Code first
a = [1,2,3,4,5,6,7]
b = a[0]
b = 0
a[3] = b
print(a,b)
parse
First look at the value of b
Let’s first see what b will print out
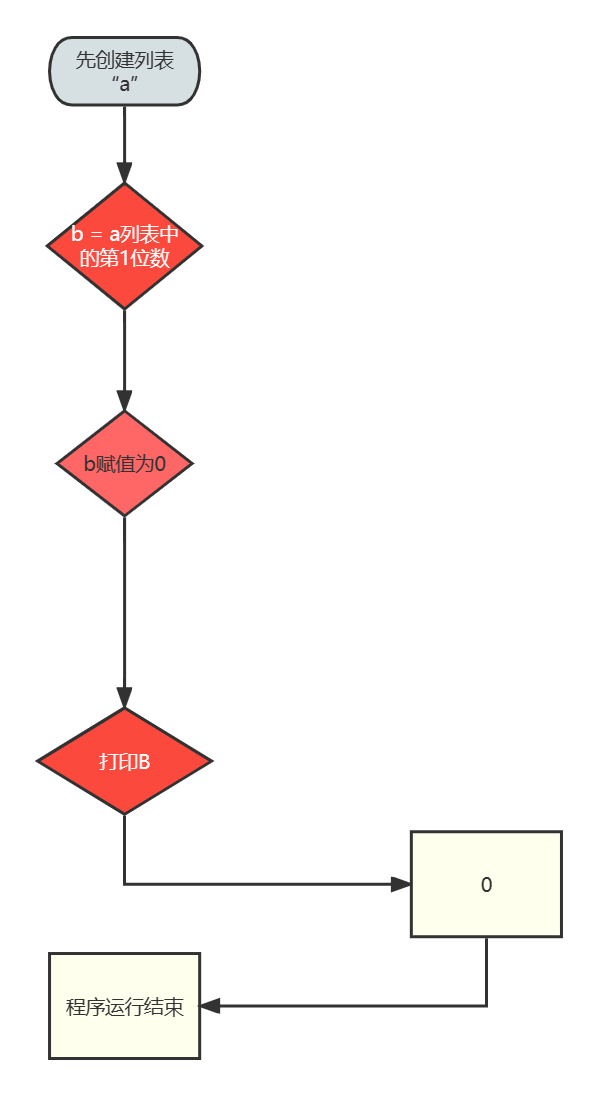
Note: In Python, 0 represents 1 , 1 represents 2 , and so on.
Look at the value of A
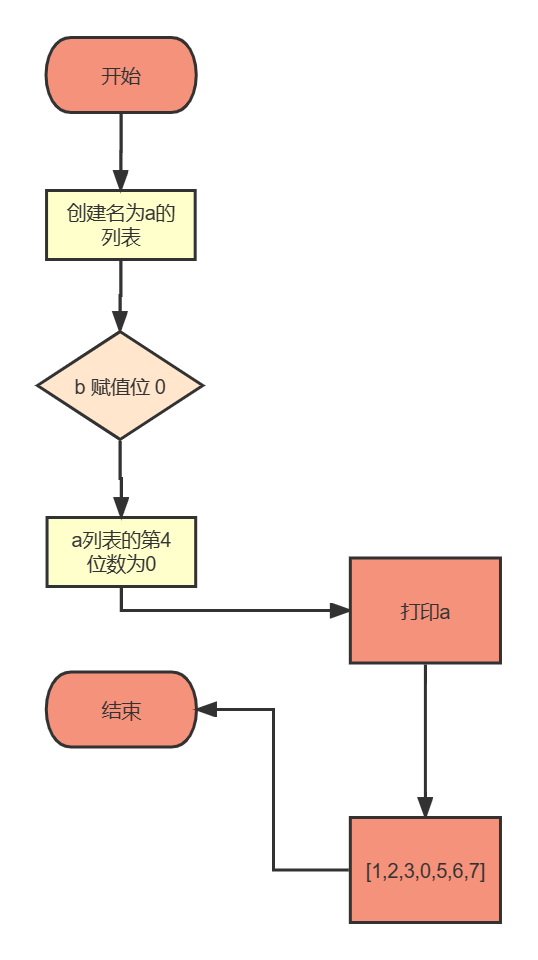
Note: In Python, 0 represents 1 , 1 represents 2 , and so on.
Overall running results
Since I did not add \n when printing , the printing is all on one line.

Summary (fake)
This is a list of the most commonly used and important knowledge in Python
continue class
Python has 4 operations on lists. These 4 are very commonly used, namely add, delete , modify, and search .
increase
append (the number to be added) is added at the end
How to call this function?
a = [1,2,3]
a.append(4) #在名为a的函数后面添加1
print(a)
控制台:
[1,2,3,4]
There is also a function
insert (index, number to be added) is added in front of the specified position
a = [1,3,4]
a.insert(1,2) #在列表的第1个数的前面添加2
print(a)
控制台:
[1,2,3,4]
delete
remove(element to be removed) specifies the element to be removed, not the index
a = [1,2,3]
a.remove(1)
print(a)
控制台:
[2,3]
clear() clear
a = [1,2,3]
a.clear()
print(a)
控制台:
[]
change
Fill in the index directly
a = [1,2,3]
a[1] = 1
print(a)
控制台:
[1,1,3]
The index can also be filled in as a range index
a = [1,2,3]
a[:1] = 0,0 #也可以写成a[0:1] = 0,0
print(a)
控制台:
[0,0,3]
You can also sort them
sort()
a = [3,1,2]
a.sort() #从小到达
print(a,"\n")
a = [3,1,2]
a.sort(reverse=True) #从大到小
print(a)
控制台:
[1,2,3]
[3,2,1]
check
index(value, the head value of the search range, the tail value of the search range) fill in the values in the list and output the value position
a = [1,2,3]
a.index(1)
print(a.index(2))
控制台:
1
Summary (really)
In this lesson, we learned about adding, deleting, modifying, and checking lists. After you finish learning, you will feel that your Python skills have improved!
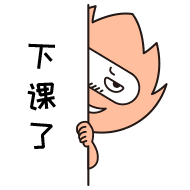