前言:
2018年复试的最后一道机试题关于文件的,我发现我好像不太会备份文件。所以就先放在一边,把2019年的做了。
跟之前的一样,题目都是回忆版。可能会有题目阐述不清楚的情况,多多谅解
一、编程计算 1/1!-1/3!+1/5!-...+(-1)(n+1)/(2n-1)!
源码
#include<iostream>
using namespace std;
int function(int n){
if(n==1){
return 1;
}
return n*function(n-1);
}
int main(){
cout<<"please input n:";
int n;
cin>>n;
while(n<1){
cout<<"error! n need larger than 1 or equal to 1!"<<endl;
cout<<"please input n:";
cin>>n;
}
int k=2*n-1;
int m=1; //系数
double result=0;
for(int i=1;i<=k;i=i+2){
result += 1.0/function(i)*m;
m=-m;
}
cout<<"result = "<<result<<endl;
}
结果:
please input n:5
result = 0.841471
二、甲乙丙对一次竞赛名次进行预测。
甲:A 第 1 B 第 3
乙:C 第 1 D 第 4
丙:D 第 1 B 第 3 。
他们都说对了一半,求 ABCD 正确的名次(ABCD 不会出现相同的名次)。
按师兄的说法这道题数据不是原题,记不太清,但解题方法是一样的
有一说一,这道题用的方法特别的烂,用的穷举法,里面各种for循环和判断结束的if
但我着实没想出来什么好办法
#include<iostream>
using namespace std;
int main(){
int A,B,C,D;
bool flag = false;
for(A=1;A<=4 && flag == false;A++)
{
for(B=1;B<=4 && flag == false;B++)
{
for(C=1;C<=4 && flag == false;C++)
{
for(D=1;D<=4 && flag == false;D++)
{
if( ( A==1 && B!=3 ) || (A!=1 && B==3) ) //甲对一半
{
if( (C==1 && D!=4) || (C!=1 && D==4)) //乙对一半
{
if( (D==1 && B!=3) || (D!=1 && B==3) ) //丙对一半
{
//判断是否有相同的排名
if( A==B || A==C || A==D || B==C || B==D || D==C)
continue;
else{
flag=true;
break;
}
}
}
}
}
if (flag == true)
{
/* code */
break;
}
}
if (flag == true)
{
/* code */
break;
}
}
if (flag == true)
{
/* code */
break;
}
}
cout<<"A:"<<A<<endl;
cout<<"B:"<<B<<endl;
cout<<"C:"<<C<<endl;
cout<<"D:"<<D<<endl;
}
结果:
扫描二维码关注公众号,回复:
9382034 查看本文章
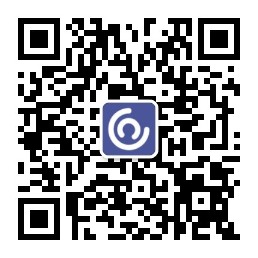
A:4
B:3
C:1
D:2
三、给定链表节点的定义 struct Node{ int data; Node *next; } 请编写一个函数,用递归的方式:对两个有序链表合并成一个有序链表。
这题可能是近年来最难的一题了
#include<iostream>
using namespace std;
struct Node{
int data;
Node *next;
};
Node* Sort(Node *L1,Node *L2){
Node *p;
if(L1 == NULL)
return L2;
if(L2 == NULL)
return L1;
if(L1->data < L2->data){
p=L1;
p->next = Sort(L1->next,L2);
}
else
{
p = L2;
p->next = Sort(L2->next,L1);
}
return p;
}
void print(Node *p){
Node *t=p;
while(t){
cout<<t->data<<" ";
t=t->next;
}
cout<<endl;
}
int main(){
Node *l1 = new Node();
Node *l2 = new Node();
Node *p;
p=l1;
cout<<"input l1:";
for(int i=0;i<5;i++)
{
cin>>p->data;
if(i!=4)
{
p->next = new Node();
p=p->next;
}
}
p=l2;
cout<<"input l2:";
for(int i=0;i<5;i++)
{
cin>>p->data;
if(i!=4)
{
p->next = new Node();
p=p->next;
}
}
print(l1);
print(l2);
Node *l3 = Sort(l1,l2);
print(l3);
}
结果:
input l1:1 3 4 5 8
input l2:3 6 9 10 11
1 3 4 5 8
3 6 9 10 11
1 3 3 4 5 6 8 9 10 11
四、酒店场景。
要求:
定义一个客人类 Guest。包含成员属性:编号 Num、姓名 Name、房费 Fee、当前酒店入住人数 Count。
其中编号 Num 需要程序自动生成。
现在要求实现以下 Guest 的成员函数:构造函数、Show()显示 Guest 的信息、 GetCount()返回当前酒店入住的人数、GetTotalIncome()返回当前酒店的总收入。
并定义 3 个 Guest 对象来对成员函数进行测试。
源码:
#include<iostream>
#include<string>
using namespace std;
int Total = 0; //累计人数,不排除退房的旅客,用于作为编号
class Guest{
private:
int Num;
string Name;
double Fee;
static int Count;
public:
Guest(string,double);
void Show();
static int GetCount(){ return Count ;}
};
Guest:: Guest(string name,double fee){
Num=++Total;
Name=name;
Fee=fee;
Count++;
}
int Guest::Count=0;
void Guest::Show(){
cout<<endl<<"Name:"<<Name<<endl;
cout<<"Num:"<<Num<<endl;
cout<<"Fee:"<<Fee<<endl;
}
int main(){
