Table of contents
1. Namespace
1.1 Definition
1.2 Use
2.Default parameters
2.1 Concept
2.2 Classification
3. Function overloading
4. Quote
4.1 Concept
4.2 Features
4.3 Frequently quoted
4.4 The difference between references and pointers
5. Inline functions
1. Namespace
In
C/C++
, there are a large number of variables, functions, and classes to be learned later. The names of these variables, functions, and classes will all exist in the global scope, which may cause many conflicts. The purpose of using namespaces is to localize the names of identifiers
.
In order to
avoid naming conflicts or name pollution
,
the namespace
keyword appears to address this problem.
#include <stdio.h>
#include <stdlib.h>
int rand = 0;
// C语言没办法解决类似这样的命名冲突问题,所以C++提出了namespace来解决
int main()
{
printf("%d\n", rand);
return 0;
}
// 编译后后报错:error C2365: “rand”: 重定义;以前的定义是“函数”
1.1 Definition
To define a namespace, you need to use the
namespace
keyword
, followed by
the name of the namespace
,
and then followed by a pair of
{}
.
The {} are the members of the namespace.
// 1. Normal namespace definition
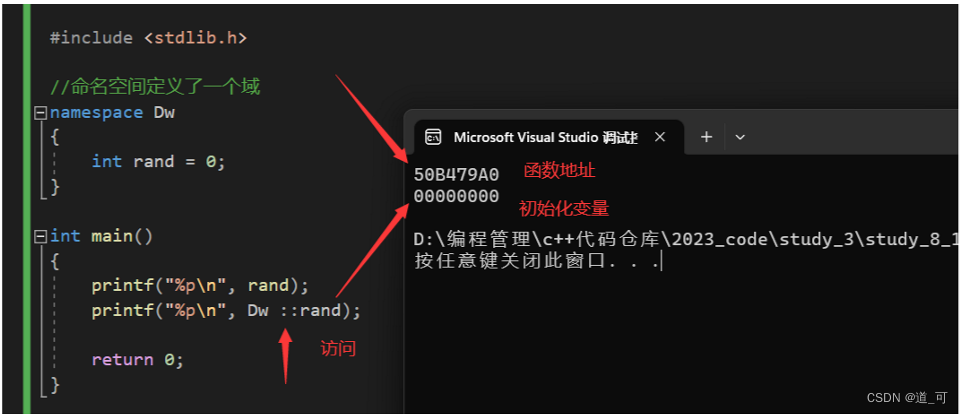
// Dw is the name of the namespace. In general development, the project name is used as the namespace name.
To put it simply: namespaces can define functions, variables, and structures. Relative to the compiler, are the rules to look for.
//2. Namespaces can be nested
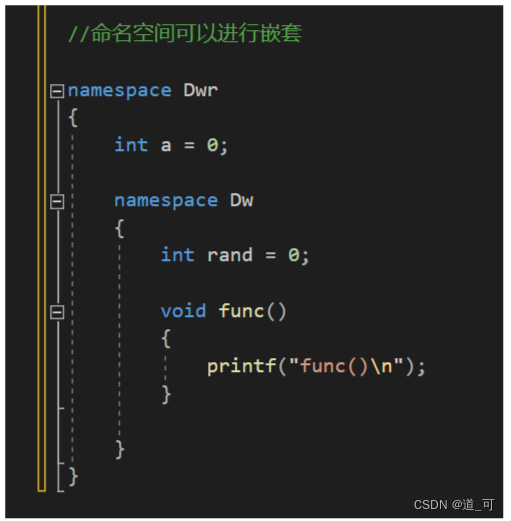
//3. Multiple namespaces with the same name are allowed to exist in the same project, and the compiler will eventually synthesize them into the same namespace.
