Go语言提供了称为Goroutines的特殊函数。 Goroutine是一个函数或与你的程序中存在的任何其他Goroutines同时独立执行的方法。换句话说, 每个Go语言中同时执行的活动称为Goroutines。你可以将Goroutine视为轻量级线程。与线程相比, 创建Goroutines的成本非常小。每个程序至少包含一个Goroutine, 并且该Goroutine被称为主Goroutine。如果主Goroutine终止, 则所有Goroutine在主Goroutine之下运行, 那么程序中存在的所有Goroutine也将终止。 Goroutine始终在后台运行。
如何创建Goroutine?
你只需使用go关键字作为函数或方法调用的前缀即可创建自己的Goroutine, 如以下语法所示:
语法如下:
func name(){
// statements
}
// using go keyword as the
// prefix of your function call
go name()
例子:
// Go program to illustrate
// the concept of Goroutine
package main
import "fmt"
func display(str string) {
for w := 0; w < 6; w++ {
fmt.Println(str)
}
}
func main() {
// Calling Goroutine
go display( "Welcome" )
// Calling normal function
display( "lsbin" )
}
输出如下:
lsbin
lsbin
lsbin
lsbin
lsbin
lsbin
在上面的示例中, 我们只需创建一个显示()函数, 然后以两种不同的方式调用此函数, 第一种是Goroutine, 即去显示("欢迎")另一个是正常函数, 即显示(" lsbin")。但是有一个问题, 它只显示普通函数的结果, 而不显示Goroutine的结果, 因为当执行新的Goroutine时, Goroutine调用会立即返回。控件不像普通函数那样等待Goroutine完成执行, 它们总是在Goroutine调用后一直前进到下一行, 并忽略Goroutine返回的值。因此, 为了正确执行Goroutine, 我们对程序进行了一些更改, 如以下代码所示:
修改示例:
// Go program to illustrate the concept of Goroutine
package main
import (
"fmt"
"time"
)
func display(str string) {
for w := 0; w < 6; w++ {
time .Sleep(1 * time .Second)
fmt.Println(str)
}
}
func main() {
// Calling Goroutine
go display( "Welcome" )
// Calling normal function
display( "lsbin" )
}
输出如下:
Welcome
lsbin
lsbin
Welcome
Welcome
lsbin
lsbin
Welcome
Welcome
lsbin
lsbin
我们添加了Sleep()方法在我们的程序中, 该程序使主Goroutine在新Goroutine执行的1秒钟之间休眠1秒钟, 并显示"欢迎", 然后在1秒钟主Goroutine重新安排时间并执行其操作后终止。这个过程一直持续到z <6之后, 主Goroutine终止。在这里, Goroutine和普通函数同时工作。
Goroutines的优点
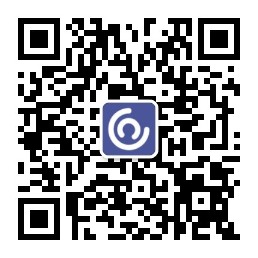
- Goroutine比线程便宜。
- Goroutine存储在堆栈中, 并且堆栈的大小可以根据程序的要求而增大和缩小。但是在线程中, 堆栈的大小是固定的。
- Goroutine可以使用通道进行通信, 并且这些通道经过特殊设计, 可以防止在使用Goroutines访问共享内存时出现争用情况。
- 假设一个程序有一个线程, 并且该线程有许多与之关联的Goroutine。如果由于资源需求, 任何Goroutine阻塞线程, 则所有其余Goroutine将分配给新创建的OS线程。所有这些细节对程序员都是隐藏的。
匿名Goroutine
在Go语言中, 你还可以为匿名函数启动Goroutine, 换句话说, 你只需使用go关键字作为该函数的前缀即可创建匿名Goroutine, 如以下语法所示:
语法如下:
// Anonymous function call
go func (parameter_list){
// statement
}(arguments)
例子:
// Go program to illustrate how
// to create an anonymous Goroutine
package main
import (
"fmt"
"time"
)
// Main function
func main() {
fmt.Println( "Welcome!! to Main function" )
// Creating Anonymous Goroutine
go func() {
fmt.Println( "Welcome!! to lsbin" )
}()
time .Sleep(1 * time .Second)
fmt.Println( "GoodBye!! to Main function" )
}
输出如下:
Welcome!! to Main function
Welcome!! to lsbin
GoodBye!! to Main function
更多Golang开发相关内容请参考:lsbin - IT开发技术:https://www.lsbin.com/
查看以下更多JS相关的内容: