gitee地址如下
如何获取摄像头验证码和ip

首先获取摄像头底部的验证码及ip(测试使用的是萤石摄像头,需要PC下载萤石客户端查看ip)

未连接之前可以通过VLC进行测试
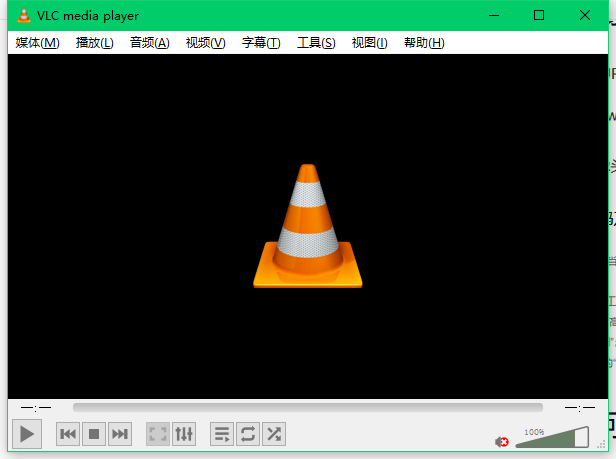
在左上角(媒体)--》(流)--》(网络)--》输入rtsp链接
如下就是rtsp链接
rtsp://admin:[email protected]:554/h264/ch1/main/av_stream
测试还可以使用该rtsp链接
rtmp://mobliestream.c3tv.com:554/live/goodtv.sdp
项目结构
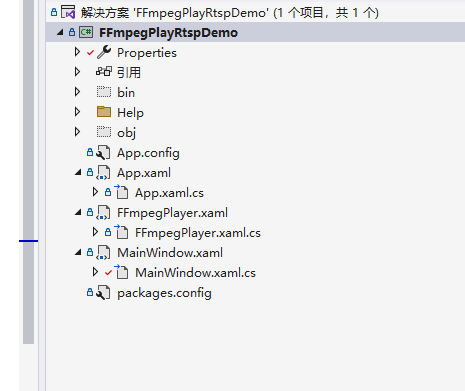
源码
(1)MainWindow.xaml.cs
using FFmpeg.AutoGen;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Interop;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace FFmpegPlayRtspDemo
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
player1.RtspUrl = "rtsp://admin:[email protected]:554/h264/ch1/main/av_stream";
//player1.RtspUrl = "rtsp://wowzaec2demo.streamlock.net/vod/mp4:BigBuckBunny_115k.mp4";
}
}
}
(2)MainWindow.xaml
<Window x:Class="FFmpegPlayRtspDemo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:FFmpegPlayRtspDemo"
mc:Ignorable="d"
Title="FFmpeg播放" Height="480" Width="800" WindowStartupLocation="CenterScreen" ResizeMode="NoResize">
<Grid>
<local:FFmpegPlayer x:Name="player1"/>
</Grid>
</Window>
(3)FFmpegPlayer.xaml
<UserControl x:Class="FFmpegPlayRtspDemo.FFmpegPlayer"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:FFmpegPlayRtspDemo"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800">
<Grid>
<Image Name="videodest01" Tag="01" Margin="386,210,0,-3"></Image>
<Image Name="videodest02" Tag="02" Margin="0,211,427,3"></Image>
<Image Name="videodest03" Tag="03" Margin="385,4,0,234"></Image>
<Image Name="videodest04" Tag="04" Margin="0,0,428,236"></Image>
</Grid>
</UserControl>
(4)FfmpegPlayer.xaml.cs
using FFmpeg.AutoGen;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Interop;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace FFmpegPlayRtspDemo
{
/// <summary>
/// FFmpegPlayer.xaml 的交互逻辑
/// </summary>
public partial class FFmpegPlayer : UserControl
{
private string _RtspUrl;
public string RtspUrl
{
get { return _RtspUrl; }
set
{
_RtspUrl = value;
Play();
}
}
public bool CanRun;
Thread thPlayer;
public FFmpegPlayer()
{
InitializeComponent();
