background:
Use the enterprise WeChat to develop the scanning function, tools: developed in Ruoyi mobile terminal ruoyi-app.
Trusted domain name verification
(1) The trusted domain name of Enterprise WeChat needs to be consistent with the filing entity of Enterprise WeChat.
The domain name filing subject can view the domain name filing subject through the webmaster tool. https://icp.chinaz.com/
Enterprise WeChat filing entities can consult the administrator
(2) Configure the domain name attribution verification txt file through nginx
For details, see https://blog.csdn.net/zhaolulu916/article/details/128613264?spm=1001.2014.3001.5502
(3) The successful effect of web page authorization and JS-SDK configuration is as follows
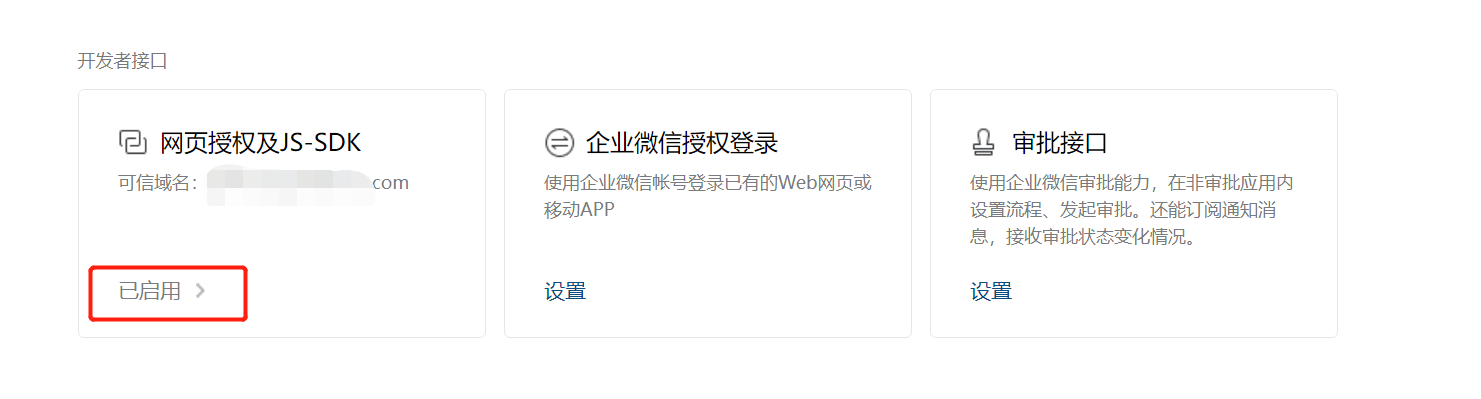
(4) The trusted domain name configuration is correct, but it cannot pass the verification, you can ask questions in the developer center. You can also configure an address with a port after redirection
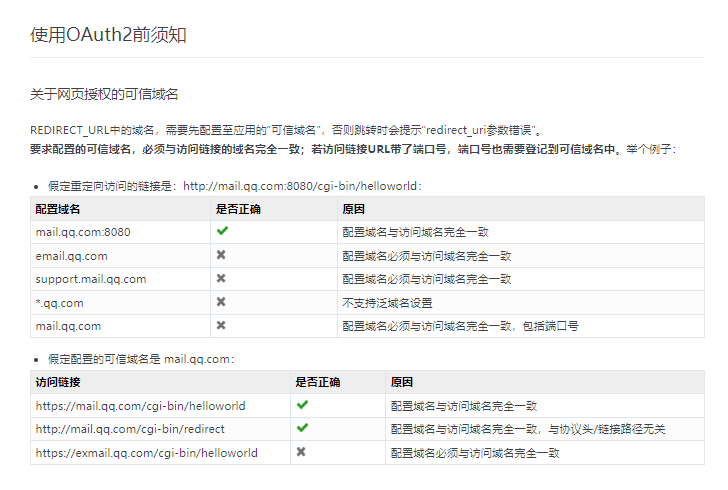
2. Whitelist configuration
The code is finally deployed to the server, and the server's IP is configured in the white list, so that debugging will not prompt that the IP is not in the white list. This interface is available in the system administrator interface.