1.2 Use
There are three ways to use namespaces:
- Add namespace name and scope qualifier
int main()
{
printf("%d\n", N::a);
return 0;
}
- Use using to introduce a member of the namespace
using N::b;
int main()
{
printf("%d\n", N::a);
printf("%d\n", b);
return 0;
}
- Use using namespace namespace name introduction
using namespce N;
int main()
{
printf("%d\n", N::a);
printf("%d\n", b);
Add(10, 20);
return 0;
}
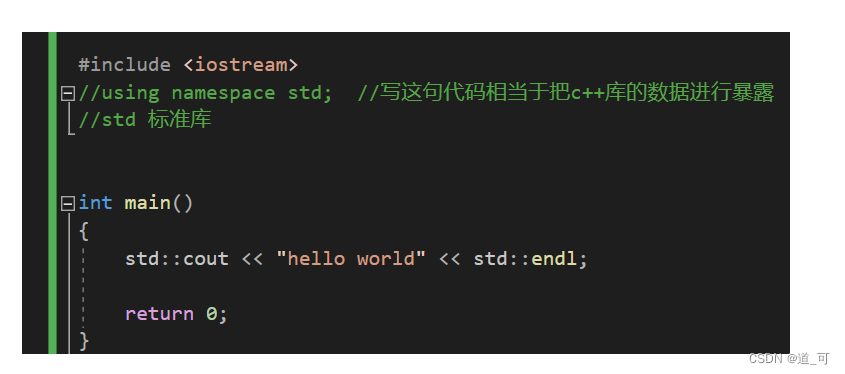
Therefore, for the sake of safety, it is recommended that when writing a project, try not to expand the std standard library, or expand commonly used parts:
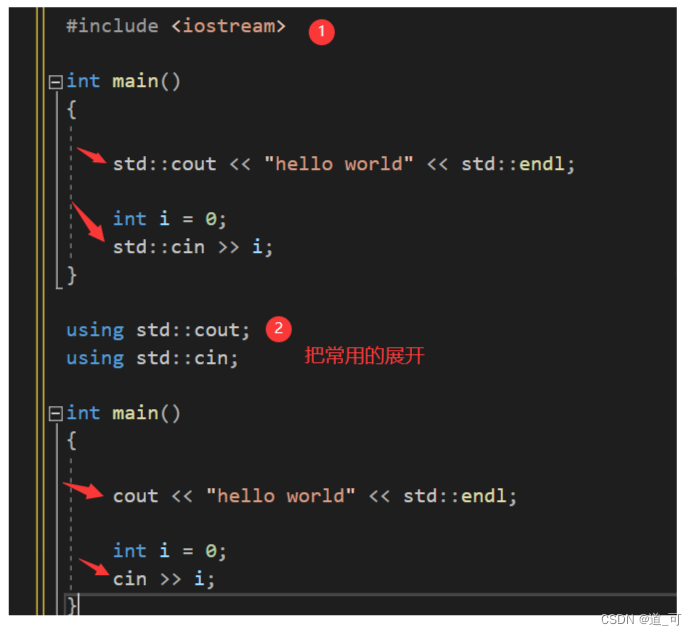
2.Default parameters
2.1 Concept
Default parameters
specify a default value for the function's parameters
when declaring or defining a function
. When calling this function, if no actual parameter is specified, the default value of the formal parameter is used, otherwise the specified actual parameter is used.
