For those who need more related information, please see the profile on the homepage!
Injecting through configuration is often too troublesome. In actual work, you generally don't take troublesome methods, because time is money and time determines whether you work overtime. Is there an easier way to inject? Of course there is!
How Spring injects beans
- Annotation injection
Case practice
Annotation injection
For bean injection, in addition to using xml configuration, the configuration of annotations simplifies the speed of development and makes the program look more concise. For the interpretation of annotations, Spring has a special interpreter for annotations, which parses the defined annotations, realizes the injection of corresponding bean objects, and realizes the reflection technology.
1.加入spring-aop jar包spring-aop-4.3.2.RELEASE.jar
2. Xml configuration: add context namespace and xsd address
3. Add context:annotation-config/configuration
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:annotation-config/>
<bean id="userDao" class="com.xxx.demo.UserDao"></bean>
<bean id="userService" class="com.xxx.demo.UserService"></bean>
</beans>
Common annotation types for bean injection
@Autowired attribute field or set method
@Resource attribute field or set method
Difference: @Autowired matches by bean type by default and can be modified. Match by name and use with @Qualifier. @Resource is assembled by name by default. The name can be specified by the name attribute. If the name attribute is not specified, when the annotation is written on the field, By default, the field name is used for matching injection. If the annotation is written on the setter method, the attribute name is used for assembly by default. When no bean matching the name is found, the assembly is performed according to the type. But it should be noted that once the name attribute is specified, it will only be assembled according to the name.
The @Resource annotation is recommended to belong to J2EE, which reduces the coupling with spring.
Expand
IoC collection type attribute injection
list collection injection
<bean id="listDI" class="com.xxx.demo.ListDI">
<property name="list">
<list>
<value>河南烩面</value>
<value>南方臊子面</value>
<value>油泼面</value>
<value>方便面</value>
</list>
</property>
</bean>
set collection injection
<bean id="listDI" class="com.xxx.demo.ListDI">
<property name="set">
<set>
<value>快乐小馒头</value>
<value>北方大馒头</value>
<value>天津麻花</value>
<value>新疆大饼</value>
</set>
</property>
</bean>
Map type attribute injection
<bean id="userServiceImpl"class="com.xxx.demo.ListDI">
<property name="map">
<map>
<entry>
<key><value>河南</value></key>
<value>云台山风景</value>
</entry>
<entry>
<key><value>上海</value></key>
<value>宝塔</value>
</entry>
<entry>
<key><value>北京</value></key>
<value>紫禁城</value>
</entry>
</map>
</property>
</bean>
for(Map.Entry<String,Object> entry:map.entrySet()){
System.out.println("key:"+entry.getKey()+":value"+entry.getValue());
}
properties injection
<bean id="userServiceImpl" class="com.xxx.demo.ListDI">
<property name="prop">
<props>
<prop key="北京">长城</prop>
<prop key="上海">东方明珠</prop>
<prop key="西安">兵马俑</prop>
</props>
</property>
</bean>
public void printProperties(){
Set<Map.Entry<Object,Object>> set=properties.entrySet();
Iterator<Map.Entry<Object,Object>> iterator=set.iterator;
while(iterator.hasNext()){
Map.Entry<Object,Object> entry=iterator.next();
System.out.println(entry.getKey()+"...."+entry.getValue())
}
}
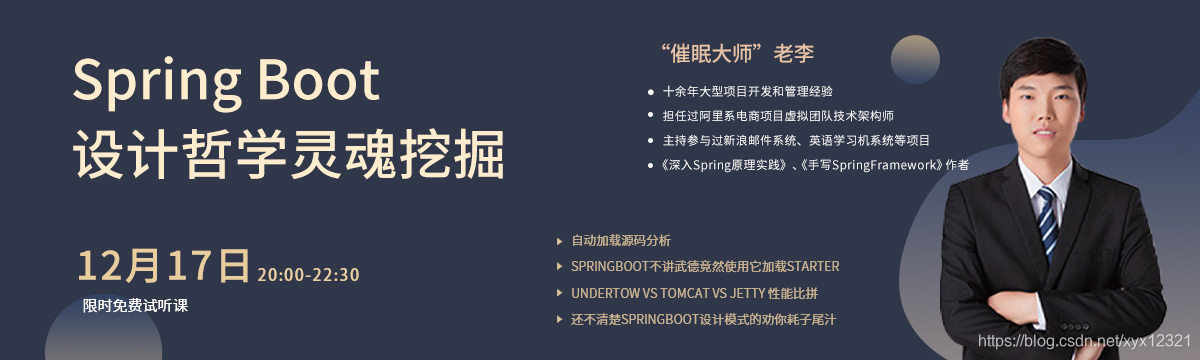