background
Recently, I used JavaScript to write some browser RPA scripts. I encountered some problems in the process of using the scripts. The data used by the scripts is often stored in the excel sheet, but only json data can be read during runtime , resulting in frequent conversion of excel to json manually , which is inefficient.
After encountering a problem, I quickly searched for the excel to json gadget, and found that there are very few tools that can be used directly. Basically, only part of the code is provided, and there is no graphical interface.
Still fun to do it yourself. This article introduces the use of Python to develop an excel one-click to json tool, which converts any selected excel xlsx and xls files into json files, and displays the results in the interface.
Table of contents
1. Excel one-key to json gadget tutorial
The gadget uses Python Tkinter as the graphical GUI . To be honest, it is a bit ugly, but it is practical. The Window system can be run by double-clicking directly.
(1) Program running interface
The running effect of the gadget is as shown in the figure below:
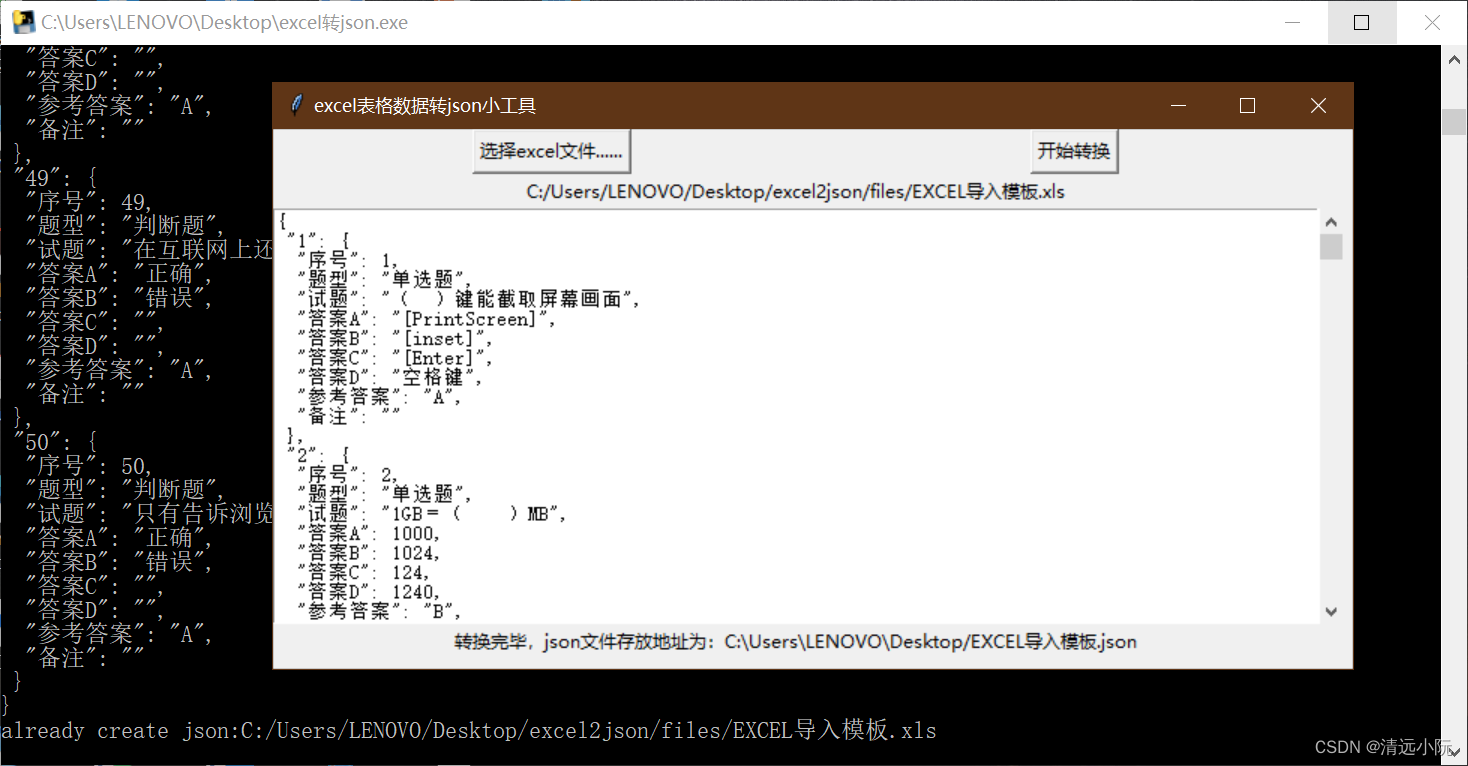
The comparison effect before and after converting excel to json , as shown in the figure below:
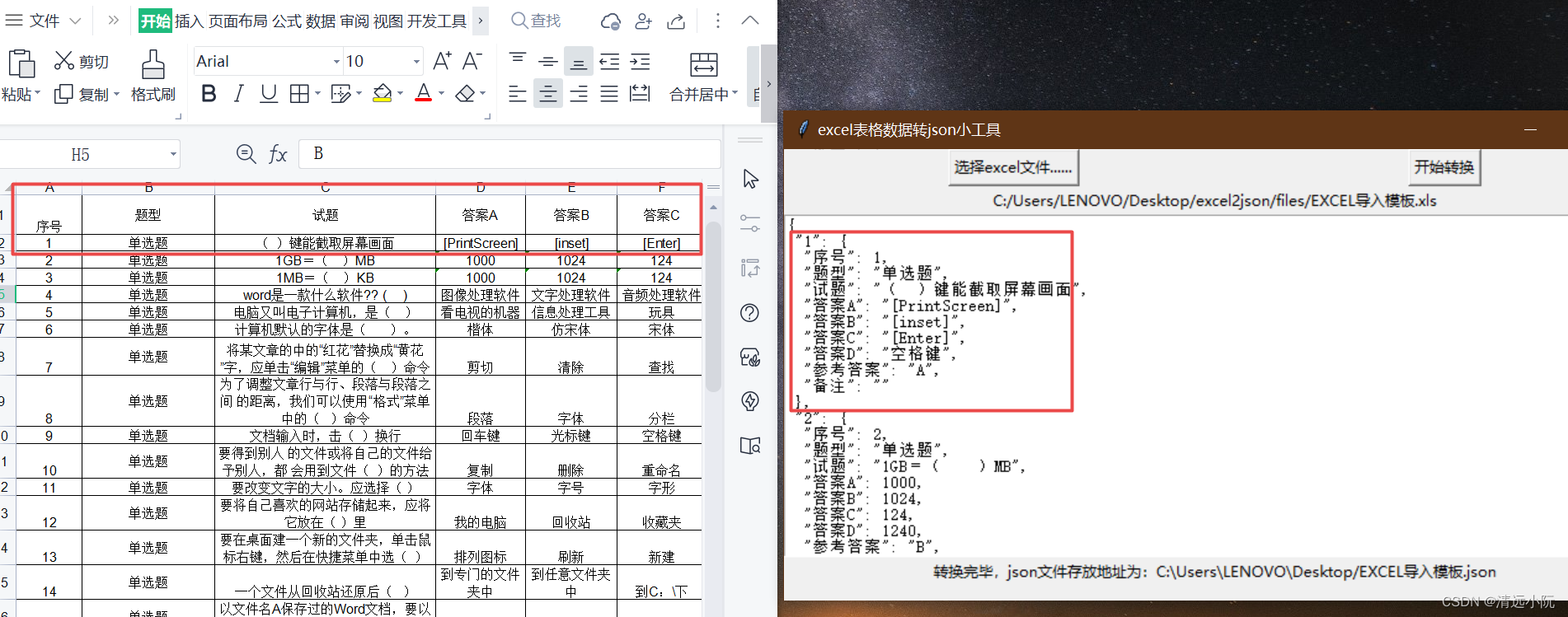
(2) Gadget operation steps
1) Select the excel file to be converted. It supports xlsx and xls formats. The form should be as simple as possible. It does not support complex nested json format conversion for the time being.
