table of Contents
5. Specific requirements and recommended implementation steps
Six, matters needing attention
Document description:
1. Language and environment
- Implementation language: Java.
- Environmental requirements: MyEclipse, JDK 1.6 or above, MySQL.
2. Technical requirements
The system uses SWING technology with JDBC to use JAVA programming language to complete desktop application development.
3. Functional requirements
An e-commerce company needs to develop a user order management system to facilitate customer service to view user order information. The system is required to realize the following functions:
1. After starting the program, enter the main interface and display all order information, the effect is shown in Figure 1:
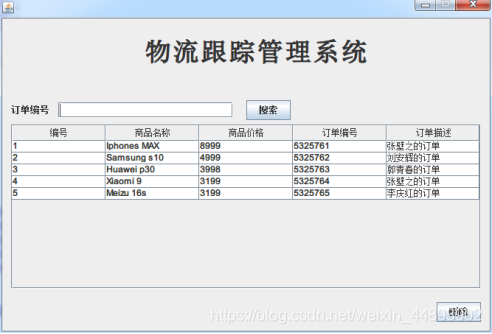
2. Enter the correct order number in the order number text box to display the order information, as shown in Figure 2:
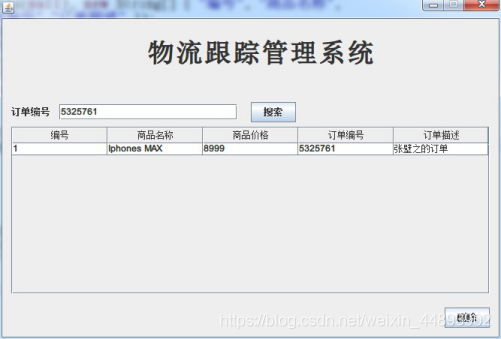
3. After selecting an order, click the delete button to prompt whether you really want to delete it, and delete the data after confirming. As shown in Figure 3:
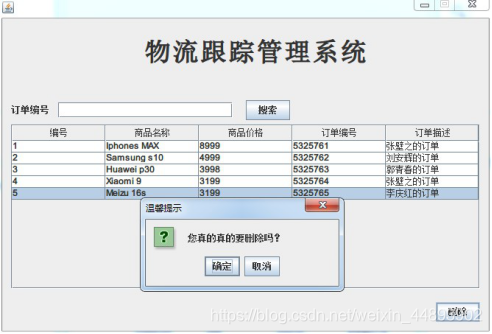
4. After the deletion is successful, the deleted order information will be displayed. As shown in Figure 4:
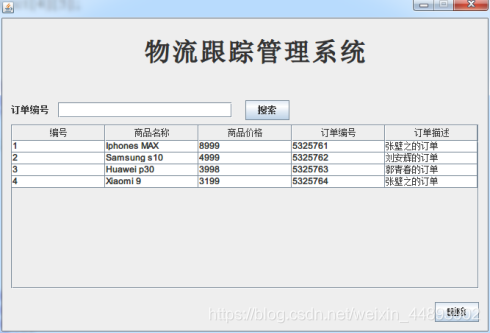
Four, database design
The database is named OrderDb, and the table structure is as follows.
Table 1 Order table (tb_order)
Column name |
meaning |
type of data |
Constraint (description) |
id |
Numbering |
int |
Primary key, automatic growth |
name |
product name |
varchar(20) |
non empty |
price |
price |
decimal(5,2) |
non empty |
orderID |
Owned order number |
varchar(20) |
non empty |
descinfo |
description |
varchar(100) |
|
5. Specific requirements and recommended implementation steps
- Create database and table
- Create an order entity class
- Create the BaseDAO class to complete the database connection and close
- Create OrderDAO to complete the query and delete function of the order
- Create MainFrame class and add corresponding components to complete the interface design
- Add JTable to MainFrame to complete data display.
