¡Enseñarle a escribir un formulario de calculadora simple en lenguaje C# (con archivos de código fuente y video de demostración)!
Tabla de contenido
Introducción e instalación de herramientas de desarrollo:
Proceso de escritura de la calculadora:
Aquí está el código fuente (archivo Form1.cs):
1. Insertar y cargar un nuevo formulario (ventana):
2. Inserte una imagen y haga que se muestre apropiadamente:
El siguiente es el código fuente del "huevo de Pascua" (archivo Form2.cs):
Demostración del efecto final:
Introducción a C#:
C# es un lenguaje de programación orientado a objetos seguro, estable, simple y elegante derivado de C y C++. Hereda las potentes características de C y C++ mientras elimina algunas de sus características complejas (como no tener macros y no permitir la herencia múltiple). C# combina la operación visual simple de VB y la alta eficiencia operativa de C++. Con su poderosa capacidad de operación, estilo de sintaxis elegante, funciones de lenguaje innovadoras y soporte de programación orientado a componentes conveniente , se ha convertido en el lenguaje preferido para el desarrollo de .NET .
Introducción e instalación de herramientas de desarrollo:
VS2022 se usa aquí, puede ir al sitio web oficial para descargar e instalar (enlace a continuación) Visual Studio: IDE y editor de código para desarrolladores de software y equipos
Además de su gran tamaño, se puede decir que Visual Studio 2022 es un IDE muy potente. Puede escribir en varios idiomas y tiene un compilador incorporado. La función de finalización de código también es muy buena.
Proceso de escritura de la calculadora:
En primer lugar, después de crear un nuevo archivo de formulario, lo que aparece es un formulario en blanco (ventana), el valor predeterminado es Form1, y la interfaz de usuario de nuestra calculadora se dibujará en él. Aquí usamos "controles", que es, como Botones y cuadros de texto son las cosas que necesitamos en uso.
Si la caja de herramientas al lado no se muestra después de abrir vs2022, puede abrirla en Ver --> Caja de herramientas o CTRL+ALT+X;
Las categorías aquí nos facilitan encontrar las herramientas que necesitamos
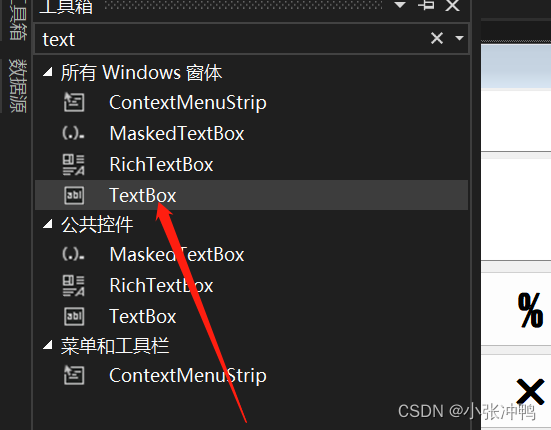
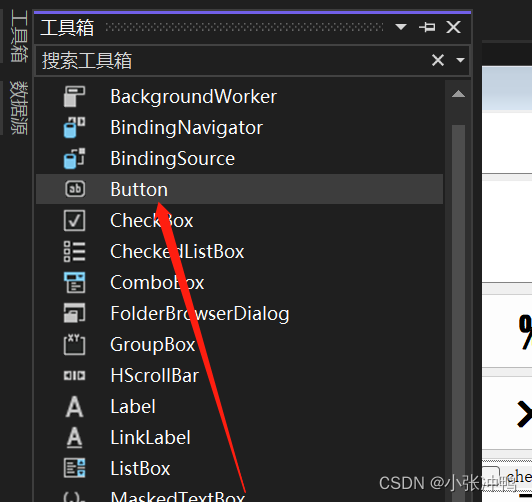
Después de crear un nuevo control, encontraremos que necesitamos establecer su título, tamaño y fuente del contenido dentro, etc.
Aquí se necesitan atributos.
Necesitamos hacer clic en el control, seleccionarlo, hacer clic derecho, luego hacer clic en Propiedades y luego modificar las propiedades.