void Func(int a = 0)
{
cout<<a<<endl;
}
int main()
{
Func(); // 没有传参时,使用参数的默认值
Func(10); // 传参时,使用指定的实参
return 0;
}
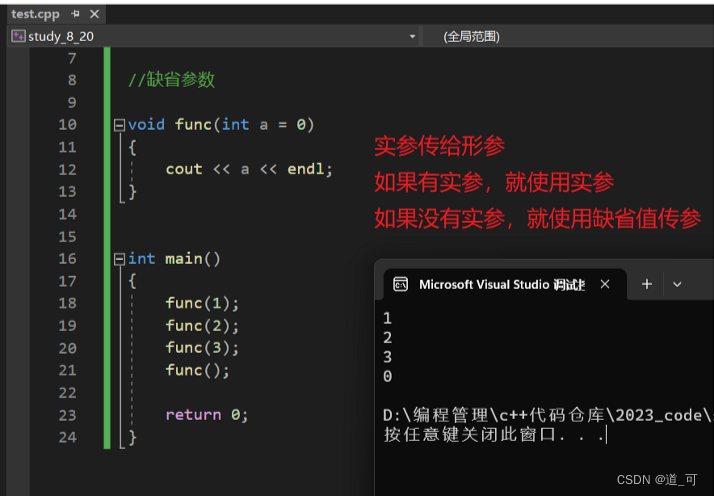
2.2 Classification
void Func(int a = 10, int b = 20, int c = 30)
{
cout<<"a = "<<a<<endl;
cout<<"b = "<<b<<endl;
cout<<"c = "<<c<<endl;
}
void Func(int a, int b = 10, int c = 20)
{
cout<<"a = "<<a<<endl;
cout<<"b = "<<b<<endl;
cout<<"c = "<<c<<endl;
}
scenes to be used:
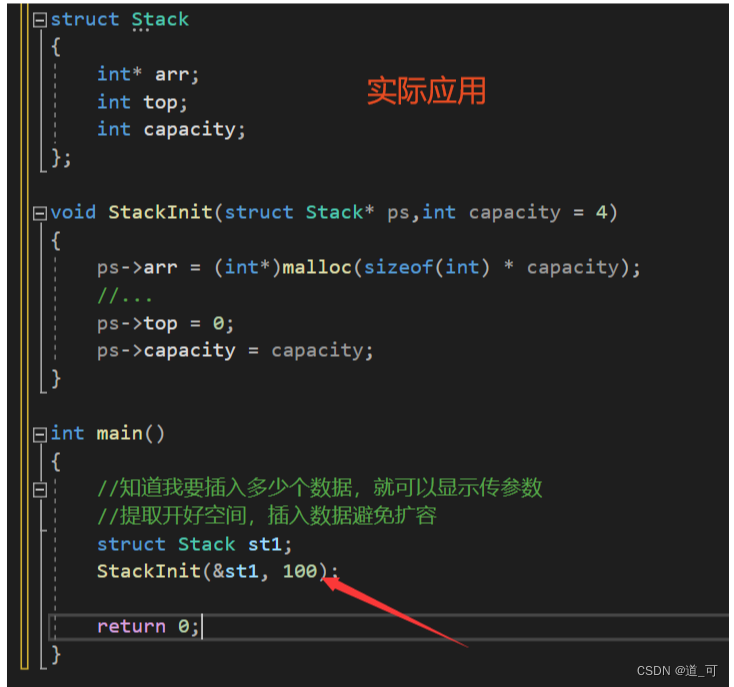
Notice:
1.
Semi-default parameters must be given
sequentially from right to left
, and cannot be given at intervals.
2. Default parameters cannot appear in the function declaration and definition at the same time (
if the declaration
and definition locations appear at the same time, and the values provided in the two locations happen to be different, the compiler cannot determine which
default value should be used.)
3.
The default value must be a constant or global variable
4. C
language is not supported (the compiler does not support it)
3. Function overloading
Function overloading:
It is a special case of functions.
C++
allows you
to declare several functions of the same name with similar functions in
the same scope
. These functions with the same name have different formal parameter lists ( number of parameters or types or type order ) . This is often used to deal with Implement functionally similar data types
Different questions.
- Parameter types are different
#include<iostream>
using namespace std;
int Add(int left, int right)
{
cout << "int Add(int left, int right)" << endl;
return left + right;
}
double Add(double left, double right)
{
cout << "double Add(double left, double right)" << endl;
return left + right;
}
- The number of parameters is different
void f()
{
cout << "f()" << endl;
}
void f(int a)
{
cout << "f(int a)" << endl;
}
- Parameter type order is different
void f(int a, char b)
{
cout << "f(int a,char b)" << endl;
}
void f(char b, int a)
{
cout << "f(char b, int a)" << endl;
}
To briefly mention, why does the c language not support function overloading, but c++ does?