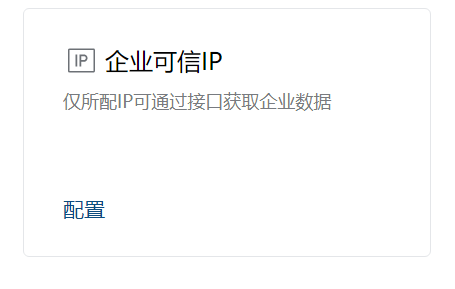
3. The front-end code obtains the enterprise WeChat signature and configures the JSSDK permission
(1) npm install weixin-js-sdk
npm install weixin-js-sdk --save
(2) Introduce weixin-js-sdk globally in main.js
import wx from 'weixin-js-sdk'
Vue.prototype.$wx = wx
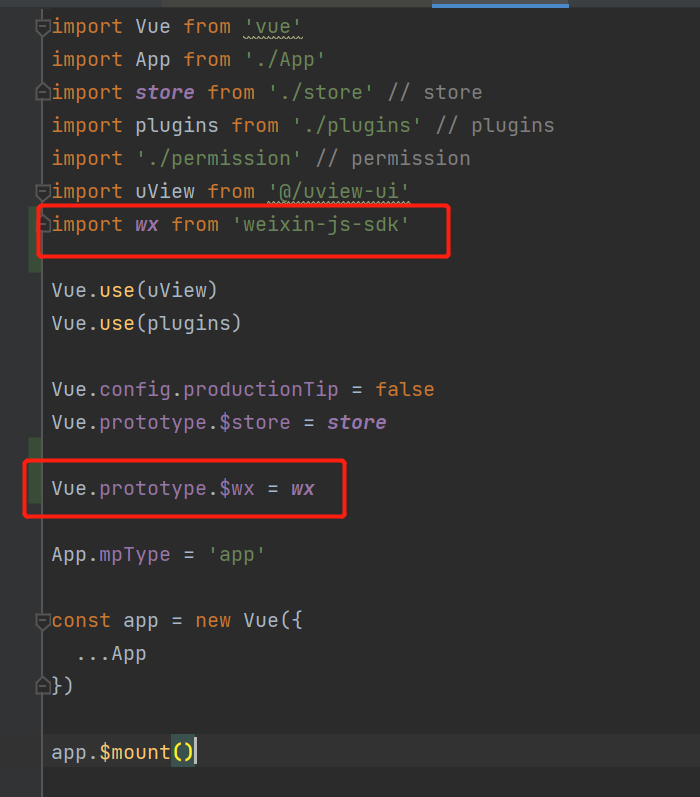
(3) Configure to obtain enterprise WeChat signature and use JSSDK rights
The url obtained at this time can be the ported one after domain name redirection, but this url needs to be registered as a trusted domain name on WeChat Work.
in the onload function
// Best to call in onLoad
onLoad: function () {
this.getCofig();
},
//Use this.$wx. in the getConfig function to call, otherwise an error will be reported.
// 配置信息
getCofig() {
const that = this;
let url = window.location.href.split('#')[0];
let params={'url':url}
getJsapiSignature(params).then(res => {
const result = res;
if (res) {
that.wxConfig(
result.appId,
result.timestamp,
result.nonceStr,
result.signature
);
} else {
alert('获取配置信息返回为空');
}
})
},
//wx.config的配置
wxConfig(appId, timestamp, nonceStr, signature) {
this.$wx.config({
debug: true, // 开启调试模式,
appId: appId, // 必填,企业号的唯一标识
timestamp: timestamp, // 必填,生成签名的时间戳
nonceStr: nonceStr, // 必填,生成签名的随机串
signature: signature, // 必填,签名
jsApiList:
[
'chooseImage',
'previewImage',
'uploadImage',
'downloadImage',
