Vorwort: Einem Mann das Fischen beizubringen ist besser, als ihm das Fischen beizubringen . Es gibt viele Artikel über die Verwendung von ASM und es gibt auch viele einfache Demos. Welche Fähigkeiten hat ASM also? Wie lerne ich, ASM-Code zu schreiben? Welche Szenarien erfordern den Einsatz von ASM? Lassen Sie uns diesen Artikel unter Berücksichtigung dieser Fragen lesen.
Dieser Abschnitt wurde aus Platzgründen gelöscht (Teil 6: Vergleich von TreeApi und CoreApi, Einführung in die Kern-API-Klassen usw.). Bei Interesse können Sie sich zur Kommunikation an den Autor wenden.
Persönlich denke ich, dass der Kern im fünften Teil liegt: Wie man überprüft, wie man den ASM-Code einer Klasse schreibt, die man schreiben möchte, und wie man die Fähigkeiten von ASM vollständig versteht, sodass wir später in bestimmten Szenarien wissen, dass wir es können Verwenden Sie es, um die Funktionen zu erreichen, die wir ausführen möchten.
1. Einführung in ASM
1. Was ist ASM?
ASM ist ein universelles Java-Bytecode-Manipulations- und Analyse-Framework. Es kann verwendet werden, um vorhandene Klassen zu ändern oder Klassen direkt dynamisch in binärer Form zu generieren. ASM bietet eine Reihe gängiger Bytecode-Transformations- und Analysealgorithmen, aus denen benutzerdefinierte komplexe Transformations- und Codeanalysetools erstellt werden können. ASM bietet ähnliche Funktionen wie andere Java-Bytecode-Frameworks, konzentriert sich jedoch auf die Leistung. Da es möglichst klein und schnell konzipiert und implementiert ist, eignet es sich gut für den Einsatz in dynamischen Systemen (kann aber natürlich auch statisch, etwa in einem Compiler, eingesetzt werden).
Eine .java-Datei generiert eine .class-Datei, nachdem sie vom Java-Compiler (javac) kompiliert wurde. In der .class-Datei werden Bytecode-Daten (ByteCode) gespeichert. Das von ASM betriebene Objekt ist Bytecode (ByteCode), und in vielen Fällen ist die spezifische Form von Bytecode (ByteCode) eine .class-Datei.
Die Art und Weise, wie ASM Bytecode (ByteCode) verarbeitet, ist „split-modify-merge“.
Bytecode-Tools | Klassenerstellung | Schnittstelle implementieren | Methodenaufruf | Klassenerweiterung | Aufruf der Methode der übergeordneten Klasse | Vorteil | Mangel | Häufige Verwendungen | Lernkosten |
---|---|---|---|---|---|---|---|---|---|
Java-Proxy | Unterstützung | Unterstützung | Unterstützung | nicht unterstützt | nicht unterstützt | Einfacher dynamischer Proxy bevorzugt | Eingeschränkte Funktionalität und keine Erweiterungsunterstützung | spring-aop,MyBatis | 1 Stern |
asm | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Beliebiges Einfügen von Bytecodes nahezu ohne Einschränkungen | Schwer zu erlernen und viel zu programmieren | cglib | 5 Sterne |
Javanisch | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Java-Originalsyntax, Einfügen von Zeichenfolgen, intuitives Schreiben | Unterstützt keine Syntax von jdk1.5 oder höher, z. B. Generika, erweitert für | Fastjson, MyBatis | 2 Sterne |
cglib | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Sieht ähnlich aus wie bytebuddy | Wird von bytebuddy eingestellt | EasyMock, jackson-databind | 3 Sterne |
bytebuddy | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Unterstützung | Unterstützt das Abfangen in jeder Dimension und kann Originalklassen, Methoden, Proxy-Klassen und alle Parameter abrufen | Nicht sehr intuitiv, erfordert etwas Lern- und Verständnisaufwand und verfügt über viele APIs | SkyWalking, Mockito, Hibernate, Powermock | 3 Sterne |
Vergleichstabellenreferenz: http://xingyun.jd.com/shendeng/article/detail/7826
- Offizielle ASM-Website: https://asm.ow2.io/
- ASM-Quellcode: https://gitlab.ow2.org/asm/asm
- Entwicklerhandbuch: https://asm.ow2.io/developer-guide.html
2. Was kann ASM tun?
Generieren, Ändern, Löschen (Schnittstellen, Klassen, Felder, Methoden ...) ASM kann Bytecode-Daten analysieren, generieren und transformieren. ASM kann anschaulich als Tür am Rande der „Java-Sprachwelt“ verstanden werden. Durch diese Öffnung Die Tür kann uns helfen, in die „Welt des Bytecodes“ einzutreten.
3. Tatsächliche ASM-Nutzungsszenarien
3.1. ASM im Frühjahr
Das erste Anwendungsszenario ist AOP im Spring Framework. In vielen Java-Projekten wird das Spring-Framework verwendet, und AOP (Aspect Oriented Programming) im Spring-Framework basiert auf ASM. Insbesondere kann Springs AOP über den dynamischen Proxy des JDK oder über CGLIB implementiert werden. Unter anderem basiert CGLib (Code Generation Library) auf ASM, sodass Spring AOP ASM indirekt verwendet. (Von 8.6 Proxying-Mechanismen der Spring Framework-Referenzdokumentation verwiesen).
3.2. ASM im JDK
Das zweite Anwendungsszenario ist der Lambda-Ausdruck im JDK. Eine sehr wichtige Funktion, die in Java 8 eingeführt wurde, ist die Unterstützung von Lambda-Ausdrücken. Mit Lambda-Ausdrücken können Methoden als Parameter übergeben werden, wodurch der Code prägnanter und kompakter wird. Möglicherweise ist uns jedoch nicht aufgefallen, dass der Aufruf von Lambda-Ausdrücken zu diesem Zeitpunkt (Java 8-Version) tatsächlich über ASM implementiert wird.
Das Paket jdk.internal.org.objectweb.asm in der Datei rt.jar enthält den integrierten ASM-Code des JDK. In der JDK 8-Version wird die ASM 5.0-Version verwendet.
Wenn wir die Codierungsimplementierung des Lambda-Ausdrucks verfolgen, finden wir die Methode InnerClassLambdaMetafactory.spinInnerClass(). In dieser Methode werden wir sehen: JDK verwendet jdk.internal.org.objectweb.asm.ClassWriter, um eine Klasse zu generieren, die den Code des Lambda-Ausdrucks umschließt.
LambdaMetafactory.metafactory() Der erste Schritt besteht darin, diese Methode zu finden. InnerClassLambdaMetafactory.buildCallSite() Der zweite Schritt besteht darin, diese Methode zu finden.
InnerClassLambdaMetafactory.spinInnerClass() Der dritte Schritt besteht darin, diese Methode zu finden
4. Zwei Komponenten von ASM
In Bezug auf die Kompositionsstruktur ist ASM in zwei Teile unterteilt: Der eine ist die Kern-API und der andere ist die Baum-API.
Darunter umfasst die Core-API asm.jar, asm-util.jar und asm-commons.jar; darunter umfasst die Tree-API asm-tree.jar und asm-analysis.jar.
asm.jar enthält folgende Funktionen: ClassReader, ClassVisitor, ClassWriter, FieldVisitor, FieldWriter, MethodVisitor, MethodWriter, Label, Opcodes, Type
Die ClassReader-Klasse ist dafür verantwortlich, den Inhalt der .class-Datei zu lesen und sie dann in verschiedene Teile aufzuteilen. Die ClassVisitor-Klasse ist für die Änderung der Informationen in einem bestimmten Teil der .class-Datei verantwortlich. Die ClassWriter-Klasse ist dafür verantwortlich, die verschiedenen Teile wieder zu einer vollständigen .class-Datei zusammenzusetzen.
Kernklassen in asm-util.jar
Klassen, die mit Check beginnen, sind hauptsächlich dafür verantwortlich, zu prüfen (Check), ob der Inhalt der generierten .class-Datei korrekt ist. Klassen, die mit Trace beginnen, sind hauptsächlich dafür verantwortlich, den Inhalt der .class-Datei in der Textausgabe auszugeben. Basierend auf den ausgegebenen Textinformationen können die internen Informationen der .class-Datei untersucht oder verfolgt werden (Trace).
5、Klassendatei
Wir alle wissen, dass in der .class-Datei ByteCode-Daten gespeichert sind. Diese ByteCode-Daten sind jedoch nicht chaotisch, sondern folgen einer bestimmten Datenstruktur.
