前面说到了使用Statement接口对数据库进行增加数据操作;
现在我们来看一下修改操作和删除操作;
其实基本原理是一样的,就是sql语句有点不一样;
1.修改操作;
我们新建一个update_sql类:
package Month01.Day08.Demo02;
import java.sql.Connection;
import java.sql.Statement;
import Month01.Day08.DbUtil.DbUtil;
import Month01.Day08.Model.Book;
public class update_sql {
private static DbUtil dbUtil = new DbUtil();
private static int updateBook(Book book) throws Exception {
// 连接数据库
Connection con = dbUtil.getCon();
// 获取Statement接口
Statement stmt = con.createStatement();
// 写sql语句
String sql = "update t_book set bookName='" + book.getBookName() + "',price=" + book.getPrice() + ",author='"
+ book.getAuthor() + "',bookTypeId=" + book.getBookTypeId() + " where id=" + book.getId() + "";
// 执行sql语句
int result = stmt.executeUpdate(sql);
// 关闭数据库连接
dbUtil.close(stmt, con);
return result;
}
public static void main(String[] args) throws Exception {
Book book = new Book(5, "Web设计", 65, "大奶牛", 2);
int result = updateBook(book);
if (result == 1) {
System.out.println("数据修改成功!");
} else {
System.out.println("数据修改失败!");
}
}
}
将表t_book中ID为5的数据修改如上;
原先表中内容为:
程序执行修改之后为:
可以看到数据修改成功!
扫描二维码关注公众号,回复:
4847766 查看本文章
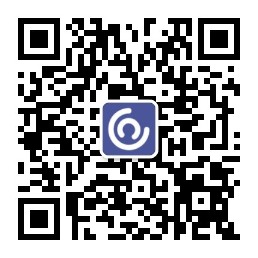
2.删除操作;
我们新建一个delete_sql类:
package Month01.Day08.Demo02;
import java.sql.Connection;
import java.sql.Statement;
import Month01.Day08.DbUtil.DbUtil;
public class delete_sql {
private static DbUtil dbUtil = new DbUtil();
private static int deleteBook(int id) throws Exception {
// 连接数据库
Connection con = dbUtil.getCon();
// 获取Statement接口
Statement stmt = con.createStatement();
// sql语句
String sql = "delete from t_book where id=" + id + "";
// 执行sql语句
int result = stmt.executeUpdate(sql);
// 关闭数据库连接
dbUtil.close(stmt, con);
return result;
}
public static void main(String[] args) throws Exception {
int result = deleteBook(6);
if (result == 1) {
System.out.println("数据删除成功!");
} else {
System.out.println("数据删除失败!");
}
}
}
将表中ID为6的那条数据删除掉;
原表中数据为:
程序执行修改之后为:
可以明显看到ID为6的那条数据已经删除成功!