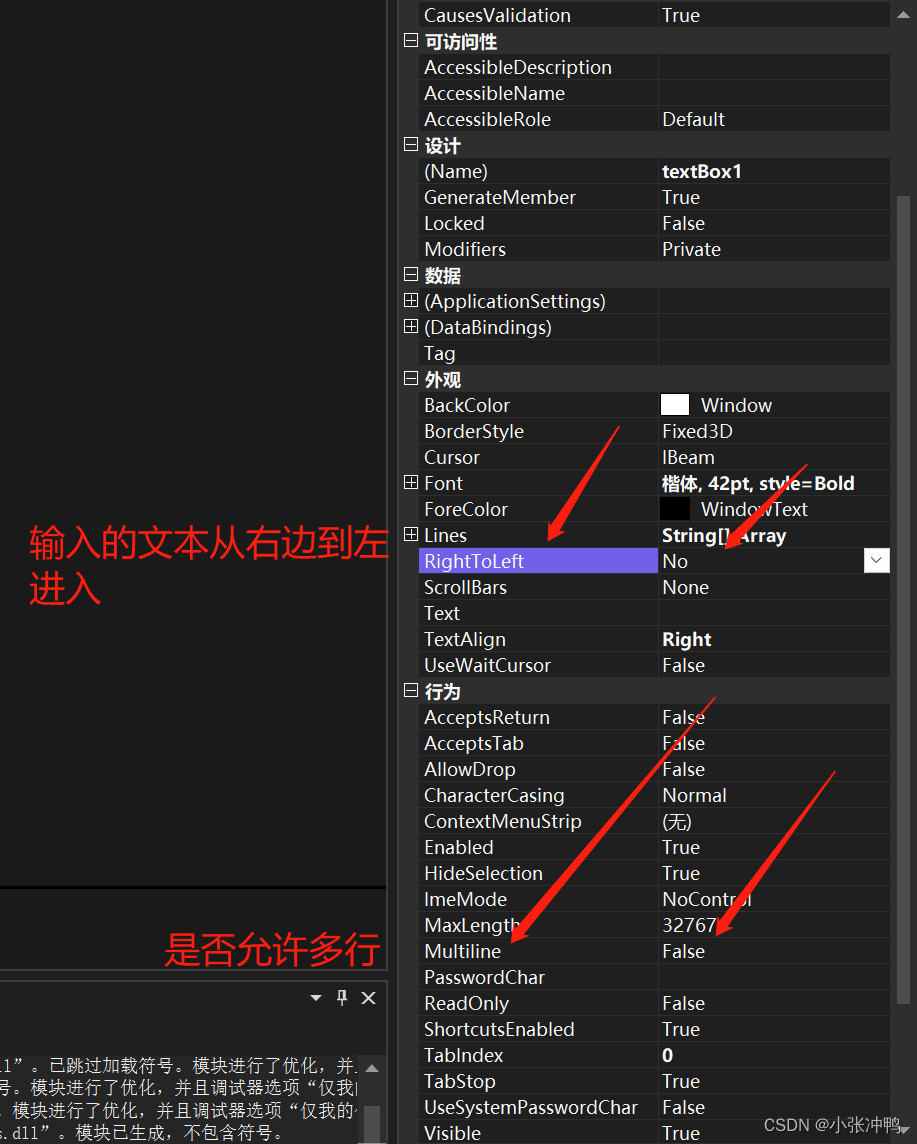
Hacemos doble clic en cualquier botón (control) para ingresar al área de edición de código
Encontraremos que cada control, incluido el formulario, corresponde a una función, el resto de nuestro trabajo es editar el código, completar estas funciones y conectarlas para que el código se ejecute sin problemas y logre el efecto lógico que queremos.