Die Datenstruktur, der diese .class-Datei folgt, ist das in der Java Virtual Machine Specification definierte Klassendateiformat .
6. Gemeinsame Bytecode-Bibliotheken
Apache Commons BCEL: BCEL ist die Abkürzung für Byte Code Engineering Library.
Javassist: Javassist表示Java-Programmierassistent
ObjectWeb ASM: Das Thema dieses Kurses.
Byte Buddy: Eine auf ASM basierende Klassenbibliothek.
2. Erschaffe etwas aus dem Nichts
1. Generieren Sie eine neue Schnittstelle
Erwartete Ziele:
Generieren Sie eine .class-Datei, die eine normale Schnittstellenstruktur definiert
public interface ASMInterface {
byte byteType = 1;
short shortType = 1;
int intType = 1;
char charType = 's';
float floatType = 1.1F;
double doubleType = 1.2;
long longType = 1L;
boolean booleanType = false;
Byte ByteType = 1;
Short ShortType = Short.valueOf((short)1);
Integer IntegerType = 1;
String StringType = "s";
Float FloatType = 1.1F;
Double DoubleType = 1.1;
Long LongType = 1L;
Boolean BooleanType = true;
void function();
default String defaultFunction(Integer integer) {
System.out.println("param = " + integer);
return String.valueOf(integer);
}
static Integer getInteger(String str) {
return Integer.valueOf(str);
}
}
Codierungsimplementierung:
public class InterfaceGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/ASMGenerateInterface.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法,调用顺序和说明如下
/*
* visit
* [visitSource][visitModule][visitNestHost][visitPermittedSubclass][visitOuterClass]
* (visitAnnotation |
* visitTypeAnnotation |
* visitAttribute)*
* (visitNestMember |
* visitInnerClass |
* visitRecordComponent |
* visitField |
* visitMethod)*
* visitEnd
* []: 表示最多调用一次,可以不调用,但最多调用一次。
* ()和|: 表示在多个方法之间,可以选择任意一个,并且多个方法之间不分前后顺序。
* *: 表示方法可以调用0次或多次。
* */
//定义接口
/*
*visit(version, access, name, signature, superName, interfaces)
*version: 表示当前类的版本信息。在下述示例代码中,其取值为Opcodes.V1_8,表示使用Java 8版本。
*access: 表示当前类的访问标识(access flag)信息。在下面的示例中,access的取值是ACC_PUBLIC + ACC_ABSTRACT + ACC_INTERFACE,也可以写成ACC_PUBLIC | ACC_ABSTRACT | ACC_INTERFACE。如果想进一步了解这些标识的含义,可以参考Java Virtual Machine Specification的Chapter 4. The class File Format部分。
*name: 表示当前类的名字,它采用的格式是Internal Name的形式。在.java文件中,我们使用Java语言来编写代码,使用类名的形式是Fully Qualified Class Name,例如java.lang.String;将.java文件编译之后,就会生成.class文件;在.class文件中,类名的形式会发生变化,称之为Internal Name,例如java/lang/String。因此,将Fully Qualified Class Name转换成Internal Name的方式就是,将.字符转换成/字符。
*signature: 表示当前类的泛型信息。因为在这个接口当中不包含任何的泛型信息,因此它的值为null。
*superName: 表示当前类的父类信息,它采用的格式是Internal Name的形式。
*interfaces: 表示当前类实现了哪些接口信息。
**/
cw.visit(
V1_8, // version
ACC_PUBLIC + ACC_ABSTRACT + ACC_INTERFACE, // access
"sample/ASMGenerateInterface", // name
null, // signature
"java/lang/Object", // superName
null // interfaces
);
//定义字段-基本类型
/*
* visitField(access, name, descriptor, signature, value)
*access参数:表示当前字段或方法带有的访问标识(access flag)信息,例如ACC_PUBLIC、ACC_STATIC和ACC_FINAL等。
*name参数:表示当前字段或方法的名字。
*descriptor参数:表示当前字段或方法的描述符。这些描述符,与我们平时使用的Java类型是有区别的。byte-B、short-S、int-I、char-C、具体可以参考如下示例代码
*signature参数:表示当前字段或方法是否带有泛型信息。换句话说,如果不带有泛型信息,提供一个null就可以了;如果带有泛型信息,就需要给它提供某一个具体的值。
*value参数:是visitField()方法的第5个参数。这个参数的取值,与当前字段是否为常量有关系。如果当前字段是一个常量,就需要给value参数提供某一个具体的值;如果当前字段不是常量,那么使用null就可以了。
* */
{
FieldVisitor fv1 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "byteType", "B", null, new Integer(1));
fv1.visitEnd();
}
{
FieldVisitor fv2 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "shortType", "S", null, new Integer(1));
fv2.visitEnd();
}
{
FieldVisitor fv3 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "intType", "I", null, new Integer(1));
fv3.visitEnd();
}
{
FieldVisitor fv4 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "charType", "C", null, 's');
fv4.visitEnd();
}
{
FieldVisitor fv5 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "floatType", "F", null, new Float("1.1"));
fv5.visitEnd();
}
{
FieldVisitor fv6 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "doubleType", "D", null, new Double("1.2"));
fv6.visitEnd();
}
{
FieldVisitor fv7 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "longType", "J", null, new Long(1L));
fv7.visitEnd();
}
{
FieldVisitor fv8 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "booleanType", "Z", null, new Integer(0));
fv8.visitEnd();
}
//定义变量-包装类型
{
FieldVisitor fv11 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "ByteType", "Ljava/lang/Byte;", null, null);
fv11.visitEnd();
}
{
FieldVisitor fv12 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "ShortType", "Ljava/lang/Short;", null,null);
fv12.visitEnd();
}
{
FieldVisitor fv13 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "IntegerType", "Ljava/lang/Integer;", null,null);
fv13.visitEnd();
}
{
FieldVisitor fv14 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "StringType", "Ljava/lang/String;", null, "s");
fv14.visitEnd();
}
{
FieldVisitor fv15 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "FloatType", "Ljava/lang/Float;", null,null);
fv15.visitEnd();
}
{
FieldVisitor fv16 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "DoubleType", "Ljava/lang/Double;", null,null);
fv16.visitEnd();
}
{
FieldVisitor fv17 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "LongType", "Ljava/lang/Long;", null, null);
fv17.visitEnd();
}
{
FieldVisitor fv18 = cw.visitField(ACC_PUBLIC + ACC_STATIC + ACC_FINAL, "BooleanType", "Ljava/lang/Boolean;", null, null);
fv18.visitEnd();
}
//定义方法-抽象方法
/*
* visitMethod(access, name, descriptor, signature, exceptions)
*access参数:表示当前字段或方法带有的访问标识(access flag)信息,例如ACC_PUBLIC、ACC_STATIC和ACC_FINAL等。
*name参数:表示当前字段或方法的名字。
*descriptor参数:表示当前字段或方法的描述符。这些描述符,与我们平时使用的Java类型是有区别的。()内为入参,后面为反参
*signature参数:表示当前字段或方法是否带有泛型信息。换句话说,如果不带有泛型信息,提供一个null就可以了;如果带有泛型信息,就需要给它提供某一个具体的值。
*exceptions参数:是visitMethod()方法的第5个参数。这个参数的取值,与当前方法声明中是否具有throws XxxException相关。
* */
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC + ACC_ABSTRACT, "function", "()V", null, null);
mv1.visitEnd();
}
//定义方法-默认方法
{
MethodVisitor mv2 = cw.visitMethod(ACC_PUBLIC, "defaultFunction", "(Ljava/lang/Integer;)Ljava/lang/String;", null, null);
mv2.visitCode();
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitTypeInsn(NEW, "java/lang/StringBuilder");
mv2.visitInsn(DUP);
mv2.visitMethodInsn(INVOKESPECIAL, "java/lang/StringBuilder", "<init>", "()V", false);
mv2.visitLdcInsn("param = ");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/lang/StringBuilder", "append", "(Ljava/lang/String;)Ljava/lang/StringBuilder;", false);
mv2.visitVarInsn(ALOAD, 1);
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/lang/StringBuilder", "append", "(Ljava/lang/Object;)Ljava/lang/StringBuilder;", false);
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/lang/StringBuilder", "toString", "()Ljava/lang/String;", false);
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitVarInsn(ALOAD, 1);
mv2.visitMethodInsn(INVOKESTATIC, "java/lang/String", "valueOf", "(Ljava/lang/Object;)Ljava/lang/String;", false);
mv2.visitInsn(ARETURN);
mv2.visitMaxs(3, 2);
mv2.visitEnd();
}
//定义方法-静态方法
{
MethodVisitor mv3 = cw.visitMethod(ACC_PUBLIC | ACC_STATIC, "getInteger", "(Ljava/lang/String;)Ljava/lang/Integer;", null, null);
mv3.visitCode();
mv3.visitVarInsn(ALOAD, 0);
mv3.visitMethodInsn(INVOKESTATIC, "java/lang/Integer", "valueOf", "(Ljava/lang/String;)Ljava/lang/Integer;", false);
mv3.visitInsn(ARETURN);
mv3.visitMaxs(1, 1);
mv3.visitEnd();
}
{
MethodVisitor mv4 = cw.visitMethod(ACC_STATIC, "<clinit>", "()V", null, null);
mv4.visitCode();
mv4.visitInsn(ICONST_1);
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Byte", "valueOf", "(B)Ljava/lang/Byte;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "ByteType", "Ljava/lang/Byte;");
mv4.visitInsn(ICONST_1);
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Short", "valueOf", "(S)Ljava/lang/Short;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "ShortType", "Ljava/lang/Short;");
mv4.visitInsn(ICONST_1);
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Integer", "valueOf", "(I)Ljava/lang/Integer;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "IntegerType", "Ljava/lang/Integer;");
mv4.visitLdcInsn(new Float("1.1"));
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Float", "valueOf", "(F)Ljava/lang/Float;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "FloatType", "Ljava/lang/Float;");
mv4.visitLdcInsn(new Double("1.1"));
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Double", "valueOf", "(D)Ljava/lang/Double;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "DoubleType", "Ljava/lang/Double;");
mv4.visitInsn(LCONST_1);
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Long", "valueOf", "(J)Ljava/lang/Long;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "LongType", "Ljava/lang/Long;");
mv4.visitInsn(ICONST_1);
mv4.visitMethodInsn(INVOKESTATIC, "java/lang/Boolean", "valueOf", "(Z)Ljava/lang/Boolean;", false);
mv4.visitFieldInsn(PUTSTATIC, "sample/ASMInterface", "BooleanType", "Ljava/lang/Boolean;");
mv4.visitInsn(RETURN);
mv4.visitMaxs(2, 0);
mv4.visitEnd();
}
cw.visitEnd(); // 注意,最后要调用visitEnd()方法
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse:
Ist die generierte Schnittstelle korrekt?