Code generation files generally require 4 stages:
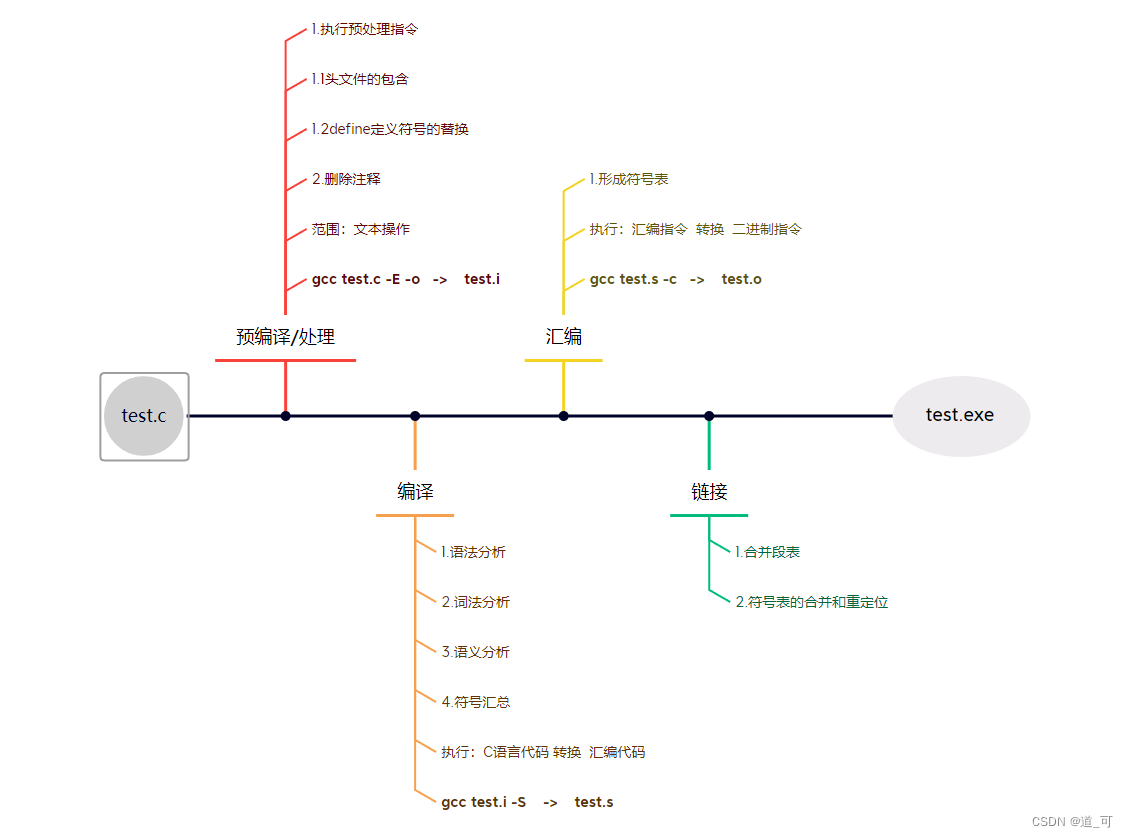
When C++ forms a symbol table, there are special function name modification rules. Even if the function name is the same but the parameter types are different, the symbol table formed will not be the same. C language cannot support overloading because functions with the same name cannot be distinguished. C ++ is distinguished by function modification rules. As long as the parameters are different, the modified names are different, and overloading is supported . In addition, if the function names and parameters of two functions are the same, but different return values do not constitute overloading, because the compiler cannot distinguish them when calling.
4. Quote
4.1 Concept
A reference
does not define a new variable,
but
gives an alias to an existing variable . The compiler will not allocate memory space for the reference variable. It shares the same memory space with the variable it refers to.
4.2 Features
1.
References
must be initialized when defined
2.
A variable can have multiple references
3.
Once a reference refers to an entity, it cannot refer to other entities.