Aquí está el código fuente (archivo Form1.cs):
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Runtime.Remoting.Messaging;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 计算器
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button2_Click(object sender, EventArgs e)
{
textBox1.Text += "1";
}
private void button1_Click(object sender, EventArgs e)
{
textBox1.Text += "2";
}
private void button9_Click(object sender, EventArgs e)
{
textBox1.Text += "3";
}
private void button5_Click(object sender, EventArgs e)
{
textBox1.Text += "4";
}
private void button4_Click(object sender, EventArgs e)
{
textBox1.Text += "5";
}
private void button3_Click(object sender, EventArgs e)
{
textBox1.Text += "6";
}
private void button8_Click(object sender, EventArgs e)
{
textBox1.Text += "7";
}
private void button7_Click(object sender, EventArgs e)
{
textBox1.Text += "8";
}
private void button6_Click(object sender, EventArgs e)
{
textBox1.Text += "9";
}
private void button11_Click(object sender, EventArgs e)
{
textBox1.Text += "0";
}
private void button12_Click(object sender, EventArgs e)
{
if (textBox1.Text.Contains(".")) return;
else
{ if (textBox1.Text != "") textBox1.Text += ".";
}
}
private void button10_Click(object sender, EventArgs e)
{
textBox1.Text = "";
textBox2.Text = "";
chu.Enabled = true;
cheng.Enabled = true;
jia.Enabled = true;
jian.Enabled = true;
}
private void button13_Click(object sender, EventArgs e)
{
if (textBox1.Text != ""&& textBox1.Text != "-") textBox1.Text = Convert.ToString(double.Parse(textBox1.Text) * -1);
else if (textBox1.Text != "-") textBox1.Text += "-";
}
private void button14_Click(object sender, EventArgs e)
{
if(textBox1.Text!=""&& textBox1.Text != "-") textBox1.Text = Convert.ToString(double.Parse(textBox1.Text) * 0.01);
}
private void chu_Click(object sender, EventArgs e)
{
chu.Enabled = false;
cheng.Enabled = true;
jia.Enabled = true;
jian.Enabled = true;
if(textBox1.Text!="")
{
textBox2.Text = textBox1.Text;
textBox1.Text = "";
}
else textBox2.Text = "0";
}
private void cheng_Click(object sender, EventArgs e)
{
chu.Enabled = true;
cheng.Enabled = false;
jia.Enabled = true;
jian.Enabled = true;
if (textBox1.Text != "")
{
textBox2.Text = textBox1.Text;
textBox1.Text = "";
}
else textBox2.Text = "0";
}
private void jian_Click(object sender, EventArgs e)
{
chu.Enabled = true;
cheng.Enabled = true;
jia.Enabled = true;
jian.Enabled = false;
if (textBox1.Text != "")
{
textBox2.Text = textBox1.Text;
textBox1.Text = "";
}
else textBox2.Text = "0";
}
private void jia_Click(object sender, EventArgs e)
{
chu.Enabled = true;
cheng.Enabled = true;
jia.Enabled = false;
jian.Enabled = true;
if (textBox1.Text != "")
{
textBox2.Text = textBox1.Text;
textBox1.Text = "";
}
else textBox2.Text = "0";
}
private void button19_Click(object sender, EventArgs e)
{
double hou;
double qian = double.Parse(textBox2.Text);
if (textBox1.Text == "") hou = 0;
else hou = double.Parse(textBox1.Text);
if(chu.Enabled==false)
{
if (hou != 0)
{
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(qian / hou);
chu.Enabled = true;
}
else
{
MessageBox.Show("除数不能为0", "温馨提示");
textBox1.Text = "";
return;
}
}
if (cheng.Enabled == false)
{
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(qian * hou);
cheng.Enabled = true;
}
if (jia.Enabled == false)
{
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(qian + hou);
jia.Enabled = true;
}
if (jian.Enabled == false)
{
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(qian - hou);
jian.Enabled = true;
}
if (mod.Enabled == false)
{
double s = double.Parse(textBox1.Text);
int a = (int)s;
if (a != s)
{
MessageBox.Show("小数不能取余数!");
textBox1.Text = "";
return;
}
else
{
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(qian % hou);
mod.Enabled = true;
}
}
}
private void button15_Click(object sender, EventArgs e)
{
double r=double.Parse(textBox1.Text);
if(r<0)