}
private void Play()
{
if (string.IsNullOrEmpty(RtspUrl))
return;
thPlayer = new Thread(DeCoding);
thPlayer.SetApartmentState(ApartmentState.MTA);//设置单线程
thPlayer.IsBackground = true;
thPlayer.Start();
}
object _bitMapLocker = new object();
private unsafe void DeCoding()
{
try
{
//if (ClassHelp.Instance.SysSettingModel.Camera1 != 0)
//{
ClassHelp.Instance.FFmpegHelp = new FFmpegHelp();
ClassHelp.Instance.FFmpegHelp.Register();
//}
string strResult = "";
lock (_bitMapLocker)
{
// 更新图片显示
FFmpegHelp.ShowBitmap show = (bmp) =>
{
Dispatcher.BeginInvoke(new Action(() =>
{
Bitmap oldBitMap;
Bitmap autobitmap;
if (bmp != null)
{
oldBitMap = bmp;
autobitmap = bmp;
ImageSource videSource = ImageSourceForBitmap(oldBitMap);
this.videodest01.Source = videSource;
this.videodest02.Source = videSource;
this.videodest03.Source = videSource;
this.videodest04.Source = videSource;
ClassHelp.Instance.IsAlert = false;
}
else
{
ClassHelp.Instance.IsAlert = true;
ClassHelp.Instance.IsNetwork = true;
}
}));
};
ClassHelp.Instance.FFmpegHelp.Start(show, RtspUrl, out strResult);
//Start(show, RtspUrl, out strResult);
if (!string.IsNullOrEmpty(strResult) && ClassHelp.Instance.IsAlert == true)
{
ClassHelp.Instance.IsAlert = false;
ClassHelp.Instance.IsNetwork = false;
this.Dispatcher.Invoke(new Action(() =>
{
MessageBox.Show($"错误信息:{strResult}");
}));
}
}
}
finally
{
thPlayer.DisableComObjectEagerCleanup();
thPlayer = null;
thPlayer = new Thread(DeCoding);
thPlayer.IsBackground = true;
thPlayer.Start();
}
}
[DllImport("gdi32.dll", EntryPoint = "DeleteObject")]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool DeleteObject([In] IntPtr hObject);
public ImageSource ImageSourceForBitmap(Bitmap bmp)
{
var handle = bmp.GetHbitmap();
try
{
ImageSource newSource = Imaging.CreateBitmapSourceFromHBitmap(handle, IntPtr.Zero, Int32Rect.Empty, BitmapSizeOptions.FromEmptyOptions());
DeleteObject(handle);
return newSource;
}
catch (Exception ex)
{
DeleteObject(handle);
return null;
}
}
}
}
截图
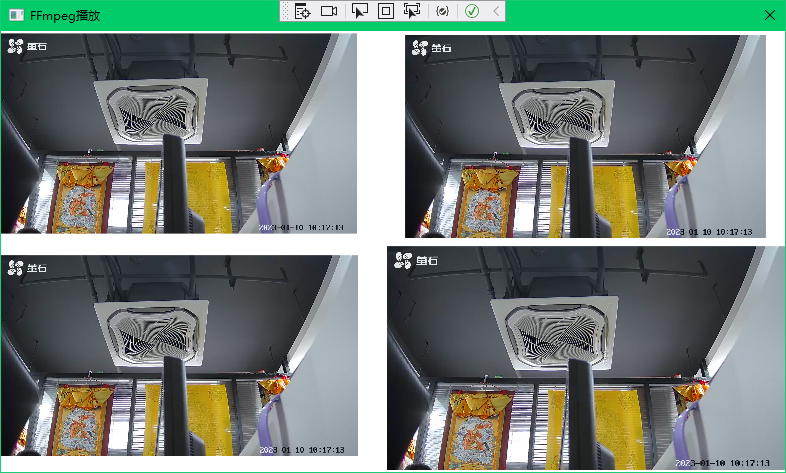