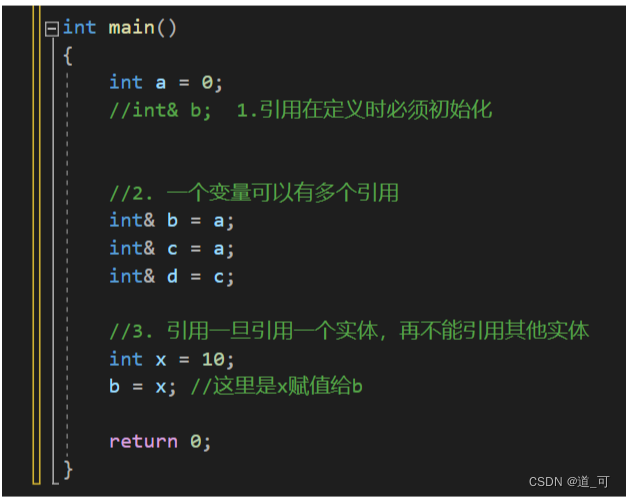
scenes to be used:
1. Make parameters
- Make output parameters
- Pass parameters through large objects to improve efficiency
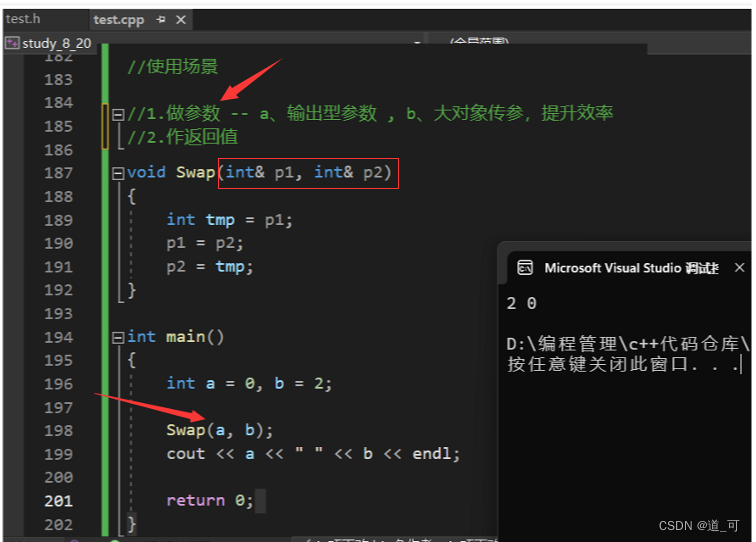
2. Make the return value
- Output type return object, the caller can modify the return object
- Reduce copies and improve efficiency
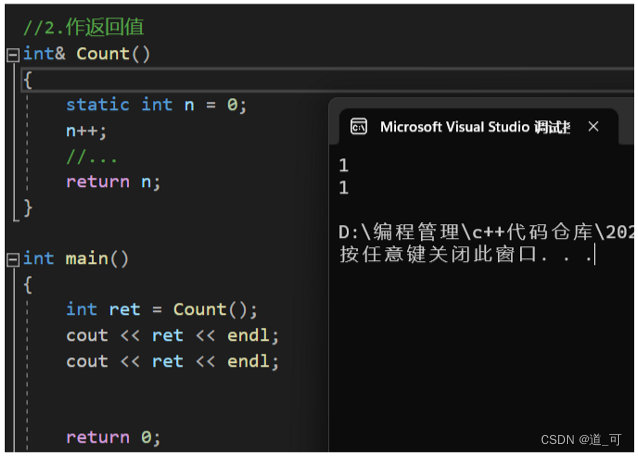
Note: If the function scope is exceeded and the returned object will be destroyed, then you must not use reference return, but use value-passing return.