- Add Jbutton in MainFrame to complete data deletion
- Add a text box in MainFrame to complete a single data search
Six, matters needing attention
Topic: Logistics Tracking Management System |
||
The scoring criteria for this program are as follows: |
||
10 |
Database and table |
|
|
6 points for correct creation of database and table, 4 points for test data. Must be submitted in a separate database or script, otherwise the item will not be scored |
|
5 |
Create and write entity classes correctly, including all attributes and methods |
|
10 |
Create and write BaseDAO correctly to complete the database connection (5 points) and close (5 points). |
|
30 |
Create and write OrderDAO correctly to complete data query and delete |
|
|
10 |
List data query |
|
10 |
Single data query |
|
10 |
Data deletion |
40 |
Create and write MainFrame class to complete interface design and function call |
|
|
5 |
The design of each component in Figure 1 |
|
10 |
Data display in Figure 1 |
|
10 |
A single piece of data in Figure 2 and correctly displayed in JTable |
|
5 |
Single data deletion query in Figure 3 |
|
10 |
The data in Figure 4 is deleted and the new data is displayed in the Jtable. |
5 |
Overall programming techniques |
|
|
2 |
Coding Standards |
|
3 |
Perfect annotation |
Total score |
100 points |
Implementation code:
One, the database
-- ----------------------------
-- Table structure for tb_order
-- ----------------------------
DROP TABLE IF EXISTS `tb_order`;
CREATE TABLE `tb_order` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(20) NOT NULL,
`price` decimal(10,2) NOT NULL,
`orderid` varchar(20) DEFAULT NULL,
`descinfo` varchar(100) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of tb_order
-- ----------------------------
INSERT INTO `tb_order` VALUES ('1', 'iphones Max', '8999.00', '5325781', '杨明金的订单');
INSERT INTO `tb_order` VALUES ('2', '小米10', '3655.00', '20201005', '杨杨的订单');
INSERT INTO `tb_order` VALUES ('3', '华为荣耀10 =', '3200.00', '20201102', '小白的订单');
二、Java Swing
com.ynavc.Bean
tb_order.java
package com.ynavc.Base;
public class tb_order {
private int id;
private String name;
private double price;
private int orderID;
private String descinfo;
public tb_order() {
super();
}
public tb_order(int id, String name, double price, int orderID, String descinfo) {
super();
this.id = id;
this.name = name;
this.price = price;
this.orderID = orderID;
this.descinfo = descinfo;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getOrderID() {
return orderID;
}
public void setOrderID(int orderID) {
this.orderID = orderID;
}
public String getDescinfo() {
return descinfo;
}
public void setDescinfo(String descinfo) {
this.descinfo = descinfo;
}
}
com.ynavc.BaseDAO
DbConnection.java
package com.ynavc.BaseDAO;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.JOptionPane;
import com.mysql.jdbc.Statement;
public class DbConnection {
//驱动类的类名
private static final String DRIVERNAME="com.mysql.jdbc.Driver";
//连接数据的URL路径
private static final String URL="jdbc:mysql://127.0.0.1:3306/orderdb";
//数据库登录账号
private static final String USER="root";
//数据库登录密码
private static final String PASSWORD="root123";
//加载驱动
static{
try {
Class.forName(DRIVERNAME);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
//获取数据库连接
public static Connection getConnection() {
try {
return DriverManager.getConnection(URL,USER,PASSWORD);
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
//查询
public static ResultSet query(String sql) {
System.out.println(sql);
//获取连接
Connection connection=getConnection();
PreparedStatement psd;
try {
psd = connection.prepareStatement(sql);
return psd.executeQuery();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null,"执行语句出错\n"+e.toString());
e.printStackTrace();
}
return null;
}
//增、删、改、查
public static int updataInfo(String sql) {
System.out.println(sql);
//获取连接
Connection connection=getConnection();
try {
PreparedStatement psd=connection.prepareStatement(sql);
