先看效果图:
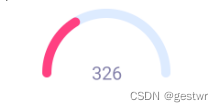
自定义属性
<declare-styleable name="QQSteps">
<attr name="roundWidth" format="dimension" />
<attr name="roundColor" format="color" />
<attr name="progressColor" format="color" />
<attr name="progressStep" format="integer" />
<attr name="maxStep" format="integer" />
<attr name="textColor" format="color" />
<attr name="textSize" format="dimension" />
</declare-styleable>
自定义view 仿qq步数
package com.demo;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.Rect;
import android.graphics.RectF;
import android.util.AttributeSet;
import android.view.View;
import androidx.annotation.Nullable;
import com.demo.myapp.R;
public class QQSteps extends View {
private int mRoundWidth;
private int mRoundColor;
private int mProgressColor;
private int mProgressStep;
private int mMaxStep;
private int mTextSize;
private int mTextColor;
private static final Paint mPaint = new Paint();
private int mViewWidth;
private int mViewHeight;
private int colorPrimary;
private int colorAccent;
private float percent;
private RectF oval;
public QQSteps(Context context) {
super(context);
}
public QQSteps(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
initView(context, attrs, 0);
}
public QQSteps(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initView(context, attrs, defStyleAttr);
}
private void initView(Context context, AttributeSet attrs, int defStyleAttr) {
colorPrimary = 0x33559bff;
colorAccent = 0xFFFF4081;
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.QQSteps);
mRoundWidth = typedArray.getDimensionPixelSize(R.styleable.QQSteps_roundWidth, 20);
mRoundColor = typedArray.getColor(R.styleable.QQSteps_roundColor, colorPrimary);
mProgressColor = typedArray.getColor(R.styleable.QQSteps_progressColor, colorAccent);
mProgressStep = typedArray.getInt(R.styleable.QQSteps_progressStep, 0);
mMaxStep = typedArray.getInt(R.styleable.QQSteps_progressStep, 100);
mTextColor = R.color.colorAAA;
mTextSize = typedArray.getDimensionPixelSize(R.styleable.QQSteps_progressStep, 40);
typedArray.recycle();
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
mViewWidth = getMeasuredWidth();
mViewHeight = getMeasuredHeight();
}
@Override
protected void onDraw(Canvas canvas) {
int centerX = mViewWidth / 2;
int centerY = mViewHeight / 2;
mPaint.setStrokeWidth(mRoundWidth);
mPaint.setStrokeCap(Paint.Cap.ROUND);
mPaint.setStrokeJoin(Paint.Join.ROUND);
mPaint.setColor(mRoundColor);
mPaint.setStyle(Paint.Style.STROKE);
int radius = (int) (centerX - mRoundWidth/2);
oval = new RectF(centerX - radius, centerY - radius, centerX + radius, centerY + radius);
canvas.drawArc(oval, 180, 180, false, mPaint);
mPaint.setColor(mProgressColor);
percent = (float) mProgressStep / mMaxStep;
canvas.drawArc(oval, 180, percent * 180, false, mPaint);
mPaint.reset();
mPaint.setAntiAlias(true);
mPaint.setTextSize(mTextSize);
mPaint.setColor(mTextColor);
String mStep = ((int) (percent * mMaxStep)) + "";
Rect textBounds = new Rect();
mPaint.getTextBounds(mStep, 0, mStep.length(), textBounds);
int dx = (getWidth() - textBounds.width()) / 2;
Paint.FontMetrics fontMetrics = mPaint.getFontMetrics();
int baseLine = (int) (getHeight() / 2 + (fontMetrics.bottom - fontMetrics.top) / 2 - fontMetrics.bottom);
canvas.drawText(mStep, dx, baseLine, mPaint);
}
public synchronized void setMaxStep(int maxStep) {
if (maxStep < 0) {
throw new IllegalArgumentException("maxStep 不能小于0!");
}
this.mMaxStep = maxStep;
}
public synchronized int getMaxStep() {
return mMaxStep;
}
public synchronized void setProgress(int progress) {
if (progress < 0) {
throw new IllegalArgumentException("progress 不能小于0!");
}
this.mProgressStep = progress;
invalidate();
}
public synchronized int getProgress() {
return mProgressStep;
}
public float getPercent() {
return percent;
}
public void setRoundWidth(int mRoundWidth) {
this.mRoundWidth = mRoundWidth;
}
public void setRoundColor(int mRoundColor) {
this.mRoundColor = mRoundColor;
}
public void setProgressColor(int mProgressColor) {
this.mProgressColor = mProgressColor;
}
public void setTextSize(int mTextSize) {
this.mTextSize = mTextSize;
}
public void setTextColor(int mTextColor) {
this.mTextColor = mTextColor;
}
public void setColorPrimary(int colorPrimary) {
this.colorPrimary = colorPrimary;
}
public void setColorAccent(int colorAccent) {
this.colorAccent = colorAccent;
}
}