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
Class<?> clazz = classLoader.loadClass("sample.ASMGenerateInterface");
Field[] declaredFields = clazz.getDeclaredFields();
if (declaredFields.length > 0) {
System.out.println("fields:");
for (Field f : declaredFields) {
Object value = f.get(null);
System.out.println(" " + f.getName() + ": " + value);
}
}
Method[] declaredMethods = clazz.getDeclaredMethods();
if (declaredMethods.length > 0) {
System.out.println("methods:");
for (Method m : declaredMethods) {
System.out.println(" " + m.getName());
}
}
}
}
Die Darstellungen lauten wie folgt:
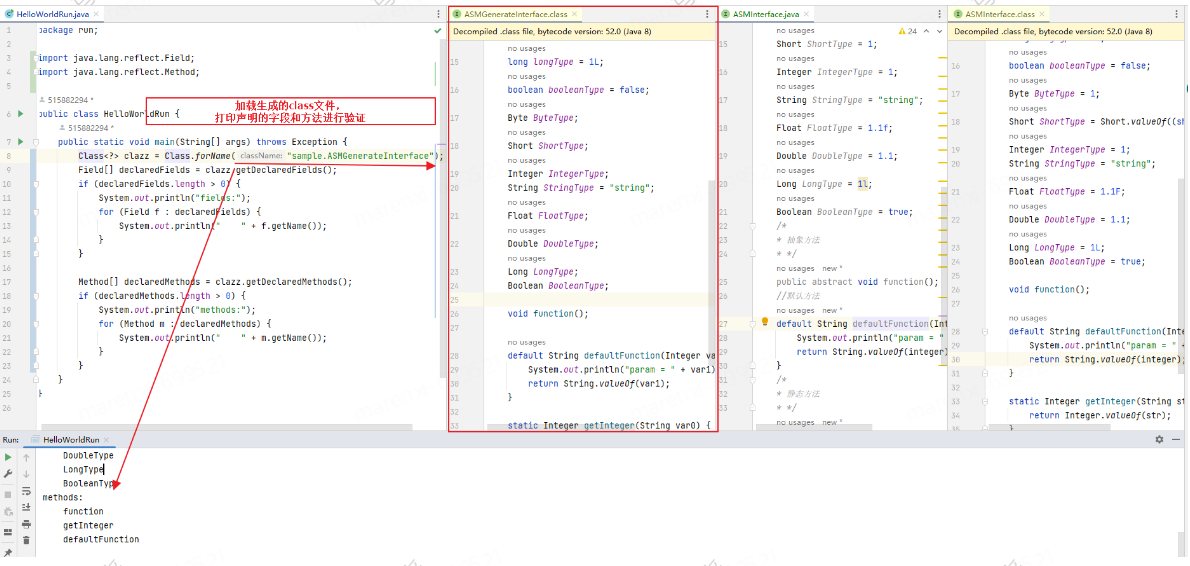
2. Generieren Sie neue Klassen
Erwartete Ziele:
Generieren Sie eine .class-Datei, die eine normale Klassenstruktur definiert
public class ASMClass {
//定义变量-基本类型
byte byteType = 1;
short shortType = 1;
int intType = 1;
char charType = 's';
float floatType = 1.1f;
double doubleType = 1.2;
long longType = 1;
boolean booleanType = false;
//定义变量-包装类型
Byte ByteType = 1;
Short ShortType = 1;
Integer IntegerType = 1;
String StringType = "string";
Float FloatType = 1.1f;
Double DoubleType = 1.1;
Long LongType = 1l;
@Deprecated
Boolean BooleanType = true;
/*
* 静态方法
* */
public static Integer getInteger(String str) {
return Integer.valueOf(str);
}
/*
* 实例方法
* */
public String instanceMethod(Integer integer) {
return String.valueOf(integer);
}
}
Codierungsimplementierung:
public class ClassGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/ASMGenerateClass.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法,调用顺序和说明如下
/*
* visit
* [visitSource][visitModule][visitNestHost][visitPermittedSubclass][visitOuterClass]
* (visitAnnotation |
* visitTypeAnnotation |
* visitAttribute)*
* (visitNestMember |
* visitInnerClass |
* visitRecordComponent |
* visitField |
* visitMethod)*
* visitEnd
* []: 表示最多调用一次,可以不调用,但最多调用一次。
* ()和|: 表示在多个方法之间,可以选择任意一个,并且多个方法之间不分前后顺序。
* *: 表示方法可以调用0次或多次。
* */
//定义接口
/*
*visit(version, access, name, signature, superName, interfaces)
*version: 表示当前类的版本信息。在下述示例代码中,其取值为Opcodes.V1_8,表示使用Java 8版本。
*access: 表示当前类的访问标识(access flag)信息。在下面的示例中,access的取值是ACC_PUBLIC + ACC_ABSTRACT + ACC_INTERFACE,也可以写成ACC_PUBLIC | ACC_ABSTRACT | ACC_INTERFACE。如果想进一步了解这些标识的含义,可以参考Java Virtual Machine Specification的Chapter 4. The class File Format部分。
*name: 表示当前类的名字,它采用的格式是Internal Name的形式。在.java文件中,我们使用Java语言来编写代码,使用类名的形式是Fully Qualified Class Name,例如java.lang.String;将.java文件编译之后,就会生成.class文件;在.class文件中,类名的形式会发生变化,称之为Internal Name,例如java/lang/String。因此,将Fully Qualified Class Name转换成Internal Name的方式就是,将.字符转换成/字符。
*signature: 表示当前类的泛型信息。因为在这个接口当中不包含任何的泛型信息,因此它的值为null。
*superName: 表示当前类的父类信息,它采用的格式是Internal Name的形式。
*interfaces: 表示当前类实现了哪些接口信息。
**/
cw.visit(
V1_8, // version
ACC_PUBLIC + ACC_SUPER, // access
"sample/ASMGenerateClass", // name
null, // signature
"java/lang/Object", // superName
null // interfaces
);
//定义字段-基本类型
/*
* visitField(access, name, descriptor, signature, value)
*access参数:表示当前字段或方法带有的访问标识(access flag)信息,例如ACC_PUBLIC、ACC_STATIC和ACC_FINAL等。
*name参数:表示当前字段或方法的名字。
*descriptor参数:表示当前字段或方法的描述符。这些描述符,与我们平时使用的Java类型是有区别的。byte-B、short-S、int-I、char-C、具体可以参考如下示例代码
*signature参数:表示当前字段或方法是否带有泛型信息。换句话说,如果不带有泛型信息,提供一个null就可以了;如果带有泛型信息,就需要给它提供某一个具体的值。
*value参数:是visitField()方法的第5个参数。这个参数的取值,与当前字段是否为常量有关系。如果当前字段是一个常量,就需要给value参数提供某一个具体的值;如果当前字段不是常量,那么使用null就可以了。
* */
{
FieldVisitor fv1 = cw.visitField(0, "byteType", "B", null, new Byte("1"));
fv1.visitEnd();
}
{
FieldVisitor fv2 = cw.visitField(0, "shortType", "S", null, new Short("1"));
fv2.visitEnd();
}
{
FieldVisitor fv3 = cw.visitField(0, "intType", "I", null, new Integer(1));
fv3.visitEnd();
}
{
FieldVisitor fv4 = cw.visitField(0, "charType", "C", null, "s");
fv4.visitEnd();
}
{
FieldVisitor fv5 = cw.visitField(0, "floatType", "F", null, new Float("1.1"));
fv5.visitEnd();
}
{
FieldVisitor fv6 = cw.visitField(0, "doubleType", "D", null, new Double("1.2"));
fv6.visitEnd();
}
{
FieldVisitor fv7 = cw.visitField(0, "longType", "J", null, new Long(1));
fv7.visitEnd();
}
{
FieldVisitor fv8 = cw.visitField(0, "booleanType", "Z", null, false);
fv8.visitEnd();
}
//定义变量-包装类型
{
FieldVisitor fv11 = cw.visitField(0, "ByteType", "Ljava/lang/Byte;", null, 1);
fv11.visitEnd();
}
{
FieldVisitor fv12 = cw.visitField(0, "ShortType", "Ljava/lang/Short;", null, 1);
fv12.visitEnd();
}
{
FieldVisitor fv13 = cw.visitField(0, "IntegerType", "Ljava/lang/Integer;", null, 1);
fv13.visitEnd();
}
{
FieldVisitor fv14 = cw.visitField(0, "StringType", "Ljava/lang/String;", null, "s");
fv14.visitEnd();
}
{
FieldVisitor fv15 = cw.visitField(0, "FloatType", "Ljava/lang/Float;", null, 1.1f);
fv15.visitEnd();
}
{
FieldVisitor fv16 = cw.visitField(ACC_PUBLIC, "DoubleType", "Ljava/lang/Double;", null, 1.1);
fv16.visitEnd();
}
{
FieldVisitor fv17 = cw.visitField(0, "LongType", "Ljava/lang/Long;", null, 1l);
fv17.visitEnd();
}
{
FieldVisitor fv18 = cw.visitField(ACC_DEPRECATED, "BooleanType", "Ljava/lang/Boolean;", null, true);
{
AnnotationVisitor annotationVisitor0 = fv18.visitAnnotation("Ljava/lang/Deprecated;", true);
annotationVisitor0.visitEnd();
}
fv18.visitEnd();
}
/*
* visitMethod(access, name, descriptor, signature, exceptions)
*access参数:表示当前字段或方法带有的访问标识(access flag)信息,例如ACC_PUBLIC、ACC_STATIC和ACC_FINAL等。
*name参数:表示当前字段或方法的名字。
*descriptor参数:表示当前字段或方法的描述符。这些描述符,与我们平时使用的Java类型是有区别的。()内为入参,后面为反参
*signature参数:表示当前字段或方法是否带有泛型信息。换句话说,如果不带有泛型信息,提供一个null就可以了;如果带有泛型信息,就需要给它提供某一个具体的值。
*exceptions参数:是visitMethod()方法的第5个参数。这个参数的取值,与当前方法声明中是否具有throws XxxException相关。
* */
//定义方法-静态代码块
{
MethodVisitor mv2 = cw.visitMethod(ACC_STATIC, "<clinit>", "()V", null, null);
mv2.visitCode();
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("class initialization method");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitInsn(RETURN);
mv2.visitMaxs(2, 0);
mv2.visitEnd();
}
//定义方法-无参构造器
{
MethodVisitor methodVisitor = cw.visitMethod(ACC_PUBLIC, "<init>", "()V", null, null);
methodVisitor.visitCode();
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitMethodInsn(INVOKESPECIAL, "java/lang/Object", "<init>", "()V", false);
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "byteType", "B");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "shortType", "S");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "intType", "I");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitIntInsn(BIPUSH, 115);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "charType", "C");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitLdcInsn(new Float("1.1"));
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "floatType", "F");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitLdcInsn(new Double("1.2"));
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "doubleType", "D");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(LCONST_1);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "longType", "J");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_0);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "booleanType", "Z");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Byte", "valueOf", "(B)Ljava/lang/Byte;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "ByteType", "Ljava/lang/Byte;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Short", "valueOf", "(S)Ljava/lang/Short;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "ShortType", "Ljava/lang/Short;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Integer", "valueOf", "(I)Ljava/lang/Integer;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "IntegerType", "Ljava/lang/Integer;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitLdcInsn("string");
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "StringType", "Ljava/lang/String;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitLdcInsn(new Float("1.1"));
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Float", "valueOf", "(F)Ljava/lang/Float;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "FloatType", "Ljava/lang/Float;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitLdcInsn(new Double("1.1"));
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Double", "valueOf", "(D)Ljava/lang/Double;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "DoubleType", "Ljava/lang/Double;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(LCONST_1);
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Long", "valueOf", "(J)Ljava/lang/Long;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "LongType", "Ljava/lang/Long;");
methodVisitor.visitVarInsn(ALOAD, 0);
methodVisitor.visitInsn(ICONST_1);
methodVisitor.visitMethodInsn(INVOKESTATIC, "java/lang/Boolean", "valueOf", "(Z)Ljava/lang/Boolean;", false);
methodVisitor.visitFieldInsn(PUTFIELD, "sample/ASMGenerateClass", "BooleanType", "Ljava/lang/Boolean;");
methodVisitor.visitInsn(RETURN);
methodVisitor.visitMaxs(3, 1);
methodVisitor.visitEnd();
}
//定义方法-静态方法
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC | ACC_STATIC, "getInteger", "(Ljava/lang/String;)Ljava/lang/Integer;", null, null);
mv1.visitCode();
mv1.visitVarInsn(ALOAD, 0);
mv1.visitMethodInsn(INVOKESTATIC, "java/lang/Integer", "valueOf", "(Ljava/lang/String;)Ljava/lang/Integer;", false);
mv1.visitInsn(ARETURN);
mv1.visitMaxs(1, 1);
mv1.visitEnd();
}
//定义方法-实例方法
{
MethodVisitor mv2 = cw.visitMethod(ACC_PUBLIC, "instanceMethod", "(Ljava/lang/Integer;)Ljava/lang/String;", null, null);
mv2.visitCode();
mv2.visitVarInsn(ALOAD, 1);
mv2.visitMethodInsn(INVOKESTATIC, "java/lang/String", "valueOf", "(Ljava/lang/Object;)Ljava/lang/String;", false);
mv2.visitInsn(ARETURN);
mv2.visitMaxs(1, 2);
mv2.visitEnd();
}
cw.visitEnd(); // 注意,最后要调用visitEnd()方法
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse:
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("sample.ASMGenerateClass");
Method method = clazz.getDeclaredMethod("instanceMethod",Integer.class);
Object instance = clazz.newInstance();
Object invoke = method.invoke(instance, new Integer(12));
Class<?> aClass = invoke.getClass();
System.out.println("aClass = " + aClass);
}
}
或者
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
Class<?> clazz = classLoader.loadClass("sample.ASMGenerateClass");
Field[] declaredFields = clazz.getDeclaredFields();
if (declaredFields.length > 0) {
for (Field f : declaredFields) {
Object value = f.get(null);
System.out.println(" " + f.getName() + ": " + value);
}
}
Method[] declaredMethods = clazz.getDeclaredMethods();
if (declaredMethods.length > 0) {
for (Method m : declaredMethods) {
System.out.println(" " + m.getName());
}
}
}
}
Die Darstellungen lauten wie folgt:
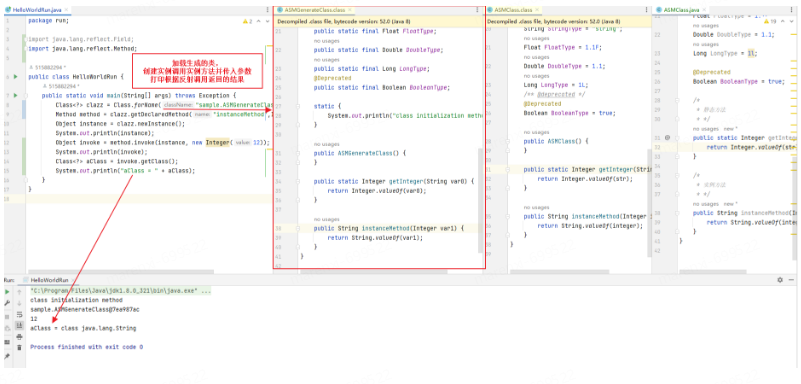
Die Aufrufsequenz von visitXxx() in ClassVisitor
visit
[visitSource][visitModule][visitNestHost][visitPermittedSubclass][visitOuterClass]
(
visitAnnotation |
visitTypeAnnotation |
visitAttribute
)*
(
visitNestMember |
visitInnerClass |
visitRecordComponent |
visitField |
visitMethod
)*
visitEnd
其中,涉及到一些符号,它们的含义如下:
[]: 表示最多调用一次,可以不调用,但最多调用一次。
()和|: 表示在多个方法之间,可以选择任意一个,并且多个方法之间不分前后顺序。
*: 表示方法可以调用0次或多次。
Die Aufrufsequenz von visitXxx() in FieldVisitor
(
visitAnnotation |
visitTypeAnnotation |
visitAttribute
)*
visitEnd
Die Aufrufsequenz von visitXxx() in MethodVisitor
(visitParameter)*
[visitAnnotationDefault]
(visitAnnotation | visitAnnotableParameterCount | visitParameterAnnotation | visitTypeAnnotation | visitAttribute)*
[
visitCode
(
visitFrame |
visitXxxInsn |
visitLabel |
visitInsnAnnotation |
visitTryCatchBlock |
visitTryCatchAnnotation |
visitLocalVariable |
visitLocalVariableAnnotation |
visitLineNumber
)*
visitMaxs
]
visitEnd
第一组,在visitCode()方法之前的方法。这一组的方法,主要负责parameter、annotation和attributes等内容
第二组,在visitCode()方法和visitMaxs()方法之间的方法。这一组的方法,主要负责当前方法的“方法体”内的opcode内容。其中,visitCode()方法,标志着方法体的开始,而visitMaxs()方法,标志着方法体的结束。
第三组,是visitEnd()方法。这个visitEnd()方法,是最后一个进行调用的方法。
Die visitXxx()-Methoden verschiedener MethodVisitor-Objekte sind unabhängig voneinander. Solange sie der Aufrufreihenfolge der Methoden folgen, können sie die richtigen Ergebnisse erzielen.
3. Die Zibetkatze tauscht gegen den Prinzen
1. Ändern Sie die Version der Klasse
Implementierung der ClassVisitor-Unterklasse
public class ClassChangeVersionVisitor extends ClassVisitor {
public ClassChangeVersionVisitor(int api, ClassVisitor classVisitor) {
super(api, classVisitor);
}
@Override
public void visit(int version, int access, String name, String signature, String superName, String[] interfaces) {
super.visit(Opcodes.V1_7, access, name, signature, superName, interfaces);
}
}
Verwenden Sie ClassChangeVersionVisitor, eine Unterklasse von ClassVisitor, um die Klassenversion zu ändern
public class ASMModifyClass {
public static void main(String[] args) throws Exception {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
byte[] bytes = FileUtils.readBytes(filepath);
//(1)构建ClassReader
ClassReader cr = new ClassReader(bytes);
//(2)构建ClassWriter
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
//(3)串连ClassVisitor
int api = Opcodes.ASM9;