'scanQRCode',
'checkJsApi'
], // 必填,需要使用的JS接口列表
});
this.$wx.ready(() => {
this.$wx.checkJsApi({ //判断当前客户端版本是否支持指定JS接口
jsApiList: [
'chooseImage',
'previewImage',
'uploadImage',
'downloadImage',
'scanQRCode',
],
success: function (res) {// 以键值对的形式返回,可用true,不可用false。如:{"checkResult":{"scanQRCode":true},"errMsg":"checkJsApi:ok"}
this.$modal.msgSuccess(res.checkResult)
if(res.checkResult.scanQRCode != true){
this.$modal.msgSuccess('抱歉,当前客户端版本不支持扫一扫')
}
},
fail: function (res) { //检测getNetworkType该功能失败时处理
this.$modal.msgSuccess(res)
alert('checkJsApi error');
}
});
console.log("wxConfig配置完成,扫码前准备完成");
this.$modal.msgSuccess("wxConfig配置完成,扫码前准备完成")
})
this.$wx.error(function (res) {
this.$modal.msgSuccess('wxConfig出错了:' + res.errMsg)
console.log('wxConfig出错了:' + res.errMsg);
//wx.config配置错误,会弹出窗口哪里错误,然后根据微信文档查询即可。
});
},
(4) Add qywx.js to write api interface
export function getJsapiSignature(query) {
return request({
url: '/system/qywx/signature/',
method: 'get',
params: query
})
}
使用时引入
import { getJsapiSignature } from "@/api/notice/qywx.js"
4. 后端代码通过weixin-java-cp开发工具获取签名
后端使用的是https://github.com/binarywang/的weixin-java-cp开发工具
(1)引入企业微信开发工具
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-cp</artifactId>
<version>3.8.0</version>
</dependency>
(2)新增QywxController
@RestController
public class QywxController {
@Autowired
private QywxService qywxService;