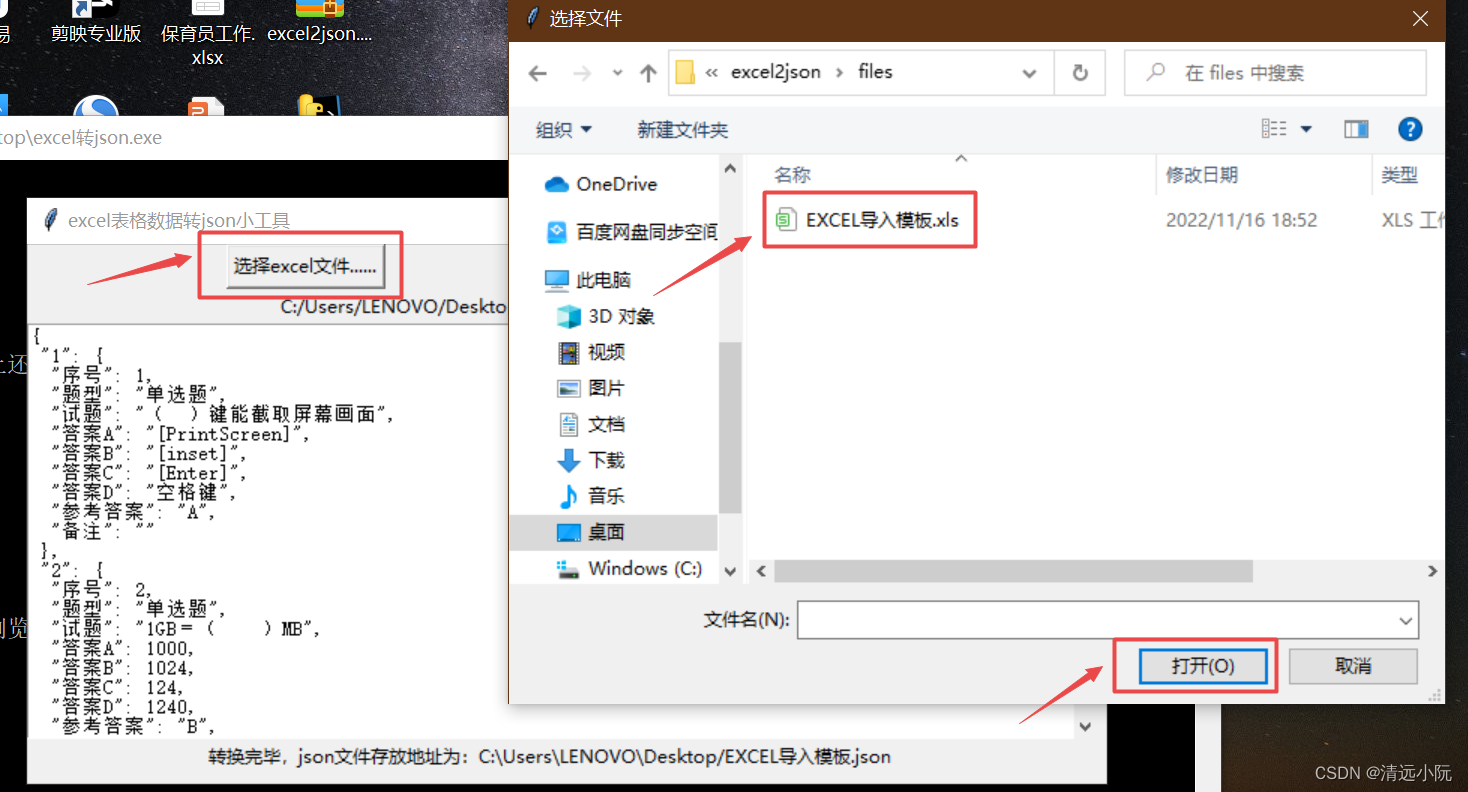
2) Click to start the conversion, and the converted result will be displayed in the interface program, which can be copied to other places at any time.
