53. Maximum subarray sum
53. Maximum subarray sum
Topic description:
Give you an integer array nums
, please find a continuous subarray with the maximum sum (the subarray contains at least one element), and return its maximum sum.
Subarray is a continuous part of the array. 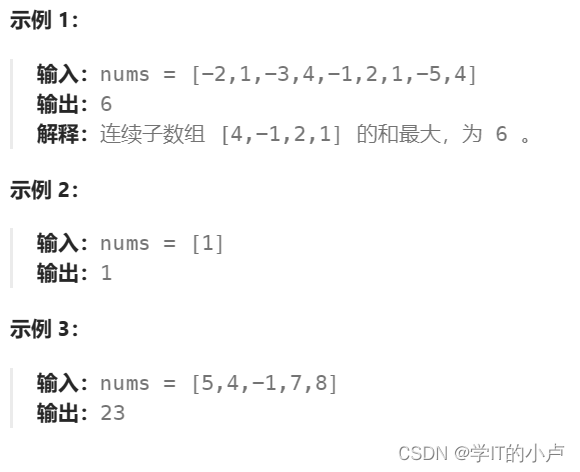
Problem-solving ideas:
Status means:
dp[i] represents the sum of elements of the largest subarray ending at position i
State transition equation:
dp[i]=max(dp[i-1],0)+nums[i];
initialization:
dp【0】=nums【0】
Order of filling in the form: left to right
Return value: maximum value in the range 0-n-1
Solution code:
ass Solution {
public:
int maxSubArray(vector<int>& nums) {
int n=nums.size();
vector<int>dp(n,0);
dp[0]=nums[0];
for(int i=1;i<n;i++)
dp[i]=max(dp[i-1],0)+nums[i];
int ret=INT_MIN;
for(int i=0;i<n;i++)ret=max(ret,dp[i]);
return ret;
}
};
918. Maximum sum of circular subarrays
918. Maximum sum of circular subarrays
Topic description:
Given a circular integer array of length n
, return of the non-empty subarrays of 4> . nums
nums
Ring array means that the end of the array will be connected to the beginning to form a ring. Formally, the next element of nums[i]
is nums[(i + 1) % n]
and the previous element of nums[i]
is nums[(i - 1 + n) % n]
.
The subarray can contain each element in the fixed buffer nums
at most once. Formally, for the subarray nums[i], nums[i + 1], ..., nums[j]
, there does not exist i <= k1, k2 <= j
where k1 % n == k2 % n
.
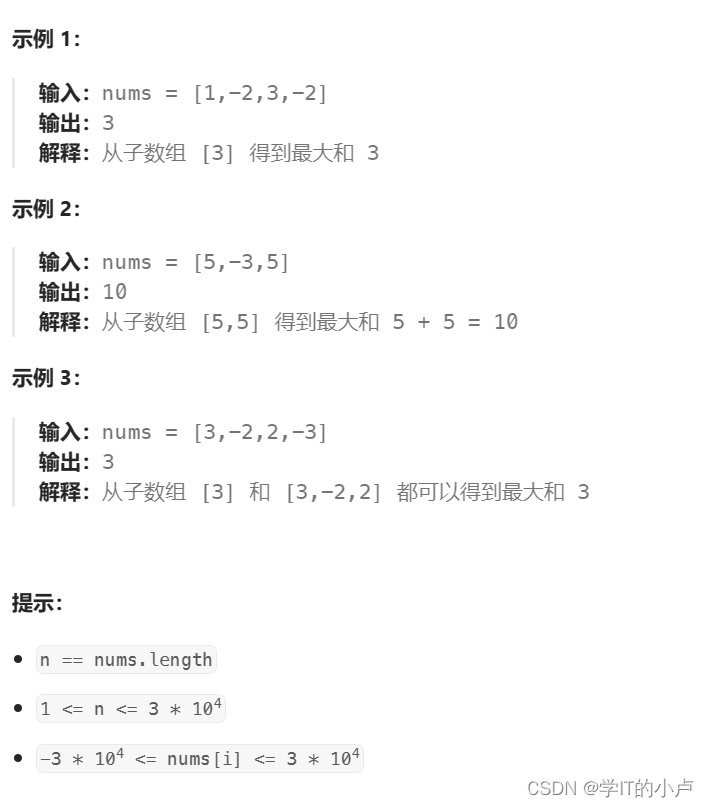
Problem-solving ideas:
The difference between this question and "Maximum subarray sum" is that when considering the problem, you must not only analyze the "continuous area within the array", but also consider
Consider the part of "arrays connected end to end". The possible results are divided into the following two situations:
i.