{
MessageBox.Show("被开方数不能为0");
textBox1.Text = "";
return;
}
else
{
textBox1.Text = Convert.ToString(Math.Sqrt(r));
}
}
private void button16_Click(object sender, EventArgs e)
{
double s = double.Parse(textBox1.Text);
int a=(int)s;
if(a!=s)
{
MessageBox.Show("小数不能取余数!");
textBox1.Text = "";
return;
}
else
{
mod.Enabled = false;
chu.Enabled = true;
cheng.Enabled = true;
jia.Enabled = true;
jian.Enabled = true;
if (textBox1.Text != "")
{
textBox2.Text = textBox1.Text;
textBox1.Text = "";
}
else textBox2.Text = "0";
}
}
private void button17_Click(object sender, EventArgs e)
{
if (textBox1.Text != "") textBox1.Text = textBox1.Text.Substring(0, textBox1.Text.Length - 1);
else return;
}
private void button16_Click_1(object sender, EventArgs e)
{
Form2 B2 = new Form2();
B2.Show();
}
private void button18_Click(object sender, EventArgs e)
{
double r = double.Parse(textBox1.Text);
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(r*r);
}
private void button20_Click(object sender, EventArgs e)
{
double s = double.Parse(textBox1.Text);
int a = (int)s;
if (a != s)
{
MessageBox.Show("小数不能求阶乘");
textBox1.Text = "";
return;
}
else
{
if(a==0)
{
textBox1.Text = "1";
return;
}
int cnt = 1;
for(int i = 1; i <= a; i++)
{
cnt *= i;
}
textBox2.Text = "结果如下";
textBox1.Text = Convert.ToString(cnt);
}
}
}
}
Huevos de Pascua:
Esta calculadora también tiene otra función de "huevo de pascua" (como se muestra a continuación)
Aquí tenemos dos cosas que aprender:
1. Insertar y cargar un nuevo formulario (ventana):
Primero, abra el Explorador de soluciones en la vista
Luego seleccione el elemento, haga clic con el botón derecho del mouse y busque el formulario en Agregar .
2. Inserte una imagen y haga que se muestre apropiadamente:
Primero, inserte el control PictureBox deseado
Después de hacer clic para seleccionar, haga clic derecho para seleccionar la imagen
Luego seleccione el archivo local e inserte
Luego modifique las propiedades de la imagen y modifique su método de llenado de tamaño
Luego, diseñamos la interfaz de usuario de la misma manera que Form1 y escribimos y completamos las funciones.
El siguiente es el código fuente del "huevo de Pascua" (archivo Form2.cs):
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 计算器
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button16_Click(object sender, EventArgs e)
{
if(textBox1.Text=="")
{
MessageBox.Show("请输入年收入!");
return;
}
else
{
double r=double.Parse(textBox1.Text);
double cnt = 0;
if (r <= 36000) cnt = r * 0.03;
else if (r <= 144000) cnt = 36000 * 0.03 + (r-36000) * 0.1;
else if (r <= 300000) cnt = 36000 * 0.03 + (144000 - 36000) * 0.1+ (r- 144000)*0.2;
else if (r <= 420000) cnt = 36000 * 0.03 + (144000 - 36000) * 0.1 + (300000- 144000) * 0.2+(r-30000)*0.25;
else if (r <= 660000) cnt = 36000 * 0.03 + (144000 - 36000) * 0.1 + (300000 - 144000) * 0.2 + (420000 - 30000) * 0.25+(r-420000)*0.3;
else if (r <= 960000) cnt = 36000 * 0.03 + (144000 - 36000) * 0.1 + (300000 - 144000) * 0.2 + (420000 - 30000) * 0.25 + (660000 - 420000) * 0.3+(r-660000)*0.35;
else if (r > 960000) cnt = 36000 * 0.03 + (144000 - 36000) * 0.1 + (300000 - 144000) * 0.2 + (420000 - 30000) * 0.25 + (660000 - 420000) * 0.3 + (960000 - 660000) * 0.35+(r-960000)*0.45;
textBox2.Text=Convert.ToString(cnt);
}
}
private void Form2_Load(object sender, EventArgs e)
{
}
}
}
Demostración del efecto final:
Demostración del efecto de la calculadora simple de C#
¡Ayuda a la gente hasta el final! El enlace para compartir el archivo del código fuente es el siguiente:
Haga clic en mi enlace para ir directamente, ¡recuerde darle me gusta y apoyarlo! https://pan.baidu.com/s/1EmbrvxnQRJIWXbFyMayNzQ?pwd=6666 Código de extracción: 6666
(¡Al recién llegado Xiaobai que publica que a los grandes no les gusta, no lo rocíes!)
Si tiene alguna pregunta, no dude en ponerse en contacto conmigo. ! !
¡Gracias por el apoyo! ! !