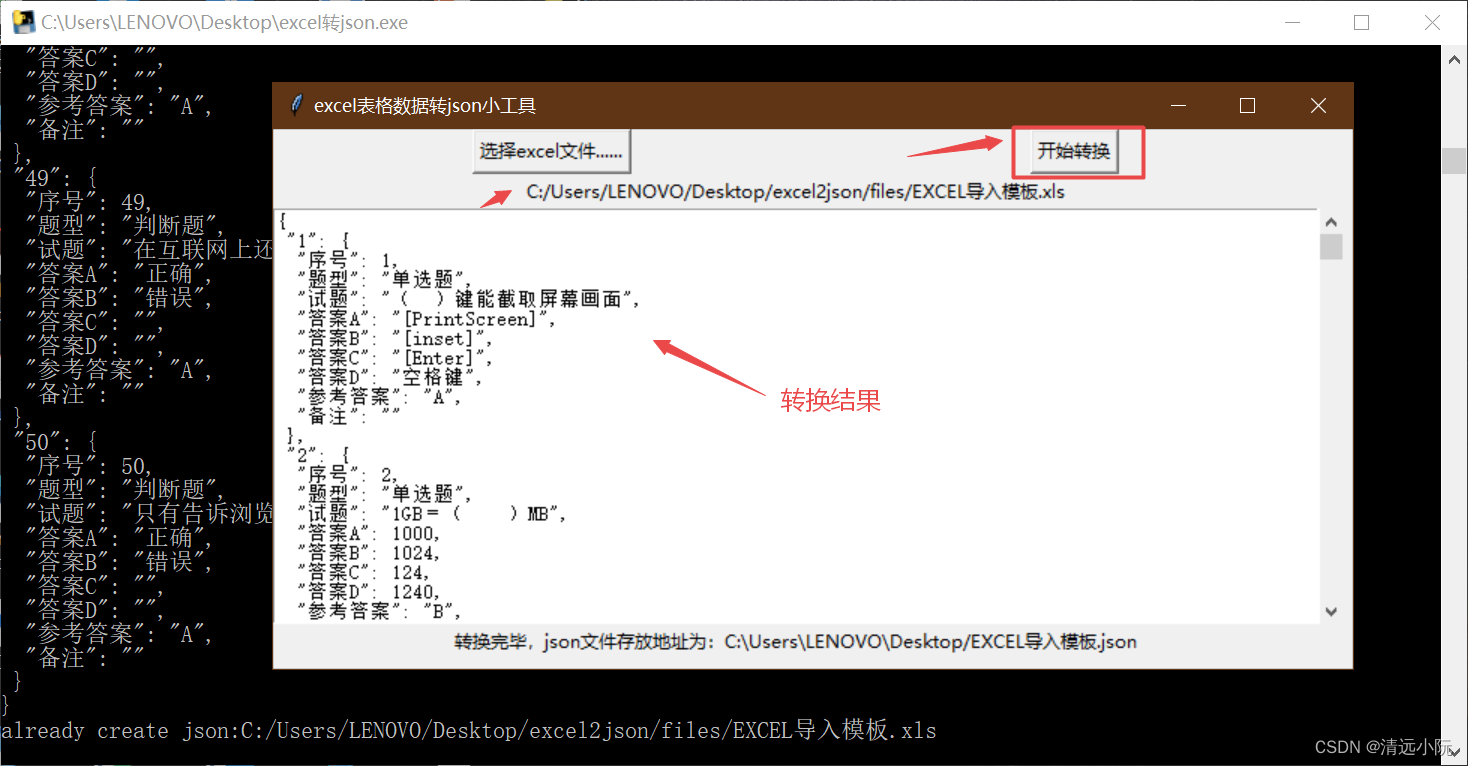
2. Source code analysis
(1) Gadget Graphical Interface Design
The widget interface needs to include an excel file selection click button, a conversion result status display bar, and a conversion result text display area with a slider, as shown in the following figure for the design interface.
Here , Tkinter is used to make the interface, and the .gird layout is used. The code is as follows:
from tkinter import Tk, Label, Button, StringVar, filedialog, Text, Scrollbar
# 显示文件路径组件,_label_filepath_text为文件路径
_label_filepath_text = None
_text = None
_label_state_text = None
def open_window():
# 创建窗口
root = Tk()
# 设置窗口的标题
root.title("excel表格数据转json小工具")
root.geometry("720x360")
_button = Button(root, text="选择excel文件......", command=open_file)
_button.grid(row=1, column=0)
# 创建label可变文本,用于动态更新选中的文件路径
global _label_filepath_text,_text
_label_filepath_text = StringVar()
_label_filepath = Label(root, textvariable=_label_filepath_text)
_label_filepath.grid(row=2, column=0,columnspan=2)
#创建滑块,并绑定文本框
_yscrollbar = Scrollbar(root)
_yscrollbar.grid(row=3,column=3,sticky="NS")
_text = Text(root,height=21,width=99)
_text.grid(row=3,column=0,columnspan=2)
_yscrollbar.config(command=_text.yview)
_text.config(yscrollcommand=_yscrollbar.set)
# 创新开始、停止按钮
_button_start = Button(root, text="开始转换", command=start_transform_file)
_button_start.grid(row=1, column=1)
# 创建label可变文本,用于动态更新发送文件状态
global _label_state_text
_label_state_text = StringVar()
_label_state_text.set("待转换")
_label_state = Label(root, textvariable=_label_state_text)
_label_state.grid(row=4, column=0,columnspan=2)
# 显示窗口
root.mainloop()
(2) Use the xlrd2 library to read excel files
Use the xlrd2 library to read the excel file row by row, read the table header, column header, and table data. The main thing here is that the latest version of xlrd does not support xlsx , and xlrd2 needs to be installed .