ClassVisitor cv = new ClassChangeVersionVisitor(api, cw);
//(4)结合ClassReader和ClassVisitor
int parsingOptions = ClassReader.SKIP_DEBUG | ClassReader.SKIP_FRAMES;
cr.accept(cv, parsingOptions);
//(5)生成byte[]
byte[] bytes2 = cw.toByteArray();
FileUtils.writeBytes(filepath, bytes2);
}
}
Überprüfung und Wirkung
Mit dem Befehl javap -p -v HelloWorld können Sie sehen, dass die Versionsnummerninformationen von 52 auf 51 angepasst wurden
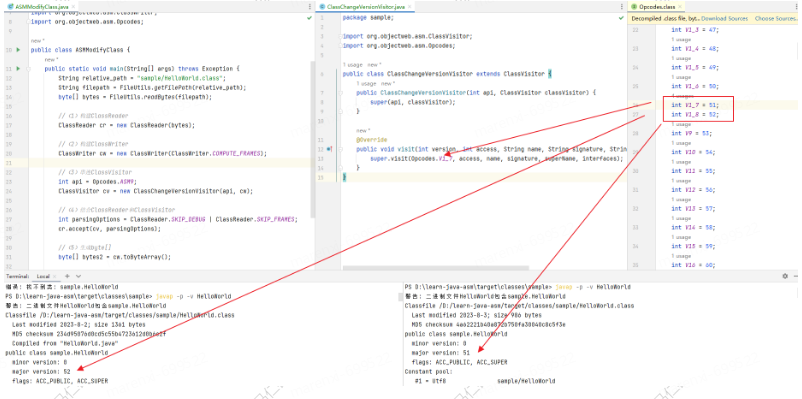
2. Fügen Sie jeder Methode die Berechnungsaufrufzeit hinzu
Ändern und tauschen Sie die Methoden der Zielklasse aus. Fügen Sie für jede Methode eine Zeitberechnung hinzu
public class HelloWorld {
public int add(int a, int b) throws InterruptedException {
int c = a + b;
Random rand = new Random(System.currentTimeMillis());
int num = rand.nextInt(300);
Thread.sleep(100 + num);
return c;
}
public int sub(int a, int b) throws InterruptedException {
int c = a - b;
Random rand = new Random(System.currentTimeMillis());
int num = rand.nextInt(400);
Thread.sleep(100 + num);
return c;
}
}
Implementierung der ASM-Codierung
public class MethodTimerVisitor2 extends ClassVisitor {
private String owner;
private boolean isInterface;
public MethodTimerVisitor2(int api, ClassVisitor classVisitor) {
super(api, classVisitor);
}
@Override
public void visit(int version, int access, String name, String signature, String superName, String[] interfaces) {
super.visit(version, access, name, signature, superName, interfaces);
owner = name;
isInterface = (access & ACC_INTERFACE) != 0;
}
@Override
public MethodVisitor visitMethod(int access, String name, String descriptor, String signature, String[] exceptions) {
MethodVisitor mv = super.visitMethod(access, name, descriptor, signature, exceptions);
if (!isInterface && mv != null && !"<init>".equals(name) && !"<clinit>".equals(name)) {
boolean isAbstractMethod = (access & ACC_ABSTRACT) != 0;
boolean isNativeMethod = (access & ACC_NATIVE) != 0;
if (!isAbstractMethod && !isNativeMethod) {
// 每遇到一个合适的方法,就添加一个相应的字段
FieldVisitor fv = super.visitField(ACC_PUBLIC | ACC_STATIC, getFieldName(name), "J", null, null);
if (fv != null) {
fv.visitEnd();
}
mv = new MethodTimerAdapter2(api, mv, owner, name);
}
}
return mv;
}
private String getFieldName(String methodName) {
return "timer_" + methodName;
}
private class MethodTimerAdapter2 extends MethodVisitor {
private final String owner;
private final String methodName;
public MethodTimerAdapter2(int api, MethodVisitor mv, String owner, String methodName) {
super(api, mv);
this.owner = owner;
this.methodName = methodName;
}
@Override
public void visitCode() {
// 首先,处理自己的代码逻辑
super.visitFieldInsn(GETSTATIC, owner, getFieldName(methodName), "J"); // 注意,字段名字要对应
super.visitMethodInsn(INVOKESTATIC, "java/lang/System", "currentTimeMillis", "()J", false);
super.visitInsn(LSUB);
super.visitFieldInsn(PUTSTATIC, owner, getFieldName(methodName), "J"); // 注意,字段名字要对应
// 其次,调用父类的方法实现
super.visitCode();
}
@Override
public void visitInsn(int opcode) {
// 首先,处理自己的代码逻辑
if ((opcode >= IRETURN && opcode <= RETURN) || opcode == ATHROW) {
super.visitFieldInsn(GETSTATIC, owner, getFieldName(methodName), "J"); // 注意,字段名字要对应
super.visitMethodInsn(INVOKESTATIC, "java/lang/System", "currentTimeMillis", "()J", false);
super.visitInsn(LADD);
super.visitFieldInsn(PUTSTATIC, owner, getFieldName(methodName), "J"); // 注意,字段名字要对应
}
// 其次,调用父类的方法实现
super.visitInsn(opcode);
}
}
}
Konvertieren Sie eine Methode
public class HelloWorldTransformCore {
private static final int DEFAULT_BUFFER_SIZE = 1024 * 4;
public static void main(String[] args) {
String relative_path = "sample/HelloWorld.class";
String dir = HelloWorldTransformCore.class.getResource("/").getPath();
String filepath = dir + relative_path;
File file = new File(filepath);
try {
InputStream in = new FileInputStream(file);
in = new BufferedInputStream(in);
ByteArrayOutputStream bao = new ByteArrayOutputStream();
copyLarge(in, bao, new byte[DEFAULT_BUFFER_SIZE]);
byte[] bytes1 = bao.toByteArray();
//(1)构建ClassReader
ClassReader cr = new ClassReader(bytes1);
//(2)构建ClassWriter
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
//(3)串连ClassVisitor
int api = Opcodes.ASM9;
ClassVisitor cv = new MethodTimerVisitor2(api, cw);
//(4)结合ClassReader和ClassVisitor
int parsingOptions = ClassReader.SKIP_DEBUG | ClassReader.SKIP_FRAMES;
cr.accept(cv, parsingOptions);
//(5)生成byte[]
byte[] bytes2 = cw.toByteArray();
OutputStream out = new FileOutputStream(filepath);
BufferedOutputStream buff = new BufferedOutputStream(out);
buff.write(bytes2);
buff.flush();
buff.close();
System.out.println("file://" + filepath);
} catch (IOException e) {
e.printStackTrace();
}
}
public static long copyLarge(final InputStream input, final OutputStream output, final byte[] buffer)
throws IOException {
long count = 0;
int n;
while (-1 != (n = input.read(buffer))) {
output.write(buffer, 0, n);
count += n;
}
return count;
}
}
Validierungsergebnisse
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
// 第一部分,先让“子弹飞一会儿”,让程序运行一段时间
HelloWorld instance = new HelloWorld();
Random rand = new Random(System.currentTimeMillis());
for (int i = 0; i < 10; i++) {
boolean flag = rand.nextBoolean();
int a = rand.nextInt(50);
int b = rand.nextInt(50);
if (flag) {
int c = instance.add(a, b);
String line = String.format("%d + %d = %d", a, b, c);
System.out.println(line);
}
else {
int c = instance.sub(a, b);
String line = String.format("%d - %d = %d", a, b, c);
System.out.println(line);
}
}
// 第二部分,来查看方法运行的时间
Class<?> clazz = HelloWorld.class;
Field[] declaredFields = clazz.getDeclaredFields();
for (Field f : declaredFields) {
String fieldName = f.getName();
f.setAccessible(true);
if (fieldName.startsWith("timer")) {
Object FieldValue = f.get(null);
System.out.println(fieldName + " = " + FieldValue);
}
}
}
}
3. Methodenparameter und Rückgabewerte drucken
Ändern Sie die Methode der Zielklasse. Fügen Sie jeder Methode Druckeingabeparameter und Ausgabeparameter hinzu
public class HelloWorld {
public int test(String name, int age, long idCard, Object obj) {
int hashCode = 0;
hashCode += name.hashCode();
hashCode += age;
hashCode += (int) (idCard % Integer.MAX_VALUE);
hashCode += obj.hashCode();
return hashCode;
}
}
Das erwartete Ziel, das wir erreichen möchten: Drucken Sie die „von der Methode empfangenen Parameterwerte“ und den „Rückgabewert der Methode“ aus.
public class HelloWorld {
public int test(String name, int age, long idCard, Object obj) {
int hashCode = 0;