4.3 Frequently quoted
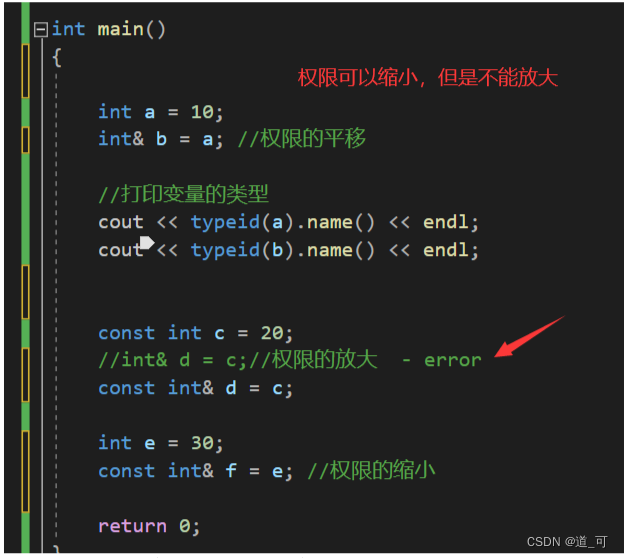
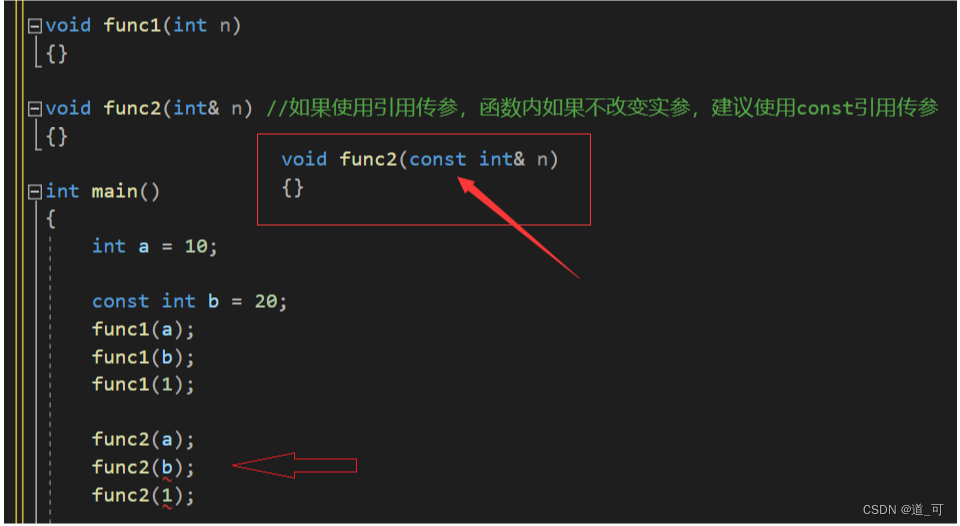
4.4 The difference between references and pointers
In
the grammatical concept,
a reference is an alias, which has no independent space and shares the same space with the entity it refers to.
There is actually space in
the underlying implementation , because
references are implemented in pointer fashion .
The difference between references and pointers
:
1.
Reference conceptually defines an alias of a variable, and a pointer stores a variable address.
2.
References
must be initialized
when defined
, and there are no requirements for pointers.
3. After a
reference
refers to an entity during initialization,
it can no
longer refer to other entities , and a pointer can point to anything at any time.
an entity of the same type
4.
There is no
NULL
reference
, but there is a
NULL
pointer
5. The meaning
in
sizeof
is different
:
the reference
result is
the size of the reference type
, but
the pointer
is always
the number of bytes occupied by the address space
(32
4
bytes
under bit platform
)
6.
The reference is self-increasing, that is, the referenced entity is increased by
1
, and the pointer is self-increasing, that is, the pointer is offset backward by the size of the type.
7.
There are multi-level pointers, but no multi-level references
8.
The way to access entities is different.
The pointer needs to be explicitly dereferenced, and the reference compiler handles it by itself.
9.
