Singleton
Singleton design pattern Introduction
Singleton design pattern called class, is to take a certain way to ensure the entire software system, for a class there is only an object instance , and the class provides only one method (static method) to obtain its object instance.
Such as Hibernate 's SessionFactory , it acts as a proxy data storage source, and is responsible for creating Session objects. SessionFactory not lightweight, under normal circumstances, a project usually requires only a SessionFactory is enough, this is will be used to singleton.
Singleton design pattern eight ways
1) starving formula (static constants)
2) starving formula (static code block)
3) lazy man's (thread safe)
4) lazy man's (thread-safe, synchronization method)
5) lazy formula (thread-safe, synchronized block)
6) double-checked
7) static inner classes
8) enumeration
Hungry man type (static constants)
Hungry man type (static constant) Application Examples
The following steps :
1) constructor privatization (preventing new)
Internal 2) class to create objects
3) exposing a public static method outwardly. getInstance
4) code implementation
SingletonTest01.java
package com.atguigu.singleton.type1;
public class SingletonTest01 {
public static void main(String[] args) {
//测试
Singleton instance = Singleton.getInstance();
Singleton instance2 = Singleton.getInstance();
System.out.println(instance == instance2); // true
System.out.println("instance.hashCode=" + instance.hashCode());
System.out.println("instance2.hashCode=" + instance2.hashCode());
}
}
//饿汉式(静态变量)
class Singleton {
//1. 构造器私有化, 外部不能new
private Singleton() {
}
//2.本类内部创建对象实例
private final static Singleton instance = new Singleton();
//3. 提供一个公有的静态方法,返回实例对象
public static Singleton getInstance() {
return instance;
}
}
Explain the advantages and disadvantages :
1) advantages: such an approach is relatively simple, that is, at the time of loading the class instantiation is completed . Avoid thread synchronization issues .
2) disadvantage: When loading is completed the class instantiation, did not reach Lazy Loading effect . If you have never used this example from start to finish, it will result in a waste of memory
3) In this way based on classloder mechanism avoids the synchronization problem multithreading , however, instance to instance of the class is loaded at the time, in most cases a single mode are calling getInstance method , but there are several reasons why the class loading, and therefore can not determine other ways (or other static methods) cause the class loader, it will not reach instance initialization lazy loading effect
4) Conclusion: This singleton available , may result in wasted memory
Starving formula (static code block)
SingletonTest02.java
package com.atguigu.singleton.type2;
public class SingletonTest02 {
public static void main(String[] args) {
//测试
Singleton instance = Singleton.getInstance();
Singleton instance2 = Singleton.getInstance();
System.out.println(instance == instance2); // true
System.out.println("instance.hashCode=" + instance.hashCode());
System.out.println("instance2.hashCode=" + instance2.hashCode());
}
}
//饿汉式(静态代码块)
class Singleton {
//1. 构造器私有化, 外部不能new
private Singleton() {
}
//2.本类内部创建对象实例
private static Singleton instance;
static { // 在静态代码块中,创建单例对象
instance = new Singleton();
}
//3. 提供一个公有的静态方法,返回实例对象
public static Singleton getInstance() {
return instance;
}
}
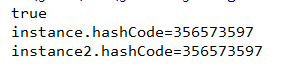
Explain the advantages and disadvantages :
1) in this manner and the above embodiment are actually quite similar, except that the class instantiation process on static code block , but also at the time of loading of the class, code execution on static code block, initialized instance of the class. Advantages and disadvantages and the above is the same.
2) Conclusion: Single This embodiment mode can be used, but may result in wasted memory
Lazy man's ( thread safe )
SingletonTest03.java
package com.atguigu.singleton.type3;
public class SingletonTest03 {
public static void main(String[] args) {
System.out.println("懒汉式1 , 线程不安全~");
Singleton instance = Singleton.getInstance();
Singleton instance2 = Singleton.getInstance();
System.out.println(instance == instance2); // true
System.out.println("instance.hashCode=" + instance.hashCode());
System.out.println("instance2.hashCode=" + instance2.hashCode());
}
}
class Singleton {
private static Singleton instance;
private Singleton() {}
//提供一个静态的公有方法,当使用到该方法时,才去创建 instance
//即懒汉式
public static Singleton getInstance() {
if(instance == null) {
instance = new Singleton();
}
return instance;
}
}
Explain the advantages and disadvantages :
1) plays a Lazy Loading effect, but can only be used in single-threaded.
2) If in a multi-threaded, a thread into the if (singleton == null) Analyzing statement block, had time to perform down, but also by another thread Analyzing this statement will produce a plurality of time instances. So in a multithreaded environment can not be used in this way
3) Conclusion: In the actual development, do not use this approach
Lazy man's ( thread-safe, synchronization method )
SingletonTest04.java
package com.atguigu.singleton.type4;
public class SingletonTest04 {
public static void main(String[] args) {
System.out.println("懒汉式2 , 线程安全~");
Singleton instance = Singleton.getInstance();
Singleton instance2 = Singleton.getInstance();
System.out.println(instance == instance2); // true
System.out.println("instance.hashCode=" + instance.hashCode());
System.out.println("instance2.hashCode=" + instance2.hashCode());
}
}
// 懒汉式(线程安全,同步方法)
class Singleton {
private static Singleton instance;
private Singleton() {}
//提供一个静态的公有方法,加入同步处理的代码,解决线程安全问题
//即懒汉式
public static synchronized Singleton getInstance() {
if(instance == null) {
instance = new Singleton();
}
return instance;
}
}
Explain the advantages and disadvantages :
1) to solve the problem of insecurity thread
2) efficiency is too low, each thread would like to get the class instance when executed getInstance () method must be synchronized. And in fact, this method only once instance of code is enough, I want to get behind this kind of instance, direct return on the line. Inefficient methods to synchronize
3) Conclusion: In the actual development, in this way is not recommended