Guest gue[3]={
Guest("Mike",300),
Guest("Cherry",450),
Guest("Kobe",888)
};
for(int i=0;i<3;i++)
gue[i].Show();
cout<<endl<<"Now Count:"<<Guest::GetCount()<<endl;
}
结果:
Name:Mike
Now Count:3
PS C:\Users\YiTse\Desktop\大四\考研上机真题> g++ 2019_04.cpp
PS C:\Users\YiTse\Desktop\大四\考研上机真题> ./a.exe
Name:Mike
Num:1
Fee:300
Name:Cherry
Num:1
Fee:300
Name:Cherry
Num:2
Fee:450
Name:Kobe
Num:3
Fee:888
五、抽象类 Shape
要求:它拥有一系列虚函数:Input()输入类需要的信息、Show() 显示类的信息、Perimeter()计算周长、Area()计算面积。
先定义 Circle、Square、 Triangle 来继承 Shape 并实现其虚函数。要求创建 Circle、Square、Triangle 的对象,用基类指针指向这些对象,并调用成员函数
#include<iostream>
#include<cmath>
using namespace std;
class Shape{
public:
virtual void Input()=0;
virtual void Show()=0;
virtual double Perimeter()=0;
virtual double Area()=0;
};
class Circle:public Shape{
private:
double radius;
public:
void Input();
void Show();
double Perimeter();
double Area();
};
class Square:public Shape{
private:
double width;
double height;
public:
void Input();
void Show();
double Perimeter();
double Area();
};
class Triangle:public Shape{
private:
double length_A,length_B,length_C; //三条边的长度
public:
void Input();
void Show();
double Perimeter();
double Area();
};
void Circle::Input(){
cout<<"input radius:";
cin>>radius;
while(radius<=0){
cout<<"error!"<<endl;
cout<<"input radius:";
cin>>radius;
}
}
void Square::Input(){
cout<<"input width and height:";
cin>>width>>height;
while(width<=0 || height<=0){
cout<<"error!"<<endl;
cout<<"input width and height:";
cin>>width>>height;
}
}
void Triangle::Input(){
cout<<"input length of each side:";
cin>>length_A>>length_B>>length_C;
while(length_C<=0 || length_B<=0 || length_A<0 ||
length_A+length_B<=length_C || length_A+length_B<=length_C || length_B+length_C<=length_A){
cout<<"error!"<<endl;
cout<<"input length of each side:";
cin>>length_A>>length_B>>length_C;
}
}
void Circle::Show(){
cout<<"Radius:"<<radius<<endl;
}
void Square::Show(){
cout<<"width:"<<width<<endl;
cout<<"height:"<<height<<endl;
}
void Triangle::Show(){
cout<<"Lenght_A:"<<length_A<<endl;
cout<<"Lenght_B:"<<length_B<<endl;
cout<<"Lenght_C:"<<length_C<<endl;
}
double Circle::Area(){
return radius*radius*3.14519;
}
double Square::Area(){
return width*height;
}
double Triangle::Area(){
double p=0.5*(length_A+length_B+length_C);
return sqrt(p*(p-length_A)*(p-length_B)*(p-length_C));
}
double Circle::Perimeter(){
return 2*3.14159*radius;
}
double Square::Perimeter(){
return (width+height)*2;
}
double Triangle::Perimeter(){
return length_B+length_C+length_A;
}
int main(){
Shape *base;
Circle circle;
Triangle triangle;
Square square;
base=&circle;
base->Input();
base->Show();
cout<<"Area="<<base->Area()<<endl;
cout<<"Perimeter="<<base->Perimeter()<<endl;
cout<<endl;
base=▵
base->Input();
base->Show();
cout<<"Area="<<base->Area()<<endl;
cout<<"Perimeter="<<base->Perimeter()<<endl;
cout<<endl;
base=□
base->Input();
base->Show();
cout<<"Area="<<base->Area()<<endl;
cout<<"Perimeter="<<base->Perimeter()<<endl;
return 0;
}
结果:
input radius:2
Radius:2
Area=12.5808
Perimeter=12.5664
input length of each side:3 4 5
Lenght_A:3
Lenght_B:4
Lenght_C:5
Area=6
Perimeter=12
input width and height: 4 6
width:4
height:6
Area=24