The result is inside the array, including the entire array;
ii.
The result is in the part connected to the beginning and end of the array.
Among them, for the first case, we only need to follow the method of "maximum subarray sum" to get the result, which is recorded as
fmax
.
For the second situation, we can analyze:
i.
If the part connected from the beginning to the end of the array is the largest array sum, then there will be an empty part in the middle of the array;
ii.
Because the sum of the array
sum
is unchanged, then the continuous part in the middle The sum of must be the smallest;
Therefore, we can draw a conclusion that the maximum sum in the second case should be equal to
sum - gmin
, Among them
gmin
represents the "minimum subarray sum" in the array.
The maximum value in the two situations is the result we want.
However, since the array may all contain negative numbers, the result in the first case is the maximum value in the array (which is a negative number), and the result in the first case is the maximum value in the array (which is a negative number).
In the two cases
gmin == sum
, the result will be
0
. If you directly find the maximum value of the two, it will be
0
. But
The actual result should be the maximum value in the array. For this situation, we need to make a special judgment.
Since the method of "maximum subarray sum" has already been explained, here we only mention the solution process of "minimum subarray sum", which is actually the same as "maximum subarray sum".
The calculation methods for large subarrays and "" are consistent. Use
f
to represent the maximum sum, and
g
to represent the minimum sum. .
1.
Status display:
g[i]
means: "all subarrays" ending with
i
The minimum value of the sum.
2.
State transfer process:
All possibilities for g[i]
can be divided into the following two types:
i.
number of children
1
: At this time
g[i] = nums[i]
;
ii.
The length of the number of children
1
:At this time < a i=4>g[i]
应该等于 i - 1 As a result of the "ownership number group" Japanese
Minimum re-addition
nums[i]
, also is
g[i - 1 ] + nums[i]
.
Since what we want is the minimum sum of subarrays, it should be the minimum value in the two situations, so the transfer equation can be obtained:
g[i] = min(nums[i], g[i - 1] + nums[i])
。
3.
Initialization:
We can add an auxiliary node at the front to help us initialize. There are two points to note when using this technique:
i.
The value in the auxiliary node must ensure that the subsequent form filling is correct;
ii. Mapping relationship of
subscripts.
In this question, add a grid at the front and let
g[0] = 0
.
4.
Filling order:
According to the state transfer process, the order of filling out the form is "from left to right".
5.
Reply:
a.
Advanced
f
Maximum surface level ->
;
fmax
b.
reach
g
surface minimum ->
gmin
;
c.
sum ->
sum
;
b.
返回
sum == gmin ? fmax : max(fmax,sum-gmin)
Solution code:
class Solution {
public:
int maxSubarraySumCircular(vector<int>& nums) {
int n=nums.size();
if(n==1)return nums[0];
vector<int>f(n,0);
vector<int>g(n,0);
f[0]=nums[0],g[0]=nums[0];
int sum=nums[0];
for(int i=1;i<n;i++)
{
f[i]=max(f[i-1],0)+nums[i];
g[i]=min(g[i-1],0)+nums[i];
sum+=nums[i];
}
int max_num=INT_MIN;
int min_num=INT_MAX;
for(int i=0;i<n;i++)
{
max_num=max(max_num,f[i]);
min_num=min(min_num,g[i]);
}
if(sum==min_num)return max_num;
return max(max_num,sum-min_num);
}
};