return psd.executeUpdate();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null,"执行语句出错\n"+e.toString());
e.printStackTrace();
}
return 0;
}
//关闭连接
public static void colse(ResultSet rs,Statement stmt,Connection conn) throws Exception{
try {
if (rs != null){
rs.close();
}
if (stmt != null){
stmt.cancel();
}
if (conn != null) { conn.close(); }
} catch (Exception e) {
e.printStackTrace(); throw new Exception();
}
}
}
com.ynavc.OrderDAO
Update.java
package com.ynavc.OrderDAO;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import com.ynavc.Base.tb_order;
import com.ynavc.BaseDAO.DbConnection;
public class Update {
//查询主页信息
public Object[][] getMainInfo(String id) {
String sql;
if (id.equals("")) {
sql = "select * from tb_order";
}else {
sql = "select * from tb_order WHERE orderid LIKE '"+id+"%';";
}
ResultSet re = DbConnection.query(sql);
ArrayList<tb_order> list = new ArrayList<tb_order>();
try {
while (re.next()) {
tb_order tb = new tb_order();
tb.setId(re.getInt(1));
tb.setName(re.getString(2));
tb.setPrice(re.getDouble(3));
tb.setOrderID(re.getInt(4));
tb.setDescinfo(re.getString(5));
list.add(tb);
}
} catch (SQLException e) {
e.printStackTrace();
}
Object[][] ob = new Object[list.size()][5];
for (int i = 0; i < list.size(); i++) {
ob[i][0] = list.get(i).getId();
ob[i][1] = list.get(i).getName();
ob[i][2] = list.get(i).getPrice();
ob[i][3] = list.get(i).getOrderID();
ob[i][4] = list.get(i).getDescinfo();
}
return ob;
}
//删除数据
public int addData(int id) {
String sql = "DELETE FROM tb_order WHERE id='"+id+"';";
return DbConnection.updataInfo(sql);
}
}
com.ynavc.Vive
MainFrame.java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
import com.ynavc.OrderDAO.Update;
import javax.swing.JButton;
public class MainFrame extends JFrame {
private JTextField orderid;
Update update = new Update();
Object[] header = {"编号","商品名称","商品价格","订单编号","订单描述"};
Object[][] data = update.getMainInfo("");
DefaultTableModel df = new DefaultTableModel(data, header);
public MainFrame() {
super("物流a跟踪管理系统");
this.setBounds(0, 0, 700, 500);
getContentPane().setLayout(null);
this.setLocationRelativeTo(null);
this.setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JLabel title = new JLabel("物流跟踪管理系统");
title.setFont(new Font("宋体", Font.BOLD, 40));
title.setBounds(182, 13, 336, 50);
getContentPane().add(title);
JLabel orderid_text = new JLabel("订单编号");
orderid_text.setBounds(14, 70, 72, 18);
getContentPane().add(orderid_text);
orderid = new JTextField();
orderid.setBounds(80, 67, 179, 24);
getContentPane().add(orderid);
orderid.setColumns(10);
JButton seach = new JButton("搜索");
seach.setBounds(273, 66, 90, 27);
getContentPane().add(seach);
seach.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (orderid.equals("")) {
JOptionPane.showMessageDialog(null, "请输入要查询的订单编号!");
} else {
String id = orderid.getText();
data = update.getMainInfo(id);
df.setDataVector(data, header);
}
}
});
JTable jTable = new JTable(df);
JScrollPane scrollPane = new JScrollPane(jTable);
scrollPane.setBounds(14, 104, 666, 302);
getContentPane().add(scrollPane);
JButton delete = new JButton("删除");
delete.setBounds(590, 425, 90, 27);
getContentPane().add(delete);
delete.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (jTable.getSelectedColumn()<0) {
JOptionPane.showMessageDialog(null, "请选择要删除的数据!");
} else {
int num = JOptionPane.showConfirmDialog(null, "您真的真的要删除吗?","温馨提示", 0,1);
int id = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 0).toString());
if(num == JOptionPane.OK_OPTION){
int result = update.addData(id);
if (result>0) {
JOptionPane.showMessageDialog(null, "删除成功!");
orderid.setText("");
} else {
JOptionPane.showMessageDialog(null, "删除失败!");
}
}
}
}
});
}
public static void main(String[] args) {
new MainFrame().setVisible(true);
}
}
com.ynavc.Test
Test.java
package com.ynavc.Test;
import com.ynavc.Vive.MainFrame;
public class Test {
public static void main(String[] args) {
new MainFrame().setVisible(true);
}
}