hashCode += name.hashCode();
hashCode += age;
hashCode += (int) (idCard % Integer.MAX_VALUE);
hashCode += obj.hashCode();
System.out.println(hashCode);
return hashCode;
}
}
Die Idee, diese Funktion zu implementieren: Wenn die „Methode eintritt“, wird der „von der Methode empfangene Parameterwert“ ausgedruckt; wenn die „Methode beendet wird“, wird der „Rückgabewert der Methode“ ausgedruckt.
首先,我们添加一个ParameterUtils类,在这个类定义了许多print方法,这些print方法可以打印不同类型的数据。
public class ParameterUtils {
private static final DateFormat fm = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
public static void printValueOnStack(boolean value) {
System.out.println(" " + value);
}
public static void printValueOnStack(byte value) {
System.out.println(" " + value);
}
public static void printValueOnStack(char value) {
System.out.println(" " + value);
}
public static void printValueOnStack(short value) {
System.out.println(" " + value);
}
public static void printValueOnStack(int value) {
System.out.println(" " + value);
}
public static void printValueOnStack(float value) {
System.out.println(" " + value);
}
public static void printValueOnStack(long value) {
System.out.println(" " + value);
}
public static void printValueOnStack(double value) {
System.out.println(" " + value);
}
public static void printValueOnStack(Object value) {
if (value == null) {
System.out.println(" " + value);
}
else if (value instanceof String) {
System.out.println(" " + value);
}
else if (value instanceof Date) {
System.out.println(" " + fm.format(value));
}
else if (value instanceof char[]) {
System.out.println(" " + Arrays.toString((char[])value));
}
else {
System.out.println(" " + value.getClass() + ": " + value.toString());
}
}
public static void printText(String str) {
System.out.println(str);
}
}
In der folgenden Klasse verwenden wir die Klasse, um uns beim Drucken von Informationen zu helfen.MethodParameterVisitor2
ParameterUtils
Implementierung der ASM-Codierung
public class MethodParameterVisitor2 extends ClassVisitor {
public MethodParameterVisitor2(int api, ClassVisitor classVisitor) {
super(api, classVisitor);
}
@Override
public MethodVisitor visitMethod(int access, String name, String descriptor, String signature, String[] exceptions) {
MethodVisitor mv = super.visitMethod(access, name, descriptor, signature, exceptions);
if (mv != null && !name.equals("<init>")) {
boolean isAbstractMethod = (access & ACC_ABSTRACT) != 0;
boolean isNativeMethod = (access & ACC_NATIVE) != 0;
if (!isAbstractMethod && !isNativeMethod) {
mv = new MethodParameterAdapter2(api, mv, access, name, descriptor);
}
}
return mv;
}
private static class MethodParameterAdapter2 extends MethodVisitor {
private final int methodAccess;
private final String methodName;
private final String methodDesc;
public MethodParameterAdapter2(int api, MethodVisitor mv, int methodAccess, String methodName, String methodDesc) {
super(api, mv);
this.methodAccess = methodAccess;
this.methodName = methodName;
this.methodDesc = methodDesc;
}
@Override
public void visitCode() {
// 首先,处理自己的代码逻辑
boolean isStatic = ((methodAccess & ACC_STATIC) != 0);
int slotIndex = isStatic ? 0 : 1;
printMessage("Method Enter: " + methodName + methodDesc);
Type methodType = Type.getMethodType(methodDesc);
Type[] argumentTypes = methodType.getArgumentTypes();
for (Type t : argumentTypes) {
int sort = t.getSort();
int size = t.getSize();
String descriptor = t.getDescriptor();
int opcode = t.getOpcode(ILOAD);
super.visitVarInsn(opcode, slotIndex);
if (sort >= Type.BOOLEAN && sort <= Type.DOUBLE) {
String methodDesc = String.format("(%s)V", descriptor);
printValueOnStack(methodDesc);
}
else {
printValueOnStack("(Ljava/lang/Object;)V");
}
slotIndex += size;
}
// 其次,调用父类的方法实现
super.visitCode();
}
@Override
public void visitInsn(int opcode) {
// 首先,处理自己的代码逻辑
if ((opcode >= IRETURN && opcode <= RETURN) || opcode == ATHROW) {
printMessage("Method Exit: " + methodName + methodDesc);
if (opcode >= IRETURN && opcode <= DRETURN) {
Type methodType = Type.getMethodType(methodDesc);
Type returnType = methodType.getReturnType();
int size = returnType.getSize();
String descriptor = returnType.getDescriptor();
if (size == 1) {
super.visitInsn(DUP);
}
else {
super.visitInsn(DUP2);
}
String methodDesc = String.format("(%s)V", descriptor);
printValueOnStack(methodDesc);
}
else if (opcode == ARETURN) {
super.visitInsn(DUP);
printValueOnStack("(Ljava/lang/Object;)V");
}
else if (opcode == RETURN) {
printMessage(" return void");
}
else {
printMessage(" abnormal return");
}
}
// 其次,调用父类的方法实现
super.visitInsn(opcode);
}
private void printMessage(String str) {
super.visitLdcInsn(str);
super.visitMethodInsn(INVOKESTATIC, "sample/ParameterUtils", "printText", "(Ljava/lang/String;)V", false);
}
private void printValueOnStack(String descriptor) {
super.visitMethodInsn(INVOKESTATIC, "sample/ParameterUtils", "printValueOnStack", descriptor, false);
}
}
}
Führen Sie eine Konvertierung durch
public class HelloWorldTransformCore {
public static void main(String[] args) {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
byte[] bytes1 = FileUtils.readBytes(filepath);
//(1)构建ClassReader
ClassReader cr = new ClassReader(bytes1);
//(2)构建ClassWriter
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
//(3)串连ClassVisitor
int api = Opcodes.ASM9;
ClassVisitor cv = new MethodParameterVisitor2(api, cw);
//(4)结合ClassReader和ClassVisitor
int parsingOptions = ClassReader.SKIP_DEBUG | ClassReader.SKIP_FRAMES;
cr.accept(cv, parsingOptions);
//(5)生成byte[]
byte[] bytes2 = cw.toByteArray();
FileUtils.writeBytes(filepath, bytes2);
}
}
Validierungsergebnisse
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
HelloWorld instance = new HelloWorld();
int hashCode = instance.test("Tomcat", 10, System.currentTimeMillis(), new Object());
int remainder = hashCode % 2;
if (remainder == 0) {
System.out.println("hashCode is even number.");
}
else {
System.out.println("hashCode is odd number.");
}
}
}
4. Nicht-sequentielle Struktur
1. if-Anweisung
public class HelloWorld {
public void test(int value) {
if (value == 0) {
System.out.println("value is 0");
}
else {
System.out.println("value is not 0");
}
}
}
Implementierung der ASM-Codierung
public class HelloWorldGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法
cw.visit(V1_8, ACC_PUBLIC + ACC_SUPER, "sample/HelloWorld",
null, "java/lang/Object", null);
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC, "<init>", "()V", null, null);
mv1.visitCode();
mv1.visitVarInsn(ALOAD, 0);