@GetMapping(value = "/system/qywx/signature/")
public WxJsapiSignature getJsapiSignature(@RequestParam("url") String url) {
try { // 直接调用wxMpServer 接口
System.out.println("访问WxJsapiSignature=====/system/qywx/signature/"+url+"");
WxJsapiSignature wxJsapiSignature = qywxService.getJsapiSignture(url);
System.out.println("AppId==="+wxJsapiSignature.getAppId());
System.out.println("Timestamp==="+wxJsapiSignature.getTimestamp());
System.out.println("NonceStr==="+wxJsapiSignature.getNonceStr());
System.out.println("Signature==="+wxJsapiSignature.getSignature());
return wxJsapiSignature;
} catch (WxErrorException e) {
return null;
}
}
(3)新增QywxService,使用weixin-java-cp中的WxCpServiceImpl生成签名
@Service
public class QywxService {
//获取对应应用的签名
public WxJsapiSignature getJsapiSignture(String url) throws WxErrorException {
// 替换成自己应用的appId和secret,agentId
Integer agentId = 1111111;
String corpId="XXXXXXXX";
String corpSecret = "XXXXXXXX";
WxCpDefaultConfigImpl config = new WxCpDefaultConfigImpl();
config.setCorpId(corpId); // 设置微信企业号的appid
config.setCorpSecret(corpSecret); // 设置微信企业号的app corpSecret
config.setAgentId(agentId); // 设置微信企业号应用ID
WxCpServiceImpl wxCpService = new WxCpServiceImpl();
wxCpService.setWxCpConfigStorage(config);
System.out.println("WxJsapiSignature===url==="+url);
WxJsapiSignature wxJsapiSignature = wxCpService.createJsapiSignature(url);
