Hardworking programmers will type code in their dreams,
The so-called person is lying on the bed, the technology naturally rises,
This issue will take you into dreamlike programming,
The content is abstract, please have your pillow ready.
content:
1. The concept and structure of binary tree
2. Implementation of binary tree chain structure
1. The concept and structure of binary tree
①Concept : A binary tree is a finite set of nodes , which is either empty or consists of a root node plus two binary trees called left subtree and right subtree .
②Characteristics of binary tree :
- Each node has at most two subtrees , that is, there are no nodes with degree greater than 2 in a binary tree. (degrees up to 2)
- The subtrees of a binary tree are divided into left and right, and the order of the subtrees cannot be reversed.
③ Real binary tree :
When an ordinary person sees such a tree, he may think: a good standard tree
When a programmer sees such a tree, he may think: It is like a binary tree in a data structure, and it is still full of binary trees.
④ Binary tree in data structure :
Note: A binary tree has at most two degrees
⑤ Special binary tree:
- Full binary tree: A binary tree, if the number of nodes in each layer reaches the maximum value, the binary tree is a full binary tree. That is, if a binary tree has K levels and the total number of nodes is (2^k) -1 , then it is a full binary tree.
- Complete binary tree : A complete binary tree is a highly efficient data structure, and a complete binary tree is derived from a full binary tree. For a binary tree of depth K with n nodes, it is called a complete binary tree if and only if each node of the tree corresponds one-to-one with nodes numbered from 1 to n in the full binary tree of depth K. Note that a full binary tree is a special kind of complete binary tree.
⑥ Storage structure of binary tree: Binary tree can generally be stored in two structures, a sequential structure and a chain structure .
⑦ The properties of the binary tree:
- If the level of the root node is specified as 1, then there are at most 2^(i-1) nodes on the i-th level of a non-empty binary tree.
- If the number of levels of the root node is specified to be 1, the maximum number of nodes of a binary tree with a depth of h is 2^h-1.
- For any binary tree, if the number of leaf nodes of degree 0 is n0, and the number of branch nodes of degree 2 is n2, then n0=n2+1
- If the level of the root node is specified as 1, the depth of a full binary tree with n nodes, h=log₂n+1
⑧ Exercise questions
2. Implementation of binary tree chain structure
① Traversal of binary tree chain structure:
The so-called traversal (traversal) refers to follow a certain search route, and sequentially visit each node in the tree once and only once. The operations performed by the access node depend on the specific application problem. Traversal is one of the most important operations on a binary tree, and it is the basis for other operations on a binary tree.
Preorder/midorder/postorder recursive structure traversal: Named according to the location where the access node operation occurs
- Preorder (root first): first visit the root node, then visit the left subtree, and finally visit the right subtree
- Inorder (middle root): first visit the left node, then visit the root node, and finally visit the right subtree
- Post-order (post-root): first visit the left node, then visit the right subtree, and finally visit the root node
First define a structure type:
typedef char BTDataType;
typedef struct BinarytreeNode
{
BTDataType data;
struct BinarytreeNode* left;
struct BinarytreeNode* right;
}BTNode;
prologue:
void Preamble(BTNode* p)//前序
{
if (p == NULL)
{
printf("NULL ");
return;
}
printf("%c ", p->data);
Preamble(p->left);
Preamble(p->right);
}
Intermediate sequence:
void Morder(BTNode* p)//中序
{
if (p == NULL)
{
printf("NULL ");
return;
}
Morder(p->left);
printf("%c ", p->data);
Morder(p->right);
}
Post sequence:
void Porder(BTNode* p)//后序
{
if (p == NULL)
{
printf("NULL ");
return;
}
Porder(p->left);
Porder(p->right);
printf("%c ", p->data);
}
Find the number of nodes in a binary tree:
int treeSize(BTNode* p)//结点个数
{
return p == NULL ? 0 : treeSize(p->left) + treeSize(p->right)+1;
}
Find the number of leaf nodes:
int treeLeafSize(BTNode* p)//叶子结点个数
{
if (p == NULL)
{
return 0;
}
if (p->left == NULL&&p->right == NULL)
{
return 1;
}
return treeLeafSize(p->left) + treeLeafSize(p->right);
}
That's it for this issue. . .
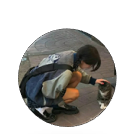