mv1.visitMethodInsn(INVOKESPECIAL, "java/lang/Object", "<init>", "()V", false);
mv1.visitInsn(RETURN);
mv1.visitMaxs(0, 0);
mv1.visitEnd();
}
{
MethodVisitor mv2 = cw.visitMethod(ACC_PUBLIC, "test", "(I)V", null, null);
Label elseLabel = new Label();
Label returnLabel = new Label();
// 第1段
mv2.visitCode();
mv2.visitVarInsn(ILOAD, 1);
mv2.visitJumpInsn(IFNE, elseLabel);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("value is 0");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第2段
mv2.visitLabel(elseLabel);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("value is not 0");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
// 第3段
mv2.visitLabel(returnLabel);
mv2.visitInsn(RETURN);
mv2.visitMaxs(0, 0);
mv2.visitEnd();
}
cw.visitEnd();
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("sample.HelloWorld");
Object obj = clazz.newInstance();
Method method = clazz.getDeclaredMethod("test", int.class);
method.invoke(obj, 0);
method.invoke(obj, 1);
}
}
2. switch-Anweisung
public class HelloWorld {
public void test(int val) {
switch (val) {
case 1:
System.out.println("val = 1");
break;
case 2:
System.out.println("val = 2");
break;
case 3:
System.out.println("val = 3");
break;
case 4:
System.out.println("val = 4");
break;
default:
System.out.println("val is unknown");
}
}
}
Implementierung der ASM-Codierung
public class HelloWorldGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法
cw.visit(V1_8, ACC_PUBLIC + ACC_SUPER, "sample/HelloWorld",
null, "java/lang/Object", null);
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC, "<init>", "()V", null, null);
mv1.visitCode();
mv1.visitVarInsn(ALOAD, 0);
mv1.visitMethodInsn(INVOKESPECIAL, "java/lang/Object", "<init>", "()V", false);
mv1.visitInsn(RETURN);
mv1.visitMaxs(0, 0);
mv1.visitEnd();
}
{
MethodVisitor mv2 = cw.visitMethod(ACC_PUBLIC, "test", "(I)V", null, null);
Label caseLabel1 = new Label();
Label caseLabel2 = new Label();
Label caseLabel3 = new Label();
Label caseLabel4 = new Label();
Label defaultLabel = new Label();
Label returnLabel = new Label();
// 第1段
mv2.visitCode();
mv2.visitVarInsn(ILOAD, 1);
mv2.visitTableSwitchInsn(1, 4, defaultLabel, new Label[]{caseLabel1, caseLabel2, caseLabel3, caseLabel4});
// 第2段
mv2.visitLabel(caseLabel1);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("val = 1");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第3段
mv2.visitLabel(caseLabel2);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("val = 2");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第4段
mv2.visitLabel(caseLabel3);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("val = 3");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第5段
mv2.visitLabel(caseLabel4);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("val = 4");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第6段
mv2.visitLabel(defaultLabel);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("val is unknown");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
// 第7段
mv2.visitLabel(returnLabel);
mv2.visitInsn(RETURN);
mv2.visitMaxs(0, 0);
mv2.visitEnd();
}
cw.visitEnd();
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse
import java.lang.reflect.Method;
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("sample.HelloWorld");
Object obj = clazz.newInstance();
Method method = clazz.getDeclaredMethod("test", int.class);
for (int i = 1; i < 6; i++) {
method.invoke(obj, i);
}
}
}
3. zur Aussage
public class HelloWorld {
public void test() {
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
}
}
Implementierung der ASM-Codierung
import org.objectweb.asm.*;
import static org.objectweb.asm.Opcodes.*;
public class HelloWorldGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法
cw.visit(V1_8, ACC_PUBLIC + ACC_SUPER, "sample/HelloWorld",
null, "java/lang/Object", null);
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC, "<init>", "()V", null, null);
mv1.visitCode();
mv1.visitVarInsn(ALOAD, 0);
mv1.visitMethodInsn(INVOKESPECIAL, "java/lang/Object", "<init>", "()V", false);
mv1.visitInsn(RETURN);
mv1.visitMaxs(0, 0);
mv1.visitEnd();
}
{
MethodVisitor methodVisitor = cw.visitMethod(ACC_PUBLIC, "test", "()V", null, null);
Label conditionLabel = new Label();
Label returnLabel = new Label();
// 第1段
methodVisitor.visitCode();
methodVisitor.visitInsn(ICONST_0);
methodVisitor.visitVarInsn(ISTORE, 1);
// 第2段
methodVisitor.visitLabel(conditionLabel);
methodVisitor.visitVarInsn(ILOAD, 1);
methodVisitor.visitIntInsn(BIPUSH, 10);
methodVisitor.visitJumpInsn(IF_ICMPGE, returnLabel);
methodVisitor.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
methodVisitor.visitVarInsn(ILOAD, 1);
methodVisitor.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(I)V", false);
methodVisitor.visitIincInsn(1, 1);
methodVisitor.visitJumpInsn(GOTO, conditionLabel);
// 第3段
methodVisitor.visitLabel(returnLabel);
methodVisitor.visitInsn(RETURN);
methodVisitor.visitMaxs(0, 0);
methodVisitor.visitEnd();
}
cw.visitEnd();
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("sample.HelloWorld");
Object obj = clazz.newInstance();
Method method = clazz.getDeclaredMethod("test");
method.invoke(obj);
}
}
4. Try-Catch-Anweisung
public class HelloWorld {
public void test() {
try {
System.out.println("Before Sleep");
Thread.sleep(1000);
System.out.println("After Sleep");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Implementierung der ASM-Codierung
public class HelloWorldGenerateCore {
public static void main(String[] args) throws Exception {
String relative_path = "sample/HelloWorld.class";
String filepath = FileUtils.getFilePath(relative_path);
// (1) 生成byte[]内容
byte[] bytes = dump();
// (2) 保存byte[]到文件
FileUtils.writeBytes(filepath, bytes);
}
public static byte[] dump() throws Exception {
// (1) 创建ClassWriter对象
ClassWriter cw = new ClassWriter(ClassWriter.COMPUTE_FRAMES);
// (2) 调用visitXxx()方法
cw.visit(V1_8, ACC_PUBLIC + ACC_SUPER, "sample/HelloWorld",
null, "java/lang/Object", null);
{
MethodVisitor mv1 = cw.visitMethod(ACC_PUBLIC, "<init>", "()V", null, null);
mv1.visitCode();
mv1.visitVarInsn(ALOAD, 0);
mv1.visitMethodInsn(INVOKESPECIAL, "java/lang/Object", "<init>", "()V", false);
mv1.visitInsn(RETURN);
mv1.visitMaxs(0, 0);
mv1.visitEnd();
}
{
MethodVisitor mv2 = cw.visitMethod(ACC_PUBLIC, "test", "()V", null, null);
Label startLabel = new Label();
Label endLabel = new Label();
Label exceptionHandlerLabel = new Label();
Label returnLabel = new Label();
// 第1段
mv2.visitCode();
// visitTryCatchBlock可以在这里访问