References are relatively safer to use than pointers
In simple terms:
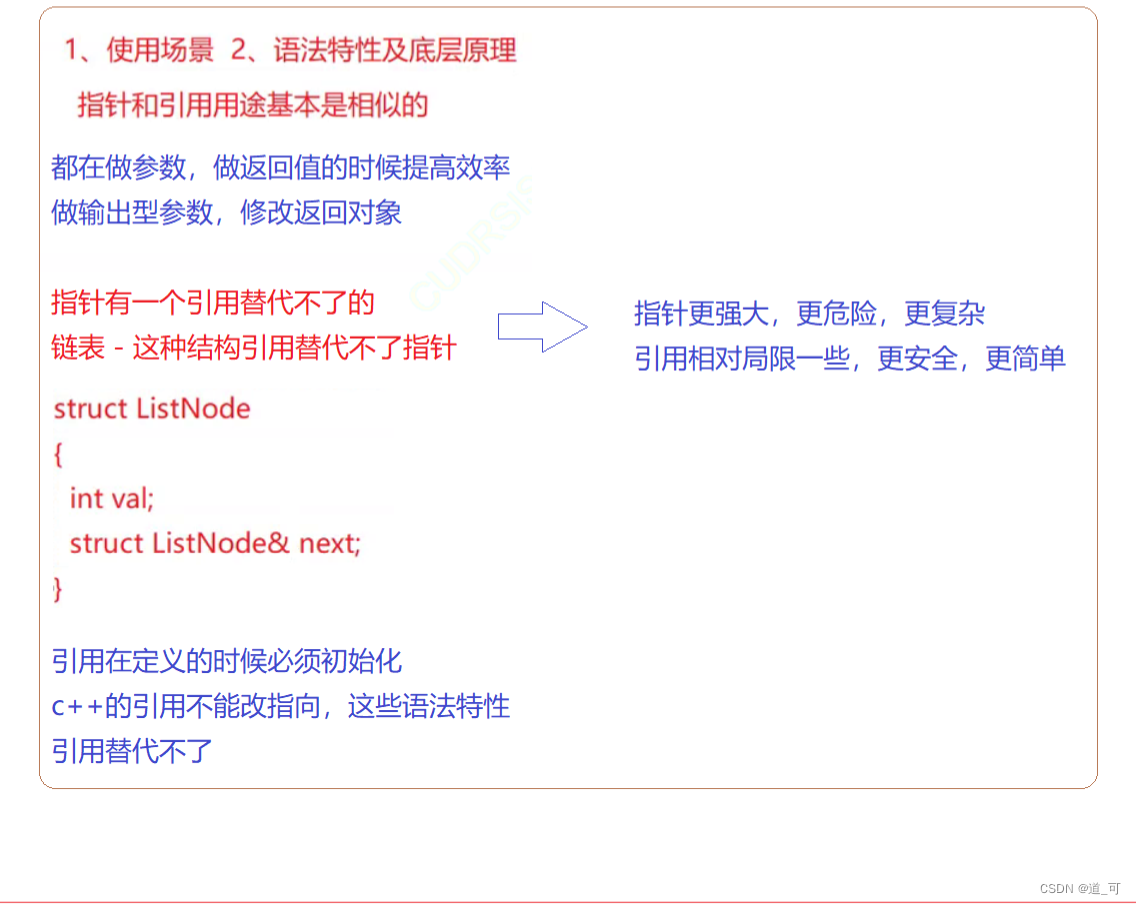
5. Inline functions
5.1
Concept
Functions modified with
inline
are called inline functions. When compiling , the C++ compiler will expand the inline function where it is called . There is no overhead of establishing a stack frame for function calls. Inline functions improve the efficiency of program operation.
5.2
Features
1. Inline
is a method of
exchanging space for time
. If the compiler treats a function as an inline function, during the
compilation phase, it will
Replace function calls with function bodies
. Disadvantages: the target file may become larger. Advantages: less calling overhead and improved program operation.
row efficiency.
2.
Inline
is just a suggestion for compilers. Different compilers may have different
inline
implementation mechanisms
. Generally, it is recommended that
Suggestion: Make
the function smaller
(
that is, the function is not very long, there is no accurate statement, it depends on the internal implementation of the compiler
)
,
not
Functions that
are recursive and frequently called
are inline
modified, otherwise the compiler will ignore
the inline
feature.
The picture below shows the suggestions on inline
in the fifth edition of
"C++prime " :
3. Inline
does not recommend the separation of declaration and definition, as separation will cause link errors. Because
inline
is expanded, there is no function address
, the link will not be found.
What are the pros and cons of macros?
advantage:
1.
Enhance code reusability.
2.
Improve performance.
shortcoming:
1.
It is inconvenient to debug macros. (Because of the replacement during the pre-compilation stage)
2.
This results in poor code readability, poor maintainability, and easy misuse.
3.
No type safety checks.
What techniques are available in C++
to replace macros
?
1.
Change constant definition to
const enum
2.
Replace short function definitions with inline functions
Note: The above conceptual content comes from Bit Technology.