Android功能脚本
注:这里只写了登录和退出功能,以下不提供app的包名,下面我使用的是线上包
准备:1、Eclipse的Java环境;2、Appium环境;3、Android真机一台。
创建一个Maven Project
这里省略了一万个字。。。。。。
修改pom.xml文档:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com</groupId> <artifactId>testmobike</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>testmobike</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>io.appium</groupId> <artifactId>java-client</artifactId> <version>5.0.1</version> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>3.5.2</version> </dependency> </dependencies> </project>
连接真机,启动电脑的cmd.exe运行adb命令
查看手机是否连接正常,并查看deviceName:adb shell;exit;adb devices
查看手机里的包名:adb shell;su;cd /data/data;ls 或者 adb shell;pm list package -f
启动Appium
点击放大图标,在Desired Capabilities里设置相关系数
(
扫描二维码关注公众号,回复:
6017026 查看本文章
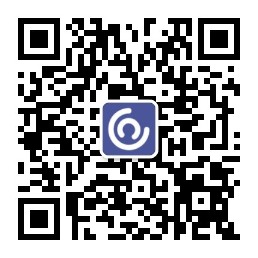
"appPackage": "", "appActivity": "", "platformName": "android", "deviceName": ""
)
启动后可以相应的录制脚本,这里又省略了一万个字。。。。。。
调试代码:
package test2.hejiaqin_login; import io.appium.java_client.MobileElement; import io.appium.java_client.TouchAction; import io.appium.java_client.android.AndroidDriver; import org.junit.After; import org.junit.Before; import org.junit.Test; import java.io.IOException; import static org.junit.Assert.assertTrue; import java.io.File; import java.net.MalformedURLException; import java.net.URL; import org.openqa.selenium.remote.DesiredCapabilities; public class hejiaqin3_9 { private AndroidDriver driver; @Before public void setUp() throws MalformedURLException { DesiredCapabilities desiredCapabilities = new DesiredCapabilities(); File appDir = new File(System.getProperty("user.dir"), "apps"); File app = new File(appDir, " 包 ");//包放在apps文件夹里面 desiredCapabilities.setCapability("app", app.getAbsolutePath()); desiredCapabilities.setCapability("appPackage", " 包名 "); desiredCapabilities.setCapability("appActivity", ".splash.activity.SplashActivity"); desiredCapabilities.setCapability("platformName", "android"); desiredCapabilities.setCapability("deviceName", "设备标识");//vivoX7 desiredCapabilities.setCapability("noReset", "false"); // desiredCapabilities.setCapability("unicodeKeyboard", "ture"); // desiredCapabilities.setCapability("resetKeyboard", "ture"); //desiredCapabilities.setCapability("newCommandTimeout")=30; URL remoteUrl = new URL("http://localhost:4723/wd/hub"); driver = new AndroidDriver(remoteUrl, desiredCapabilities); } @After public void tearDown() throws Exception { driver.quit(); } public void waittimeout(String s,int type,int timeout) throws InterruptedException {//等待方式 switch(type){ case 1: while(driver.findElementsById(s).size()==0){ Thread.sleep(1000); timeout--; if(timeout<0){ break; } } break; case 2: while(driver.findElementsByXPath(s).size()==0){ System.out.println("==="+timeout); Thread.sleep(1000); timeout--; if(timeout<0){ break; } } break; case 3: while(driver.findElementsByClassName(s).size()==0){ Thread.sleep(1000); timeout--; if(timeout<0){ break; } } break; default: while(driver.findElementsByAccessibilityId(s).size()==0){ Thread.sleep(1000); timeout--; if(timeout<0){ break; } } break; } } @Test public void sampleTest() throws InterruptedException { //滑动功能 Thread.sleep(2000); TouchAction action = new TouchAction(driver); int width = driver.manage().window().getSize().width; int height = driver.manage().window().getSize().height; int x1 =width*7/8; int x2 =width*1/8; action.longPress(x1,height/2).moveTo(x2,height/2).release().perform(); Thread.sleep(2000); action.longPress(x1,height/2).moveTo(x2,height/2).release().perform(); Thread.sleep(2000); action.longPress(x1,height/2).moveTo(x2,height/2).release().perform(); Thread.sleep(2000); //driver.findElementById("com.cmri.universalapp:id/go_login").click();//立即进入 (new TouchAction(driver)).tap(527, 1758).perform(); //assertTrue("ok",driver.findElementById("com.cmri.universalapp:id/switch_login_type").isDisplayed());//找到“切换通行证账号登录”键 Thread.sleep(5000); driver.findElementById("com.cmri.universalapp:id/switch_login_type").click();//切换通行证账号登录 Thread.sleep(2000); waittimeout("com.cmri.universalapp:id/etPhone",1,30); MobileElement el2 = (MobileElement) driver.findElementById("com.cmri.universalapp:id/etPhone"); el2.sendKeys("账号手机号"); Thread.sleep(2000); int i=10; while(!el2.getText().toString().replaceAll("\\s*","").equals("账号手机号")){ el2.sendKeys("账号手机号"); Thread.sleep(2000); i--; if(i<0){ break; } } //输入的用户名去掉空格,换行,制表符之后如果不和150这个值一样,那么就把这个el2设置成150这个值 MobileElement elh = (MobileElement) driver.findElementById("com.cmri.universalapp:id/ivShowPassword"); elh.click(); Thread.sleep(2000); MobileElement el3 = (MobileElement) driver.findElementById("com.cmri.universalapp:id/etPassword"); el3.sendKeys("密码"); Thread.sleep(2000); int t=10; while(!el3.getText().toString().replaceAll("\\s*","").equals("密码")){ el3.sendKeys("密码"); Thread.sleep(2000); t--; if(t<0){ break; } } MobileElement el4 = (MobileElement) driver.findElementById("com.cmri.universalapp:id/tvLogin"); el4.click(); //登录 Thread.sleep(2000); //下面去弹窗 /* waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout",2,30); driver.findElementById("com.cmri.universalapp:id/tv_next_step").click(); waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout",2,30); driver.findElementById("com.cmri.universalapp:id/tv_next_step").click(); waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout",2,30); driver.findElementById("com.cmri.universalapp:id/tv_next_step").click(); waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout",2,30); driver.findElementById("com.cmri.universalapp:id/tv_next_step").click(); //waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.LinearLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout/android.widget.ImageView",2,30); //driver.findElementByXPath("/hierarchy/android.widget.FrameLayout/android.widget.LinearLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout/android.widget.ImageView").click(); Thread.sleep(5000); float a1 = (float) (990.0/1080); //得出长宽在手机的比例,这里的是 华为P10手机 分辨率是 1080X1920,这样得出比例,换手机也可以实现控件位置。 double b1 = 130.0/1920; int c1 = (int) (a1*width); int d1 = (int) (b1*height); action.tap(c1,d1).perform(); waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout/android.widget.ImageView[1]",2,30); driver.findElementById("com.cmri.universalapp:id/ivCancelDlg").click();//把最后一张图叉了 */ waittimeout("/hierarchy/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.FrameLayout/android.widget.RelativeLayout/android.widget.ImageView[1]",2,30); driver.findElementById("com.cmri.universalapp:id/iv_close").click();//直接X掉跳过 //我的-退出 waittimeout("com.cmri.universalapp:id/check_box_bottom_tab_mine",1,30); driver.findElementById("com.cmri.universalapp:id/check_box_bottom_tab_mine").click();//我的 按钮 Thread.sleep(2000); action.longPress(width*1/2,height*3/4).moveTo(width*1/2,height*1/4).release().perform(); Thread.sleep(2000); waittimeout("com.cmri.universalapp:id/nav_linear_setting",1,30); driver.findElementById("com.cmri.universalapp:id/nav_linear_setting").click();//设置 按钮 driver.findElementById("com.cmri.universalapp:id/tvLogout").click();//退出按钮 Thread.sleep(2000); driver.findElementById("com.cmri.universalapp:id/dialog_ok_btn").click();//退出登录 按钮 Thread.sleep(2000); } }
这笔记,感觉写了没有营养,后期修改。。。