mv2.visitTryCatchBlock(startLabel, endLabel, exceptionHandlerLabel, "java/lang/InterruptedException");
// 第2段
mv2.visitLabel(startLabel);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("Before Sleep");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
mv2.visitLdcInsn(new Long(1000L));
mv2.visitMethodInsn(INVOKESTATIC, "java/lang/Thread", "sleep", "(J)V", false);
mv2.visitFieldInsn(GETSTATIC, "java/lang/System", "out", "Ljava/io/PrintStream;");
mv2.visitLdcInsn("After Sleep");
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/io/PrintStream", "println", "(Ljava/lang/String;)V", false);
// 第3段
mv2.visitLabel(endLabel);
mv2.visitJumpInsn(GOTO, returnLabel);
// 第4段
mv2.visitLabel(exceptionHandlerLabel);
mv2.visitVarInsn(ASTORE, 1);
mv2.visitVarInsn(ALOAD, 1);
mv2.visitMethodInsn(INVOKEVIRTUAL, "java/lang/InterruptedException", "printStackTrace", "()V", false);
// 第5段
mv2.visitLabel(returnLabel);
mv2.visitInsn(RETURN);
// 第6段
// visitTryCatchBlock也可以在这里访问
// mv2.visitTryCatchBlock(startLabel, endLabel, exceptionHandlerLabel, "java/lang/InterruptedException");
mv2.visitMaxs(0, 0);
mv2.visitEnd();
}
cw.visitEnd();
// (3) 调用toByteArray()方法
return cw.toByteArray();
}
}
Validierungsergebnisse
public class HelloWorldRun {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("sample.HelloWorld");
Object obj = clazz.newInstance();
Method method = clazz.getDeclaredMethod("test");
method.invoke(obj);
}
}
5. Sehen Sie sich den ASM-Code der Klassendatei an
1.Drucken
Wenn wir ASM über eine bestimmte Klasse lernen möchten oder nicht wissen, wie wir die Funktion implementieren sollen, die wir implementieren möchten, können wir die folgende Klasse verwenden, um den ASM-Code auszugeben und anzuzeigen
public class ASMPrint {
public static void main(String[] args) throws IOException {
// (1) 设置参数
String className = "sample.HelloWorld";
int parsingOptions = ClassReader.SKIP_FRAMES | ClassReader.SKIP_DEBUG;
boolean asmCode = true;
// (2) 打印结果
Printer printer = asmCode ? new ASMifier() : new Textifier();
PrintWriter printWriter = new PrintWriter(System.out, true);
TraceClassVisitor traceClassVisitor = new TraceClassVisitor(null, printer, printWriter);
new ClassReader(className).accept(traceClassVisitor, parsingOptions);
}
}
Der className-Wert wird auf den vollständig qualifizierten Namen der Klasse festgelegt. Dabei kann es sich um eine von uns selbst geschriebene Klasse handeln, z. B. „sample.HelloWorld“, oder um eine Klasse, die mit dem JDK geliefert wird, z. B. „java.lang.Comparable“.
Der asmCode-Wert wird auf true oder false gesetzt. Wenn es wahr ist, kann der entsprechende ASM-Code gedruckt werden. Wenn es falsch ist, kann die der Methode entsprechende Anweisung gedruckt werden.
Der parsingOptions-Wert ist auf eine Kombination aus ClassReader.SKIP_CODE, ClassReader.SKIP_DEBUG, ClassReader.SKIP_FRAMES und ClassReader.EXPAND_FRAMES gesetzt. Er kann auch auf 0 gesetzt werden, um Informationen mit unterschiedlichen Detaillierungsebenen auszudrucken.
2. Plug-in
Wenn Sie IDEA sind, können Sie ASM ByteCode Viewer installieren, dann die Java-Datei auswählen, die Sie anzeigen möchten, mit der rechten Maustaste klicken und auswählen, und Sie können den entsprechenden ByteCode, ASMified und Groovified im ASPPlugin auf der rechten Seite sehen. Oder das Plug-in ASM Bytecode Outline (die von mir verwendete Community-Version von IDEA hat den Effekt nicht erzielt)
6. TreeApi
Der Unterschied zwischen Core API und Tree API
- Vorteile der Tree API: Benutzerfreundlichkeit: Wenn eine Person noch nie mit Core API und Tree API in Kontakt gekommen ist, ist der Einstieg in die Tree API einfacher. Funktionalität: Bei der Implementierung komplexerer Funktionen ist die Tree API einfacher zu implementieren als die Core API.
- Vorteile der Core-API: Ausführungseffizienz: Unter der Voraussetzung, dass die gleiche Funktion realisiert wird, weist die Core-API eine höhere Ausführungseffizienz auf und benötigt weniger Zeit als die Tree-API. Speichernutzung: Die Core-API benötigt weniger Speicherplatz als die Tree-API.
- Der erste Punkt ist, dass in ASM, unabhängig davon, ob es sich um eine Kern-API oder eine Baum-API handelt, Vorgänge zur Klassengenerierung, Klassentransformation und Klassenanalyse ausgeführt werden können.
- Der zweite Punkt ist, dass Core API und Tree API ihre eigenen Vorteile haben. Die Tree-API ist benutzerfreundlich und ermöglicht die Implementierung komplexer Vorgänge; die Core-API wird schneller ausgeführt und benötigt weniger Speicherplatz.
Dieser Artikel wurde aus Platzgründen gelöscht. Bei Interesse wenden Sie sich bitte zur Kommunikation an den Autor.
7. In diesem Artikel verwendete Tools
1、FileUtils
public class FileUtils {
public static String getFilePath(String relativePath) {
String dir = FileUtils.class.getResource("/").getPath();
return dir + relativePath;
}
}
8. Überlegen Sie, was wir in Zukunft mit ASM machen können?
1. Klassen generieren ---- Klassen basierend auf Vorlagen generieren (kombiniert mit Gerüsten zum schnellen Erstellen von Projekten und frühen Projektmodulen zum schnellen Erstellen)
2. Ändern Sie die Klasse - Ändern Sie die Klasse gemäß der Vorlage (kombinieren Sie die spezifische Struktur, um die Klasse beim Kompilieren des Java-Quellcodes zu ändern und die .class-Bytecode-Datei zu generieren, um den Zweck der Verbesserung der Systemeffizienz und der Freigabe von Entwicklungsarbeitskräften zu erreichen. Dies ist möglich kann mit der IDEA-Plug-In-Entwicklung kombiniert werden. Entwickeln Sie Plug-Ins, die Lombok ähneln oder leistungsfähiger sind.)
Microsoft startet neue „Windows-App“ Xiaomi gibt offiziell bekannt, dass Xiaomi Vela vollständig Open Source ist und der zugrunde liegende Kernel NuttX Vite 5 ist . Alibaba Cloud 11.12 wurde offiziell veröffentlicht. Die Ursache des Fehlers wurde offengelegt: Anomalie des Access Key-Dienstes (Access Key). . GitHub-Bericht: TypeScript ersetzt Java und wird zum drittbeliebtesten. Die wundersame Operation des Sprachoperators: das Netzwerk im Hintergrund trennen, Breitbandkonten deaktivieren, Benutzer zum Wechseln optischer Modems zwingen ByteDance: Verwendung von KI zur automatischen Optimierung der Linux-Kernel-Parameter Microsoft Open Source Terminal Chat Spring Framework 6.1 offiziell GA OpenAI, ehemaliger CEO und Präsident Sam Altman & Greg Brockman, wechselt zu MicrosoftAutor: JD Health Ma Renxi
Quelle: JD Cloud Developer Community Bitte geben Sie beim Nachdruck die Quelle an