(3, "1"); // vector含3个string,string="1"
// 拓展
vector<string> vs4_0{"abc"}; // vector只有1个string,string="abc"
// vector<string> vs4_1("abc"); // 错误,注意string s3_1("s3");是可行的
vector<string> vs4_2{4}; //4是int,int与string不匹配,故vector有4个默认的string。 若是vector<int> vi{4};,类型匹配,vector有1个int, 其值为4
vector<string> vs4_3(4); // vector有4个默认的string
vector<string> vs4_4{4, "a"}; //4是int,int与string不匹配,故vector有4个string。"a"是string,类型匹配,故4个string的值都为"a"
vector<string> vs4_5(4, "b"); // vector有4个string,其值都为"b"
cout_vi("vi1_0", vi1_0);
cout_vi("vi1_1", vi1_1);
cout << "----------------------------------------------------" << endl;
cout_vs("vs2_0", vs2_0);
cout_vs("vs2_1", vs2_1);
cout << "----------------------------------------------------" << endl;
cout_vs("vs3_0", vs3_0);
// cout_vs("vs3_1", vs3_1);
cout_vs("vs3_2", vs3_2);
cout_vs("vs3_2", vs3_3);
cout_vs("vs3_3", vs3_4);
cout << "----------------------------------------------------" << endl;
cout_vs("vs4_0", vs4_0);
// cout_vs("vs4_1", vs4_1);
cout_vs("vs4_2", vs4_2);
cout_vs("vs4_3", vs4_3);
cout_vs("vs4_4", vs4_4);
cout_vs("vs4_5", vs4_5);
cout << "----------------------------------------------------" << endl;
return 0;
}
int cout_vi(string name, vector<int> vi)
{
cout << name << "=";
for (auto a : vi)
{
cout << a << " ";
}
cout << endl;
return 0;
}
int cout_vs(string name, vector<string> vs)
{
cout << name << "=";
for (auto a : vs)
{
cout << a << " ";
}
cout << endl;
return 0;
}
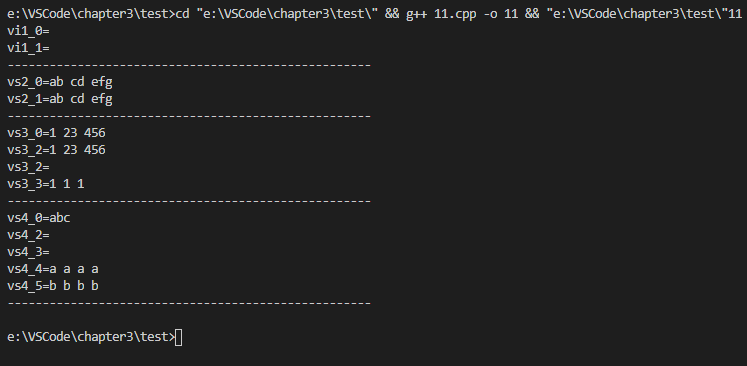
3. array initialization
#include <iostream>
#include <string>
using namespace std;
int cout_int(string name, int i[], unsigned int n);
int cout_char(string name, char c[], unsigned int n);
int cout_str(string name, string s[], unsigned int n);
// 函数外部默认初始化
int i1_0[3];
int main()
{
// 函数内部默认初始化,数组是内置复合类型,其元素值未定义
int i1_1[3];
// 拷贝初始化
int i2_0[3] = {}; //函数外部的int i1_0[3];类似,i2_0有3个元素,其值都为0
int i2_1[3] = {1, 2, 3}; //初始值个数与数组容量相对应
// int i2_2[3] = i2_1;//数组不允许拷贝赋值
char c2_0[3] = {'a', 'b', '\0'}; // 若进行字符串相关操作,一定确保以'\0'结尾,'\0'也是数组元素,计算数组容量时需注意
char c2_1[3] = "ab"; //其后会自动添加'\0','\0'也是数组元素,计算数组容量时需注意
string s2_0[3] = {"ab", "cde"};
// 直接初始化
int i3_0[3]{};
int i3_1[3]{1, 2, 3};
// int i3_2[3]();// 无法用()初始化,类内数据成员和列表初始化不能用()
cout_int("i1_0", i1_0, 3);
cout_int("i1_1", i1_1, 3);
cout << "----------------------------------------------------" << endl;
cout_int("i2_0", i2_0, 3);
cout_int("i2_1", i2_1, 3);
cout_char("c2_0", c2_0, 3);
cout_char("c2_1", c2_1, 3);
cout_str("s2_0", s2_0, 3);
cout << "----------------------------------------------------" << endl;
cout_int("i3_0", i3_0, 3);
cout_int("i3_1", i3_1, 3);
cout << "----------------------------------------------------" << endl;
return 0;
}
int cout_int(string name, int i[], unsigned int n)
{
cout << name << "=";
for (unsigned int ui = 0; ui != n; ++ui)
{
cout << i[ui] << " ";
}
cout << endl;
return 0;
}
int cout_char(string name, char c[], unsigned int n)
{
cout << name << "=";
for (unsigned int ui = 0; ui != n; ++ui)
{
cout << c[ui] << " ";
}
cout << endl;
return 0;
}
int cout_str(string name, string s[], unsigned int n)
{
cout << name << "=";
for (unsigned int ui = 0; ui != n; ++ui)
{
cout << s[ui] << " ";
}
cout << endl;
return 0;
}
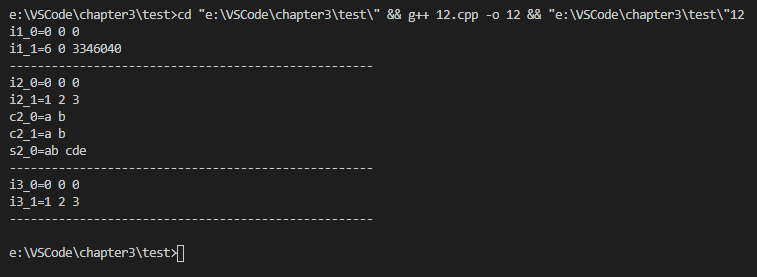