题目:
Given a string containing just the characters '('
, ')'
, '{'
, '}'
, '['
and ']'
, determine if the input string is valid.
An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Note that an empty string is also considered valid.
Example 1:
Input: "()" Output: true
Example 2:
Input: "()[]{}" Output: true
Example 3:
Input: "(]" Output: false
Example 4:
Input: "([)]" Output: false
Example 5:
Input: "{[]}" Output: true
扫描二维码关注公众号,回复:
2839222 查看本文章
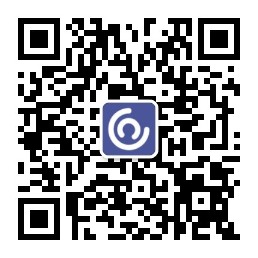
AC:
class Solution {
boolean isValidParentheses(char begin, char end) {
if (('{' == begin && '}' == end) ||
('[' == begin && ']' == end) ||
('(' == begin && ')' == end)){
return true;
}
return false;
}
public boolean isValid(String s) {
if (null == s || "" == s) {
return true;
}
LinkedList<Character> result = new LinkedList<>();
for (char e : s.toCharArray()) {
if (false == result.isEmpty() && true == isValidParentheses(result.getFirst(), e)) {
result.removeFirst();
}
else {
result.addFirst(e);
}
}
return true == result.isEmpty() ? true : false;
}
}
符号要配对,一前一后,一一对应。一对一对的消除的话,最后应该全部消除才是合法。
考虑使用栈,当前符号和栈顶的符号配对的话,则栈顶元素弹出,否则压栈。最后栈空即为合法。