import os
import json
import xlrd2
fileTypeArray = [".xlsx",".xls"]
def readAllExecl():
currentPath = os.getcwd()+"/files"
for dir in [x for x in os.listdir(currentPath)]:
localPath = os.path.join(currentPath, dir)
if os.path.isfile(localPath):
filesp = os.path.splitext(localPath)
for k in fileTypeArray:
if filesp[1] == k:
filename = os.path.basename(localPath)
readExecl(localPath,filename.split('.')[0])
def readExecl(path,name):
workbook = xlrd2.open_workbook(path)
sheet2_name = workbook.sheet_names()[0]
sheet=workbook.sheet_by_name(sheet2_name) # sheet索引从0开始
# sheet的名称,行数,列数
adict = {}
for i in range(1,sheet.nrows):
data = {}
for j in range(0,sheet.ncols):
value = TransformationType(sheet.cell_value(i,j))
if isinstance(value , str):
if isJsonString(value):
data[TransformationType(sheet.cell_value(0,j))] = eval(value)
else:
data[TransformationType(sheet.cell_value(0,j))] = value
else:
data[TransformationType(sheet.cell_value(0,j))] = value
adict[TransformationType(sheet.cell_value(i,0))]= data
data = json.dumps(adict,indent=1,ensure_ascii=False)
_json_save_path = os.getcwd() + "/" + name + '.json'
f=open(_json_save_path,'w')
f.write(data)
f.close()
print("already create json:" + path)
return data,_json_save_path
def isJsonString(str):
try:
eval(str)
except Exception as e :
return False
return True
def TransformationType(var):
if isinstance(var ,float) : #type(var) == 'float':
str1 = int(var)
elif isinstance(var, str): #type(var) == 'unicode':
str1 = var
else:
raise Exception("type is not deal")
str1 = var
return str1
(3) Python dictionary format to json format
Store the read excel data into a dictionary.
adict = {}
for i in range(1,sheet.nrows):
data = {}
for j in range(0,sheet.ncols):
value = TransformationType(sheet.cell_value(i,j))
if isinstance(value , str):
if isJsonString(value):
data[TransformationType(sheet.cell_value(0,j))] = eval(value)
else:
data[TransformationType(sheet.cell_value(0,j))] = value
else:
data[TransformationType(sheet.cell_value(0,j))] = value
adict[TransformationType(sheet.cell_value(i,0))]= data
Convert dictionary data into json format. It should be noted here that the ensure_ascii=False parameter needs to be added, otherwise garbled characters will appear when displaying strings .
data = json.dumps(adict,indent=1,ensure_ascii=False)
(4) Save the json format file and display the result on the interface
Display the converted json text on the program interface, the text can be copied and edited.
# 点击,转换文件
def start_transform_file():
global _label_filepath_text,_text,_label_state_text
_file_path = _label_filepath_text.get()
_file_type_list = [".xlsx", ".xls"]
if _file_path is not None:
if os.path.isfile(_file_path):
filesp = os.path.splitext(_file_path)
for k in _file_type_list:
if filesp[1] == k:
filename = os.path.basename(_file_path)
_json_data = excel2json.readExecl(_file_path, filename.split('.')[0])
_text.insert("end",_json_data[0])
_label_state_text.set("转换完毕,json文件存放地址为:"+_json_data[1])
3. Download address
(1) excel to json gadget.exe, executable file download address
https://download.csdn.net/download/qq616491978/87097155
(2) The source code download address of the excel to json widget