//wxJsapiSignature中可以直接获取签名信息 且方法内部添加了缓存功能
return wxJsapiSignature;
}
}
(4)签名的结果验证
https://developer.work.weixin.qq.com/devtool/signature,其中jsapi_ticket是企业的ticket,不是应用的ticket
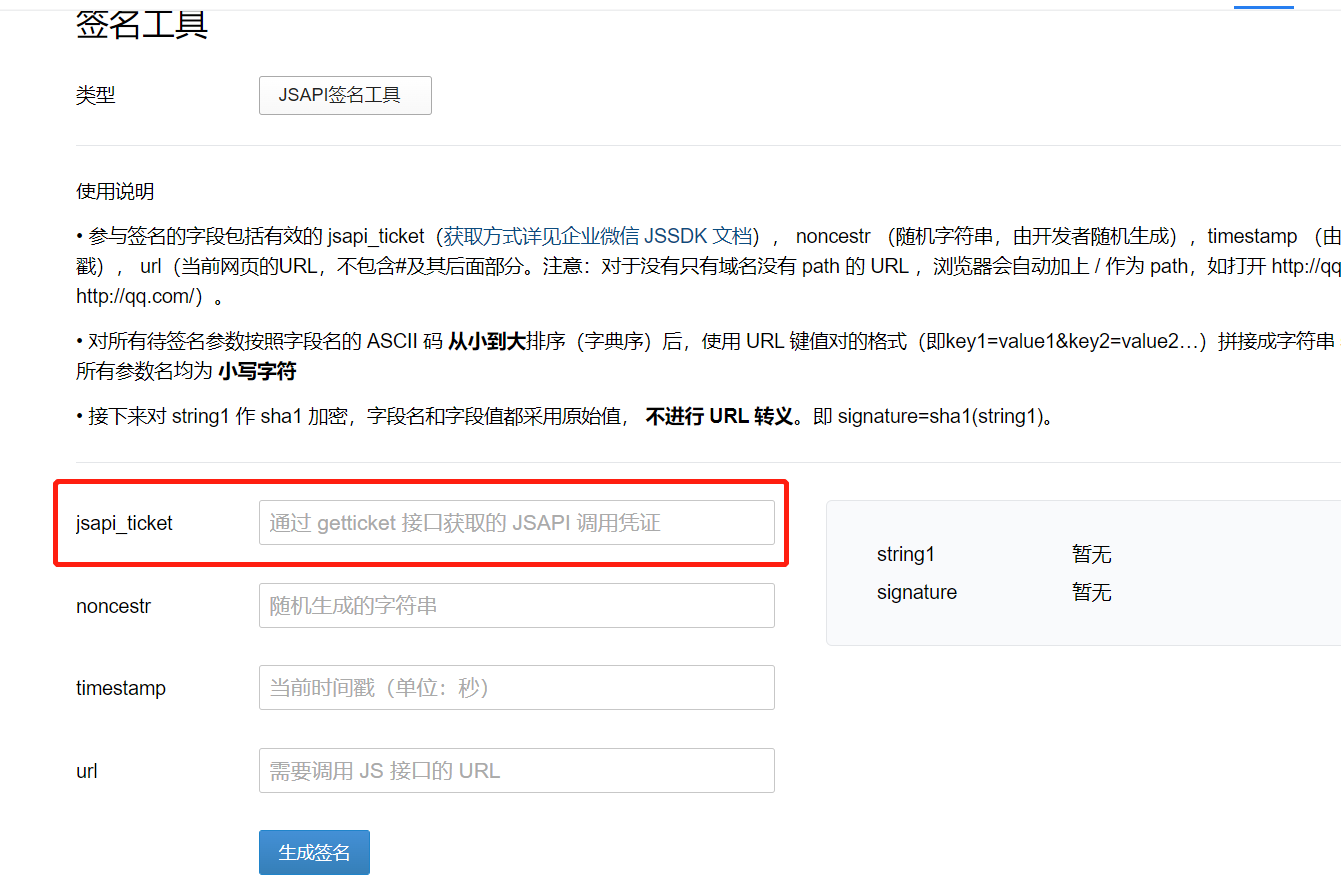
5. 扫一扫调用JSSDK代码
使用 this.$wx.进行调用,否则会报错。
onH5Scan(){
const that = this;
this.$modal.msgSuccess("onH5Scan进来了");
console.log('onH5Scan进来了');
this.$wx.scanQRCode({
needResult: 1, // 默认为0,扫描结果由微信处理,1则直接返回扫描结果,
scanType: ['qrCode', 'barCode'], // 可以指定扫二维码还是一维码,默认二者都有
success: function (res) {
var result = res.resultStr; // 当 needResult 为 1 时,扫码返回的结果
var resultArr = result.split(','); // 扫描结果以逗号分割数组
var codeContent = resultArr[resultArr.length - 1]; // 获取数组最后一个元素,也就是最终的内容
console.log('onH5Scan——success');
that.Info.qrcode=codeContent;
console.log("onH5Scan"+result+"==="+resultArr+"==="+codeContent);
},
fail: function (response) {
if(res.errMsg.indexOf('function_not_exist') > 0){
console.log('onH5Scan版本过低请升级');
}
uni.showToast({
icon: "none",
title: "onH5Scan——调用扫码失败"+response.errMsg
})
},
});
},
6. 扫一扫最终效果如下
以下效果图是代码部署到服务器上,配置成功提示如下
手机端
提示:
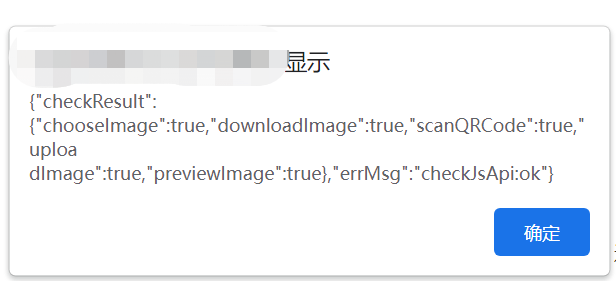
手机端效果:
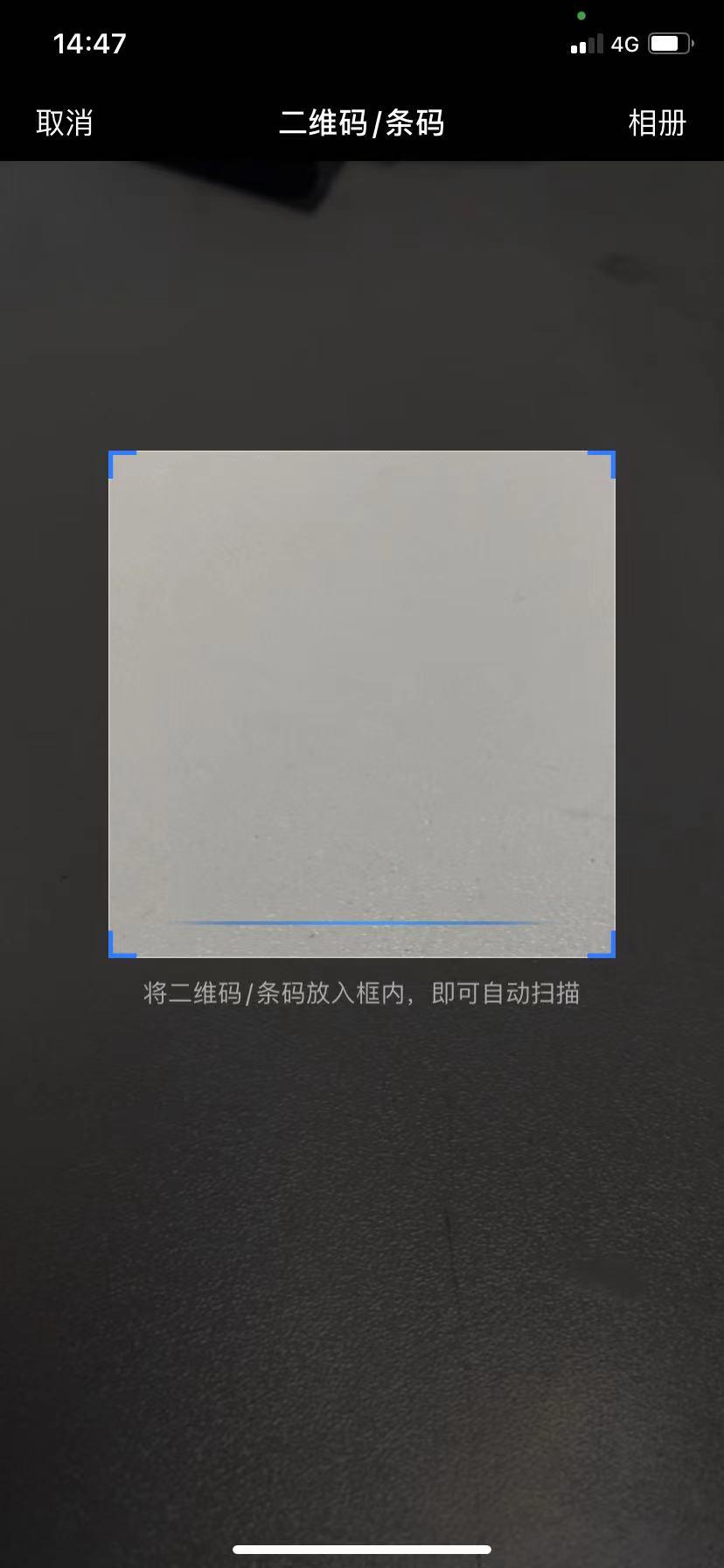
显示正常后,关闭调试模式
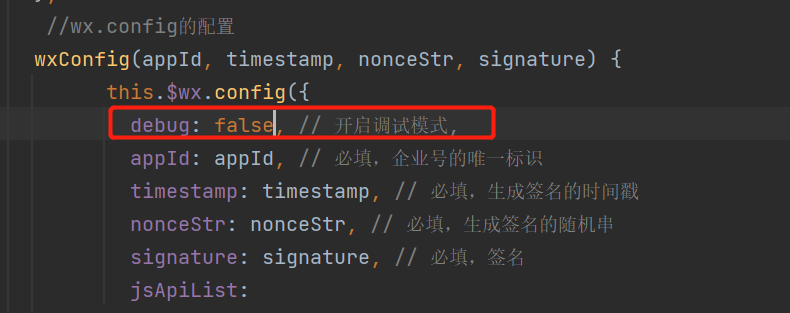
7. 参考文章
https://blog.csdn.net/weixin_40816738/article/details/123170569
https://developer.work.weixin.qq.com/document/path/90547
https://blog.csdn.net/weixin_45243487/article/details/125101558